Understanding Git Rebase: Best Practices and Common Pitfalls
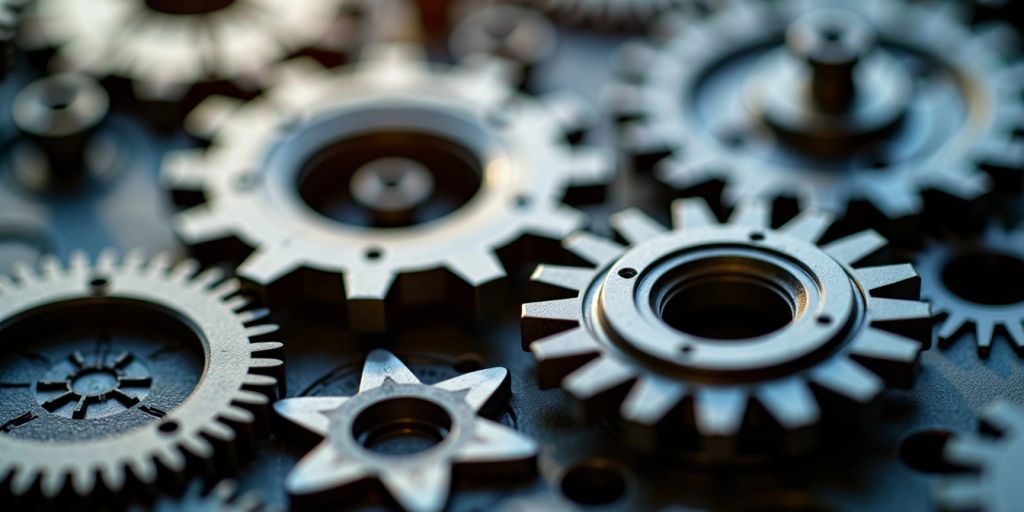
Git is a powerful tool that helps developers manage their code changes. One of its key features is called ‘rebase.’ Understanding how to use rebase effectively can help you keep your project’s history clean and organized. In this article, we will explore the best practices and common mistakes to avoid when using Git rebase.
Key Takeaways
- Git rebase helps create a straight and clear commit history.
- Always back up your work before using rebase to prevent data loss.
- Rebasing can make resolving conflicts easier if done correctly.
- Avoid rebasing shared branches to prevent issues with your team.
- Use interactive rebase to tidy up and combine multiple commits.
What is Git Rebase?
Definition and Purpose
Git rebase is a command in Git that allows you to move or combine a series of commits to a new base commit. This means you can take all the changes made in one branch and apply them onto another branch. For example, if you have a feature branch and a main branch, you can rebase the feature branch onto the main branch, making it look like the feature was created from the latest commit on the main branch.
How Git Rebase Works
When you run the command git rebase
, Git takes the commits from your current branch and replays them on top of the target branch. This process changes the base of your branch, which can help in keeping a clean and linear project history. Here’s a simple illustration:
Before Rebase:
main: A - B - C
\
feature: X - Y - Z
After Rebase:
main: A - B - C
\
feature: X' - Y' - Z'
Differences Between Rebase and Merge
While both rebase and merge are used to integrate changes from one branch to another, they do so in different ways:
Feature | Rebase | Merge |
---|---|---|
History | Linear | Non-linear (branching) |
Commit Messages | Clean, fewer messages | More messages due to merges |
Conflicts | Resolved one at a time | Resolved all at once |
In summary, git rebase simplifies the commit history by making it linear, which can be easier to follow. However, it’s important to use it carefully, especially on shared branches, to avoid complications.
Advantages of Using Git Rebase
Creating a Linear History
Using Git rebase helps create a linear history of commits. This means that the commit history looks like a straight line, making it easier to follow the changes made over time. A linear history is beneficial because it allows developers to understand the project’s evolution without getting lost in a maze of branches and merges.
