Mastering Data Structures and Algorithms: A Comprehensive Guide for Beginners
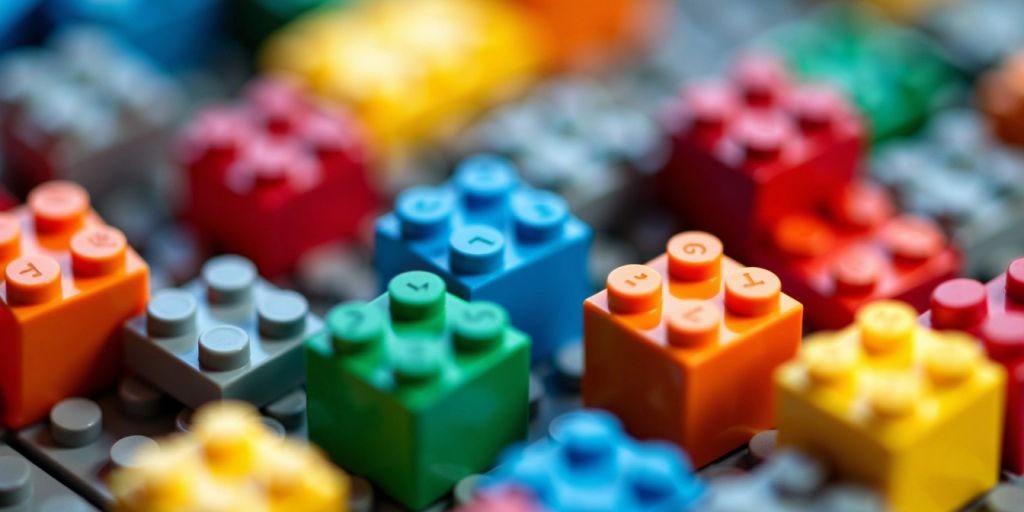
Learning about data structures and algorithms is essential for anyone interested in programming. These concepts help you organize data and solve problems effectively. This guide will take you through the basics, essential types, and practical applications, making it easier to understand and use these important tools in coding.
Key Takeaways
- Data structures help organize data efficiently, making it easier to access and modify.
- Algorithms are step-by-step processes for solving problems and performing tasks.
- Understanding complexity helps you choose the best algorithm for your needs.
- Sorting and searching algorithms are crucial for managing and finding data quickly.
- Dynamic programming is a powerful technique for solving complex problems by breaking them down into smaller parts.
Understanding the Basics of Data Structures and Algorithms
What Are Data Structures?
Data structures are the fundamental building blocks of computer programming. They define how data is organized, stored, and manipulated within a program. Here are some common types of data structures:
- Arrays: A collection of elements of the same type stored in contiguous memory locations.
- Linked Lists: A dynamic collection where each element points to the next.
- Stacks: A Last-In-First-Out (LIFO) structure where elements are added and removed from the top.
- Queues: A First-In-First-Out (FIFO) structure where elements are added to the end and removed from the front.
What Are Algorithms?
An algorithm is a set of instructions designed to solve a specific problem or perform a task. It takes input, processes it, and produces output. Common types of algorithms include:
- Sorting Algorithms: Arrange elements in a specific order.
- Searching Algorithms: Find specific elements in a data structure.
- Graph Algorithms: Traverse and manipulate graph data structures.
Importance of Data Structures and Algorithms
Understanding data structures and algorithms is crucial for writing efficient and scalable code. They help in:
- Optimizing performance: Choosing the right data structure can significantly improve the speed of your program.
- Problem-solving: Algorithms provide a systematic way to approach and solve problems.
- Technical interviews: Many coding interviews focus on your knowledge of data structures and algorithms.
Mastering data structures and algorithms is essential for any aspiring programmer. It not only enhances your coding skills but also prepares you for real-world challenges.
