Unlocking the Power of Python Enumerate: A Comprehensive Guide to Simplifying Your Loops
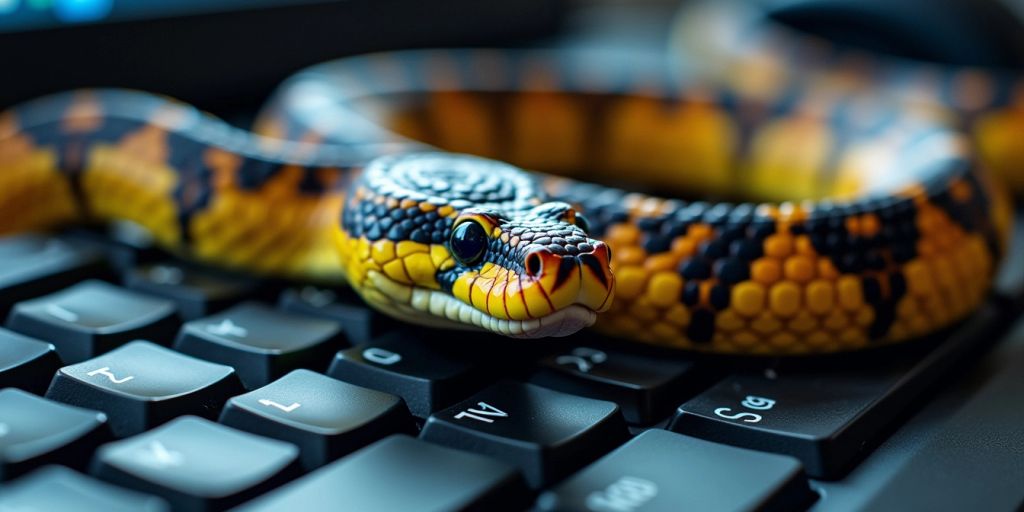
If you’re looking to simplify your Python loops, the `enumerate` function is a game changer. It allows you to keep track of both the index and the value of items in a list or other iterable without the hassle of manual counting. This guide will break down how `enumerate` works, its benefits, and practical ways to use it in your coding projects.
Key Takeaways
- `enumerate` helps you track both index and value in loops, making your code cleaner.
- You can start counting from any number using the `start` parameter.
- Using `enumerate` improves code readability and reduces errors.
- It works with various data structures like lists, tuples, and dictionaries.
- Combining `enumerate` with other functions, like `zip`, can enhance your coding efficiency.
Understanding Python Enumerate
What is Python Enumerate?
The enumerate function in Python is a built-in tool that helps you keep track of the index of items in a collection, like a list or a tuple. Instead of manually counting the position of each item, you can use this function to automatically pair each element with its index. This makes your code cleaner and easier to read.
How Does Python Enumerate Work?
When you use enumerate, it returns a special object that contains pairs of indices and values. For example, if you have a list of fruits, using enumerate will give you both the index and the fruit in each loop iteration. This is especially useful when you need to know the position of an item while processing it.
Basic Syntax of Python Enumerate
The basic syntax for using enumerate is:
enumerate(iterable, start=0)
- iterable: This is the collection you want to loop through, like a list or a string.
- start: This optional parameter allows you to set a custom starting index. By default, it starts at 0.
Here’s a simple example:
fruits = ['apple', 'banana', 'cherry']
for index, fruit in enumerate(fruits):
print(index, fruit)
This will output:
0 apple
1 banana
2 cherry
Key Benefits of Using Enumerate
- Cleaner Code: Reduces the need for manual index tracking.
- Easier Access: Directly retrieves both the index and the value.
- Versatile: Works with various data structures like lists, tuples, and dictionaries.
Using enumerate can significantly enhance your coding experience by simplifying loops and improving readability. It’s a powerful tool that every Python programmer should know!
