Mastering the JavaScript forEach: A Comprehensive Guide to Array Iteration
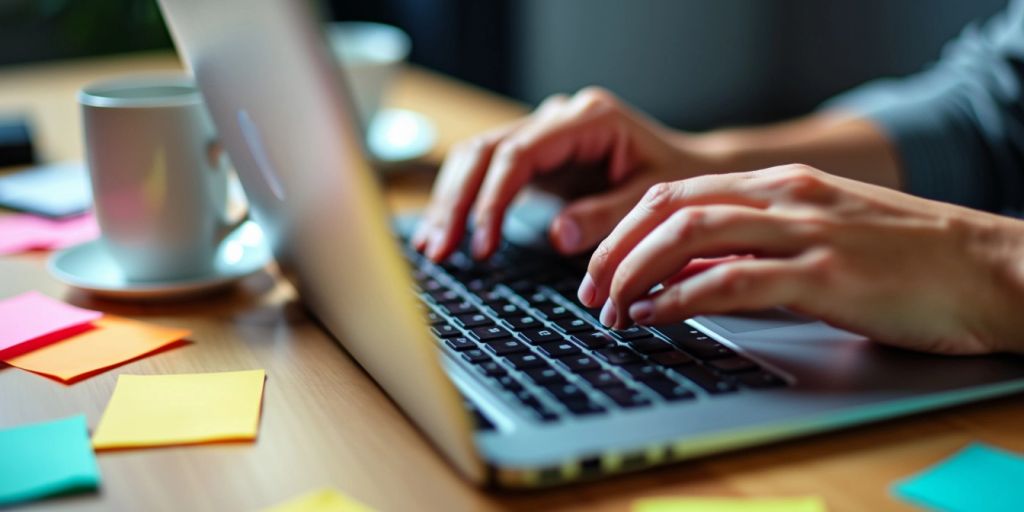
In this article, we will explore the JavaScript forEach method, a powerful tool for looping through arrays. This method simplifies the process of performing actions on each element in an array without the need for traditional loops. By understanding its features, you can make your code cleaner and more efficient. We’ll cover everything from basic syntax to advanced examples, ensuring that you have a solid grasp of how to use forEach effectively in your coding projects.
Key Takeaways
- The forEach method allows easy iteration over array elements without manual index management.
- Using callback functions with forEach makes your code more organized and easier to understand.
- You can access the current element, its index, and the entire array within the forEach loop.
- Arrow functions can simplify your code even more when using forEach.
- Avoid changing the array while using forEach to prevent unexpected results.
Understanding the Basics of JavaScript forEach
What is forEach?
The forEach method in JavaScript is a feature that allows you to go through an array and run a function on each item. It offers an effective method to cycle through array elements without the need for traditional loops. This makes your code cleaner and easier to read.
Basic Syntax and Parameters
The syntax for using forEach is simple:
array.forEach(callback(currentValue, index, array), thisArg);
- callback: A function that is executed for each element in the array.
- currentValue: The current element being processed.
- index: The index of the current element (optional).
- array: The original array (optional).
- thisArg: A value to use as
this
when executing the callback (optional).
How forEach Differs from Other Looping Methods
Unlike traditional loops, forEach does not return a new array or value. Instead, it simply executes the callback function for each element. Here’s how it compares to other methods:
Method | Returns a New Array | Modifies Original Array | Iterates Over Elements |
---|---|---|---|
forEach | No | No | Yes |
map | Yes | No | Yes |
filter | Yes | No | Yes |
reduce | Yes | No | Yes |
Understanding the purpose of forEach helps you appreciate its value in simplifying array iteration. It serves as a powerful tool for performing actions on each element, making your code more elegant and efficient.
By grasping these basics, you can effectively utilize forEach in your JavaScript projects, enhancing both readability and functionality.
Defining and Passing Callbacks in forEach
Role of Callback Functions
In JavaScript, the forEach method requires a callback function to perform actions on each element of an array. This callback function can take up to three parameters:
- currentValue: The current element being processed.
- index: The index of the current element.
- array: The original array that forEach is called on.
By using these parameters, you can easily manipulate or access the elements during iteration.
Examples of Callback Functions
You can define callback functions in two main ways:
- Inline Function:
const numbers = [1, 2, 3, 4, 5]; numbers.forEach(function(element) { console.log(element); });
- Named Function:
function logElement(element) { console.log(element); } const numbers = [1, 2, 3, 4, 5]; numbers.forEach(logElement);
Using a named function is helpful for more complex tasks, allowing for better code organization.
Common Mistakes to Avoid
When using forEach, keep these points in mind:
- Avoid return statements: Unlike other methods, forEach does not return a new array.
