Mastering Dynamic Programming Interview Questions: Strategies for Success
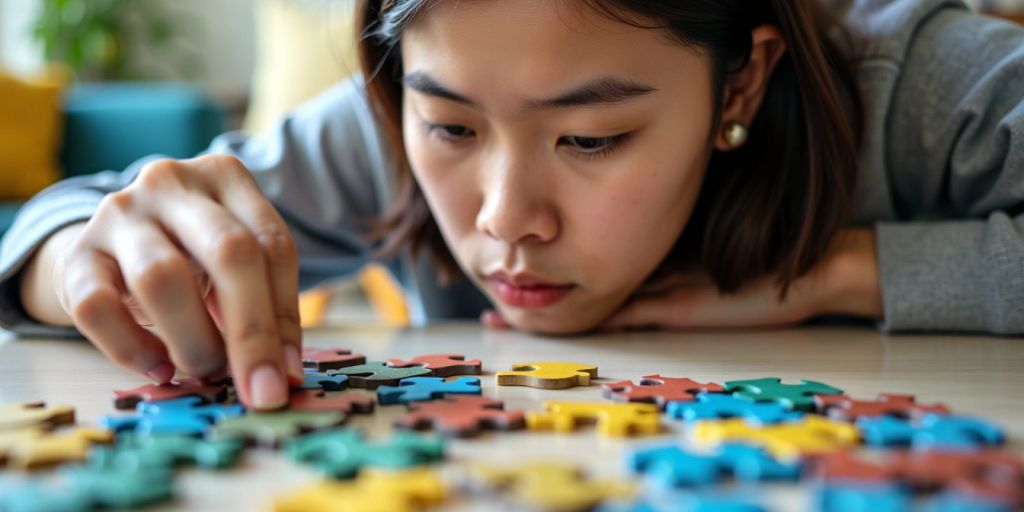
Dynamic Programming (DP) can seem tricky, especially when preparing for coding interviews. However, with the right strategies and practice, anyone can master it. This article will guide you through the basics of DP, how to spot problems in interviews, and effective techniques to tackle them. Whether you’re a beginner or looking to sharpen your skills, these insights will help you succeed in your coding journey.
Key Takeaways
- Understand the basics of dynamic programming to build a strong foundation.
- Practice recognizing DP problems during interviews to improve your chances of success.
- Break down complex problems into smaller parts to make them easier to solve.
- Use online resources and communities for extra practice and support.
- Learn from your mistakes and keep improving your problem-solving skills.
Understanding the Fundamentals of Dynamic Programming
What is Dynamic Programming?
Dynamic programming, often called DP, is a method used in mathematics and computer science to solve complex problems by breaking them down into simpler subproblems. This approach helps in finding solutions efficiently by storing the results of these subproblems to avoid redundant calculations.
Key Concepts: Memoization and Tabulation
There are two main techniques in dynamic programming:
- Memoization: This is a top-down approach where you solve the problem recursively and store the results of subproblems in a table to avoid recalculating them.
- Tabulation: This is a bottom-up approach where you solve all possible subproblems first and use their results to build up solutions to larger problems.
Technique | Description |
---|---|
Memoization | Top-down approach, stores results of subproblems in a table. |
Tabulation | Bottom-up approach, solves all subproblems first before larger problems. |
Common Misconceptions
Many people misunderstand dynamic programming. Here are some common misconceptions:
- DP is only for optimization problems: While many optimization problems use DP, it can also solve counting problems.
- DP is always slow: With proper implementation, DP can be very efficient.
- You need to know everything about recursion: Understanding recursion helps, but it’s not mandatory to start learning DP.
Dynamic programming is not just about solving problems; it’s about solving them efficiently by reusing solutions to subproblems.
By grasping these fundamentals, you can start to recognize when and how to apply dynamic programming techniques effectively in various scenarios.