Cleaner Commit History
One of the main benefits of rebasing is that it results in a cleaner commit history. By eliminating unnecessary merge commits, the history becomes less cluttered. This makes it simpler to review changes and understand the project’s development. Here’s a quick comparison:
Merge Commits | Rebase Commits |
---|---|
Cluttered | Clean |
Harder to follow | Easier to follow |
More complex | More straightforward |
Easier Conflict Resolution
When conflicts arise, rebasing allows for easier conflict resolution. Instead of dealing with multiple conflicts at once, you can resolve them one at a time as each commit is applied. This step-by-step approach can make the process less overwhelming and more manageable.
Rebasing gives you the cleanest possible history, and preserves the fact that you did work in a branch. It also makes it easy to revert an entire “merge.”
In summary, using Git rebase can significantly improve your workflow by providing a linear and cleaner commit history, as well as simplifying conflict resolution. These advantages make it a valuable tool for developers looking to maintain an organized project history.
When to Use Git Rebase
Feature Branches
Rebasing is particularly useful for feature branches. It helps keep the commit history clean and organized. Here are some reasons to use rebase in this context:
- It allows you to apply your changes on top of the latest commits from the main branch.
- It makes it easier to review changes before merging.
- It helps avoid unnecessary merge commits.
Pull Requests
When preparing a pull request, rebasing can be beneficial. It ensures that your feature branch is up-to-date with the main branch. This can help in:
- Reducing the chances of conflicts when merging.
- Making the review process smoother for your team.
- Keeping the project history tidy.
Maintaining a Clean History
If you want to keep a clean commit history, rebasing is your best friend. It allows you to:
- Eliminate unnecessary merge commits.
- Create a linear history that is easier to follow.
- Focus on the important changes without distractions.
Using Git rebase wisely can lead to a more organized and efficient workflow. It’s essential to know when to apply it to maximize its benefits.
Common Pitfalls of Git Rebase
History Alteration Issues
One major concern with using Git rebase is that it can change the commit history. This can lead to confusion, especially if you’ve already shared your branch with others. If someone else has based their work on your commits, rebasing can create a mess. Always be cautious when rebasing shared branches.
Potential for Conflicts
When you rebase, you might run into conflicts. This can be frustrating, especially if you have to resolve the same conflict multiple times. If your branch has been around for a while, it may have diverged significantly from the main branch, making conflicts more likely. Here are some tips to handle conflicts:
- Take your time to understand the conflict.
- Use tools like
git mergetool
to help resolve issues. - Communicate with your team to avoid overlapping changes.
Losing Commit Metadata
Rebasing can sometimes cause you to lose important commit metadata. For example, if you squash commits, you might lose details like the Co-Authored-By
tag. This can be a problem if you want to give credit to multiple contributors. Always check your commit messages after a rebase to ensure you haven’t lost any important information.
Remember, while rebasing can help keep your history clean, it’s essential to be aware of these pitfalls. Understanding the risks can help you use Git rebase more effectively and avoid common mistakes.
Best Practices for Git Rebase
Communicate with Your Team
Before starting a rebase, it’s important to communicate with your team. Let them know your plans to avoid confusion. Here are some tips:
- Inform your team about the rebase schedule.
- Discuss any potential conflicts that might arise.
- Ensure everyone is on the same page regarding the branch status.
Backup Before Rebasing
Always create a backup before you start a rebase. This can save you from losing important work. You can do this by:
- Creating a new branch from your current branch.
- Using
git stash
to save your changes temporarily. - Making sure your work is pushed to a remote repository.
Use Git Reflog to Undo Mistakes
If something goes wrong during a rebase, you can use Git Reflog to recover your work. Here’s how:
- Run
git reflog
to see a list of your recent actions. - Identify the commit you want to return to.
- Use
git reset --hard <commit>
to go back to that point.
Remember, rebasing frequently, ideally daily or multiple times a week, helps keep branches up-to-date with the main branch, reducing the chance and complexity of conflicts.
By following these best practices, you can make your experience with Git rebase smoother and more efficient.
Handling Merge Conflicts During Rebase
Identifying Conflicts
When you perform a rebase, you might run into merge conflicts. This happens when changes in your branch clash with changes in the branch you are rebasing onto. Here are some signs that you have conflicts:
- Git will pause the rebase process.
- You will see messages indicating which files have conflicts.
- The affected files will be marked as unmerged.
