Mastering the Basics: A Step-by-Step Guide on How to Code Effectively
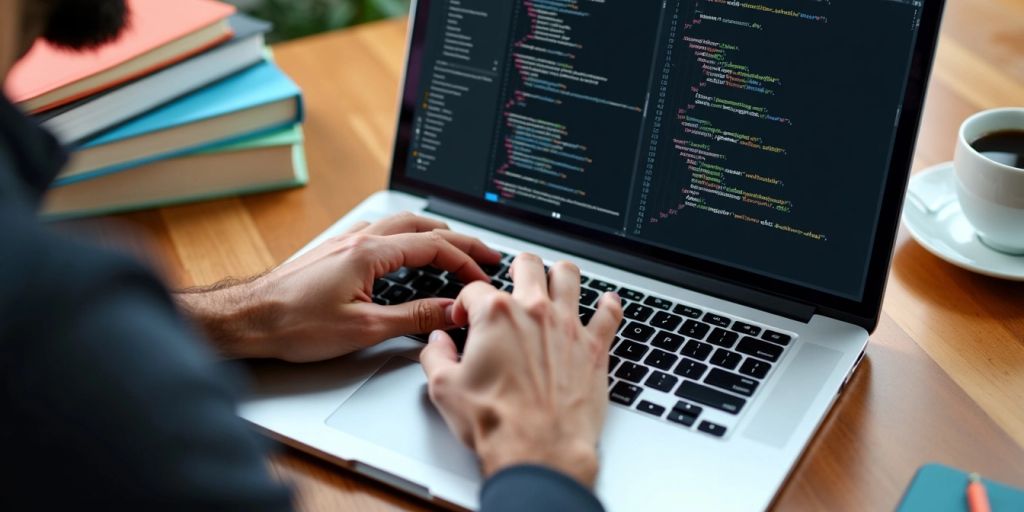
Learning to code can be a rewarding journey filled with challenges and discoveries. This guide breaks down the essential steps needed to become an effective coder, from understanding the basics to preparing for coding interviews. Whether you are just starting out or looking to sharpen your skills, this article provides valuable insights to help you on your coding adventure.
Key Takeaways
- Coding is about solving problems, not just writing code.
- Understanding the basics is crucial for learning more advanced concepts.
- Writing clean code helps you and others understand your work better.
- Practice patience; learning to code takes time and effort.
- Use available resources like online tutorials and coding communities to enhance your learning.
Understanding the Basics of How to Code
Defining Coding and Its Importance
Coding is the process of writing instructions for computers to follow. It’s essential because it allows us to create software, websites, and applications that make our lives easier. Here are some key points about coding:
- Problem-solving: Coding helps develop strong problem-solving skills.
- Creativity: It allows you to bring your ideas to life.
- Career opportunities: Many jobs require coding skills today.
Common Misconceptions About Coding
Many people think coding is only for geniuses or math whizzes. However, anyone can learn to code with practice and patience. Here are some common myths:
- You need to be a math expert.
- Coding is too hard for beginners.
- You can’t learn coding on your own.
The Evolution of Coding Languages
Coding languages have changed a lot over the years. They started simple and have become more complex and powerful. Here’s a brief overview:
Year | Language | Description |
---|---|---|
1950 | Fortran | One of the first high-level languages |
1995 | JavaScript | Essential for web development |
2000 | Python | Known for its readability and ease |
Learning to code is like learning a new language. It takes time, but the rewards are worth it!
In summary, understanding the basics of coding is crucial for anyone looking to dive into the tech world. With the right mindset and resources, you can start your coding journey today!
Choosing the Right Programming Language
Factors to Consider When Selecting a Language
When picking a programming language, think about these important factors:
- Your goals: What do you want to create?
- Ease of learning: Is it beginner-friendly?
- Community support: Are there resources available to help you?
Popular Programming Languages for Beginners
Here are some of the most recommended languages for those just starting out:
Language | Description |
---|---|
HTML | Great for web structure; easy to learn. |
JavaScript | Makes web pages interactive; essential for web development. |
Python | Known for its readability and versatility. |
C | Good for understanding programming basics. |
Pros and Cons of Different Languages
Each language has its strengths and weaknesses. Here’s a quick overview:
- HTML:
- Pros: Simple and straightforward.