Identifying Dynamic Programming Problems in Interviews
Recognizing Patterns
Dynamic programming (DP) problems often share common patterns. Here are some key indicators:
- Optimal Substructure: The problem can be broken down into smaller, simpler subproblems.
- Overlapping Subproblems: The same subproblems are solved multiple times.
- Recurrence Relation: A formula that expresses the solution in terms of smaller subproblems.
Common Problem Types
In interviews, you might encounter various types of DP problems. Some of the most common include:
- Knapsack Problems: Such as the 0/1 Knapsack.
- Sequence Problems: Like Longest Common Subsequence.
- Partition Problems: For example, Coin Change.
When to Use Dynamic Programming
Knowing when to apply dynamic programming is crucial. Consider using DP when:
- The problem can be divided into overlapping subproblems.
- You can define a clear recurrence relation.
- You need to optimize for time or space complexity.
Identifying the problem can be solved using DP is the first step to mastering these types of questions. Understanding the variables and expressing the recurrence relation clearly will guide you through the solution process.
Breaking Down Complex Problems into Subproblems
The Importance of Subproblem Overlap
Dynamic programming (DP) is all about breaking down complex problems into smaller, manageable parts called subproblems. Recognizing overlapping subproblems is crucial because it allows you to save time by storing results instead of recalculating them. This is where techniques like memoization come into play, helping you avoid redundant calculations.
State Transition Functions
Understanding how to transition between states is key in dynamic programming. A state transition function defines how to move from one subproblem to another. Here’s a simple way to think about it:
- Identify the current state.
- Determine the possible actions that can be taken from this state.
- Define how these actions lead to new states.
Examples of Subproblem Decomposition
To illustrate how to break down problems, consider the following examples:
- Fibonacci Sequence: Instead of calculating Fibonacci numbers directly, you can break it down into smaller Fibonacci numbers, like
F(n) = F(n-1) + F(n-2)
. - 0/1 Knapsack Problem: Here, you can decide whether to include an item or not, leading to two subproblems for each item.
- Longest Common Subsequence: This can be broken down into comparing characters and finding subsequences in smaller strings.
Remember, the key to mastering dynamic programming is to understand how to break down problems into subproblems effectively. This will not only help you solve DP problems but also build your confidence in tackling complex coding challenges.
Top Dynamic Programming Problems to Practice
Dynamic programming (DP) is a crucial skill for coding interviews. Here are some of the most important problems you should practice:
0/1 Knapsack Problem
- This problem involves selecting items with given weights and values to maximize total value without exceeding a weight limit.
- Key points to remember:
- Each item can either be included or excluded.
- Use a table to keep track of maximum values at each weight limit.
Longest Common Subsequence
- This problem finds the longest sequence that appears in the same relative order in two sequences.
- Important aspects:
- It’s not necessary for the characters to be contiguous.
- Use a 2D array to store lengths of common subsequences.
Coin Change Problem
- The goal is to find the number of ways to make a certain amount using given coin denominations.
- Remember:
- You can use each coin multiple times.
- A table can help track combinations for each amount.
Problem | Description | Key Technique |
---|---|---|
0/1 Knapsack | Maximize value with weight limit | Tabulation |
Longest Common Subsequence | Find longest sequence in two sequences | Memoization |
Coin Change | Count ways to make a certain amount | Dynamic Programming |
Practicing these problems will help you build a strong foundation in dynamic programming. Focus on understanding the key concepts behind each problem to improve your problem-solving skills!
Strategies for Efficient Problem Solving
The FAST Method
The FAST Method is a structured approach to tackle dynamic programming problems effectively. It stands for:
- Familiarize yourself with the problem.
- Analyze the requirements and constraints.
- Solve the problem using a systematic approach.
- Test your solution against various cases.
Top-Down vs. Bottom-Up Approaches
When solving dynamic programming problems, you can choose between two main strategies:
- Top-Down Approach: This involves breaking the problem into smaller subproblems and solving them recursively, often using memoization to store results.
