Mastering TypeScript: Conditionally Add Object to Array with Ease
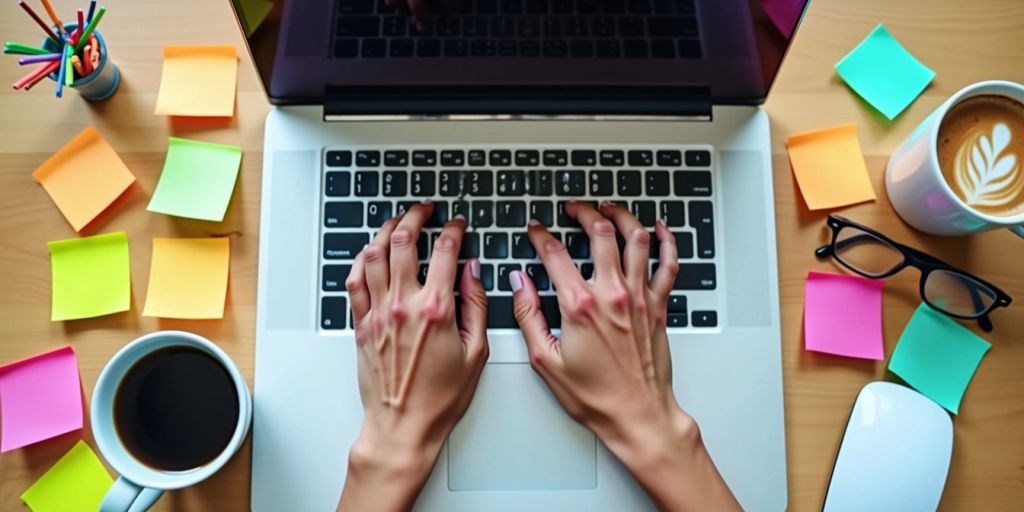
In this article, we will explore how to easily add objects to arrays in TypeScript based on certain conditions. We’ll break down the different ways to use conditional logic, so you can enhance your programming skills and write cleaner code. Whether you are a beginner or looking to sharpen your TypeScript knowledge, this guide will provide helpful insights and practical examples.
Key Takeaways
- Conditional logic helps in making decisions in your code.
- Using the spread operator can simplify adding elements to arrays.
- Ternary operators are a neat way to handle conditions in a single line.
- Type guards ensure that the right types are used in your code.
- Keeping your conditions simple makes your code easier to read and maintain.
Understanding Conditional Logic in TypeScript
In TypeScript, conditional logic is essential for making decisions in your code. It allows you to execute different actions based on certain conditions. Here’s a breakdown of the key concepts:
Basic Conditional Statements
- Conditional statements help you control the flow of your program.
- Common types include
if
,else if
, andelse
. - Example:
if (condition) { // do something } else { // do something else }
Using Ternary Operators
- Ternary operators provide a shorthand way to write simple conditional statements.
- The syntax is
condition ? expr1 : expr2
. - Example:
const result = condition ? "Yes" : "No";
Short-Circuit Evaluation
- Short-circuit evaluation is a technique where the second operand is evaluated only if necessary.
- This is commonly used with logical operators like
&&
and||
. - Example:
const value = condition && "This will only run if condition is true";
Conditional logic is a powerful tool that helps you write flexible and dynamic code. Understanding how to use it effectively can greatly enhance your programming skills.
In TypeScript, conditional types enable types to be defined based on a condition. Using the syntax t extends u ? x : y
, the type evaluates to x
if t
extends u
. This feature allows for more robust type definitions and enhances type safety in your applications.
Adding Objects to Arrays Conditionally
In TypeScript, you can easily add objects to arrays based on certain conditions. This allows for more dynamic and flexible code. Here are some common methods to achieve this:
Using If Statements
Using if statements is one of the most straightforward ways to add an object to an array. Here’s how you can do it:
- Create an empty array.
- Check a condition using an if statement.