Essential Data Structures Every Programmer Should Know
Understanding data structures is vital for any programmer. They help in organizing and storing data efficiently. Here are some key data structures every programmer should be familiar with:
Arrays and Their Applications
Arrays are collections of items stored at contiguous memory locations. They allow quick access to elements using an index. Arrays are fundamental for many algorithms and applications, such as:
- Storing lists of items
- Implementing other data structures like stacks and queues
- Performing mathematical computations
Linked Lists and Their Variants
Linked lists consist of nodes, where each node contains data and a pointer to the next node. They are useful for:
- Dynamic memory allocation
- Inserting and deleting elements efficiently
- Implementing stacks and queues
Stacks and Queues: LIFO and FIFO Structures
Stacks and queues are essential for managing data in specific orders:
- Stacks (Last In, First Out) are used in:
- Undo mechanisms in software
- Function call management in programming
- Queues (First In, First Out) are used in:
- Task scheduling
- Managing requests in servers
Trees and Graphs: Hierarchical and Networked Data
Trees and graphs are more complex structures:
- Trees are used for:
- Hierarchical data representation (like file systems)
- Efficient searching and sorting (like binary search trees)
- Graphs are used for:
- Representing networks (like social networks)
- Solving problems like shortest path and connectivity
Understanding these data structures is crucial for solving complex problems efficiently. They form the backbone of many algorithms and applications in programming.
By mastering these essential data structures, you will be better equipped to tackle various programming challenges and improve your coding skills. Remember, knowing the right data structure can make a significant difference in your programming journey!
Algorithmic Fundamentals: Building Blocks of Problem Solving
Understanding Algorithm Complexity
Algorithm complexity helps us understand how efficient an algorithm is. It measures how the time or space needed by an algorithm grows as the input size increases. Knowing this is crucial for optimizing code. Here are two main types of complexity:
- Time Complexity: How long an algorithm takes to run.
- Space Complexity: How much memory an algorithm uses.
Big O Notation: Measuring Efficiency
Big O notation is a way to express the efficiency of an algorithm. It describes the worst-case scenario of an algorithm’s performance. Here are some common Big O notations:
Notation | Description |
---|---|
O(1) | Constant time |
O(n) | Linear time |
O(n^2) | Quadratic time |
O(log n) | Logarithmic time |
Common Algorithmic Paradigms
There are several key strategies for solving problems with algorithms. Here are three important ones:
- Divide and Conquer: Break a problem into smaller parts, solve each part, and combine the results.
- Dynamic Programming: Solve complex problems by breaking them down into simpler subproblems and storing their solutions.
- Greedy Algorithms: Make the best choice at each step, hoping to find the global optimum.
Understanding these paradigms is essential for developing effective solutions to various problems.
In summary, mastering these algorithmic fundamentals will greatly enhance your problem-solving skills and prepare you for more complex challenges in programming.
Sorting and Searching Algorithms
Introduction to Sorting Algorithms
Sorting algorithms are essential tools in programming. A sorting algorithm is used to rearrange a given array or list of elements according to a comparison operator on the elements. Here are some common sorting algorithms:
- Bubble Sort: Repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order.
- Merge Sort: Divides the array into halves, sorts them, and then merges them back together.
- Quick Sort: Selects a ‘pivot’ element and partitions the other elements into two sub-arrays according to whether they are less than or greater than the pivot.
Common Sorting Techniques: Quick Sort, Merge Sort, and More
Sorting Technique | Time Complexity (Best) | Time Complexity (Worst) |
---|---|---|
Bubble Sort | O(n) | O(n^2) |
Merge Sort | O(n log n) | O(n log n) |
Quick Sort | O(n log n) | O(n^2) |
Efficient Searching Methods: Binary Search and Beyond
Searching algorithms help find specific elements in data. Here are two popular methods:
- Linear Search: Checks each element until the desired one is found.
- Binary Search: Efficiently finds an element in a sorted array by repeatedly dividing the search interval in half.
Searching algorithms are crucial for quickly locating data in large datasets. Understanding these methods can greatly enhance your programming skills.
Mastering Dynamic Programming
Principles of Dynamic Programming
Dynamic programming, often called DP, is a powerful technique used to solve problems by breaking them down into smaller, manageable parts. This method is especially useful for problems that can be divided into overlapping subproblems. Here are some key principles:
- Optimal Substructure: The optimal solution to a problem can be constructed from optimal solutions of its subproblems.
- Overlapping Subproblems: The problem can be broken down into subproblems that are reused several times.
- Memoization: Storing the results of expensive function calls and reusing them when the same inputs occur again.