- Cons: Limited to web structure.
- JavaScript:
- Pros: Dynamic and widely used.
- Cons: Can be complex for beginners.
- Python:
- Pros: Easy to read and has many libraries.
- Cons: Slower than some other languages.
- C:
- Pros: Teaches fundamental programming concepts.
- Cons: More complex syntax.
Choosing the right programming language is crucial for your coding journey. It can shape your learning experience and influence your future projects.
In summary, when deciding on a language, consider your goals and what you want to achieve. What’s the best programming language to learn first? This guide is for anyone who is new to programming in order to help give a better idea of the best programming language to learn first.
Setting Up Your Development Environment
Essential Tools for Coding
To start coding effectively, you need the right tools. Here are some essentials:
- Code Editor: A good code editor helps you write and manage your code. Popular options include Notepad++, Sublime Text, and Visual Studio Code.
- Integrated Development Environment (IDE): IDEs like PyCharm or Eclipse provide a complete environment for coding, debugging, and testing.
- Version Control System: Tools like Git help you track changes in your code and collaborate with others.
Installing and Configuring Code Editors
Setting up your code editor is crucial. Here’s how to do it:
- Download the editor: Go to the official website and download the latest version.
- Install the editor: Follow the installation instructions.
- Configure settings: Adjust settings like themes and shortcuts to suit your preferences.
Using Integrated Development Environments (IDEs)
IDEs can make coding easier. Here’s why:
- They offer built-in tools for debugging and testing.
- They often include features like code completion and error highlighting.
- Familiarize yourself with the interface: Spend some time exploring the features to maximize your productivity.
Remember: Setting up your developer environment is the first step to coding success. Make sure you have a reliable internet connection and access to a command line interface (CLI) on your local machine. This will help you run commands and manage your projects effectively.
Mastering Core Programming Concepts
Variables and Data Types
Variables are like containers that hold information. Understanding how to create and use them is very important. Here are some common data types:
- Integers: Whole numbers like 1, 2, or -3.
- Floats: Numbers with decimals, such as 3.14.
- Strings: Text, like "Hello, World!".
- Booleans: True or false values.
Control Structures and Logic
Control structures help decide the flow of a program. Here are the main types:
- Conditional Statements: These include
if
,else
, andswitch
statements that help make decisions. - Loops: Such as
for
andwhile
, which repeat actions until a condition is met. - Switch Statements: A way to choose between many options based on a variable.
Functions and Scope
Functions are blocks of code that perform a specific task. They help keep your code organized and reusable. Here are some key points:
- Parameters: Inputs that a function can take.
- Return Types: What a function gives back after it runs.
- Scope: Refers to where a variable can be accessed in your code. Variables can be local (inside a function) or global (accessible everywhere).
Mastering these core concepts is essential for any programmer. They form the foundation for more complex topics and help you write better code.
Understanding Data Structures and Algorithms
Common Data Structures
Data structures are ways to organize and store data. Here are some common types:
- Arrays: A collection of items stored at contiguous memory locations.
- Lists: A sequence of elements that can grow or shrink in size.
- Stacks: A collection of elements that follows the Last In First Out (LIFO) principle.
- Queues: A collection that follows the First In First Out (FIFO) principle.
- Dictionaries: A collection of key-value pairs for fast data retrieval.
Basic Algorithms Every Coder Should Know
Algorithms are step-by-step procedures for solving problems. Here are a few essential ones:
- Sorting Algorithms: Such as Bubble Sort and Quick Sort, which arrange data in a specific order.
- Searching Algorithms: Like Linear Search and Binary Search, which help find specific data.
- Recursion: A method where a function calls itself to solve smaller instances of a problem.
The Importance of Algorithm Efficiency
Understanding how efficient an algorithm is can save time and resources. Here’s why it matters:
- Speed: Faster algorithms can handle larger datasets.
- Resource Usage: Efficient algorithms use less memory and processing power.
- Scalability: Good algorithms can adapt as data grows.
Data structures and algorithms (DSA) are fundamental in computer science that help us to organize and process data efficiently. They are used in solving common problems and are essential for writing effective code.
By mastering these concepts, you will be better equipped to tackle coding challenges and improve your programming skills.
