Mastering the Coding Interview: A Step-by-Step Guide to Solving Coding Interview Problems
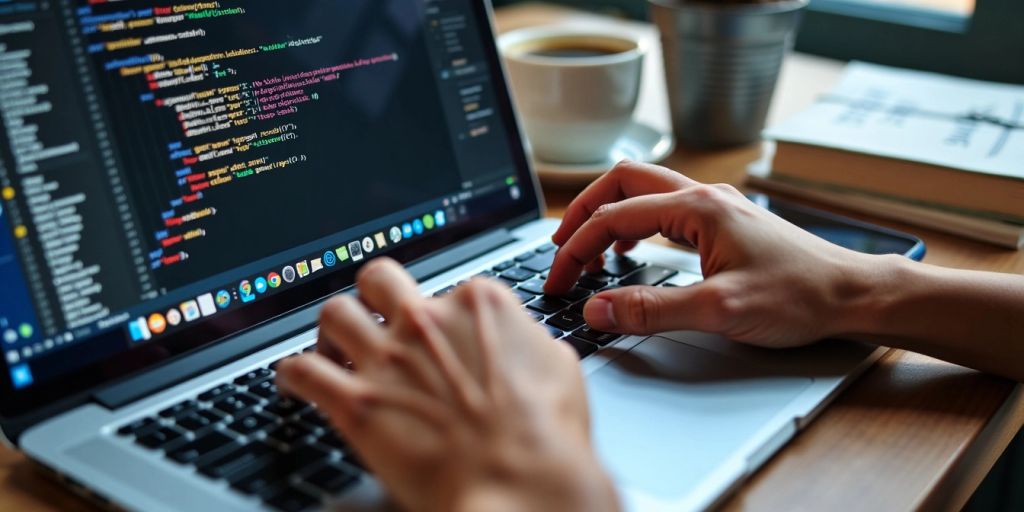
Preparing for a coding interview can feel overwhelming, but with the right strategies and practice, you can excel. This guide will help you navigate the coding interview process, focusing on essential skills, techniques, and tips to boost your confidence and performance. Let’s dive into the key takeaways that will set you up for success!
Key Takeaways
- Understand the coding interview process and what to expect.
- Practice coding problems regularly to build your skills.
- Communicate your thought process clearly during the interview.
- Use the STAR method to prepare for behavioral questions.
- Manage your time wisely and stay calm under pressure.
Understanding the Coding Interview Process
What to Expect in a Coding Interview
In a coding interview, you will face a broad question that tests your problem-solving skills. You might be asked to design a system, like "design YouTube", and sketch out different components. Expect to communicate your thought process clearly and receive feedback from the interviewer.
Types of Coding Interviews
Coding interviews can vary widely. Here are some common types:
- Phone Screen: A preliminary interview where you solve problems over the phone.
- On-Site Interview: A face-to-face interview where you solve problems on a whiteboard.
- Take-Home Assignment: You receive a problem to solve at your own pace and submit your solution later.
Common Interview Formats
Most coding interviews follow a structured format. Here’s a quick overview:
Format Type | Description |
---|---|
Technical | Focuses on coding skills and algorithms. |
Behavioral | Assesses soft skills and cultural fit. |
System Design | Evaluates your ability to design complex systems. |
Understanding the coding interview process is crucial. It helps you prepare effectively and boosts your confidence during the interview.
Mastering Data Structures and Algorithms
To master the coding interview, understanding data structures and algorithms is essential. This section will help you grasp the core concepts, implement common algorithms, and optimize your code for better performance.
Essential Data Structures to Know
Here are some key data structures you should be familiar with:
- Arrays: Basic structure for storing elements.
- Linked Lists: Useful for dynamic data storage.
- Stacks and Queues: Important for managing data flow.
- Hash Maps: Great for quick data retrieval.
- Trees: Essential for hierarchical data representation.
- Graphs: Crucial for network-related problems.
Key Algorithms for Coding Interviews
Familiarize yourself with these important algorithms:
- Sorting Algorithms: Understand mergesort and quicksort.
- Searching Algorithms: Master binary search techniques.