- If the condition is true, push the object into the array.
Example:
let toys: string[] = [];
let newToy = 'Teddy Bear';
if (newToy) {
toys.push(newToy);
}
console.log(toys); // Output: ['Teddy Bear']
Leveraging Ternary Operators
Ternary operators can also be used for adding objects conditionally. This method is more concise:
let toys: string[] = [];
let newToy = 'Action Figure';
newToy ? toys.push(newToy) : null;
console.log(toys); // Output: ['Action Figure']
Applying Logical AND (&&)
Another way to conditionally add an object is by using the logical AND operator. This is a neat trick:
let toys: string[] = [];
let newToy = 'Doll';
newToy && toys.push(newToy);
console.log(toys); // Output: ['Doll']
Remember: Using these methods can help keep your code clean and efficient.
By mastering these techniques, you can effectively manage your arrays and ensure that only the right objects are added based on your conditions. This is especially useful when working with objects and arrays in TypeScript.
Practical Examples of Conditional Array Manipulation
In this section, we will explore various ways to add objects to arrays based on certain conditions. Understanding these methods can help you write cleaner and more efficient code.
Example with If Statements
Using if statements is one of the most straightforward ways to conditionally add elements to an array. Here’s how you can do it:
- Initialize an empty array.
- Check a condition using an if statement.
- Push the object into the array if the condition is true.
let myArray: string[] = [];
let condition = true;
if (condition) {
myArray.push('Item 1');
}
Example with Ternary Operators
You can also use the ternary operator to add elements conditionally. This method is more concise and can be very useful in one-liners. For example:
let myArray = [condition ? 'Item 1' : 'Item 2'];
This example demonstrates using the ternary operator to include elements in an array based on a simple condition.
Example with Logical AND (&&)
Another way to conditionally add elements is by using the logical AND (&&) operator. This method is particularly useful for short conditions:
let myArray: string[] = [];
condition && myArray.push('Item 1');
In this case, ‘Item 1’ will only be added if the condition is true.
Tip: Using these methods can help you keep your code clean and readable. Always choose the method that best fits your situation!
Advanced Techniques for Conditional Array Updates
Using Spread Operator
The spread operator is a powerful tool in TypeScript that allows you to easily add elements to an array based on certain conditions. For example:
const items = [
'apple',
...true ? ['banana'] : [],
...false ? ['cherry'] : [],
];
console.log(items); // Output: ['apple', 'banana']
In this example, if the condition is true, the array will include ‘banana’. If false, it will not add anything.
Combining Multiple Conditions
When you want to add elements based on more than one condition, you can use logical operators. Here’s how:
- Use
&&
for multiple true conditions: - Use
||
for at least one true condition: - Combine both for complex logic:
Handling Complex Objects
When dealing with complex objects, you can still use conditional logic to add them to an array. For instance:
const users = [];
const user = { name: 'John', age: 30 };
const isAdult = user.age >= 18;
if (isAdult) {
users.push(user);
}
console.log(users); // Output: [{ name: 'John', age: 30 }]
Remember: This advanced technique allows you to create more precise types for array operations, ensuring that array lengths fall within specific ranges at compile-time.
By mastering these techniques, you can efficiently manage your arrays in TypeScript, making your code cleaner and more effective.
TypeScript Type Guards and Conditional Types
Introduction to Type Guards
Type guards are mechanisms in TypeScript that allow developers to narrow down the type of a variable within a conditional block. This ensures type safety at runtime and helps prevent errors. Here are some common ways to use type guards:
- Using
typeof
: Check the type of a variable. - Using
instanceof
: Check if an object is an instance of a class. - Custom type guards: Create functions that return a boolean to check types.
Using Type Guards with Arrays
When working with arrays, type guards can help ensure that the elements are of the expected type. For example:
function isStringArray(arr: any[]): arr is string[] {
return arr.every(item => typeof item === 'string');
}
This function checks if all elements in the array are strings, providing a clear way to handle different types safely.
