Mastering Python Datetime: A Comprehensive Guide to Date and Time Handling in Python
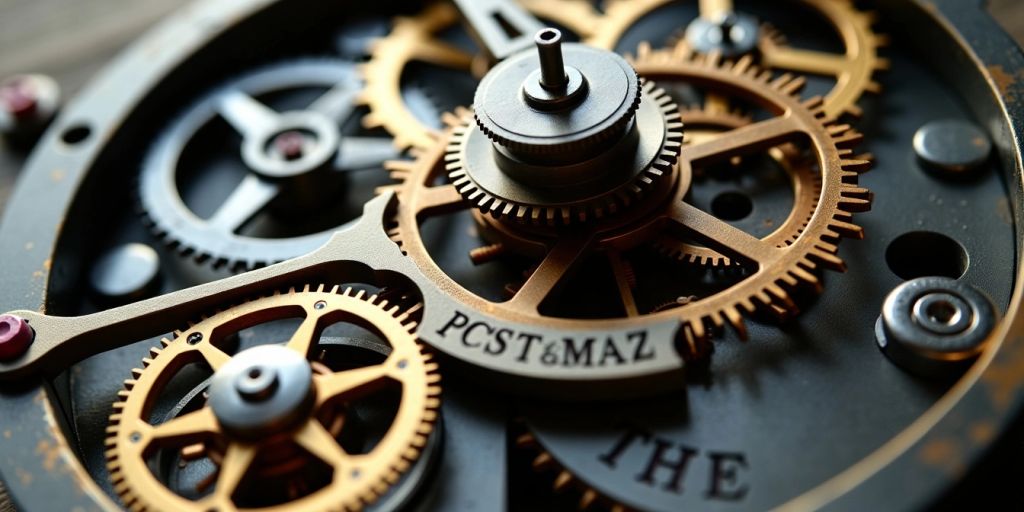
In programming, knowing how to work with dates and times is super important. Python has a special tool called the datetime module that makes it easy to handle all sorts of date and time tasks. This guide will help you understand how to use this module effectively, whether you’re scheduling events or analyzing data over time.
Key Takeaways
- The datetime module is essential for managing dates and times in Python.
- You can create date and time objects easily using built-in classes.
- Formatting and parsing dates is straightforward with the datetime module.
- Handling time zones can be done efficiently using additional libraries like pytz.
- Understanding timedelta helps in calculating time intervals and differences.
Understanding the Python Datetime Module
The datetime module in Python is a built-in library that helps you work with dates and times. It provides several classes, including Date, Time, Datetime, and Timedelta, each designed for specific tasks. To start using this module, you need to import it into your Python script. You can do this with the following command:
import datetime
Overview of the Datetime Module
The datetime module is essential for handling various date and time operations. Here are some key points about it:
- It allows you to create and manipulate date and time objects.
- You can perform arithmetic operations on dates and times.
- It supports timezone-aware datetime objects.
Importing the Datetime Module
To use the datetime module, you can import it in two ways:
- Import the entire module:
import datetime
- Import specific classes directly:
from datetime import datetime
Key Classes in the Datetime Module
The main classes in the datetime module include:
Class | Description |
---|---|
date |
Represents a date (year, month, day). |
time |
Represents a time (hour, minute, second). |
datetime |
Combines both date and time into one object. |
timedelta |
Represents the difference between two dates. |
The datetime module is a powerful tool for managing dates and times in Python. It simplifies many common tasks related to date and time handling, making it easier for developers to implement time-related features in their applications.
Creating and Manipulating Date Objects
Creating Date Objects
To create a Date object, you can use the date()
constructor from the datetime
module. This constructor requires three arguments: year, month, and day. Here’s how you can do it:
import datetime
my_date = datetime.date(2022, 1, 1)
print(my_date)
Output:
2022-01-01
Accessing Date Components
Once you have a Date object, you can easily access its components. Here are some attributes you can use:
year
: Gets the year of the date.month
: Gets the month of the date.day
: Gets the day of the date.
For example:
print(f"Year: {my_date.year}") # Year: 2022
print(f"Month: {my_date.month}") # Month: 1
print(f"Day: {my_date.day}") # Day: 1
Comparing Date Objects
You can compare Date objects using standard comparison operators. Here’s how:
>
: Checks if one date is after another.<
: Checks if one date is before another.==
: Checks if two dates are the same.