- Dynamic Programming: Learn to solve problems by breaking them down into simpler subproblems.
- Backtracking: Practice generating all possible solutions.
- Graph Algorithms: Know how to traverse and manipulate graphs.
Optimizing Code for Efficiency
When coding, always aim for efficiency. Here are some tips:
- Analyze Time Complexity: Understand how your code performs as input size grows.
- Use the Right Data Structure: Choose the most suitable structure for your problem.
- Avoid Unnecessary Computations: Reuse results when possible to save time.
Mastering data structures and algorithms is not just about memorizing; it’s about understanding how to apply them effectively in real-world scenarios.
By focusing on these areas, you can significantly improve your chances of success in coding interviews. Remember, practice is key!
Systematic Problem-Solving Techniques
Breaking Down Problems Effectively
When you first encounter a problem, it’s important to ask questions to clarify your understanding. Here are some key questions to consider:
- What is the size of the input?
- Are there any negative numbers involved?
- What are some extreme cases to think about?
These questions help you get a clear picture and avoid mistakes.
Writing Pseudocode Before Coding
Before diving into coding, take a moment to write pseudocode. This step-by-step outline helps you organize your thoughts and plan your approach. Think about the data structures you will use and clearly outline your steps. This makes the actual coding much easier.
Testing and Debugging Your Code
After writing your code, it’s crucial to test it thoroughly. Walk through examples and check edge cases to ensure your solution is robust. Debugging is also a key part of this process. Fix any issues you find to make your code reliable.
A systematic approach to problem-solving can unlock your coding interview potential. It shows the interviewer that you can break down complex problems and find effective solutions.
In summary, mastering these techniques will not only help you in interviews but also in real-world coding challenges. Remember, practice is key!
Avoiding Common Pitfalls
Planning Before You Code
Before you start coding, take a moment to think about the problem. Jumping straight into coding can lead to mistakes. Instead, review the problem and plan your approach. This will help you avoid common errors and make your coding process smoother.
Communicating Your Thought Process
Talking through your thought process is important. It shows the interviewer how you think and solve problems. Here are some tips:
- Restate the question to confirm your understanding.
- Break the problem into smaller parts.
- Explain each step as you go along.
Handling Mistakes During the Interview
Mistakes can happen, and that’s okay! If you make a mistake, don’t panic. Here’s how to handle it:
- Acknowledge the mistake calmly.
- Explain how you would fix it.
- Learn from the experience for next time.
Taking a moment to plan and communicate can greatly improve your performance in coding interviews. It helps you avoid mistakes to avoid during onsite interviews and shows your problem-solving skills effectively.
Consistent Practice and Mock Interviews
Consistent practice is crucial for success in coding interviews. Mock interviews can significantly boost your confidence and help you refine your skills. Here’s how to make the most of your practice:
Utilizing Online Resources
- Explore various online platforms like LeetCode and HackerRank for coding problems.
- Use sites like interviewing.io and Pramp for mock interviews.
- These resources provide a wide range of problems and real interview experiences.
Conducting Mock Interviews
- Partner with a friend or colleague to simulate the interview environment.
- Take turns being the interviewer and interviewee to practice both roles.
- Recording your sessions can help you review and improve your performance later.
Simulating Real Interview Conditions
- Time yourself while solving problems to build endurance.
- Practice in a quiet space to mimic actual interview settings.
- This will help reduce anxiety and make you more comfortable on the big day.
Practicing consistently and participating in mock interviews will prepare you to handle the pressure of real coding interviews effectively.
By following these steps, you can enhance your skills and increase your chances of success in coding interviews. Remember, the more you practice, the better you will perform!
Behavioral Interview Preparation
Behavioral interviews are a key part of the hiring process. They help employers understand how you handle different situations. These interviews assess your emotional and behavioral balance. Practicing common behavioral questions can greatly improve your performance.
Crafting Your Story
When preparing for these interviews, it’s important to create engaging stories about your experiences. Use the STAR method to structure your answers:
- Situation – Describe the context or challenge you faced.
- Task – Explain your responsibilities in that situation.
- Action – Detail the steps you took to address the issue.
- Result – Share the outcome and what you achieved.