Benefits of Using Python Enumerate
Using the enumerate() function in Python can greatly enhance your coding experience. Here are some key benefits:
Enhanced Code Readability
- The
enumerate
function makes your code cleaner and easier to understand. Instead of manually tracking indices, you can focus on the elements themselves. - This leads to fewer mistakes and clearer logic in your loops.
- Readable code is easier to maintain and share with others.
Automatic Indexing
- With
enumerate
, each element is automatically paired with its index. This means you don’t have to create a separate counter variable. - This feature simplifies your code and reduces the chance of errors.
- You can easily access both the value and its index in one go.
Versatility Across Data Structures
- The
enumerate
function works with various data types, including lists, tuples, and dictionaries. - This versatility allows you to use it in many different scenarios, making it a powerful tool in your coding toolkit.
- Here’s a quick comparison of how
enumerate
can be applied:
Data Structure | Example Code |
---|---|
List | for index, value in enumerate(my_list): |
Tuple | for index, value in enumerate(my_tuple): |
Dictionary | for index, (key, value) in enumerate(my_dict.items()): |
Using enumerate not only simplifies your loops but also enhances the overall efficiency of your code. It’s a small change that can lead to big improvements!
Implementing Python Enumerate in Different Data Structures
Enumerate with Lists
Using the enumerate
function with lists is straightforward. It allows you to loop through the list while keeping track of the index. Here’s a simple example:
fruits = ['apple', 'banana', 'cherry']
for index, fruit in enumerate(fruits):
print(index, fruit)
This will output:
0 apple
1 banana
2 cherry
Enumerate with Tuples
Enumerating over tuples works just like with lists. Each element is paired with its index:
fruit_tuple = ('apple', 'banana', 'cherry')
for index, fruit in enumerate(fruit_tuple):
print(index, fruit)
Enumerate with Dictionaries
When using enumerate
with dictionaries, you can loop through the key-value pairs. Here’s how:
fruit_dict = {'apple': 1, 'banana': 2, 'cherry': 3}
for index, (key, value) in enumerate(fruit_dict.items()):
print(index, key, value)
Summary of Enumerate in Different Structures
Data Structure | Example Code | Output |
---|---|---|
List | enumerate(fruits) |
0 apple |
Tuple | enumerate(fruit_tuple) |
0 apple |
Dictionary | enumerate(fruit_dict.items()) |
0 apple 1 |
Using enumerate makes it easy to access both the index and the value in various data structures. This is especially helpful when working with Python data structures like lists, tuples, and dictionaries, as it simplifies your code and enhances readability.
Advanced Techniques with Python Enumerate
Using Start Parameter
The enumerate
function allows you to specify a starting index. This is useful when you want to begin counting from a number other than zero. For example:
fruits = ['apple', 'banana', 'cherry']
for index, fruit in enumerate(fruits, start=1):
print(f"{index}: {fruit}")
This will output:
1: apple
2: banana
3: cherry
Reverse Looping with Enumerate
You can also loop through a list in reverse order using enumerate
. This is handy when you want to process items from the end to the beginning. Here’s how:
fruits = ['apple', 'banana', 'cherry']
for index, fruit in reversed(list(enumerate(fruits))):
print(f"Rank {index + 1}: {fruit}")
This will give you:
Rank 3: cherry
Rank 2: banana
Rank 1: apple
Conditional Iteration
Using enumerate
can also help with conditional checks during iteration. For instance, if you want to print only the fruits that start with the letter ‘b’:
fruits = ['apple', 'banana', 'cherry']
for index, fruit in enumerate(fruits):
if fruit.startswith('b'):
print(f"{index}: {fruit}")
This will output:
1: banana
Master Python’s enumerate for efficient coding. By using these advanced techniques, you can make your loops cleaner and more effective.
These techniques not only simplify your code but also enhance its readability and efficiency. Learn how to effectively use Python’s enumerate function for clean, efficient code.
