Mastering Data Import: How to Use Pandas Read_CSV for Efficient Data Analysis
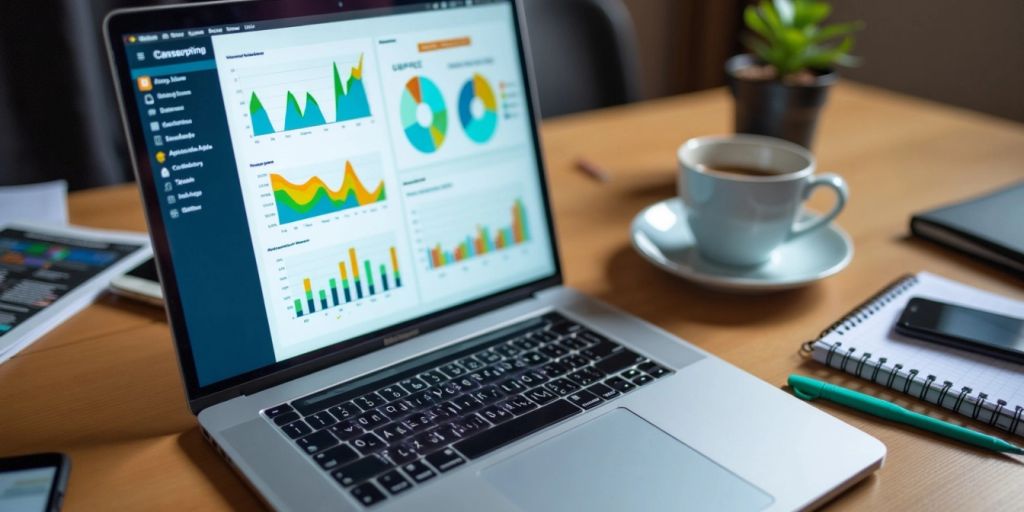
In this article, we will explore how to efficiently use the Pandas `read_csv` function for data analysis. This function is essential for importing data from CSV files into Python, making it a key tool for data scientists and analysts. We will cover its basic features, advanced techniques, and tips for handling different types of data. By mastering `read_csv`, you can streamline your data analysis process and make better use of your data.
Key Takeaways
- Pandas `read_csv` is crucial for loading CSV data into Python.
- Using parameters like `chunksize` helps manage large datasets efficiently.
- Handling different delimiters and encodings is easy with `read_csv`.
- You can identify and handle missing data using built-in options.
- Setting a column as an index while reading can simplify data manipulation.
Understanding the Basics of Pandas Read_CSV
What is Pandas Read_CSV?
The pandas read_csv function is a powerful tool in Python for importing data from CSV files into a DataFrame. This function allows you to read a CSV file from both your local directory and from an online source. It is essential for data analysis as it helps you load data efficiently.
Why Use Pandas Read_CSV?
Using pandas read_csv has several advantages:
- Easy to Use: The function is straightforward and user-friendly.
- Flexible Options: You can customize how you read the data with various parameters.
- Handles Different Formats: It can manage different delimiters and encodings, making it versatile for various datasets.
Basic Syntax and Parameters
The basic syntax for using pandas read_csv is:
import pandas as pd
data = pd.read_csv('your_file.csv')
Here are some important parameters you might use:
- delimiter: Specify the character that separates values (default is a comma).
- header: Define which row to use as column names.
- na_values: Customize how missing data is represented.
Remember: Always check the structure of your CSV file to ensure you use the correct parameters when reading it.
Parameter | Description |
---|---|
delimiter |
Character that separates values (e.g., , ) |
header |
Row number to use as column names |
na_values |
Strings to recognize as NA/NaN |
By understanding these basics, you can effectively use pandas read_csv to import and analyze your data.
Reading Large Datasets with Pandas Read_CSV
Using the Chunksize Parameter
When working with large datasets, it’s important to manage memory effectively. The chunksize parameter allows you to read a CSV file in smaller parts, making it easier to handle. Here’s how you can do it:
- Import Pandas: Start by importing the Pandas library.
import pandas as pd
- Read in Chunks: Specify the chunksize when reading the CSV file.
for chunk in pd.read_csv('large_file.csv', chunksize=1000): # Process each chunk
- Process Each Chunk: You can filter, aggregate, or analyze each chunk as needed.
Memory Management Techniques
To ensure smooth processing of large datasets, consider these techniques:
- Use Chunks: As mentioned, reading in chunks helps manage memory.
- Optimize Data Types: Specify data types to reduce memory usage.
- Drop Unnecessary Columns: Only load the columns you need for your analysis.