- Bottom-Up Approach: This method builds up solutions from the smallest subproblems, filling out a table iteratively.
Optimizing Space and Time Complexity
Efficiency is key in coding interviews. Here are some tips to optimize your solutions:
- Prioritize Time Complexity: Aim for the fastest solution possible.
- Minimize Space Usage: Use data structures wisely to conserve memory.
- Understand Big O Notation: Familiarize yourself with how to evaluate the efficiency of your algorithms.
Remember, mastering these strategies will not only help you solve problems faster but also make you a more effective coder overall. Dynamic programming can be challenging, but with practice and the right techniques, you can excel in interviews!
Common Pitfalls and How to Avoid Them
Overcomplicating the Problem
One of the biggest mistakes in dynamic programming is overcomplicating the problem. When faced with a challenge, it’s easy to get lost in the details. Here are some tips to simplify your approach:
- Break the problem down into smaller parts.
- Focus on the main goal rather than getting bogged down by every detail.
- Use diagrams or flowcharts to visualize the problem.
Ignoring Base Cases
Another common error is ignoring base cases. Base cases are essential for recursive solutions and dynamic programming. Without them, your solution may not work correctly. To avoid this pitfall:
- Identify the simplest version of the problem.
- Ensure your solution handles these cases before moving on to more complex scenarios.
- Test your solution with these base cases to confirm it works.
Misunderstanding State Transitions
Misunderstanding state transitions can lead to incorrect solutions. It’s crucial to clearly define how you move from one state to another. To prevent this mistake:
- Write down the state transition functions clearly.
- Use examples to illustrate how states change.
- Double-check your transitions against the problem requirements.
Remember, mastering dynamic programming is about practice and understanding. By avoiding these common pitfalls, you can improve your problem-solving skills and perform better in interviews.
Pitfall | Avoidance Strategy |
---|---|
Overcomplicating the Problem | Simplify and break down the problem |
Ignoring Base Cases | Identify and test base cases |
Misunderstanding State Transitions | Clearly define and illustrate transitions |
Leveraging Online Resources for Practice
Best Websites for Practice Problems
When preparing for dynamic programming interviews, utilizing online resources can be incredibly helpful. Here are some top websites to consider:
- LeetCode: Offers a wide range of dynamic programming problems, including almost 100 dedicated to this topic.
- HackerRank: Provides various coding challenges that help sharpen your skills.
- Codewars: A platform where you can solve problems and improve your coding abilities through practice.
Recommended Video Tutorials
Video tutorials can be a great way to visualize concepts. Here are some recommended sources:
- YouTube Channels: Many channels focus on coding interviews and dynamic programming.
- Online Courses: Platforms like Coursera and Udemy offer structured courses on dynamic programming.
- Webinars: Look for live sessions that cover dynamic programming strategies.
Community Forums and Discussions
Engaging with others can enhance your learning experience. Consider these options:
- Reddit: Join subreddits focused on coding interviews to share insights and ask questions.
- Stack Overflow: A great place to seek help on specific problems or concepts.
- Discord Servers: Many coding communities have Discord channels for real-time discussions.
Practice is essential for mastering dynamic programming. By leveraging these resources, you can build a solid foundation and improve your problem-solving skills. Remember, it’s not just about solving problems but understanding the underlying principles that will help you succeed in interviews.
Summary Table of Resources
Resource Type | Examples |
---|---|
Practice Websites | LeetCode, HackerRank, Codewars |
Video Tutorials | YouTube, Coursera, Udemy |
Community Forums | Reddit, Stack Overflow, Discord |
By utilizing these resources effectively, you can enhance your understanding and performance in dynamic programming interviews.
Real-World Applications of Dynamic Programming
Dynamic programming (DP) is not just a theoretical concept; it has many real-world applications that can significantly improve efficiency and decision-making. Here are some key areas where DP is applied:
Dynamic Programming in Game Theory
- Optimal Strategies: DP helps in determining the best strategies in competitive games, allowing players to maximize their chances of winning.