Steps to Resolve Conflicts
Resolving conflicts can be tricky, but following these steps can help:
- Check the status of your repository using
git status
to see which files are in conflict. - Open the conflicting files and look for conflict markers (e.g.,
<<<<<<<
,=======
,>>>>>>>
). These markers show the conflicting changes. - Edit the files to resolve the conflicts, then save your changes.
- Use
git add <file>
to mark the conflicts as resolved. - Finally, run
git rebase --continue
to proceed with the rebase.
Tools to Assist with Conflict Resolution
There are several tools that can help you manage merge conflicts more easily:
- Visual Merge Tools: Tools like Meld or KDiff3 provide a graphical interface to help you see and resolve conflicts.
- Git Rerere: This feature remembers how you resolved conflicts in the past, making it easier to handle similar conflicts in the future.
- IDE Integration: Many code editors have built-in tools for resolving merge conflicts, which can simplify the process.
Effective strategies to resolve merge conflicts in Git include communicating with your team. Make sure everyone knows who is working on which parts of the codebase to avoid overlapping changes.
Rebasing Long-Lived Branches
Challenges with Long-Lived Branches
Rebasing branches that have been around for a long time can be tricky. If your branch lives for a month or more, you might find yourself rebasing often, which can become frustrating. Instead of doing multiple rebases, it might be easier to just merge everything at once and handle conflicts only once.
Strategies to Minimize Pain
To make the process smoother, consider these strategies:
- Keep branches short-lived whenever possible.
- Rebase frequently to avoid large conflicts later.
- Communicate with your team about changes to avoid surprises.
When to Consider Merging Instead
Sometimes, merging is a better option than rebasing, especially if:
- The branch has many commits.
- You want to preserve the history of how changes were made.
- You are working with a team and need to avoid conflicts.
Managing long-lived branches can be challenging, but with the right strategies, you can minimize headaches and keep your project on track.
Commit Discipline and Git Rebase
Levels of Commit Discipline
Commit discipline is important when using Git rebase. Here are three common levels:
- Anything Goes: Commit messages like "wip" or "fix" are used without much thought.
- Squashing Commits: Before making a pull request, all messy commits are combined into one with a clear message.
- Atomic Beautiful Commits: Each change is split into well-defined commits, each telling a part of the story.
Squashing Commits
Squashing commits helps keep the history clean. Here’s how to do it:
- Use
git rebase -i
to start an interactive rebase. - Mark commits to squash with
s
. - Save and exit the editor to combine them.
Maintaining Atomic Commits
Maintaining atomic commits is crucial for clarity. Here are some tips:
- Make sure each commit addresses a single issue.
- Write clear and descriptive commit messages.
- Avoid mixing unrelated changes in one commit.
Good commit discipline leads to a cleaner project history, making it easier for everyone to understand changes.
Conclusion
In summary, commit discipline is key when using Git rebase. By following these practices, you can ensure a more organized and understandable commit history, which is beneficial for both individual and team projects. Mastering commit discipline can greatly enhance your Git workflow.
Avoiding Common Mistakes in Git Rebase
Stopping a Bad Rebase
When a rebase goes wrong, it’s crucial to stop it properly. Using git reset --hard
can lead to unexpected behavior. Instead, always use git rebase --abort
to safely exit the rebase process.
Avoiding Rebase on Shared Branches
Rebasing on branches that others are using can create chaos. If someone else has pulled your changes, and you force push after a rebase, it can lead to lost work. Always communicate with your team before rebasing shared branches.
Using Git Rebase -x for Testing
To ensure your commits are stable, consider using git rebase -x
to run tests at each step of the rebase. This helps catch issues early and prevents broken commits from sneaking into your history.
Summary of Common Mistakes
Here’s a quick list of common mistakes to avoid during a rebase:
- Not backing up your branch before rebasing.
- Forgetting to communicate with your team.
- Neglecting to check for conflicts before pushing.
Remember, careful planning and communication can save you from many headaches when using Git rebase. Always be cautious and double-check your actions to maintain a clean commit history.
Git Rebase in Team Environments
Coordinating with Team Members
When working in a team, communication is key. Here are some tips for effective coordination:
- Discuss your plans: Let your team know when you plan to rebase.
- Share your branches: Make sure everyone knows which branches are being worked on.
