Mastering Git: A Comprehensive Guide to Rename Branch with Ease
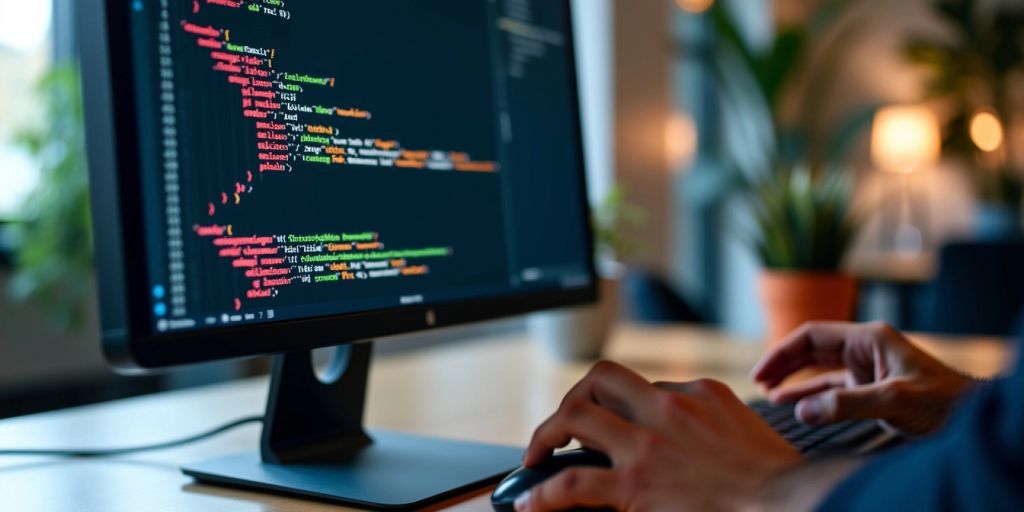
In this guide, you’ll learn how to rename branches in Git easily. Whether you’re working on a new feature or fixing bugs, knowing how to manage your branches is essential. We’ll break down the process step-by-step and provide tips to help you avoid common mistakes. By the end, you’ll feel confident in renaming branches and keeping your project organized.
Key Takeaways
- Renaming branches helps keep your project organized and clear.
- Use the command ‘git branch -m old-name new-name’ to rename a branch easily.
- Always check if a branch has been merged before deleting it.
- Consistent naming conventions make it easier for teams to collaborate.
- Automating branch management can save time and reduce errors.
Understanding Git Branches
What is a Git Branch?
A Git branch is like a separate path in your project. It allows you to work on different features or fixes without messing up the main code. Each branch points to a specific point in your project’s history, making it easy to switch back and forth.
Why Use Branches in Git?
Using branches in Git has many advantages:
- Isolation: You can work on new features without affecting the main code.
- Collaboration: Multiple people can work on different branches at the same time.
- Organization: Keep your tasks organized by dedicating branches to specific jobs.
- Version Control: Try out new ideas without the risk of breaking anything.
Creating a New Branch
Creating a new branch is simple. Here’s how you can do it:
- Check out the branch you want to base your new branch on (usually the main branch).
git checkout main
- Create a new branch using the command:
git branch [branch-name]
For example:
git branch feature-new-login
Branches are essential for managing different tasks in your project. They help keep your work organized and prevent conflicts. Git branches are commonly used when there’s a new feature, bug fix, or anything else in your code you might want to track and compare to previous versions.
The Importance of Renaming Branches
When to Rename a Branch
Renaming a branch is important when the original name no longer reflects the purpose of the branch. Here are some common situations:
- The project scope changes: If the focus of the branch shifts, a new name can clarify its purpose.
- Improving clarity: A more descriptive name can help team members understand the branch’s function better.
- Avoiding confusion: If multiple branches have similar names, renaming can help distinguish them.
Benefits of Renaming Branches
Renaming branches can lead to several advantages:
- Enhanced communication: Clear names help team members understand the work being done.
- Better organization: A well-named branch can make it easier to manage and track changes.
- Reduced errors: Clear naming reduces the chances of mistakes when merging or checking out branches.
Common Scenarios for Renaming
Here are some typical scenarios where renaming branches is beneficial:
- Feature updates: When a feature evolves, the branch name should reflect its current state.