Conditional Types in TypeScript
Conditional types allow you to create types based on conditions. They are defined using the extends
keyword and are denoted by the ?
symbol. Here’s a simple example:
type NonNullable<T> = T extends null | undefined ? never : T;
This type transforms the given type by excluding null
and undefined
. Here’s how it works:
Type Input | Resulting Type |
---|---|
`string | null` |
`number | undefined` |
`null | undefined` |
In this way, conditional types provide powerful tools for creating complex types in TypeScript, allowing for better type safety and clarity in your code.
Optimizing Performance with Conditional Array Operations
When working with arrays in TypeScript, it’s important to ensure that your operations are efficient. Here are some strategies to help you optimize performance:
Minimizing Array Mutations
- Avoid unnecessary changes: Each time you modify an array, it can lead to performance hits. Instead, try to create new arrays when possible.
- Use methods like
map
,filter
, andreduce
to return new arrays without changing the original. - Batch updates: If you need to make multiple changes, consider collecting them and applying them all at once.
Efficient Conditional Checks
- Use simple conditions: Complex conditions can slow down your code. Keep your checks straightforward.
- Short-circuit evaluation: This technique allows you to stop evaluating conditions as soon as the result is known, which can save time.
- Leverage built-in methods like
every
andsome
for quick checks on array elements.
Avoiding Unnecessary Computations
- Cache results: If you perform a calculation multiple times, store the result in a variable to avoid repeating the work.
- Use memoization techniques to remember results of expensive function calls.
- Profile your code: Use tools to identify slow parts of your code and focus on optimizing those areas.
Remember: Optimizing performance is about making your code run faster and more efficiently. Small changes can lead to significant improvements.
By applying these techniques, you can enhance the performance of your TypeScript applications, making them more responsive and efficient. Performance optimization is key to a smooth user experience!
Common Pitfalls and How to Avoid Them
When working with TypeScript, especially when adding objects to arrays, there are some common mistakes that can trip you up. Here are a few pitfalls to watch out for:
Misusing Ternary Operators
- Ternary operators can be handy, but they can also lead to confusion if overused. Make sure to keep your conditions clear and simple.
- Avoid nesting ternary operators, as they can make your code hard to read.
- Always ensure that both outcomes of the ternary operator are of the same type to prevent unexpected behavior.
Overcomplicating Conditions
- Keep your conditions straightforward. If a condition is too complex, consider breaking it down into smaller parts.
- Use helper functions to simplify your logic. This can make your code cleaner and easier to understand.
- Avoid using multiple logical operators in a single condition, as this can lead to mistakes.
Ignoring Type Safety
- Type safety is one of the main benefits of TypeScript. Always define your types clearly to avoid runtime errors.
- Use interfaces or types to ensure that the objects you are adding to arrays match the expected structure.
- Regularly check for type mismatches, especially when dealing with external data sources.
Remember: Keeping your code simple and clear will save you time and headaches in the long run.
By being aware of these common pitfalls, you can write cleaner and more effective TypeScript code. Always strive for clarity and simplicity in your conditions and logic, and you’ll find it easier to manage your arrays and objects effectively.
In summary, avoid misusing ternary operators, keep your conditions simple, and always respect type safety. This will help you add an object to an array in JavaScript without any issues!
Real-World Use Cases
Form Validation
In web development, form validation is crucial to ensure that users provide the correct information. You can conditionally add error messages to an array based on user input. For example:
- Check if the email is valid.
- Verify that the password meets security standards.
- Ensure required fields are filled out.
Dynamic Data Handling
When working with APIs, you often receive data that needs to be processed. You can conditionally add objects to an array based on certain criteria:
- Filter out unnecessary data.
- Add only items that meet specific conditions.
- Update the array as new data comes in.
Conditional Rendering in UI
In user interfaces, you might want to display certain components based on user actions. For instance:
- Show a loading spinner while data is being fetched.