Writing Clean and Efficient Code
Writing clean and efficient code is essential for any programmer. Good coding practices not only make your code easier to read but also help you and others understand it better. Here are some key points to consider:
Best Practices for Clean Code
- Follow coding standards and best practices to follow:
- Choose industry-specific coding standards
- Focus on code readability
- Use meaningful names for variables and functions
- Avoid using a single identifier for multiple purposes
Code Commenting and Documentation
- Comments are crucial for explaining your code. They help others (and your future self) understand what you were thinking when you wrote it. Here are some tips:
- Write comments that explain the purpose of complex code.
- Keep comments clear and concise.
- Avoid over-commenting; only comment where necessary.
Refactoring Techniques
- Refactoring is the process of restructuring existing code without changing its external behavior. This can help improve code efficiency and readability. Here are some techniques:
- Break down large functions into smaller, manageable ones.
- Remove any duplicate code.
- Simplify complex expressions.
Writing clean code is not just about making it work; it’s about making it understandable and maintainable for everyone involved.
By following these practices, you can ensure that your code is not only functional but also clean and efficient, making it easier for you and others to work with it in the future.
Debugging and Testing Your Code
Common Debugging Techniques
Debugging is a crucial part of coding. Here are some effective techniques to help you debug your code:
- Understand the problem: Before diving into the code, take a moment to grasp what the issue is.
- Check recent changes: If the code was working before, look at what you changed last.
- Use print statements and logs: These can help you see what your code is doing at different points.
- Isolate the problem: Try to narrow down where the issue is happening.
- Review error messages: They often provide clues about what went wrong.
Writing Unit Tests
Unit tests are small tests that check if a specific part of your code works correctly. Here’s why they are important:
- They help catch bugs early.
- They ensure that your code behaves as expected.
- They make it easier to change your code later without breaking things.
Using Debugging Tools
Debugging tools can make your life easier. Here are some popular ones:
Tool Name | Description |
---|---|
GDB | A powerful debugger for C/C++ code. |
Chrome DevTools | Great for debugging web applications. |
Visual Studio Debugger | Integrated with Visual Studio for .NET applications. |
Remember, debugging is a skill that improves with practice. The more you debug, the better you’ll get at it!
Working on Coding Projects
Choosing a Project to Start With
When you begin coding, selecting the right project is crucial. Here are some ideas to help you get started:
- A simple calculator that performs basic math operations.
- A to-do list app to manage daily tasks.
- A personal website to showcase your skills.
Breaking Down a Project into Manageable Tasks
Once you have a project in mind, break it down into smaller tasks. This makes it easier to manage and complete. Consider these steps:
- Outline the main features you want.
- Divide the project into smaller parts.
- Set deadlines for each part to stay on track.
Collaborating with Others on Coding Projects
Working with others can enhance your learning experience. Here are some benefits of collaboration:
- You can learn new coding techniques from peers.
- It helps you stay motivated and accountable.
- You can share ideas and get feedback on your work.
Remember, coding projects are not just about writing code; they are about gaining experience and building something you can show to others. Completing projects can also be the fastest way to learn coding and actually get a job. Stay dedicated and keep pushing forward!
Continuing Your Coding Education
Online Resources and Tutorials
There are many free coding courses available online today. These resources help people learn coding without financial barriers. Here are some popular options:
- Codecademy: Offers interactive lessons in various programming languages.
- freeCodeCamp: A nonprofit that provides free coding classes and projects.
- Khan Academy: Great for learning the basics of programming.
Books and Ebooks for Coders
Books can be a valuable resource for deepening your understanding of coding. Here are a few recommended titles:
- "Eloquent JavaScript" by Marijn Haverbeke
- "Python Crash Course" by Eric Matthes
- "Clean Code" by Robert C. Martin
Joining Coding Communities and Forums
Connecting with others can enhance your learning experience. Consider joining:
- Stack Overflow: A platform for asking coding questions and sharing knowledge.
- GitHub: Collaborate on projects and learn from others’ code.
- Discord servers: Many communities focus on coding topics.
Remember: The best coders are always learning and practicing. Keep coding every day to improve your skills!
Highlighted Resource
If you’re looking for structured learning, check out the best coding bootcamps online of 2024. These programs can help you gain essential skills quickly and effectively.