- Game Outcomes: It can predict outcomes based on various moves, making it easier to plan ahead.
- Resource Allocation: Players can use DP to allocate resources effectively during gameplay.
Applications in Data Science
- Predictive Modeling: DP is used in algorithms that predict future trends based on historical data.
- Data Compression: It helps in optimizing data storage by finding the most efficient way to compress information.
- Machine Learning: Many machine learning algorithms utilize DP to improve learning efficiency and accuracy.
Use Cases in Software Development
- Algorithm Optimization: Developers use DP to optimize algorithms, making them faster and more efficient.
- Pathfinding: In applications like GPS, DP helps find the shortest or most efficient routes.
- Resource Management: It assists in managing system resources effectively, ensuring optimal performance.
Dynamic programming can be a game-changer in various fields, allowing for optimal strategies in everything from game theory to software development.
Application Area | Key Benefits |
---|---|
Game Theory | Optimal strategies and outcomes |
Data Science | Predictive modeling and compression |
Software Development | Algorithm optimization and resource management |
By understanding these applications, you can see how mastering dynamic programming can lead to significant advantages in both academic and professional settings.
Preparing for the Interview Day
Mock Interviews
Mock interviews are a great way to prepare. Practice with friends or online partners to simulate real interview conditions. This helps you get used to the pressure and improves your confidence. Here are some tips for effective mock interviews:
- Set a timer to mimic real interview time limits.
- Use a whiteboard or paper to solve problems, just like in an actual interview.
- Focus on explaining your thought process clearly.
Time Management During the Interview
Managing your time well is crucial during interviews. Here are some strategies:
- Break down the problem into smaller parts.
- Allocate specific time for each part of the problem.
- Keep an eye on the clock to ensure you stay on track.
Handling Stress and Anxiety
Feeling nervous before an interview is normal. Here are some ways to manage stress:
- Take deep breaths to calm your nerves.
- Visualize a successful interview experience.
- Remind yourself that it’s okay to make mistakes; they are part of learning.
Preparing for an interview is not just about technical skills; it’s also about building confidence and managing your mindset. Embrace the challenge and view it as an opportunity to grow!
Learning from Mistakes and Iterating
Analyzing Failed Attempts
Learning from mistakes is crucial in mastering dynamic programming. After each practice session or mock interview, take a moment to reflect on what went wrong. Here are some steps to help you analyze your performance:
- Identify Mistakes: Write down the specific areas where you struggled.
- Understand the Reason: Try to figure out why you made those mistakes. Was it a lack of knowledge or a misunderstanding of the problem?
- Plan for Improvement: Create a plan to address these weaknesses in your next practice session.
Iterative Improvement
Improvement is a continuous process. Here are some strategies to help you iterate effectively:
- Set Clear Goals: Define what you want to achieve in each practice session.
- Track Progress: Keep a log of your practice sessions to see how you’re improving over time.
- Seek Feedback: Don’t hesitate to ask peers or mentors for their insights on your approach.
Seeking Feedback from Peers
Engaging with others can provide valuable perspectives. Consider these options:
- Join Study Groups: Collaborate with peers to discuss problems and solutions.
- Participate in Online Forums: Share your experiences and learn from others in the community.
- Request Mock Interviews: Simulate real interview conditions and get constructive feedback.
Remember, learning from mistakes is not a setback; it’s a stepping stone to success. Embrace challenges as opportunities to grow and improve your skills.
By following these strategies, you can turn your mistakes into valuable lessons, ultimately enhancing your problem-solving abilities in dynamic programming. Practicing consistently will help you build confidence and prepare you for real-world coding interviews.