- Bug fixes: If a branch initially created for a bug fix expands to include more changes, renaming is essential.
- Project restructuring: As projects grow, branches may need to be renamed to fit new organizational structures.
Renaming branches is a simple yet effective way to keep your Git repository organized and understandable. Effective Git management ensures that everyone on the team is on the same page, reducing confusion and improving collaboration.
Step-by-Step Guide to Renaming a Branch
Using Git Branch -m Command
Renaming a branch in Git is straightforward. You can use the [git branch -m](https://confluence.atlassian.com/display/BAMBOO/Bamboo+Best+Practice+-+Branching+and+DVCS)
command to change the name of an existing branch. Here’s how to do it:
- Open your terminal.
- Navigate to your repository using
cd path/to/your/repo
. - Run the command:
git branch -m old-branch-name new-branch-name
This will rename the branch from old-branch-name to new-branch-name.
Renaming the Current Branch
If you want to rename the branch you are currently on, you can simply use:
git branch -m new-branch-name
This command will rename the current branch without needing to specify the old name. Make sure to choose a name that reflects the purpose of the branch.
Handling Conflicts During Rename
Sometimes, renaming a branch can lead to conflicts, especially if the new name already exists. Here are steps to handle this:
- Check if the new branch name already exists using
git branch
. - If it does, you can either delete the existing branch or choose a different name.
- To delete an existing branch, use:
git branch -d existing-branch-name
- After resolving conflicts, you can proceed with the rename command again.
Tip: Always ensure that your changes are committed before renaming branches to avoid losing any work.
Best Practices for Branch Naming
Choosing Descriptive Names
When creating a branch, it’s important to use clear and meaningful names. This helps everyone understand the purpose of the branch at a glance. Here are some tips:
- Use lowercase letters.
- Replace spaces with hyphens or underscores.
- Include relevant identifiers if applicable (e.g., issue numbers).
Avoiding Common Pitfalls
To keep your branch names effective, avoid these common mistakes:
- Using vague names like "temp" or "test".
- Making names too long or complicated.
- Forgetting to follow your team’s naming conventions.
Consistency in Naming Conventions
Maintaining a consistent naming style is crucial. This can include:
- Using a specific format for feature branches (e.g.,
feature/branch-name
). - Keeping a standard for bug fixes (e.g.,
bugfix/issue-number
). - Regularly reviewing and updating naming guidelines as needed.
Keeping branch names organized and clear can significantly improve team collaboration and project management.
By following these best practices, you can ensure that your branch names are not only functional but also enhance the overall workflow of your project. Remember, this guide will cover industry best practices for naming git branches to help streamline your development process.
Managing Branches After Renaming
Updating References
After renaming a branch, it’s crucial to update any references to the old branch name. This ensures that your team and any automated systems are aware of the change. Here are some steps to follow:
- Check your local repository for any references to the old branch name.
- Update any scripts or tools that might use the old name.
- Notify your team about the change to avoid confusion.
Communicating Changes to Team
Effective communication is key when renaming branches. Here are some tips:
- Send a message to your team via email or chat.
- Update project documentation to reflect the new branch name.
- Hold a brief meeting if necessary to discuss the changes.
Ensuring Smooth Workflow
To maintain a smooth workflow after renaming a branch, consider the following:
- Monitor for issues that may arise from the rename.
- Encourage team members to pull the latest changes regularly.
- Use the
git branch -m
command to rename branches easily. For example, if you need to rename a branch, use the command:git branch -m [old-branch-name] [new-branch-name]
.
Remember: Keeping everyone informed helps prevent confusion and ensures a seamless transition after renaming branches.
Advanced Branch Management Techniques
Using Git Flow
Git Flow is a popular branching model that helps manage features, releases, and hotfixes in a structured way. This method simplifies collaboration by defining clear roles for different branches. Here’s how it works:
- Master Branch: This is the main branch that holds the production-ready code.
- Develop Branch: This branch is where all the features are integrated before they are ready for production.
- Feature Branches: Each new feature is developed in its own branch, which is created from the develop branch.
- Release Branches: When preparing for a new release, a release branch is created from the develop branch to finalize the release.
- Hotfix Branches: These branches are used to quickly address issues in the master branch.