- Don’t mix asynchronous operations: This can lead to unexpected results.
- Be cautious with modifications: Changing the array while iterating can cause issues.
Remember, the choice between inline and named functions depends on your specific needs. Both methods are valid and can be used effectively in different scenarios.
Accessing Elements, Index, and Array in forEach
Using Current Value Parameter
The forEach
method allows you to access the current value of each element in the array. This is done through the first parameter of the callback function. For example:
const numbers = [1, 2, 3, 4, 5];
numbers.forEach((num) => {
console.log(num);
});
In this example, num
represents the current value being processed in the array.
Utilizing Index Parameter
In addition to the current value, you can also access the index of each element. This is useful when you need to know the position of the element in the array. The index is the second parameter in the callback function:
const fruits = ['Apple', 'Banana', 'Cherry'];
fruits.forEach((fruit, index) => {
console.log(`Index: ${index}, Fruit: ${fruit}`);
});
Working with the Original Array
The third parameter of the callback function gives you access to the original array itself. This can be helpful if you need to reference the entire array while processing each element:
const colors = ['Red', 'Green', 'Blue'];
colors.forEach((color, index, arr) => {
console.log(`Color: ${color}, All Colors: ${arr}`);
});
Note: The forEach method is an iterative method. It calls a provided callback function once for each element in an array in ascending-index order.
Summary
- Current Value: Accessed as the first parameter.
- Index: Accessed as the second parameter.
- Original Array: Accessed as the third parameter.
By understanding these parameters, you can effectively utilize the forEach
method to iterate through arrays and perform various operations on their elements.
Leveraging the ‘thisArg’ Parameter in forEach
Purpose of ‘thisArg’
The thisArg
parameter in the forEach
method allows you to set the value of this
inside the callback function. By default, this
refers to the global object, but you can change it to a specific object by using thisArg
. This is especially useful when you want to use methods from an object as your callback.
Examples of ‘thisArg’ Usage
Here’s a simple example to illustrate how thisArg
works:
const person = {
name: 'John',
greet: function() {
console.log(`Hello, ${this.name}!`);
}
};
const names = ['Alice', 'Bob', 'Charlie'];
names.forEach(person.greet, person);
In this example, the greet
method is called for each name in the array, and this
correctly refers to the person
object.
Best Practices for ‘thisArg’
When using thisArg
, keep these tips in mind:
- Use it when necessary: Only set
thisArg
if you need to change the context ofthis
. - Avoid confusion: Make sure the context is clear to anyone reading your code.
- Test your code: Always test to ensure that
this
behaves as expected in your callback.
Understanding how to use the thisArg parameter effectively can help you control the context of your functions, making your code cleaner and more efficient.
By leveraging the thisArg
parameter, you can ensure that your callback functions operate in the correct context, enhancing the functionality of your code.
Using Arrow Functions for Concise Callbacks
Introduction to Arrow Functions
Arrow functions are a concise way to write functions, especially useful as callback functions. They allow you to create functions in a shorter and clearer manner, making your code easier to read.
Benefits of Arrow Functions in forEach
Using arrow functions with the forEach
method has several advantages:
- Cleaner Syntax: Arrow functions reduce the amount of code you need to write.
- Automatic Binding: They automatically bind the current scope, so you don’t have to worry about the
this
keyword. - Readability: They make your code more straightforward and easier to understand.
Examples of Arrow Functions in forEach
Here’s a simple example of using an arrow function with forEach
:
const numbers = [1, 2, 3, 4, 5];
numbers.forEach(element => {
console.log(element);
});
In this example, the arrow function takes element
as its parameter and logs it to the console. This is much shorter than using a traditional function.
Arrow functions help in writing cleaner and more efficient code, especially for simple operations.
Summary
In summary, arrow functions are a great tool for writing concise callbacks in JavaScript. They simplify your code and enhance readability, making them a preferred choice for many developers when using the forEach
method.
Best Practices for Using JavaScript forEach
Avoiding Modifications During Iteration
When using the forEach
method, it’s best to avoid changing the array while iterating through it. Modifying the array can lead to unexpected results. Here are some tips:
- Use
forEach
for read-only operations. - If you need to modify elements, consider using
map
instead. - Always create a copy of the array if modifications are necessary.
Using Other Array Methods Appropriately
While forEach
is useful, it’s not always the best choice. Here’s when to use other methods:
- Use
map
when you want to create a new array from the original. - Use
filter
to get a subset of the array based on a condition. - Use
reduce
for accumulating values from the array.
Ensuring Readability and Maintainability
Code should be easy to read and understand. Here are some practices to enhance clarity:
- Use descriptive names for your callback functions.
- Keep your callback functions short and focused on a single task.