Using the STAR Method
The STAR method helps you present your experiences clearly. It ensures you cover all aspects of a situation, making your answers concise. For example, when discussing a challenging project, mention the strategic steps you took and the results you achieved. Whenever possible, use numbers to quantify your success.
Common Behavioral Questions
Here are some common behavioral interview questions you might encounter:
- Tell us about a time when you were frustrated because a project wasn’t progressing as planned. How did you deal with it?
- What would be your approach to resolve conflicts at a workplace?
- What are your thoughts about a healthy work-life balance?
Practicing these questions and refining your answers can help you feel more confident during the interview. Consider conducting mock interviews to improve your responses.
By preparing effectively, you can showcase your skills and make a strong impression during your behavioral interview.
Time Management Strategies
Practicing Under Timed Conditions
Managing your time effectively during a coding interview is essential. Practice coding problems under timed conditions to get used to the pressure. Here are some tips to help you:
- Set a timer for each problem.
- Focus on understanding the core of the problem quickly.
- Outline your approach before coding.
Prioritizing Tasks During the Interview
When you start an interview, it’s important to prioritize your tasks. Here’s how:
- Read the problem carefully.
- Break it down into smaller parts.
- Identify which parts are most critical to solve first.
Managing Stress and Anxiety
Feeling anxious is normal, but managing it can help you perform better. Consider these strategies:
- Take deep breaths to calm yourself.
- Remind yourself that it’s okay to make mistakes.
- Stay positive and focus on your strengths.
A systematic approach to time management can greatly enhance your performance in coding interviews. It shows the interviewer that you can handle pressure and think clearly under stress.
Strategy | Description |
---|---|
Set Clear Goals | Define what you want to achieve in each session. |
Prioritize Tasks | Focus on the most important tasks first. |
Delegate Non-Critical Tasks | If possible, share tasks with others. |
Effective Communication Skills
Explaining Your Code Clearly
When you’re coding in an interview, it’s important to explain your code clearly. This helps the interviewer understand your thought process. Here are some tips:
- Talk through your logic as you code.
- Use simple language to avoid confusion.
- Restate the problem to confirm your understanding.
Asking Clarifying Questions
Don’t hesitate to ask questions if something isn’t clear. This shows that you are engaged and want to ensure you are on the right track. Here are some examples of clarifying questions:
- What are the input constraints?
- Are there any edge cases I should consider?
- Can you clarify the expected output?
Active Listening During the Interview
Listening is just as important as speaking. Pay attention to the interviewer’s feedback and adjust your approach accordingly. Here are some ways to practice active listening:
- Nod to show you are following along.
- Repeat back what you heard to confirm understanding.
- Avoid interrupting while the interviewer is speaking.
Effective communication is key in technical interviews. It helps ensure you are understood and allows you to showcase your problem-solving skills effectively. Remember, the goal is to make your thought process clear to the interviewer, so they can see how you tackle challenges.
By mastering these communication skills, you can significantly improve your performance in coding interviews and make a lasting impression on your interviewers.
Post-Interview Strategies
Following Up After the Interview
After your interview, it’s important to send a thank-you email to your interviewer. This shows appreciation and keeps you fresh in their mind. Here are some tips for your follow-up:
- Send it within 24 hours.
- Mention specific topics discussed during the interview.
- Reiterate your interest in the position.
Reflecting on Your Performance
Take some time to think about how the interview went. Consider the following:
- What questions did you find challenging?
- How well did you communicate your thought process?
- Were there any moments you felt particularly strong or weak?
Continuing Your Preparation
Even after the interview, your learning shouldn’t stop. Here are ways to keep improving:
- Review common technical terms and concepts.
- Practice coding problems regularly.
- Seek feedback from peers or mentors.
Remember, every interview is a learning opportunity. Reflecting on your experiences can help you improve for the next one.
By following these strategies, you can enhance your skills and be better prepared for future interviews. Consistent practice and reflection are key to mastering the coding interview process!
Leveraging Feedback for Improvement
Seeking Constructive Criticism
Feedback is a powerful tool for growth. Listening to feedback can help you identify areas where you can improve. Here are some ways to seek constructive criticism:
- Ask for feedback from peers or mentors after mock interviews.