By exploring these resources, you can continue your coding education and stay updated with the latest trends in technology.
Exploring Advanced Topics in Coding
Introduction to Object-Oriented Programming
Object-Oriented Programming (OOP) is a programming style that uses objects to represent data and methods. This approach helps in organizing code and making it more manageable. Key concepts include:
- Classes: Blueprints for creating objects.
- Objects: Instances of classes that hold data.
- Inheritance: Mechanism to create new classes based on existing ones.
Understanding Functional Programming
Functional Programming is a style that treats computation as the evaluation of mathematical functions. It emphasizes:
- Immutability: Data cannot be changed after it is created.
- First-Class Functions: Functions can be passed around as arguments.
- Higher-Order Functions: Functions that can take other functions as inputs.
Exploring Low-Code and No-Code Platforms
Low-Code and No-Code platforms allow users to create applications with minimal coding. They are great for:
- Rapid Development: Quickly build applications without extensive coding.
- Accessibility: Enable non-programmers to create software.
- Integration: Easily connect with other services and tools.
In today’s tech world, understanding these advanced topics can greatly enhance your coding skills and open up new opportunities.
Conclusion
Mastering these advanced topics can significantly improve your coding abilities and prepare you for more complex projects. Keep exploring and practicing!
Preparing for Coding Interviews
Common Interview Questions and Problems
When preparing for coding interviews, it’s essential to familiarize yourself with the types of questions you might face. Here are some common areas to focus on:
- Data Structures: Understand arrays, linked lists, stacks, and queues.
- Algorithms: Be ready to solve problems involving sorting and searching.
- System Design: Know how to design scalable systems.
Tips for Technical Interviews
To excel in your coding interviews, consider these tips:
- Practice Regularly: Use platforms like LeetCode or HackerRank to sharpen your skills.
- Mock Interviews: Conduct practice interviews with friends or use online services.
- Stay Calm: Take deep breaths and think through problems logically.
Resources for Interview Preparation
Utilizing the right resources can make a big difference. Here are some helpful tools:
- Books: "Cracking the Coding Interview" is a popular choice.
- Online Courses: Websites like Coursera offer courses on coding interviews.
- Coding Communities: Join forums like Stack Overflow to ask questions and share knowledge.
Remember, coding interviews are not just about getting the right answer; they also assess your problem-solving process.
By preparing effectively, you can boost your confidence and improve your chances of success in coding interviews. Mastering the basics of coding interviews is crucial for your career.
Getting ready for coding interviews can feel overwhelming, but it doesn’t have to be! With the right tools and guidance, you can boost your skills and confidence. Visit our website to start your journey and learn how to tackle coding challenges effectively. Don’t wait—take the first step towards your dream job today!
Conclusion
Learning the basics of coding is a journey that never really ends. By concentrating on the key ideas discussed in this guide and getting hands-on with the tools and resources available, you can create a strong base for your coding future. Remember, every expert coder started where you are now. Stay curious, keep practicing, and don’t be afraid to ask for help when you need it. With time and effort, you’ll find yourself becoming more confident and skilled in coding.
Frequently Asked Questions
What is coding and why is it important?
Coding is the process of writing instructions for computers to follow. It helps us create software, websites, and apps that make our lives easier. Understanding coding is important because it allows you to solve problems and create technology.
Do I need a special background to start coding?
No, you don’t need any special background to learn coding. Anyone can start learning, no matter their age or experience level!
What programming language should I learn first?
A good first language to learn is Python. It’s easy to understand and widely used, making it great for beginners.
How long does it take to learn coding?
The time it takes to learn coding varies. Some people can grasp the basics in a few weeks, while others may take months. The key is to practice regularly.
What are some common mistakes beginners make?
Beginners often rush through learning the basics or skip important concepts. It’s important to take your time and understand each topic fully.
How can I improve my coding skills?
You can improve your coding skills by practicing regularly, working on projects, and seeking feedback from others. Joining coding communities can also help.
What resources are available for learning to code?
There are many resources available, including online courses, tutorials, books, and coding boot camps. Websites like Codecademy and freeCodeCamp are great places to start.
What should I do if I get stuck while coding?
If you get stuck, take a break and come back to it later. You can also search online for solutions, ask for help in coding forums, or review your notes.