- Comment your code to explain complex logic.
Remember, the forEach method does not return a value. It simply executes the provided function on each element, so plan your logic accordingly.
By following these best practices, you can make your code cleaner and more efficient while using the forEach
method effectively.
Advanced Examples of JavaScript forEach
Working with Arrays of Objects
The forEach
method is great for handling arrays of objects. For example, consider the following array of user objects:
const users = [
{ id: 1, name: 'John', age: 25 },
{ id: 2, name: 'Alice', age: 30 },
{ id: 3, name: 'Bob', age: 35 }
];
users.forEach(user => {
console.log(`User ID: ${user.id}, Name: ${user.name}, Age: ${user.age}`);
});
This code will print each user’s details to the console. Using forEach makes it easy to access each object’s properties.
Modifying Array Elements
You can also modify elements in an array using forEach
. Here’s how you can square each number in an array:
const numbers = [1, 2, 3, 4, 5];
numbers.forEach((num, index, arr) => {
arr[index] = num * num;
});
console.log(numbers); // Output: [1, 4, 9, 16, 25]
Handling Asynchronous Operations
While forEach
is not designed for asynchronous tasks, you can still use it with promises. Here’s an example:
const items = [1, 2, 3, 4, 5];
const delay = (ms) => new Promise(resolve => setTimeout(resolve, ms));
items.forEach(async (item) => {
await delay(1000); // Wait for 1 second
console.log(`Processed item: ${item}`);
});
Note: Mixing asynchronous operations with forEach can lead to unexpected results. It’s often better to use a for…of loop for such cases.
Summary of Key Points
- Iterating over objects: Easily access properties of each object in an array.
- Modifying values: Change elements directly within the array.
- Asynchronous handling: Use with caution; consider alternatives for better control.
By mastering these advanced examples, you can leverage the full potential of the forEach
method in your JavaScript projects!
Real-World Applications of JavaScript forEach
Practical Examples in Web Development
The forEach
method is a game-changer in web development. Here are some common applications:
- Iterating through user data: You can easily loop through user profiles to display information on a webpage.
- Updating UI elements: Use
forEach
to modify multiple elements in the DOM based on an array of data. - Handling events: Attach event listeners to multiple elements efficiently.
Case Studies and Success Stories
Many developers have shared their success stories using forEach
. Here are a few highlights:
- E-commerce websites: Improved performance by using
forEach
to update product listings dynamically. - Social media platforms: Enhanced user experience by efficiently rendering feeds with
forEach
. - Data visualization: Simplified the process of rendering charts and graphs by iterating over data arrays.
Common Use Cases in Projects
In various projects, forEach
is often used for:
- Data processing: Transforming or filtering data before displaying it.
- Form handling: Validating multiple form fields in a single loop.
- Animation sequences: Creating smooth animations by iterating through elements.
The forEach method is a simple yet powerful way to iterate over arrays in JavaScript, making code cleaner and easier to read.
By leveraging the forEach
method, developers can create more efficient and readable code, enhancing the overall quality of their projects.
Benefits of Using JavaScript forEach
Improved Code Readability
Using the forEach method makes your code easier to read. It allows you to focus on what you want to do with each element in the array, rather than how to loop through it. This leads to cleaner and more understandable code.
Simplified Iteration Logic
The forEach method simplifies the logic of iterating through arrays. Instead of managing indices and conditions, you can directly apply a function to each element. This reduces the chances of errors and makes your code more efficient.
Enhanced Functional Programming
By using forEach, you embrace a more functional programming style. This encourages you to think about what you want to achieve rather than how to achieve it. Here are some benefits of this approach:
- Clarity: Your intentions are clearer.
- Consistency: You use a standard method for looping.
- Maintainability: Your code is easier to update and fix.
The forEach method is a powerful tool that helps you write elegant and efficient code. It abstracts away the low-level details of iteration, allowing you to focus on the logic you want to implement.
Comparison with Other Looping Methods
When comparing forEach with traditional loops, you’ll find that:
- forEach is a higher-order function that simplifies iteration with built-in array methods, while for loops offer more control and flexibility.
- Using forEach can lead to fewer lines of code, making it a more concise option.
In summary, the forEach method not only improves readability but also enhances the overall quality of your code, making it a valuable tool in your JavaScript toolkit.
Common Pitfalls and How to Avoid Them
Off-by-One Errors
One common mistake when using forEach is the off-by-one error. This happens when you accidentally skip the first or last element of the array. To avoid this, always double-check your loop boundaries. Here are some tips:
- Ensure your loop starts at index 0.
- Verify that your loop condition includes the last index.
- Use console logs to debug and confirm the loop’s behavior.
