Mastering Python: Essential Skills to Learn Python for Interviews
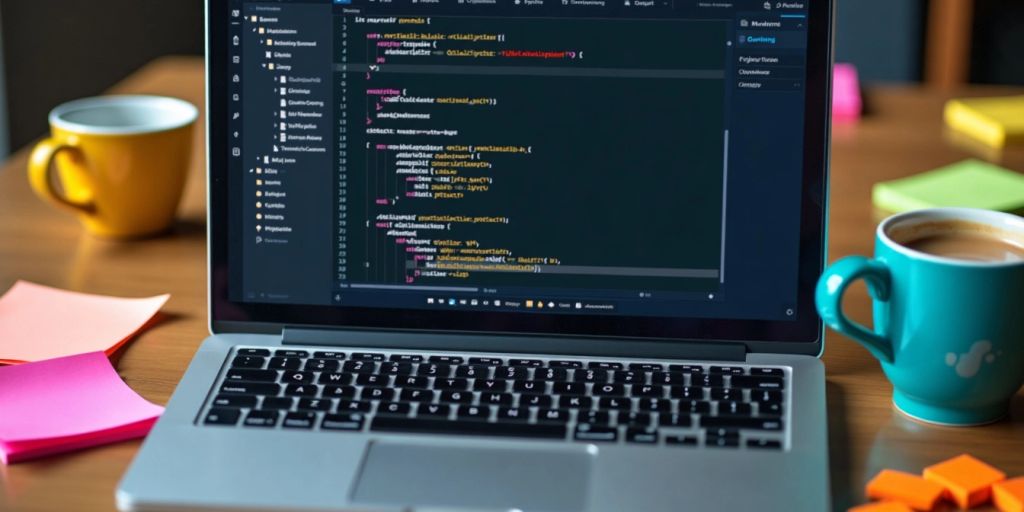
Python is a key programming language in the tech world today. Its user-friendly nature and wide-ranging applications make it a top choice for many developers. Whether you’re just starting out or looking to advance your skills, understanding Python is essential for acing coding interviews. This article will guide you through the important skills and concepts you need to master for successful interviews.
Key Takeaways
- Python is widely used in many industries, making it essential for coding interviews.
- Mastering Python basics like syntax, data structures, and functions is crucial for success.
- Intermediate skills such as error handling and object-oriented programming are important for advanced roles.
- Familiarity with popular Python libraries can give you an edge in interviews.
- Practicing coding problems and communicating clearly during interviews is key to performing well.
Understanding Python’s Role in Coding Interviews
Why Python is Popular in Interviews
Python is a favorite in coding interviews because of its simple syntax and versatility. Many companies prefer candidates who can use Python to solve problems quickly. Here are some reasons why Python stands out:
- Easy to Learn: Python’s straightforward syntax makes it accessible for beginners.
- Wide Usage: It’s used in various fields like web development, data science, and artificial intelligence.
- Strong Community: A large community means plenty of resources and libraries are available.
Industries That Use Python
Python is not just popular in tech; it’s used across many industries. Here’s a quick look:
Industry | Use Cases |
---|---|
Web Development | Building websites and applications |
Data Science | Analyzing data and machine learning |
Finance | Algorithmic trading and analysis |
Education | Teaching programming concepts |
Game Development | Creating games and simulations |
Key Python Skills for Interviews
To succeed in Python interviews, you should focus on these essential skills:
- Basic Syntax: Understanding variables, data types, and operations is crucial. Mastering Python’s basic syntax is fundamental for any coding interview.
- Data Structures: Be familiar with lists, dictionaries, sets, and tuples.
- Problem-Solving: Practice solving coding problems to improve your skills.
Mastering Python is not just about knowing the language; it’s about applying it effectively to solve real-world problems.
By focusing on these areas, you can prepare yourself for a successful coding interview experience.