Trunk-Based Development
In Trunk-Based Development, all developers work on a single branch, often called the trunk. This approach encourages frequent integration and reduces the complexity of merging. Here are some key points:
- Frequent Commits: Developers commit changes to the trunk multiple times a day.
- Short-Lived Feature Branches: If branches are used, they are short-lived and merged back into the trunk quickly.
- Continuous Integration: Automated tests run on the trunk to ensure stability.
Feature Branching
Feature branching allows developers to work on new features in isolation. This method is beneficial for larger teams. Here’s how to implement it:
- Create a new branch for each feature.
- Develop the feature independently.
- Merge back into the main branch once the feature is complete and tested.
Using these advanced techniques can greatly enhance your workflow and collaboration in Git. They help keep your project organized and make it easier to manage changes.
Summary Table of Branching Techniques
Technique | Description | Benefits |
---|---|---|
Git Flow | Structured branching model for features and releases | Clear roles and responsibilities |
Trunk-Based Development | Single branch for all development | Reduces merge complexity |
Feature Branching | Isolated development for new features | Easier testing and integration |
Tools and Commands for Branch Management
Managing branches in Git is essential for keeping your project organized. Here are some key commands and tools you can use:
Listing All Branches
To see all branches in your repository, use the following command:
git branch
This will show you both local and remote branches. To view remote branches as well, use:
git branch -a
Deleting Unused Branches
When you’re done with a branch, it’s a good idea to delete it to keep your repository clean. Use:
git branch -d [branch-name]
If the branch hasn’t been merged yet, you can force delete it with:
git branch -D [branch-name]
Merging and Rebasing Branches
Merging allows you to combine changes from one branch into another. To merge a branch, first switch to the main branch:
git checkout main
Then, merge the desired branch:
git merge [branch-name]
Important Commands Summary
Command | Description |
---|---|
git branch |
List all branches |
git branch -d [name] |
Delete a branch |
git merge [name] |
Merge a branch into the current branch |
Remember: Keeping your branches organized helps avoid confusion and conflicts in your project.
By mastering these commands, you can effectively manage your branches and maintain a smooth workflow. Using the right tools can greatly enhance your productivity in Git!
Troubleshooting Common Issues
Resolving Merge Conflicts
Merge conflicts can happen when two branches have changes in the same part of a file. Here’s how to handle them:
- Identify the conflict: Git will mark the conflicting areas in the file.
- Edit the file: Choose which changes to keep or combine them.
- Mark as resolved: After fixing, use
git add <file>
to mark the conflict as resolved.
Recovering Deleted Branches
If you accidentally delete a branch, don’t worry! You can recover it:
- Use
git reflog
to find the commit hash of the deleted branch. - Run
git checkout -b <branch-name> <commit-hash>
to restore it. - Remember: Regularly back up your branches to avoid loss.
Dealing with Unmerged Branches
Sometimes, branches may not merge properly. Here’s what to do:
- Check for unmerged changes using
git status
. - Use
git merge <branch-name>
to attempt merging again. - If issues persist, consider using
git cherry-pick
to apply specific commits.
Tip: Utilizing scripts to automate the renaming and initialization process helps mitigate these issues, ensuring a successful migration.
Issue Type | Solution |
---|---|
Merge Conflicts | Edit and resolve the conflicting files. |
Deleted Branch | Use git reflog to recover it. |
Unmerged Changes | Check status and attempt to merge again. |
Collaborative Workflows with Renamed Branches
Coordinating with Team Members
When a branch is renamed, it’s crucial for all team members to stay in sync. Here are some steps to ensure smooth collaboration:
- Notify your team about the branch name change.
- Update any documentation that references the old branch name.
- Encourage team members to rename their local copies of the branch using the
git branch -m <old_branch_name> <new_branch_name>
command.
Pull Requests and Code Reviews
Renaming branches can affect ongoing pull requests and code reviews. Here’s how to manage this:
- Check existing pull requests to see if they reference the old branch name.
- Update pull requests to point to the new branch name.
- Communicate changes to reviewers to avoid confusion.
Maintaining Branch History
Keeping track of branch history is essential for understanding project evolution. Consider the following:
- Use descriptive commit messages that reflect the changes made in the renamed branch.
- Document the reason for renaming the branch in the commit history.
- Ensure that all team members are aware of the branch’s purpose and history.