Common Dynamic Programming Problems
Here are some classic problems that can be solved using dynamic programming:
- Fibonacci Sequence: Finding the nth Fibonacci number efficiently.
- Knapsack Problem: Maximizing the total value in a knapsack without exceeding its capacity.
- Longest Common Subsequence: Finding the longest sequence that can appear in the same order in both strings.
Optimization Techniques in Dynamic Programming
To improve the efficiency of dynamic programming solutions, consider these techniques:
- Bottom-Up Approach: Start solving from the smallest subproblems and build up to the larger problem.
- Space Optimization: Instead of storing all subproblem results, keep only the necessary ones to save memory.
- Iterative vs. Recursive: Use iterative methods to avoid the overhead of recursive calls.
Dynamic programming is a method used in mathematics and computer science to solve complex problems by breaking them down into simpler subproblems.
By mastering these concepts, you can tackle a wide range of problems more effectively and efficiently!
Graph Algorithms: Navigating Networks
Basics of Graph Theory
Graphs are made up of vertices (or nodes) connected by edges. They can be represented in different ways, such as using adjacency lists or matrices. Understanding how to represent and manipulate graphs is crucial for solving many problems in computer science.
Graph Traversal Techniques: BFS and DFS
Graph traversal is the process of visiting each vertex in a graph. Think of it as navigating through a network of nodes, where each node represents a point of interest. The two main techniques for traversing graphs are:
- Breadth-First Search (BFS): Explores all neighbors at the present depth prior to moving on to nodes at the next depth level.
- Depth-First Search (DFS): Explores as far as possible along each branch before backtracking.
Advanced Graph Algorithms: Shortest Path and Cycle Detection
Graph algorithms can solve complex problems, such as finding the shortest path between two nodes or detecting cycles in a graph. Here are some common algorithms:
- Dijkstra’s Algorithm: Finds the shortest path from a starting node to all other nodes in a weighted graph.
- Bellman-Ford Algorithm: Computes shortest paths from a single source vertex to all other vertices in a graph.
- Floyd-Warshall Algorithm: A dynamic programming approach to find shortest paths between all pairs of vertices.
Understanding graph algorithms is essential for solving real-world problems, such as routing and network analysis.
Summary
Graph algorithms are powerful tools for navigating complex networks. By mastering these techniques, you can tackle a wide range of problems in computer science and beyond.
String Algorithms: Manipulating Text Data
Introduction to String Algorithms
Strings are a fundamental data structure in programming. They are used to store and manipulate text data, making them versatile for various applications. Understanding string algorithms is essential for tasks like searching, sorting, and transforming text.
Pattern Matching Techniques
Pattern matching is a crucial aspect of string algorithms. Here are some common techniques:
- Naive Search: A straightforward method that checks for a pattern in every possible position.
- Knuth-Morris-Pratt (KMP): An efficient algorithm that avoids unnecessary comparisons by using previously matched characters.
- Rabin-Karp: Utilizes hashing to find patterns quickly, especially useful for multiple pattern searches.
String Transformation and Manipulation
Manipulating strings involves various operations. Here are some common transformations:
- Concatenation: Joining two or more strings together.
- Substring Extraction: Retrieving a part of a string based on specified indices.
- Replacement: Changing specific characters or sequences within a string.
String algorithms are not just about searching; they also enable powerful transformations that can enhance data processing.
Conclusion
Mastering string algorithms is vital for any programmer. They provide the tools needed to handle text data efficiently, making them indispensable in software development and data analysis.
Practical Applications of Data Structures and Algorithms
Data structures and algorithms are not just theoretical concepts; they have real-life applications that impact our daily lives. Understanding these applications can help you appreciate their importance in programming and software development.
Real-World Use Cases in Software Development
- Web Development: Data structures like arrays and hash tables are used to manage user data and session information efficiently.
- Database Management: Algorithms help in sorting and searching through large datasets, ensuring quick access to information.
- Game Development: Trees and graphs are used to create game maps and manage character movements.
Applications in Machine Learning and AI
- Data Preprocessing: Algorithms are used to clean and organize data before analysis.
- Model Training: Efficient data structures help in managing large datasets during the training of machine learning models.