Practical Examples
Here’s a simple example of reading a large CSV file using chunks:
import pandas as pd
for chunk in pd.read_csv('large_video_game_sales.csv', chunksize=1000):
print(f'Processing chunk with {chunk.shape[0]} rows')
# Further processing here
This method allows you to efficiently handle large datasets without overwhelming your system. Using chunks can speed up your data processing by up to 250x!
Handling Non-Standard CSV Files
When working with CSV files, you might encounter files that don’t follow the usual format. This section will help you understand how to handle these non-standard CSV files effectively.
Different Delimiters
CSV files typically use commas to separate values, but sometimes they use other characters. Here are some common delimiters:
- Semicolon (
;
) - Tab (
\t
) - Pipe (
|
)
To read a CSV file with a different delimiter, you can use the delimiter
parameter in the pd.read_csv
function. For example:
import pandas as pd
odd_sales_data = pd.read_csv('odd_delimiter_sales.csv', delimiter=';')
Managing Various Encodings
CSV files can also have different encodings, which can cause issues when reading them. Here are some common encodings:
- UTF-8
- UTF-16
- ISO-8859-1
To specify the encoding, use the encoding
parameter:
odd_sales_data = pd.read_csv('odd_delimiter_sales.csv', encoding='utf-16')
Reading CSV Files with Headers
Sometimes, the header row might not be in the first row. You can specify which row to use as the header with the header
parameter. For example:
odd_sales_data = pd.read_csv('odd_delimiter_sales.csv', header=1)
Handling non-standard CSV files can be tricky, but with the right parameters, you can read them easily.
By understanding these techniques, you can effectively manage various CSV formats and ensure your data analysis runs smoothly.
Dealing with Missing Data in CSV Files
Identifying Missing Data
Missing data can be a common issue when working with CSV files. Here are some ways to identify it:
- Check for NaN values: Use
df.isna()
to find missing values in your DataFrame. - Count missing values: Use
df.isna().sum()
to see how many missing values are in each column. - Visualize missing data: Libraries like
missingno
can help visualize where data is missing.
Using na_values Parameter
When reading a CSV file, you can specify which values should be treated as missing. This is done using the na_values
parameter in pd.read_csv()
. For example:
import pandas as pd
df = pd.read_csv('data.csv', na_values=['n/a', 'NA', '--'])
This tells Pandas to treat ‘n/a’, ‘NA’, and ‘–‘ as missing values.
Filling and Dropping Missing Data
Once you’ve identified missing data, you can choose to fill it or drop it. Here are some common methods:
- Filling with a specific value: Use
df.fillna(value)
to replace missing values with a specific number. - Filling with the mean: Use
df.fillna(df.mean())
to fill missing values with the average of the column. - Dropping rows: Use
df.dropna()
to remove any rows that contain missing values.
Handling missing data is crucial for accurate analysis. Imputation of missing values can lead to better results than simply dropping them.
By understanding how to deal with missing data, you can ensure your analysis is more reliable and effective.
Parsing Dates and Times in CSV Files
Using parse_dates Parameter
When you have date and time data in your CSV files, it’s important to handle them correctly. The parse_dates parameter in Pandas is used to automatically recognize and convert date strings into datetime objects. This makes it easier to work with time series data.
Combining Multiple Columns into Datetime
Sometimes, dates might be split across multiple columns. You can combine these columns into a single datetime column using the parse_dates parameter. For example:
import pandas as pd
df = pd.read_csv('data.csv', parse_dates={'date_time': ['date', 'time']})
This code combines the date and time columns into a new column called date_time.
Handling Time Zones
When working with dates, you might also need to consider time zones. You can localize your datetime objects to a specific time zone using the dt.tz_localize() method. Here’s how:
df['date_time'] = df['date_time'].dt.tz_localize('America/New_York')
This will set the time zone for your datetime data, ensuring accurate time calculations.
Remember: Properly parsing dates and times is crucial for accurate data analysis. It helps in avoiding errors and ensures that your time series data is reliable.
Summary
- Use parse_dates to convert date strings into datetime objects.
- Combine multiple columns into a single datetime column when necessary.
- Handle time zones to ensure accurate time calculations.
By following these steps, you can effectively manage date and time data in your CSV files, making your data analysis more efficient and accurate.
Selecting Specific Columns and Rows
Using usecols Parameter
When working with large datasets, you might not need all the columns. The usecols parameter in the read_csv()
function allows you to specify which columns to load. For example:
import pandas as pd
data = pd.read_csv('your_file.csv', usecols=['column1', 'column2'])
This command will only read the specified columns, making your DataFrame smaller and easier to manage.