Mastering Python Basics for Interviews
Essential Syntax and Operations
Understanding the basic syntax of Python is crucial for any coding interview. This includes:
- Variables and data types
- Basic operations like addition and subtraction
- Control flow statements such as if-else
Working with Lists and Dictionaries
Lists and dictionaries are fundamental data structures in Python. Here are some common operations:
- Adding and removing elements
- Searching for items
- Iterating through elements
Operation | List Example | Dictionary Example |
---|---|---|
Add | my_list.append(5) |
my_dict['key'] = 1 |
Remove | my_list.remove(3) |
del my_dict['key'] |
Access | my_list[0] |
my_dict['key'] |
String Manipulation Techniques
String manipulation is another key area. Common tasks include:
- Reversing a string
- Finding substrings
- Changing case (upper/lower)
Mastering these basics will help you tackle more complex problems in interviews. Practice makes perfect!
Intermediate Python Concepts to Know
Error Handling and Exceptions
In Python, error handling is crucial for writing reliable code. You can use try
and except
blocks to manage errors gracefully. Here are some key points to remember:
- Use
try
to wrap code that might cause an error. - Use
except
to define how to handle the error. - Always consider using
finally
to execute code regardless of whether an error occurred.
Object-Oriented Programming
Object-oriented programming (OOP) helps you organize your code better. Here are some important OOP concepts:
- Classes: Blueprints for creating objects.
- Objects: Instances of classes.
- Inheritance: Allows a class to inherit properties from another class.
- Polymorphism: Lets you use the same method name for different types.
Using Python Libraries
Python has many libraries that make coding easier. Here are some popular ones:
- NumPy: For numerical computing.
- Pandas: For data manipulation.
- Matplotlib: For data visualization.
Learning these intermediate concepts will help you tackle a variety of problems in Python. Mastering these skills is essential for success in coding interviews.
Understanding these intermediate concepts will prepare you for more complex challenges in Python programming. They are essential for building a strong foundation as you advance in your coding journey. Don’t forget to practice these skills regularly!
Advanced Python Topics for Senior-Level Interviews
Advanced Data Structures
Understanding advanced data structures is crucial for senior-level interviews. You should be familiar with:
- Sets: Unordered collections of unique elements.
- Tuples: Immutable sequences that can hold mixed data types.
- Linked Lists: Data structures consisting of nodes that point to the next node.
File Handling in Python
File handling is essential for many applications. Key points include:
- Reading files: Use
open()
to read data from files. - Writing files: Use
write()
to save data. - File permissions: Understand how to manage access rights.
Operation | Method | Description |
---|---|---|
Read | open('file.txt', 'r') |
Opens a file for reading. |
Write | open('file.txt', 'w') |
Opens a file for writing. |
Append | open('file.txt', 'a') |
Opens a file for appending. |
Optimization and Performance Tuning
Optimizing your code can make a big difference. Consider:
- Algorithm efficiency: Analyze time and space complexity.
- Memory management: Use tools like
gc
to manage memory. - Profiling: Use modules like
cProfile
to identify bottlenecks.
Mastering these advanced topics can significantly enhance your coding skills and prepare you for challenging interview questions. Understanding these concepts will set you apart from other candidates.
Common Advanced Python Interview Questions
Be prepared for questions like:
- What are the differences between lists and tuples?
- How do you handle exceptions in Python?
- Can you explain the concept of decorators?
These topics are essential for demonstrating your expertise in Python during interviews, especially for senior positions. Familiarity with these concepts will help you tackle complex problems effectively.
Common Python Coding Interview Questions
Junior-Level Questions
Junior-level questions usually focus on basic concepts. Here are some common topics:
- Basic Syntax and Operations: Understanding variables, data types, and simple operations.
- List and Dictionary Operations: Manipulating lists and dictionaries, such as adding or removing items.
- String Manipulations: Tasks like reversing a string or finding substrings.
Senior-Level Questions
For senior positions, expect more complex questions:
- Advanced Data Structures: Knowledge of sets, tuples, and linked lists.
- Object-Oriented Programming: Understanding classes, inheritance, and polymorphism.
- Error Handling: Using try-except blocks to manage exceptions.
Case Study Questions
These questions present real-world problems that require practical solutions. Candidates must:
- Analyze the problem.
- Design a solution using Python.
- Implement and test the solution.
Remember: Practicing these questions can greatly improve your confidence and skills for interviews. Familiarity with the 23 top Python interview questions & answers for 2024 can be particularly helpful for job seekers and students alike.