Example:
another_date = datetime.date(2023, 1, 1)
print(my_date < another_date) # True
Remember: The datetime class combines both date and time, allowing you to create a datetime object and perform various operations. This makes it a powerful tool for handling dates and times in Python!
Working with Time Objects in Python
Creating Time Objects
To create a time object in Python, you can use the time()
constructor from the datetime
module. This constructor takes up to four arguments: hour, minute, second, and microsecond. Here’s how you can do it:
from datetime import time
# Creating a time object
my_time = time(14, 30, 15) # 2:30:15 PM
print(my_time) # Output: 14:30:15
Extracting Time Components
Once you have a time object, you can easily access its components. Here are the main attributes you can use:
- Hour:
my_time.hour
- Minute:
my_time.minute
- Second:
my_time.second
- Microsecond:
my_time.microsecond
For example:
print(f"Hour: {my_time.hour}, Minute: {my_time.minute}, Second: {my_time.second}")
Formatting Time Strings
You can format time objects into readable strings using the strftime()
method. This method allows you to specify how you want the time to be displayed. Here’s a simple example:
formatted_time = my_time.strftime("%H:%M:%S") # Format as HH:MM:SS
print(f"Formatted Time: {formatted_time}") # Output: Formatted Time: 14:30:15
Remember: Formatting helps in presenting time in a way that is easy to understand.
In summary, working with time objects in Python is straightforward. You can create them, extract their components, and format them for display. This makes handling time in your programs much easier!
Combining Date and Time with Datetime Objects
Creating Datetime Objects
To create a Datetime object, you can use the datetime()
constructor from the datetime module. This constructor requires six arguments: year, month, day, hour, minute, and second. Here’s how you can do it:
import datetime
my_datetime = datetime.datetime(2022, 1, 1, 12, 30, 45)
print(my_datetime)
Output:
2022-01-01 12:30:45
Manipulating Datetime Objects
Once you have a Datetime object, you can easily manipulate it. Here are some common operations:
- Accessing components: You can get the year, month, day, hour, minute, and second from a Datetime object.
- Comparing objects: You can compare two Datetime objects to see which one is earlier or later.
- Arithmetic operations: You can add or subtract time using Timedelta objects.
Datetime Arithmetic
Datetime arithmetic allows you to perform calculations with dates and times. For example, you can add or subtract days, hours, or minutes. Here’s a simple example:
import datetime
today = datetime.datetime.now()
# Adding 5 days
future_date = today + datetime.timedelta(days=5)
print(future_date)
Output:
2022-01-06 12:30:45 (example output)
Note: In Python, the date vs datetime object is important. Use date to represent dates without time, and datetime to represent a specific point in time inclusive of both date and time.
By understanding how to create and manipulate Datetime objects, you can effectively handle various date and time operations in your Python programs.
Timedelta: Working with Time Intervals
Creating Timedelta Objects
The timedelta
class in Python’s datetime module represents a duration, which is the difference between two dates or times. You can create a timedelta
object by specifying the duration in days, seconds, and other time units. Here’s how you can do it:
import datetime
delta = datetime.timedelta(days=7, hours=3, minutes=15)
print(delta) # Output: 7 days, 3:15:00
Adding and Subtracting Time
You can easily add or subtract time using timedelta
. Here are some examples:
- Adding time: To add 1 hour and 30 minutes to a specific time:
new_time = start_time + datetime.timedelta(hours=1, minutes=30) print(new_time) # Output: 2023-07-13 11:30:00
- Subtracting time: To find the difference between two times:
duration = end_time - start_time print(duration) # Output: 2:30:00
- Recurring events: You can also create recurring events by adding a
timedelta
in a loop.
Calculating Time Differences
To find the total seconds in a timedelta
, you can use the total_seconds()
method. For example:
import datetime
duration = datetime.timedelta(days=5, hours=3, minutes=30)
total_seconds = duration.total_seconds()
print(total_seconds) # Output: 468000.0
In summary, the timedelta class is a powerful tool for managing time intervals in Python. It allows you to perform various arithmetic operations on dates and times easily.
Summary
The timedelta
class is essential for anyone working with dates and times in Python. It helps in:
- Creating time intervals
- Adding or subtracting time
- Calculating differences between dates
By mastering timedelta
, you can handle time-related tasks with confidence and ease. Keep exploring the datetime module to unlock its full potential!