- Use pull requests: This helps in reviewing changes before they are merged.
Protected Branches and Rebase
In many teams, certain branches are protected to prevent direct changes. Here’s how to handle this:
- Rebase your feature branch: Always rebase your feature branch onto the protected branch before merging.
- Create a pull request: This allows for review and discussion.
- Follow team guidelines: Ensure you adhere to your team’s rules regarding rebasing.
Handling Pull Requests with Rebase
When dealing with pull requests, consider the following:
- Rebase before merging: This keeps the commit history clean.
- Resolve conflicts early: Address any conflicts during the rebase process.
- Communicate changes: Inform your team about any significant changes made during the rebase.
In a collaborative environment, using git rebase effectively can greatly enhance teamwork and project flow. By keeping branches updated and maintaining clear communication, teams can avoid many common pitfalls associated with rebasing.
By following these practices, teams can leverage the power of git rebase while minimizing confusion and conflicts. Remember, working in isolated environments can improve collaboration and streamline the development process.
Advanced Git Rebase Techniques
Interactive Rebase
Interactive rebase is a powerful feature that allows you to edit, reorder, or squash commits. This can help you create a cleaner commit history. Here’s how to use it:
- Run
git rebase -i HEAD~n
(replacen
with the number of commits you want to modify). - In the editor, you can change
pick
tosquash
to combine commits oredit
to modify a commit. - Save and close the editor to apply your changes.
Interactive rebase is great for cleaning up your commit history!
Rebase with Autosquash
When you have fixup commits (commits meant to correct previous ones), you can use autosquash to automatically combine them during a rebase. To do this:
- Mark your fixup commits with
fixup!
in the commit message. - Run
git rebase -i --autosquash HEAD~n
. - Git will automatically squash the fixup commits into the relevant commits.
Using Rebase to Split Commits
Sometimes, you may want to break a large commit into smaller, more manageable ones. Here’s how:
- Start an interactive rebase with
git rebase -i HEAD~n
. - Change
pick
toedit
for the commit you want to split. - After the rebase pauses, use
git reset HEAD^
to unstage the changes. - Stage and commit the changes in smaller parts.
- Continue the rebase with
git rebase --continue
.
Remember, using rebase can change your commit history, so use it wisely!
Technique | Description |
---|---|
Interactive Rebase | Edit, reorder, or squash commits. |
Rebase with Autosquash | Automatically combine fixup commits. |
Split Commits | Break large commits into smaller ones. |
If you’re looking to level up your coding skills, mastering advanced Git rebase techniques is a must! These skills can help you manage your code better and collaborate more effectively with your team. Don’t wait any longer—visit our website to start your coding journey today!
Conclusion
In summary, Git rebase is a strong tool that helps you keep your commit history neat and clear. It’s especially useful for feature branches, making it easier to see what changes were made. However, it’s important to be careful when using it, especially on shared branches. Always talk to your team before rebasing to avoid problems. By knowing when and how to use Git rebase, you can keep your projects organized and make your work smoother and more efficient.
Frequently Asked Questions
What is Git Rebase?
Git Rebase is a way to move or combine a series of changes from one branch to another. It helps keep a clean and straight commit history.
How does Git Rebase differ from Git Merge?
While Git Merge combines changes from two branches and keeps the history of both, Git Rebase takes changes from one branch and applies them to another, creating a linear history.
When should I use Git Rebase?
Use Git Rebase when working on feature branches, before making pull requests, or whenever you want to keep your commit history tidy.
What are the benefits of using Git Rebase?
Git Rebase helps create a straightforward commit history, makes it easier to resolve conflicts, and removes unnecessary merge commits.
What problems can occur with Git Rebase?
Some issues include losing commit details, facing conflicts, and accidentally changing shared branches.
How can I avoid mistakes when using Git Rebase?
Always communicate with your team, back up your work, and practice using Git Rebase to get comfortable with it.
What should I do if I face a conflict during a rebase?
You can identify the conflicts, resolve them step by step, and use tools to help with the resolution.
Is Git Rebase safe to use in team projects?
Be careful! Rebase can change commit history, so it’s best to avoid it on branches that others are using.