Effective Problem-Solving Strategies
Breaking Down Problems
When faced with a coding challenge, it’s important to break down the problem into smaller, manageable parts. This helps in understanding the requirements better. Here are some steps to follow:
- Read the problem statement carefully. Make sure you understand what is being asked.
- Identify the inputs and outputs. Knowing what you have and what you need to produce is crucial.
- Break the problem into smaller tasks. This makes it easier to tackle each part one at a time.
Writing Efficient Code
Efficiency is key in coding interviews. Here are some tips to write efficient code:
- Use built-in functions. Python has many built-in functions that can save time and effort.
- Optimize your algorithms. Always think about the time and space complexity of your solution.
- Avoid unnecessary computations. If you can store results and reuse them, do it!
Testing and Debugging
Testing your code is essential to ensure it works as expected. Here’s how to approach it:
- Test with different inputs. Use edge cases and normal cases to see how your code performs.
- Debug systematically. If something goes wrong, check your code step by step to find the issue.
- Use print statements. They can help you understand what your code is doing at each stage.
Remember, advanced python coding challenges help you hone your problem-solving skills and improve your python fundamentals so that you can prepare for and ace any interview.
By following these strategies, you can enhance your problem-solving skills and increase your chances of success in coding interviews.
Practical Python Projects for Interview Preparation
Building Real-World Applications
Working on real-world applications is a great way to prepare for interviews. Here are some project ideas:
- To-Do List App: Create a simple app to manage tasks.
- Weather App: Use an API to fetch and display weather data.
- Blog Platform: Build a basic blog where users can post and comment.
Data Science Projects
Data science is a popular field that often requires Python skills. Consider these projects:
- Data Analysis: Analyze a dataset using Pandas and visualize it with Matplotlib.
- Machine Learning Model: Build a simple model using Scikit-learn.
- Web Scraper: Create a script to scrape data from websites.
Web Development Projects
Web development is another area where Python shines. Here are some ideas:
- Flask Web App: Develop a small web application using Flask.
- Django Project: Create a more complex application with Django.
- API Development: Build a RESTful API for a service.
These Python projects are designed to help you gain practical experience with Python programming while building real-world applications.
Tips for Acing Python Coding Interviews
Understanding the Problem Statement
When you get a coding question, take your time to read it carefully. Make sure you understand what is being asked before jumping into coding. Clarifying any doubts with the interviewer can help you avoid mistakes.
Communicating Your Thought Process
As you work through the problem, explain your thought process out loud. This shows the interviewer how you think and helps them understand your approach. It’s important to keep them in the loop about your reasoning.
Handling Stress and Time Management
Coding interviews can be stressful. Here are some tips to manage your time and stress:
- Stay calm: Take deep breaths if you feel anxious.
- Pace yourself: Don’t rush; focus on solving the problem step by step.
- Practice: The more you practice, the more comfortable you will feel during the interview.
Remember, the goal is not just to solve the problem but to demonstrate your coding skills and problem-solving abilities.
Last-Minute Coding Interview Tips
Here are some last-minute tips to help you nail your interview:
- Revise the basic algorithms.
- Review the basic data structures.
- Practice the areas that you are good at.
- Prepare responses to behavioral questions.
By following these tips, you can approach your Python coding interview with confidence and clarity.
Resources for Learning Python for Interviews
Online Courses and Tutorials
There are many great resources available online to help you learn Python. Here are some popular options:
- Coursera: Offers courses from universities and companies.
- edX: Provides a variety of Python courses.
- Udacity: Known for its comprehensive technical courses.
Coding Practice Platforms
Practicing coding problems is essential for interview preparation. Here are some platforms to consider:
- LeetCode: Focuses on coding challenges and interview questions.
- HackerRank: Offers a wide range of coding problems.
- Codewars: Provides a fun way to improve your coding skills.
Books and Articles
Reading books and articles can deepen your understanding of Python. Here are some recommendations:
- "Automate the Boring Stuff with Python": A practical guide for beginners.
- "Python Crash Course": A fast-paced introduction to Python.
- "Fluent Python": For those looking to master Python.
Learning Python is not just about coding; it’s about understanding how to solve problems effectively. Practice regularly to build your skills and confidence.