Common Use Cases for Python Enumerate
Tracking Index in Loops
Using the enumerate
function is a great way to keep track of the index while looping through a collection. This is especially useful when you need to know the position of each item. Here are some common scenarios:
- Iterating through lists: Easily access both the index and the value of each item.
- Working with strings: Get the index of each character in a string.
- Processing dictionaries: Access both keys and values along with their indices.
Combining Enumerate with Zip
The enumerate
function can be combined with zip
to iterate over multiple lists simultaneously while keeping track of the index. For example:
list1 = ['a', 'b', 'c']
list2 = [1, 2, 3]
for index, (letter, number) in enumerate(zip(list1, list2)):
print(f"Index: {index}, Letter: {letter}, Number: {number}")
This allows you to work with paired data efficiently.
Enumerate in List Comprehensions
You can also use enumerate
in list comprehensions to create new lists based on the index and value. For instance:
squared = [value ** 2 for index, value in enumerate(range(5))]
This creates a list of squared values while keeping track of their indices.
Using enumerate simplifies your code by removing the need for manual index tracking, making it cleaner and easier to read.
In summary, the enumerate
function is a powerful tool that enhances your ability to work with loops in Python, making your code more efficient and readable. It is especially helpful with iterable objects like lists, strings, or dictionaries.
Handling Errors and Exceptions in Python Enumerate
Common Pitfalls
When using Python’s enumerate
, there are a few common mistakes that can lead to errors:
- Index Out of Range: Trying to access an index that doesn’t exist in the iterable.
- Incorrect Data Type: Using
enumerate
on a non-iterable object will raise aTypeError
. - Modifying the Iterable: Changing the iterable while looping can lead to unexpected behavior.
Error Handling Techniques
To manage errors effectively, consider these techniques:
- Use Try-Except Blocks: This allows you to catch and handle exceptions gracefully.
- Check Data Types: Always ensure the object you are enumerating is iterable.
- Avoid Modifications: Do not change the iterable during the loop to prevent errors.
Best Practices
To make your code more robust, follow these best practices:
- Use
except*
: By usingexcept*
instead ofexcept
, we can selectively handle only the exceptions in the group that match a certain type. This makes your error handling more precise. - Test with Different Data: Always test your code with various data types to ensure it behaves as expected.
- Read Documentation: Familiarize yourself with the
enumerate
function and its limitations to avoid common mistakes.
Remember, handling errors effectively not only improves your code’s reliability but also enhances its readability and maintainability.
Performance Considerations with Python Enumerate
Memory Overhead
Using Python’s enumerate() can lead to increased memory usage. This is because it creates additional counters for each iteration. Here are some key points to consider:
- Each call to
enumerate()
generates a new iterator. - This can be a concern when working with large datasets.
- Memory management becomes crucial in such scenarios.
Efficiency in Large Datasets
When dealing with large datasets, the performance of enumerate()
can be affected. Here’s what to keep in mind:
- Speed: While
enumerate()
is generally fast, it may slow down with very large lists. - Alternatives: For massive datasets, consider using other methods like manual indexing or libraries designed for performance.
- Testing: Always test your code with large inputs to see how it performs.
Optimizing Performance
To get the best out of enumerate()
, consider these tips:
- Use it with smaller datasets when possible.
- Avoid unnecessary conversions or operations within the loop.
- Profile your code to identify bottlenecks.
In summary, while Python’s enumerate() is a powerful tool for simplifying loops, it’s important to be aware of its performance implications, especially in memory usage and speed with large datasets. Always optimize your code for the best results!
Comparing Python Enumerate with Other Looping Techniques
When it comes to looping in Python, there are several techniques available. Each has its own strengths and weaknesses. Here, we will compare Python Enumerate with other common looping methods: the for loop and the while loop.