- Review your performance with a friend who can provide honest insights.
- Record your practice sessions and analyze them to spot mistakes.
Implementing Feedback in Practice
Once you receive feedback, it’s important to put it into action. Here’s how to effectively implement feedback:
- Identify specific areas for improvement based on the feedback.
- Create a plan to practice those areas regularly.
- Monitor your progress and adjust your practice as needed.
Tracking Your Progress Over Time
Keeping track of your improvements can motivate you and show how far you’ve come. Consider using a simple table to log your progress:
Date | Area of Improvement | Feedback Received | Action Taken |
---|---|---|---|
2023-10-01 | Code Clarity | Use simpler terms | Practiced explaining code clearly |
2023-10-08 | Problem-Solving | Break down problems | Worked on breaking down problems into steps |
2023-10-15 | Time Management | Manage time better | Timed mock interviews |
Regularly seeking and applying feedback can significantly enhance your coding skills. It shows that you are committed to improvement and willing to learn from your experiences.
By leveraging feedback effectively, you can turn weaknesses into strengths and boost your confidence for coding interviews. Remember, the goal is to grow and become a better problem solver!
Resources for Continued Learning
Recommended Books and Courses
- Cracking the Coding Interview: A must-read for coding interview preparation.
- Introduction to Algorithms: A comprehensive guide on algorithms.
- freeCodeCamp: Offers free coding lessons and projects.
Online Platforms for Practice
Platform | Description |
---|---|
LeetCode | Great for practicing coding interview questions. |
HackerRank | Offers a variety of coding challenges. |
CodeForces | Focuses on competitive programming. |
Joining Coding Communities
- Stack Overflow: Ask questions and get answers from experienced developers.
- Reddit: Subreddits like r/learnprogramming can be helpful.
- Discord Servers: Join coding-related servers for real-time help and discussions.
Learning to code is a journey. Stay curious and keep practicing to improve your skills!
If you’re eager to keep learning and improving your coding skills, visit our website today! We offer a variety of resources that can help you on your journey to becoming a coding expert. Don’t wait—start your free coding lessons now!
Conclusion
Getting ready for a coding interview can feel tough, but it’s totally doable with the right steps. By learning about data structures and algorithms, using a clear way to solve problems, and practicing for behavioral questions, you can really boost your chances of success. Remember, it’s not just about working hard; it’s also about working smart. Use helpful resources like books and online platforms to guide your study. Don’t forget to do mock interviews to build your confidence. With focus and the right tools, you can land that dream job in software engineering. Keep pushing yourself, and good luck!
Frequently Asked Questions
What is the best way to start getting ready for coding interviews?
Start by learning the basics of data structures and algorithms. Try solving problems on websites like LeetCode or HackerRank. Reading books like ‘Cracking the Coding Interview’ can also be helpful.
How important are behavioral questions in coding interviews?
Behavioral questions are really important. They help interviewers see your soft skills, teamwork, and how you solve problems. Use the STAR method (Situation, Task, Action, Result) to prepare your answers.
What should I focus on while practicing coding problems?
Make sure you understand the problem first, break it down into smaller parts, and write clean, efficient code. Don’t forget to test your solutions thoroughly.
How can I improve my time management during coding interviews?
Practice solving coding problems with a timer. This will help you learn to quickly identify the main issue, plan your approach, and explain your thinking while coding.
What are some common mistakes to avoid in coding interviews?
Avoid jumping into coding without a clear plan, not sharing your thought process, and forgetting to test your code. Always take a moment to plan your solution and talk through your approach.
How can mock interviews help in preparation?
Mock interviews simulate real interview conditions, allowing you to practice coding under pressure. They also give you feedback on your performance, helping you improve your skills.
What should I do after the interview?
After the interview, it’s good to follow up with a thank-you email. Reflect on how you did, think about what you could improve, and keep practicing.
Where can I find resources for continued learning?
You can find helpful resources like books, online courses, and coding communities. Websites like AlgoCademy offer interactive tutorials to help you prepare for coding interviews.