Infinite Loops
Another issue is creating infinite loops, which can crash your program. This often occurs when the loop condition is not properly defined. To prevent this:
- Always define a clear exit condition.
- Use break statements wisely to exit the loop when necessary.
- Test your loops with smaller arrays to ensure they terminate correctly.
Misusing the Callback Function
Misunderstanding how the callback function works can lead to unexpected results. Here’s how to avoid this pitfall:
- Remember that the callback function is called for each element in the array.
- Ensure you are using the correct parameters in your callback.
- Be cautious with asynchronous operations; it requires careful handling to avoid issues like unhandled promises or inefficient code.
By being aware of these common pitfalls, you can write more reliable JavaScript code and enhance your programming skills.
Comparing forEach with Other Array Methods
forEach vs. Map
The forEach method is great for executing a function on each element of an array, but it does not return a new array. In contrast, the map method transforms each element and creates a new array with the results. Here’s a quick comparison:
Feature | forEach | map |
---|---|---|
Returns a new array | No | Yes |
Purpose | Execute a function for each item | Transform data |
Use case | Side effects (e.g., logging) | Data transformation (e.g., squaring numbers) |
forEach vs. Filter
The filter method is used to create a new array containing only the elements that pass a certain test. Here’s how it compares to forEach:
Feature | forEach | filter |
---|---|---|
Returns a new array | No | Yes |
Purpose | Execute a function for each item | Select items based on a condition |
Use case | Logging or updating UI | Extracting even numbers from an array |
forEach vs. Reduce
The reduce method is used to reduce an array to a single value by executing a function on each element. Here’s a comparison:
Feature | forEach | reduce |
---|---|---|
Returns a new array | No | No (returns a single value) |
Purpose | Execute a function for each item | Aggregate data |
Use case | Logging or side effects | Summing numbers in an array |
In summary, while forEach is useful for executing functions on each element, methods like map, filter, and reduce are better suited for transforming data or creating new arrays. Choose the method that best fits your needs!
Future Trends and Enhancements in Array Iteration
Upcoming JavaScript Features
As JavaScript continues to evolve, new features are being introduced to improve array iteration. Some of the anticipated enhancements include:
- Performance improvements for existing methods.
- New methods that simplify complex iterations.
- Better support for asynchronous operations within loops.
Community Contributions
The JavaScript community plays a vital role in shaping the future of array iteration. Contributions often include:
- Proposals for new methods to enhance functionality.
- Libraries that extend the capabilities of built-in methods.
- Tutorials and resources that help developers adopt best practices.
Potential Improvements in forEach
The forEach
method is widely used, but there are areas for improvement:
- Optimization of loops in JavaScript: Loop optimization is crucial in JavaScript for enhancing performance, particularly in applications requiring intensive computation or handling large data sets.
- Enhanced error handling to manage exceptions during iteration.
- More flexibility in callback functions to allow for early exits or breaks in the loop.
The future of array iteration in JavaScript looks promising, with ongoing developments aimed at making coding easier and more efficient for developers.
As we look ahead, the way we work with arrays in programming is set to evolve. New methods and tools will make it easier and faster to handle data. If you’re eager to stay ahead in coding, visit our website to explore interactive tutorials that can help you master these skills. Don’t miss out on the chance to enhance your coding journey!
Conclusion
In conclusion, mastering the forEach method in JavaScript can greatly enhance your coding skills. This guide has shown you how to effectively use forEach to loop through arrays, making your code cleaner and easier to read. By practicing the examples and concepts discussed, you’ll become more comfortable with this powerful tool. Remember, the key to becoming a better programmer is to keep experimenting and learning. So, dive in, try out forEach in your projects, and enjoy the journey of coding! Happy coding!
Frequently Asked Questions
What is the forEach method in JavaScript?
The forEach method is a built-in function that lets you loop through each item in an array and perform an action on it.
How do you use the forEach method?
You call forEach on an array, providing a function that defines what to do with each item.
Can you modify the array while using forEach?
Yes, but it’s not recommended because it can lead to unexpected results.
What are some common mistakes when using forEach?
Common mistakes include trying to use return statements to break out of the loop or modifying the array during iteration.
How does forEach compare to other loop methods like map or filter?
forEach is mainly for executing functions on each item, while map creates a new array and filter selects items based on a condition.
What is the ‘thisArg’ parameter in forEach?
The ‘thisArg’ parameter allows you to set the value of ‘this’ inside the callback function.
Can I use forEach with objects?
No, forEach only works with arrays. For objects, you can use methods like Object.keys or Object.entries.
Are there performance issues with using forEach?
ForEach is generally efficient, but if you’re dealing with very large arrays, other methods might be faster.