Enumerate vs. For Loop
- Ease of Use: The
enumerate
function simplifies the process of accessing both the index and the value of elements in a list. In contrast, a traditional for loop requires manual index tracking. - Readability: Using
enumerate
makes the code cleaner and easier to read. For example:fruits = ['apple', 'banana', 'cherry'] for index, fruit in enumerate(fruits): print(index, fruit)
- Manual Indexing: Without
enumerate
, you would need to use a separate counter variable, which can lead to more complex code.
Enumerate vs. While Loop
- Control Flow: A while loop continues until a specific condition is met, making it more flexible for certain tasks. However, it can lead to infinite loops if not managed properly.
- Index Management: With a while loop, you must manually manage the index, which can be error-prone. For example:
index = 0 while index < len(fruits): print(index, fruits[index]) index += 1
- Use Cases: While loops are better suited for scenarios where the number of iterations is not known in advance.
When to Use Enumerate
- Use
enumerate
when you need both the index and the value of elements in a loop. - It is particularly useful for iterating over lists and tuples where the position of each element matters.
- For tasks that require more complex conditions or dynamic iteration, consider using a while loop instead.
Feature | Enumerate | For Loop | While Loop |
---|---|---|---|
Index Tracking | Automatic | Manual | Manual |
Readability | High | Moderate | Low |
Flexibility | Low | Moderate | High |
Use Case | Simple iterations | Iterating over items | Dynamic conditions |
In summary, Python Enumerate is a powerful tool for simplifying loops, especially when you need to track both the index and the value of elements. However, understanding when to use it versus other looping techniques is key to writing efficient and readable code.
Real-World Examples of Python Enumerate
Enumerate in Data Analysis
Using enumerate()
in data analysis can help you keep track of your data points easily. For example, when analyzing a list of sales data, you can use enumerate()
to get both the index and the value:
sales = [200, 450, 300, 500]
for index, sale in enumerate(sales):
print(f"Month {index + 1}: ${sale}")
This will output:
- Month 1: $200
- Month 2: $450
- Month 3: $300
- Month 4: $500
Enumerate in Web Development
In web development, enumerate()
can be used to loop through lists of items, such as user comments. This allows you to display the comment number along with the text:
comments = ["Great post!", "Very informative!", "Thanks for sharing!"]
for index, comment in enumerate(comments, start=1):
print(f"Comment {index}: {comment}")
This will produce:
- Comment 1: Great post!
- Comment 2: Very informative!
- Comment 3: Thanks for sharing!
Enumerate in Machine Learning
In machine learning, you might want to track the index of data points while processing them. For instance, when iterating through training data:
training_data = ["data1", "data2", "data3"]
for index, data in enumerate(training_data):
print(f"Data point {index}: {data}")
This results in:
- Data point 0: data1
- Data point 1: data2
- Data point 2: data3
Using enumerate() in Python can greatly simplify your code by pairing elements with their indices. It’s especially useful in scenarios where you need to keep track of both the index and the value, making your loops cleaner and more efficient.
In summary, enumerate()
is a powerful tool that can enhance your coding practices across various fields, from data analysis to web development and machine learning. It allows for better readability and efficiency in your code, making it a favorite among programmers.
Tips and Tricks for Mastering Python Enumerate
Unpacking Enumerate
Unpacking is a great way to make your code cleaner. Instead of using one variable for both the index and the item, you can separate them. Here’s how:
colors = ["red", "green", "blue"]
for index, color in enumerate(colors):
print(f"Index: {index}, Color: {color}")
Using the Start Parameter
You can change where the counting starts by using the start parameter. This is useful when you want to begin counting from a number other than zero. For example:
fruits = ["apple", "banana", "cherry"]
for index, fruit in enumerate(fruits, start=1):
print(f"{index}: {fruit}")
Reverse Looping with Enumerate
You can also loop in reverse! This is handy when you want to process items from the end to the start. Here’s how:
fruits = ["apple", "banana", "cherry"]
for index, fruit in reversed(list(enumerate(fruits))):
print(f"Rank {index + 1}: {fruit}")
Conditional Iteration
Sometimes, you might want to only process certain items. You can use if statements to filter items while looping:
numbers = [1, 2, 3, 4, 5]
for index, number in enumerate(numbers):
if number % 2 == 0:
print(f"Even number at index {index}: {number}")
Using enumerate() in Python is a smart way to keep track of both the index and the value in your loops. It makes your code cleaner and easier to read!