Filtering Rows Based on Conditions
You can also filter rows based on certain conditions. For instance, if you want to select rows where a specific column’s value is greater than a certain number, you can do:
data_filtered = data[data['column_name'] > 5]
This will create a new DataFrame with only the rows that meet the condition.
Reading a Subset of Data
To read a specific subset of data, you can combine the usecols and filtering techniques. Here’s how:
- Use usecols to select the columns you need.
- Apply a condition to filter the rows.
- Store the result in a new DataFrame.
For example:
data_subset = pd.read_csv('your_file.csv', usecols=['column1', 'column2'])
data_filtered = data_subset[data_subset['column1'] > 10]
By selecting specific columns and filtering rows, you can significantly reduce the amount of data you work with, making analysis faster and more efficient.
In summary, using the usecols parameter and filtering techniques in Pandas allows you to efficiently manage and analyze your data. This is especially useful when dealing with large datasets, as it helps you focus on the information that matters most.
Setting Column as Index While Reading CSV
Using index_col Parameter
When you want to set a specific column as the index while reading a CSV file, you can use the index_col parameter in the read_csv()
function. This allows you to directly specify which column should be used as the index. For example:
import pandas as pd
data = pd.read_csv('your_file.csv', index_col='column_name')
This command will read the CSV file and set the specified column as the index of the DataFrame.
Advantages of Setting Index Early
Setting the index while reading the CSV file has several benefits:
- Improved Performance: It can speed up data processing since the index is set from the start.
- Easier Data Manipulation: You can easily access rows using the index without needing to set it later.
- Cleaner Code: It reduces the number of lines of code needed for data preparation.
Examples and Use Cases
Here are some practical examples of when to set a column as the index:
- Time Series Data: When working with time series data, setting the date column as the index can simplify time-based operations.
- Unique Identifiers: If your data has a unique identifier, like a user ID or product ID, setting it as the index can make data retrieval more efficient.
- Hierarchical Data: For datasets with multiple levels of indexing, setting the index during the read process can help maintain the structure.
Setting the index early can lead to more efficient data analysis and cleaner code.
In summary, using the index_col parameter while reading a CSV file is a powerful feature in Pandas that can enhance your data analysis workflow.
Advanced Data Cleaning Techniques
Data cleaning is a crucial step in preparing your dataset for analysis. Here are some effective techniques to ensure your data is ready:
Renaming Columns
Renaming columns can make your dataset easier to understand. Use the following method:
df.rename(columns={'old_name': 'new_name'})
- This helps clarify what each column represents.
- Clear column names can improve readability.
Converting Data Types
Sometimes, data may not be in the correct format. To fix this, check the data types:
- Use
df.dtypes
to see the current types. - If a column is numeric but shows as a string, convert it:
df['col'] = df['col'].astype(float)
- This ensures calculations are accurate.
Removing Duplicates
Duplicate entries can skew your analysis. To eliminate them:
- Use
df.drop_duplicates()
to remove duplicate rows. - You can specify which columns to check for duplicates:
df.drop_duplicates(subset=['id', 'name'])
- This keeps your dataset clean and efficient.
Remember: Cleaning your data is essential for accurate analysis. A clean dataset leads to better insights.
Summary of Techniques
Here’s a quick recap of the techniques:
- Renaming columns for clarity.
- Converting data types to ensure accuracy.
- Removing duplicates to maintain data integrity.
By applying these techniques, you can enhance the quality of your data, making it more suitable for analysis. Advanced data cleaning techniques are vital for effective data analysis.
Exporting Data to CSV with Pandas
Using to_csv Method
To save your data from a Pandas DataFrame to a CSV file, you can use the to_csv() method. For example:
# Save DataFrame to CSV
data.to_csv('output.csv', index=False)
This command will create a file named output.csv without including the index column.
Common Parameters for Export
When exporting data, you can customize the output using several parameters:
- sep: Define a different delimiter (e.g.,
sep=';'
for semicolon). - header: Include or exclude column names (e.g.,
header=False
). - encoding: Specify the file encoding (e.g.,
encoding='utf-8'
).
Examples of Data Export
Here are some examples of how to export data:
- Basic Export: Save a DataFrame to a CSV file.
- Custom Separator: Use a semicolon as a separator.
- Without Header: Save data without column names.
Remember: Always check your exported file to ensure it meets your needs!
Integrating Pandas with Other Libraries
Pandas is a powerful tool for data analysis, and its integration with other libraries enhances its capabilities. Here’s how you can combine Pandas with popular libraries:
Using Pandas with Matplotlib
- Visualizing Data: You can create various types of plots using Matplotlib. For example:
import matplotlib.pyplot as plt plt.plot(df['Sales'])
- Customization: Matplotlib allows you to customize your plots with titles, labels, and legends.