Effective communication is key to ensuring that everyone is on the same page after a branch is renamed. This helps maintain a smooth workflow and prevents misunderstandings.
Automating Branch Renaming
Using Scripts for Automation
Automating the renaming of branches can save time and reduce errors. Here are some ways to do it:
- Create a script that uses the
git branch -m
command to rename branches in bulk. - Set up hooks that trigger renaming when certain conditions are met, like a push to a specific branch.
- Use configuration settings to manage branch names automatically.
Integrating with CI/CD Pipelines
Integrating branch renaming into your CI/CD pipelines can streamline your workflow. Consider the following:
- Trigger renaming when a pull request is merged.
- Automatically update branch names based on the issue tracker.
- Notify team members of changes through automated messages.
Benefits of Automation in Large Projects
Automating branch renaming can lead to several advantages:
- Consistency in naming conventions across the project.
- Reduced manual errors when renaming branches.
- Improved collaboration among team members.
Automating tasks like branch renaming can significantly enhance team productivity and maintain a clean project structure.
In summary, using scripts and integrating with CI/CD pipelines can make branch renaming easier and more efficient. Consider automating your workflow to keep your project organized and up-to-date!
Case Studies and Real-World Examples
Successful Branch Renaming Stories
In many projects, renaming branches has led to improved clarity and organization. Here are a few examples:
- Project Phoenix: The team renamed their main branch from
master
tomain
, aligning with modern practices. - E-commerce Platform: A branch named
feature/payment
was renamed tofeature/secure-payment
, making its purpose clearer. - Open Source Library: The branch
dev
was renamed todevelopment
, which helped new contributors understand its role better.
Lessons Learned from Failures
Not all renaming efforts go smoothly. Here are some lessons learned:
- Communicate Changes: Teams that failed to inform members about branch renaming faced confusion.
- Update Documentation: Neglecting to update project documentation led to misunderstandings.
- Test Before Renaming: Some teams experienced issues because they renamed branches without testing the impact on their CI/CD pipelines.
Best Practices from Industry Leaders
Industry leaders have shared valuable insights on branch renaming:
- Use Clear Naming Conventions: Names should reflect the purpose of the branch.
- Involve the Team: Get input from all team members before making changes.
- Document Changes: Keep a record of all renaming activities to avoid confusion in the future.
Renaming branches can significantly enhance project clarity and collaboration.
Project Name | Old Branch Name | New Branch Name | Reason for Change |
---|---|---|---|
Project Phoenix | master | main | Align with modern practices |
E-commerce Platform | feature/payment | feature/secure-payment | Improve clarity |
Open Source Library | dev | development | Enhance understanding for new contributors |
Explore how our students have transformed their coding skills and landed amazing jobs! Check out our real-life success stories and see how you can start your journey today. Visit our website to learn more and kickstart your coding adventure!
Conclusion
Understanding how to rename branches in Git is a key skill for anyone working with version control. This guide has shown you how to easily change branch names, which can help keep your project organized and clear. Whether you’re fixing a mistake or just want a better name, renaming branches is simple with the right commands. Remember, using clear names for your branches makes it easier for everyone on your team to understand what each branch is for. By mastering these techniques, you can improve your workflow and make collaboration smoother. Start using these tips today to enhance your Git skills!
Frequently Asked Questions
What is a Git branch?
A Git branch is like a separate path in your project. It lets you work on new features or fixes without changing the main code.
Why should I rename a branch?
Renaming a branch helps keep your project organized. If the purpose of a branch changes, a new name can make it clearer.
How do I rename a branch in Git?
You can rename a branch using the command ‘git branch -m old-name new-name’. This updates the branch name.
What happens if I delete a branch that hasn’t been merged?
If you delete a branch that hasn’t been merged, you might lose the changes in that branch unless you force delete it.
Can I rename the branch I’m currently on?
Yes, you can rename the branch you are currently working on without needing to switch to another branch.
What should I consider when naming branches?
Choose clear and descriptive names that reflect what the branch is for, like ‘feature-login’ or ‘bugfix-header’.
How can I check if a branch was merged?
You can check if a branch was merged by using ‘git branch –contains’. This shows if the branch is part of another.
What are the benefits of using branches in Git?
Branches help you work on different tasks at the same time, keep your code organized, and reduce conflicts with others.