Highlighted Resource
For beginners, consider looking for free Python courses online. One such course covers the fundamentals of Python 3.6 and Anaconda 5.0.0 using Jupyter Notebook, taking around seven hours to complete.
Common Mistakes to Avoid in Python Interviews
Neglecting Edge Cases
One of the biggest mistakes candidates make is overlooking edge cases. These are special conditions that might not be obvious but can cause your code to fail. Always think about:
- Empty inputs
- Very large or very small numbers
- Unexpected data types
Overcomplicating Solutions
Another common error is making solutions more complex than necessary. Simplicity is key! Aim for clarity and efficiency. Here are some tips:
- Use built-in functions whenever possible.
- Avoid unnecessary loops or conditions.
- Stick to straightforward algorithms.
Ignoring Python’s Built-in Functions
Many candidates forget to utilize Python’s powerful built-in functions. These can save time and make your code cleaner. Some important functions include:
len()
for lengthsum()
for totalssorted()
for ordering
Remember, the goal is to write clean and efficient code. Always test your solutions thoroughly before presenting them in an interview.
By avoiding these common mistakes, you can improve your chances of success in Python coding interviews!
Utilizing Python’s Built-in Functions and Libraries
Popular Libraries to Know
Python has many libraries that help make coding easier. Here are some important ones:
- NumPy: Great for numerical calculations.
- Pandas: Useful for data manipulation and analysis.
- Matplotlib: Helps in creating visualizations.
These libraries are collections of modules and packages that provide pre-written code to perform various tasks. They help simplify coding by providing ready-to-use functions.
Built-in Functions for Efficiency
Python comes with many built-in functions that can save you time. Here are a few:
- len(): Returns the length of an object.
- max(): Finds the largest item in an iterable.
- sum(): Adds up all the items in an iterable.
Using these functions can make your code cleaner and faster.
Practical Examples and Use Cases
Here are some examples of how to use Python’s built-in functions and libraries:
- Using NumPy to calculate the mean of a list:
import numpy as np data = [1, 2, 3, 4, 5] mean = np.mean(data) print(mean) # Output: 3.0
- Using Pandas to read a CSV file:
import pandas as pd df = pd.read_csv('data.csv') print(df.head())
Python’s built-in functions and libraries are essential tools that can help you write better code and solve problems more efficiently.
By mastering these tools, you can enhance your coding skills and prepare effectively for interviews.
Python has many built-in tools that can make coding easier and faster. By using these functions and libraries, you can solve problems more efficiently. If you’re eager to learn more about coding and improve your skills, visit our website today!
Final Thoughts on Python Interview Preparation
Getting ready for Python coding interviews means mixing what you know with hands-on practice. Focus on different question types, from simple rules to tricky problems, and practice often. This way, you’ll feel ready and sure of yourself when the big day comes. Don’t forget, it’s not just about finding the right answers; explaining how you think is just as important.
Frequently Asked Questions
What can I expect during a Python coding interview?
You will likely face a mix of questions. These can range from basic syntax and data structures to more complex problem-solving tasks. Be ready for live coding sessions and scenarios where you’ll need to think on your feet.
How should I prepare for a Python coding interview?
Practice coding problems regularly. Review both the basics and advanced topics in Python. Working on real-world projects can also help you improve your problem-solving skills.
Are there key Python libraries I should know for interviews?
Yes, it’s helpful to be familiar with popular libraries like NumPy, Pandas, Django, and Flask. Knowing these can give you an advantage, especially for senior-level positions.
What mistakes should I avoid during a coding interview?
Common mistakes include not reading the problem carefully, ignoring edge cases, and failing to test your code thoroughly. Clear communication with the interviewer is also very important.
How crucial is it to know Python’s built-in functions?
It’s very important! Knowing these functions can help you write cleaner and more efficient code. It also shows that you are skilled in Python.
Can I use an IDE while coding in the interview?
It depends on the company’s rules. Some allow IDEs, while others may ask you to code in a simple text editor or even on a whiteboard.
What types of questions are common in Python interviews?
You can expect questions on basic syntax, data structures, and algorithms. There will also be more complex questions that involve real-world problems.
How can I improve my problem-solving skills in Python?
Try to break down problems into smaller parts. Practice writing efficient code and make sure to test and debug your solutions regularly.