- Display a message if no results are found.
- Render different layouts based on user roles.
In JavaScript, the fundamental way that we group and pass around data is through objects. In TypeScript, we represent those through object types. This makes it easier to manage and manipulate data effectively.
By understanding these use cases, you can leverage TypeScript’s capabilities to create more robust applications.
Testing and Debugging Conditional Array Logic
Writing Unit Tests
When working with conditional logic in TypeScript, writing unit tests is essential. Here are some steps to follow:
- Identify the conditions you want to test.
- Create test cases for each condition.
- Use a testing framework like Jest or Mocha to run your tests.
Debugging Common Issues
Debugging can be tricky, especially when using libraries. Here are some tips:
- Use
console.log
to check values at different points in your code. This can help you see what’s happening inside your functions. - Look for unexpected results in your array manipulations.
- Check for type mismatches that might cause errors.
Tools for Testing TypeScript Code
Using the right tools can make testing easier. Here are some popular options:
- Jest: Great for unit testing and has built-in support for TypeScript.
- Mocha: A flexible testing framework that works well with TypeScript.
- TypeScript Compiler: Use it to catch type errors before running your code.
Remember, effective testing and debugging can save you a lot of time and frustration in the long run.
In summary, testing and debugging are crucial for ensuring your TypeScript code works as expected. By following these practices, you can improve the reliability of your conditional array logic.
Best Practices for Maintainable Code
Keeping Conditions Simple
When writing code, it’s important to keep your conditions straightforward. Simple conditions are easier to read and understand. Here are some tips:
- Avoid deep nesting of conditions.
- Use descriptive variable names.
- Break complex conditions into smaller functions.
Documenting Conditional Logic
Documentation helps others (and your future self) understand your code. Consider these points:
- Write comments explaining why a condition exists.
- Use clear and concise language.
- Keep documentation up to date with code changes.
Refactoring for Clarity
Refactoring is the process of restructuring existing code without changing its behavior. Here’s how to do it effectively:
- Identify complex conditions that can be simplified.
- Extract repeated logic into functions.
- Regularly review and improve your codebase.
Remember, maintainable code saves time and effort in the long run. Following these best practices will help you write cleaner and more efficient TypeScript code.
To write code that is easy to read and change, follow some simple rules. Start by keeping your code neat and organized. Use clear names for your variables and functions so others can understand what they do. Always add comments to explain tricky parts. This way, when you or someone else looks at the code later, it will be easier to follow. Want to learn more about writing great code? Visit our website to start coding for free!
Wrapping It Up
In conclusion, mastering TypeScript can really help you manage your code better. By using conditional types, you can easily change object keys based on certain rules. This is super useful when you want to make sure your data is consistent, like when you’re processing information. The TransformKeys type we talked about lets you switch keys to uppercase, lowercase, or even change the first letter’s case. As TypeScript keeps getting better, these features will help you write safer and cleaner code. So, the next time you face a tricky coding problem, remember to use conditional types to make your work easier and your code stronger. Keep practicing, and you’ll become a TypeScript pro in no time!
Frequently Asked Questions
What is TypeScript?
TypeScript is a programming language that builds on JavaScript, adding features like strong typing and better tools.
How do I add an object to an array in TypeScript?
You can add an object to an array using methods like push() or by using the spread operator.
What are conditional statements in TypeScript?
Conditional statements allow you to run different code based on whether a condition is true or false.
What is the spread operator?
The spread operator (…) lets you expand an array or object into individual elements.
How do I use ternary operators?
A ternary operator is a shortcut for if-else statements, allowing you to write conditions in a single line.
What are type guards in TypeScript?
Type guards are special checks that help TypeScript understand what type a variable is at a certain point in your code.
Why should I avoid unnecessary computations?
Avoiding unnecessary computations makes your code run faster and use fewer resources.
How can I test my TypeScript code?
You can write unit tests using frameworks like Jest or Mocha to ensure your TypeScript code works as expected.