Exploring Alternatives to Python Enumerate
When working with loops in Python, there are several alternatives to using the enumerate
function. Each method has its own advantages and can be useful in different situations. Here are some common alternatives:
Using Range and Len
- Range and Len: You can use the
range()
function along withlen()
to iterate over a list while keeping track of the index. This method is straightforward but can make the code less readable. - Example:
fruits = ['apple', 'banana', 'cherry'] for i in range(len(fruits)): print(i, fruits[i])
Manual Index Tracking
- Manual Indexing: You can manually maintain an index variable to track the position of elements in a loop. This method gives you full control but can lead to errors if not handled carefully.
- Example:
fruits = ['apple', 'banana', 'cherry'] index = 0 for fruit in fruits: print(index, fruit) index += 1
Third-Party Libraries
- Using Libraries: Some libraries, like
pandas
, offer built-in functions that can simplify looping through data structures. These libraries can provide additional functionality and make your code cleaner. - Example:
import pandas as pd df = pd.DataFrame({'fruits': ['apple', 'banana', 'cherry']}) for index, row in df.iterrows(): print(index, row['fruits'])
In summary, while enumerate is a powerful tool for simplifying loops, there are various alternatives that can be used depending on your specific needs. Each method has its pros and cons, so choose the one that best fits your coding style and requirements.
Conclusion
Exploring these alternatives can help you find the best approach for your coding tasks. Whether you prefer the simplicity of range
and len
, the control of manual indexing, or the power of third-party libraries, Python offers many ways to iterate over data effectively.
Remember, the goal is to write clear and maintainable code!
If you’re curious about different ways to handle tasks in Python without using the built-in enumerate
, you’re in the right place! Explore various alternatives that can make your coding journey smoother. Don’t forget to visit our website for more tips and resources to enhance your coding skills!
Wrapping It Up
In summary, using Python’s enumerate function can really boost your coding skills. It helps you keep track of both the item and its position in a list, making your loops much easier to read and understand. By using enumerate, you can avoid mistakes and write cleaner code. So, don’t hesitate to dive into Python and let enumerate guide you to better coding practices!
Frequently Asked Questions
What is Python Enumerate?
Python Enumerate is a built-in function that adds a counter to an iterable, like a list or a string. It helps you keep track of the index of each item while you loop through it.
How do you use Python Enumerate in a loop?
You can use Python Enumerate in a for loop like this: for index, item in enumerate(your_list):. This way, you get both the index and the item at the same time.
What are the benefits of using Python Enumerate?
Using Python Enumerate makes your code cleaner and easier to read. It also saves you from having to manually track the index, which can reduce mistakes.
Can you start counting from a different number with Enumerate?
Yes! You can specify a starting point by adding a second argument to the enumerate function, like this: enumerate(your_list, start=1). This will start counting from 1 instead of 0.
Is Python Enumerate memory efficient?
While Python Enumerate is generally efficient, it can use more memory than simple loops because it keeps track of both the index and the item.
Can you use Enumerate with different data types?
Absolutely! Python Enumerate works with various data types, including lists, tuples, and even strings. It helps you get the index and value for each item.
What are some common mistakes when using Enumerate?
A common mistake is forgetting to unpack the values. Make sure to use two variables when using Enumerate: one for the index and one for the item.
When should you use Python Enumerate instead of a regular for loop?
You should use Python Enumerate when you need both the index and the item in your loop. It makes your code cleaner and reduces the chance of errors.