Formatting and Parsing Dates and Times
Formatting Dates and Times
In Python, you can convert datetime objects into formatted strings using the strftime()
method. This method allows you to specify format codes that represent different parts of the date and time. Here are some common format codes:
Format Code | Description |
---|---|
%Y | Year (e.g., 2023) |
%m | Month (01-12) |
%d | Day of the month |
%H | Hour (00-23) |
%M | Minute (00-59) |
%S | Second (00-59) |
For example, to format a datetime object:
import datetime
dt = datetime.datetime(2023, 7, 13, 15, 30, 0)
formatted_date = dt.strftime("%Y-%m-%d %H:%M:%S")
print(formatted_date) # Output: 2023-07-13 15:30:00
Parsing Strings into Datetime Objects
To convert a string into a datetime object, you can use the strptime()
method. This method takes two arguments: the string to parse and the format of the string. For example:
date_str = "2023-07-13"
parsed_date = datetime.datetime.strptime(date_str, "%Y-%m-%d")
print(parsed_date) # Output: 2023-07-13 00:00:00
Common Formatting Challenges
When working with dates and times, you might face some challenges:
- Different string formats: Strings can come in various formats, making parsing tricky.
- Time zones: Handling time zones can complicate formatting and parsing.
- Leap years: Some dates only exist in leap years, which can cause errors.
Remember, mastering how to convert string to datetime and vice-versa in Python is essential for effective date and time handling. This skill will help you manage and format date and time values easily in your projects!
Handling Time Zones in Python
Understanding Time Zones
Time zones are regions of the Earth that have the same standard time. Handling time zones is crucial when working with datetime objects in Python. Without proper management, you might end up with incorrect time calculations.
Converting Between Time Zones
To convert datetime objects between different time zones, you can use the pytz
library. Here’s how:
- Import the library: Start by importing
pytz
. - Create a timezone-aware datetime object: Use the
localize
method to assign a time zone to a naive datetime object. - Convert to another time zone: Use the
astimezone
method to change the time zone of your datetime object.
Handling Daylight Saving Time
Daylight Saving Time (DST) can affect time calculations. Here are some key points to remember:
- DST changes can cause time to shift forward or backward.
- Always check if a date falls within DST when performing calculations.
- Use timezone-aware datetime objects to avoid confusion.
Time Zone | UTC Offset | DST Observed |
---|---|---|
America/New_York | UTC-5 | Yes |
Europe/London | UTC+0 | Yes |
Asia/Tokyo | UTC+9 | No |
Remember, localizing dates to specific time zones in Python is the process of assigning a time zone to a naive datetime object or converting an existing datetime.
By understanding and managing time zones effectively, you can ensure accurate date and time handling in your Python applications.
Advanced Datetime Operations
Generating Date Ranges
Creating a series of dates can be useful for various applications. You can generate date ranges using a simple loop or by utilizing libraries like pandas
. Here’s how you can do it:
- Using a loop:
- Using
pandas
:
Finding the Day of the Week
To determine the day of the week for a specific date, you can use the weekday()
method. This method returns an integer where Monday is 0 and Sunday is 6. Here’s a quick example:
import datetime
date = datetime.date(2023, 10, 1)
day_of_week = date.weekday()
print(day_of_week) # Output: 6 (Sunday)
Converting Between Datetime and Timestamp
Sometimes, you may need to convert between a datetime
object and a timestamp (the number of seconds since January 1, 1970). Here’s how:
- From Datetime to Timestamp:
timestamp = dt.timestamp()
- From Timestamp to Datetime:
dt = datetime.datetime.fromtimestamp(timestamp)
Note: Python’s dateutil library is a powerful extension to the standard datetime module, offering advanced date manipulation capabilities. This can simplify many of the operations mentioned above.
Common Challenges and Solutions
Parsing Dates from Strings with Varying Formats
Parsing dates from strings can be tricky due to different formats. Here are some common formats:
- MM/DD/YYYY
- DD-MM-YYYY
- YYYY/MM/DD
To handle these variations, you can use the strptime()
function from the datetime module. This function helps convert a string into a datetime object, making it easier to work with dates.
Handling Timezone Differences
When working with dates and times, timezone differences can lead to confusion. Here are some tips to manage them:
- Always store dates in UTC.
- Convert to local time only when displaying to users.