- Pattern Recognition: String algorithms are crucial for tasks like spam email detection, plagiarism detection, and search engines.
Data Structures and Algorithms in Competitive Programming
- Problem Solving: Competitors use various data structures to solve problems quickly and efficiently.
- Time Management: Understanding algorithm complexity helps in optimizing solutions within time limits.
- Collaboration: Many platforms allow programmers to share solutions and learn from each other, enhancing their skills.
In summary, mastering data structures and algorithms is essential for anyone looking to excel in programming. They are the backbone of many applications we use every day, from search engines to digital forensics. Understanding their practical applications can significantly enhance your coding skills and problem-solving abilities.
Tools and Resources for Learning Data Structures and Algorithms
Recommended Books and Online Courses
To effectively learn data structures and algorithms, consider the following resources:
- Books:
- Online Courses:
Coding Practice Platforms
Practicing coding is essential for mastering DSA. Here are some platforms to help you:
- LeetCode: Great for practicing interview questions.
- HackerRank: Offers challenges across various domains.
- CodeSignal: Focuses on coding assessments and competitions.
Communities and Forums for Peer Learning
Engaging with others can enhance your learning experience. Join these communities:
- Reddit: r/algorithms for discussions and resources.
- Stack Overflow: Ask questions and share knowledge.
- GeeksforGeeks: A vast resource for tutorials and articles.
Learning DSA is a journey that requires consistent practice and engagement with the community. Don’t hesitate to seek help and share your knowledge!
Tips for Excelling in Technical Interviews
Common Interview Questions on Data Structures and Algorithms
When preparing for technical interviews, it’s essential to familiarize yourself with common questions. Here are some frequently asked topics:
- Arrays and Strings: Manipulation and searching techniques.
- Linked Lists: Reversal and cycle detection.
- Trees and Graphs: Traversal methods and shortest path algorithms.
Strategies for Effective Problem Solving
To tackle problems efficiently during interviews, consider these strategies:
- Understand the Problem: Take time to read the question carefully.
- Plan Your Approach: Outline your solution before coding.
- Code and Test: Write your code and test it with sample inputs.
Mock Interviews and Practice Sessions
Engaging in mock interviews can significantly boost your confidence. Here are some tips:
- Simulate Real Conditions: Practice in a timed environment.
- Seek Feedback: Get insights from peers or mentors.
- Review Your Performance: Analyze mistakes to improve.
Remember, consistent practice is key to mastering data structures and algorithms. To make your interview preparation more effective, consider enrolling in the DSA to Development – Coding Guide course by GeeksforGeeks. This course offers a structured approach to learning and practicing essential concepts.
If you want to ace your technical interviews, start by practicing coding problems regularly. Focus on understanding algorithms and data structures, as they are key to solving many interview questions. Don’t forget to check out our website for free resources and interactive tutorials that can help you improve your skills and boost your confidence. Visit us today and take the first step towards your dream job!
Conclusion
Learning about data structures and algorithms can be tough, but it’s also very rewarding. With the right attitude, helpful resources, and a clear plan, you can create a solid base in this area and get better at solving tricky problems. Whether you want to compete in programming contests or get ready for job interviews, this guide will help you on your learning journey. Always remember, practice is super important—so keep coding, keep learning, and don’t lose hope!
Frequently Asked Questions
What are data structures?
Data structures are ways to organize and store data in a computer. They help in managing and accessing data efficiently.
Why are algorithms important?
Algorithms are step-by-step instructions for solving problems. They are crucial for performing tasks effectively and efficiently.
How do I start learning data structures and algorithms?
Begin with basic programming skills. Then, explore simple data structures like arrays and practice writing algorithms.
What is Big O notation?
Big O notation is a way to describe how the time or space needed for an algorithm grows as the size of the input increases.
Can I learn data structures and algorithms online?
Yes! There are many online courses and platforms where you can learn about data structures and algorithms.
What are some common data structures?
Common data structures include arrays, linked lists, stacks, queues, trees, and graphs.
How do data structures relate to coding interviews?
Many coding interviews test your knowledge of data structures and algorithms to see how well you solve problems.
What resources are available for learning?
You can find books, online courses, and coding practice platforms to help you learn data structures and algorithms.