Advanced Techniques and Lesser-Known Problems
Bitmasking in Dynamic Programming
Bitmasking is a clever technique used in dynamic programming to represent subsets of a set using binary numbers. This method is particularly useful for problems involving combinations or subsets. Here are some key points about bitmasking:
- Efficient Representation: Each bit in a binary number can represent whether an element is included in a subset.
- State Management: It allows for compact state representation, making it easier to manage multiple states in a DP solution.
- Common Problems: Problems like the Traveling Salesman Problem can benefit from this technique.
Digit DP
Digit DP is a specialized form of dynamic programming that deals with problems involving numbers and their digits. This technique is useful for counting numbers that satisfy certain properties. Key aspects include:
- State Definition: Define states based on the digits of the number.
- Transition Logic: Create transitions based on the properties you want to check.
- Base Cases: Identify base cases that help in building up the solution.
Dynamic Programming on Trees and Graphs
Dynamic programming can also be applied to tree and graph structures. Here’s how:
- Tree DP: Use depth-first search (DFS) to explore nodes and calculate values based on child nodes.
- Graph DP: Handle problems like finding the shortest path or maximum flow using DP techniques.
- State Representation: States can be represented by nodes and their properties.
Dynamic programming is not just about solving problems; it’s about understanding how to break them down into manageable parts.
Summary Table of Techniques
Technique | Use Case | Key Benefit |
---|---|---|
Bitmasking | Subset problems | Efficient state representation |
Digit DP | Counting numbers with specific properties | Focused on digit manipulation |
Tree/Graph DP | Problems involving hierarchical structures | Leverages structure for optimization |
By mastering these advanced techniques, you can tackle a wider range of dynamic programming problems and enhance your problem-solving skills in interviews. Remember, practice is key to becoming proficient in these methods!
In the world of coding, there are many advanced methods and hidden challenges that can trip you up. If you’re eager to tackle these tricky topics and improve your skills, visit our website today! We offer a range of resources to help you succeed in coding interviews and beyond. Don’t wait—start your journey now!
Wrapping It Up
In conclusion, getting good at Dynamic Programming (DP) is more than just solving a lot of problems. It’s about really understanding the ideas behind it, learning from others, and practicing often. The tips shared by the community can help anyone who wants to get better at DP. As we look ahead to 2025, keep in mind that learning never stops. Don’t be afraid to ask for help, use the resources out there, and most importantly, keep practicing. With hard work and the right approach, you can master DP and boost your problem-solving skills. Happy coding, and good luck on your DP journey!
Frequently Asked Questions
What is dynamic programming?
Dynamic programming is a way to solve complex problems by breaking them down into smaller, simpler parts. It helps to save time by remembering the results of smaller problems, so you don’t have to solve them again.
How can I recognize dynamic programming problems in interviews?
Look for problems that can be divided into overlapping subproblems or that require making decisions at each step. Common examples include problems about sequences or optimization.
What are memoization and tabulation?
Memoization is a technique where you store results of expensive function calls and reuse them when the same inputs occur again. Tabulation is a method where you build a table to solve problems in a bottom-up manner.
Why is it important to break problems into subproblems?
Breaking problems into subproblems makes them easier to solve and helps you see patterns. This can lead to more efficient solutions and a better understanding of the overall problem.
What are some common dynamic programming problems I should practice?
Some well-known problems include the 0/1 Knapsack Problem, Longest Common Subsequence, and Coin Change Problem. Practicing these can help improve your skills.
What strategies can I use for solving dynamic programming problems efficiently?
Try using the FAST method, which stands for Focus, Analyze, Solve, and Test. This approach can help you tackle problems step-by-step.
What mistakes should I avoid when working on dynamic programming problems?
Be careful not to overcomplicate the problem or ignore base cases. Also, make sure you understand how state transitions work.
Where can I find resources to practice dynamic programming?
You can find practice problems on websites like LeetCode, HackerRank, and Codewars. There are also helpful video tutorials on platforms like YouTube.