- Use libraries like
pytz
for accurate timezone handling.
Dealing with Leap Years and Leap Seconds
Leap years and leap seconds can complicate date calculations. Here’s how to manage them:
- Leap Years: Use the
calendar
module to check if a year is a leap year. - Leap Seconds: Be aware that they are rare and usually handled by the system clock.
Remember: Always test your date calculations thoroughly to avoid unexpected results!
Working with Dates in Different Calendars
Gregorian Calendar Support
The datetime module in Python mainly supports the Gregorian calendar. This is the calendar most commonly used worldwide. However, if you need to work with other calendars, you can use external libraries. Here are a few options:
- Hijri Converter: For the Hijri (Islamic) calendar.
- Jdatetime: For the Jalali (Persian) calendar.
- Dateutil: For various date manipulations.
Using External Libraries for Other Calendars
To work with different calendars, you can install libraries using pip. Here’s how:
- Open your command line.
- Type
pip install hijri-converter
for the Hijri calendar. - Type
pip install jdatetime
for the Jalali calendar.
These libraries allow you to create and manipulate dates in their respective formats easily.
Challenges with Different Calendars
When working with various calendars, you may face some challenges:
- Different month lengths: Not all calendars have the same number of days in a month.
- Leap years: Some calendars have different rules for leap years.
- Cultural differences: Some dates may have different significance in different cultures.
Working with dates in different calendars can be tricky, but with the right tools, you can manage it effectively.
Conclusion
In summary, while Python’s datetime module is great for the Gregorian calendar, using external libraries can help you handle other calendars. This flexibility is essential for applications that require date handling across different cultures and systems. For example, you can create a list of dates within a date range in Python using the date and timedelta class and also using the pandas date_range() function.
Practical Applications of Python Datetime
Scheduling Tasks
Using Python’s datetime module, you can easily schedule tasks. Here are some common applications:
- Automating reminders for events or deadlines.
- Scheduling scripts to run at specific times.
- Creating logs for tracking events over time.
Analyzing Time-Series Data
Python’s datetime capabilities are essential for data analysis, especially in time-series data. You can:
- Visualize trends over time.
- Calculate averages and other statistics based on time intervals.
- Detect anomalies in data patterns.
Timestamping Events
Timestamping is crucial for tracking when events occur. You can:
- Record the exact time an event happens.
- Use timestamps for data integrity in databases.
- Create historical records for audits or reviews.
Mastering datetime in Python opens up many possibilities for effective time management in your projects. By understanding how to manipulate dates and times, you can enhance your programming skills significantly.
Python’s datetime module is super useful for many real-life tasks. You can use it to track time, manage dates, and even calculate how long something takes. If you’re curious about how to make the most of this tool, check out our website for more tips and tricks!
Conclusion
In this guide, we learned a lot about handling dates and times in Python. We explored how to create and change date and time objects using the datetime module. We also looked at some advanced tasks, like making date ranges and figuring out time differences. Plus, we talked about common problems, such as dealing with different time zones and leap years. With this knowledge, you can confidently manage dates and times in your Python projects. Remember, practice makes perfect, so keep experimenting with the examples and features of the datetime module. Happy coding!
Frequently Asked Questions
What is the Python datetime module?
The Python datetime module is a built-in tool that helps you work with dates and times. It allows you to create, manipulate, and format date and time objects easily.
How do I create a date object in Python?
You can create a date object by using the `date` class from the datetime module. For example, you can use `datetime.date(2023, 10, 5)` to create a date for October 5, 2023.
Can I compare two date objects?
Yes, you can compare date objects in Python. You can use comparison operators like `==`, `!=`, `<`, `>`, and so on to see which date comes first.
How can I format a date in Python?
You can format a date using the `strftime` method. For example, `date_object.strftime(‘%Y-%m-%d’)` will give you a string like ‘2023-10-05’.
What is a timedelta in Python?
A timedelta is a class in the datetime module that represents a duration, or the difference between two dates or times. You can use it to add or subtract time.
How do I work with time zones in Python?
You can handle time zones using the `pytz` library. This library allows you to convert between different time zones and manage daylight saving time.
What are some common challenges when working with dates and times?
Some common challenges include parsing dates from strings, handling different time formats, and managing leap years or leap seconds.
How can I find the current date and time in Python?
You can find the current date and time by using `datetime.datetime.now()`. This will give you a datetime object representing now.