- Multiple Plots: You can create multiple plots in one figure for comparative analysis.
Combining Pandas and Seaborn
- Statistical Visualizations: Seaborn works well with Pandas DataFrames to create attractive statistical graphics.
- Built-in Themes: It offers built-in themes to improve the aesthetics of your plots.
- Complex Plots: Easily create complex visualizations like heatmaps and violin plots.
Integrating with SQL Databases
- Data Retrieval: Use Pandas to read data directly from SQL databases using the
read_sql
function. - Data Manipulation: After retrieving data, you can manipulate it using Pandas before analysis.
- Exporting Data: You can also export DataFrames back to SQL databases using the
to_sql
method.
By leveraging Pandas with these libraries, you can perform advanced data analysis and create insightful visualizations to drive your data-driven projects.
This integration makes Pandas a go-to tool for data science and analytics, allowing you to go from zero to data hero in no time!
Exploring Alternatives to Pandas Read_CSV
When it comes to reading CSV files, Pandas Read_CSV is a popular choice. However, there are other options available that can be more efficient in certain situations. One such alternative is Dask, a library designed for parallel computing in Python. Dask can handle large datasets more effectively, especially when memory is a concern.
Introduction to Dask
Dask is a powerful tool that allows you to work with large datasets without running into memory issues. It breaks down data into smaller chunks and processes them in parallel, making it a great choice for big data tasks. Here are some key points about Dask:
- Parallel Processing: Dask can run multiple operations at once, speeding up data handling.
- Memory Efficiency: It uses less memory by loading only parts of the dataset at a time.
- Familiar Syntax: Dask’s syntax is similar to Pandas, making it easy to learn for those already familiar with Pandas.
Using Dask for Large Datasets
To use Dask for reading CSV files, you can follow these simple steps:
- Import Dask: Start by importing Dask’s DataFrame functionality.
import dask.dataframe as dd
- Load Your Data: Use Dask to read your CSV file, just like you would with Pandas.
dask_df = dd.read_csv('your_large_file.csv')
- Perform Calculations: You can perform calculations on the Dask DataFrame, and it will handle the data in chunks.
average_sales = dask_df['Sales'].mean().compute()
Comparing Dask and Pandas
Here’s a quick comparison of Dask and Pandas:
Feature | Dask | Pandas |
---|---|---|
Memory Usage | Low (chunked processing) | High (loads all data) |
Speed | Fast (parallel processing) | Slower for large datasets |
Syntax | Similar to Pandas | Standard Pandas syntax |
Dask is a great alternative for those who need to handle large datasets efficiently. It allows for quick CSV data analysis with Python and Pandas, but with added benefits for memory management.
In conclusion, while Pandas Read_CSV is a powerful tool, exploring alternatives like Dask can provide significant advantages when working with large datasets. Consider your specific needs and choose the tool that best fits your project.
If you’re looking for ways to read CSV files without using Pandas, there are plenty of options to explore! Check out our website for more tips and tricks to enhance your coding skills. Don’t miss out on the chance to start coding for free today!
Conclusion
In summary, mastering the use of Pandas’ read_csv function is essential for anyone looking to analyze data effectively. This tool simplifies the process of importing data from CSV files, making it easier to handle various data types and formats. By understanding how to read large datasets in chunks and manage missing values, you can work more efficiently and avoid common pitfalls. Whether you’re just starting out or have some experience, using Pandas can greatly enhance your data analysis skills. So, dive in and start exploring the powerful capabilities of Pandas to transform your data analysis journey!
Frequently Asked Questions
What is the Pandas read_csv function?
The Pandas read_csv function is a tool in Python that helps you load data from CSV files into a DataFrame, which is like a table.
Why should I use read_csv for data analysis?
Using read_csv makes it easy to import data for analysis. It can handle different data types and large files.
How do I read a large CSV file without running out of memory?
You can use the chunksize parameter to read the file in smaller parts instead of all at once.
What if my CSV file has a different separator?
You can change the separator by using the delimiter parameter when calling read_csv.
How do I deal with missing data when using read_csv?
You can use the na_values parameter to specify what counts as missing data, and then fill or drop those values.
Can I read dates from my CSV file?
Yes, by using the parse_dates parameter, you can tell Pandas to treat certain columns as dates.
How can I select specific columns when reading a CSV file?
You can use the usecols parameter to specify which columns you want to load into your DataFrame.
Is it possible to save my DataFrame back to a CSV file?
Absolutely! You can use the to_csv method to export your DataFrame to a new CSV file.