A Comprehensive Introduction to Dynamic Programming: Unlocking the Secrets of Algorithmic Efficiency
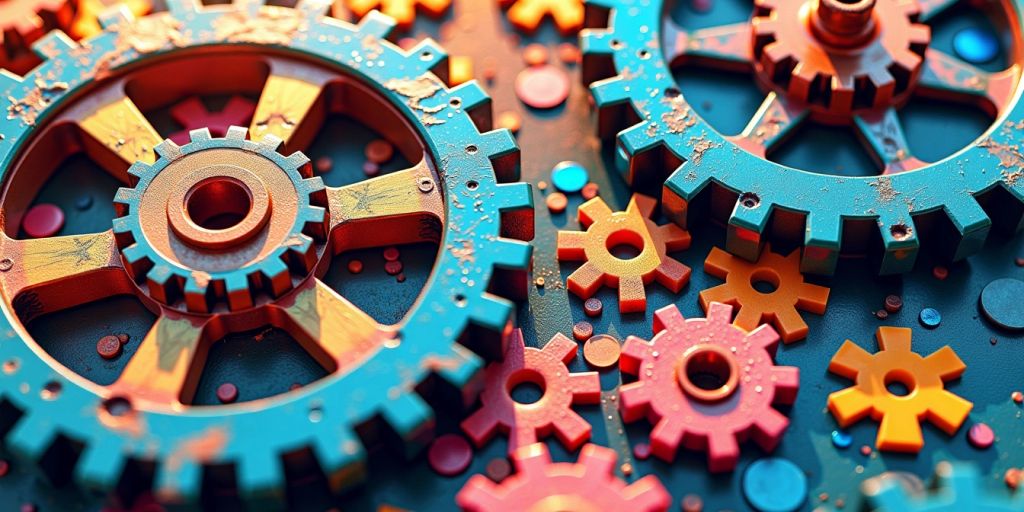
Dynamic programming is a powerful method used in computer science to solve complex problems by breaking them down into simpler subproblems. This approach helps in optimizing solutions, making them more efficient and easier to understand. In this article, we will explore the essentials of dynamic programming, its significance, and how it can be applied to various problems. Whether you’re a beginner or looking to sharpen your skills, this comprehensive guide will help you unlock the secrets of algorithmic efficiency.
Key Takeaways
- Dynamic programming is all about solving problems by using simpler, smaller problems.
- It is important to recognize when a problem can be solved using dynamic programming techniques.
- Key concepts include optimal substructure and overlapping subproblems, which are essential for applying DP.
- Common problems like the Knapsack Problem and Coin Change can be tackled effectively with dynamic programming.
- Practicing dynamic programming can significantly improve your problem-solving skills, especially in coding interviews.
Understanding the Basics of Dynamic Programming
Dynamic programming, often called DP, is a method used to solve complex problems by breaking them down into simpler subproblems. It is particularly useful when the same subproblems are solved multiple times. Here’s a brief overview of its key aspects:
Defining Dynamic Programming
Dynamic programming is a technique that helps in optimizing recursive solutions. It involves solving the problem for the first time and then using memoization to store the solutions. This way, we avoid recalculating results for the same inputs, making our algorithms more efficient.
Historical Context and Evolution
Dynamic programming was introduced by Richard Bellman in the 1950s. It has evolved significantly since then, becoming a fundamental concept in computer science. Here are some key milestones in its history:
- 1953: Richard Bellman formulates the concept.
- 1960s: DP is applied to various fields, including operations research.
- 1980s: The rise of computer algorithms leads to more widespread use.
Why Dynamic Programming Matters
Dynamic programming is crucial for several reasons:
- Efficiency: It reduces the time complexity of algorithms.
- Versatility: It can be applied to a wide range of problems, from finance to bioinformatics.
- Foundation for Advanced Techniques: Understanding DP is essential for grasping more complex algorithmic strategies.
Dynamic programming is not just a technique; it’s a powerful tool that can transform how we approach problem-solving in programming.
By mastering dynamic programming, you can unlock new levels of efficiency in your algorithms and tackle problems that once seemed insurmountable.
Key Concepts in Dynamic Programming
Optimal Substructure
Dynamic programming relies on the idea of optimal substructure. This means that the best solution to a problem can be constructed from the best solutions of its subproblems. For example, if you want to find the shortest path in a graph, you can break it down into smaller paths and find the best among them.
Overlapping Subproblems
Another important concept is overlapping subproblems. This occurs when a problem can be broken down into smaller, reusable subproblems. For instance, in the Fibonacci sequence, the same values are calculated multiple times. By storing these results, we can avoid redundant calculations, making our solution more efficient.
Memoization vs. Tabulation
When implementing dynamic programming, you can choose between two main techniques: memoization and tabulation.
- Memoization: This is a top-down approach where you store the results of expensive function calls and reuse them when the same inputs occur again.
- Tabulation: This is a bottom-up approach where you solve all possible subproblems and store their results in a table.
Technique | Description | Approach |
---|---|---|
Memoization | Top-down, stores results of function calls | Recursive |
Tabulation | Bottom-up, solves all subproblems iteratively | Iterative |
Understanding these key concepts is essential for mastering dynamic programming. They form the foundation upon which more complex algorithms are built.
By grasping these ideas, you can tackle a variety of problems more effectively and efficiently. Remember, dynamic programming is about improving existing solutions by recognizing patterns in problem-solving!
Common Problems Solved by Dynamic Programming
Dynamic programming (DP) is a powerful technique used to solve complex problems by breaking them down into simpler subproblems. Here are some of the most common problems that can be effectively tackled using DP:
The Knapsack Problem
The Knapsack Problem is a classic example where you have to maximize the total value of items you can carry in a knapsack without exceeding its weight limit. The key points include:
- Optimal Substructure: The optimal solution can be constructed from optimal solutions of its subproblems.
- Overlapping Subproblems: The same subproblems are solved multiple times.
The Coin Change Problem
In the Coin Change Problem, the goal is to find the minimum number of coins needed to make a certain amount of money. Important aspects are:
- Dynamic Programming Approach: Use a table to store results of subproblems to avoid redundant calculations.
- Greedy vs. DP: While a greedy approach may work for some cases, DP guarantees an optimal solution.
The Longest Common Subsequence Problem
This problem involves finding the longest subsequence common to two sequences. Key features include:
- Recursive Structure: The solution can be built from smaller subsequences.
- Memoization: Store results of subproblems to improve efficiency.
Dynamic programming is not just about finding solutions; it’s about understanding the patterns that can simplify complex problems.
Problem | Description | Key Technique |
---|---|---|
Knapsack Problem | Maximize value within weight limit | Optimal Substructure |
Coin Change Problem | Minimize coins for a given amount | Tabulation |
Longest Common Subsequence | Find longest subsequence in two sequences | Memoization |
By mastering these problems, you can unlock the secrets of algorithmic efficiency and improve your problem-solving skills in programming. Understanding these common problems is essential for anyone looking to excel in dynamic programming.
Steps to Approach a Dynamic Programming Problem
Identifying the Problem Type
To effectively tackle a dynamic programming (DP) problem, the first step is to identify the type of problem you are dealing with. Here are some key points to consider:
- Look for overlapping subproblems: This means the same smaller problems are solved multiple times.
- Check for optimal substructure: If solving a subproblem helps in solving the main problem, it’s a good candidate for DP.
- Understand the problem’s requirements: Make sure you know what the problem is asking for before diving in.
Breaking Down the Problem
Once you’ve identified the problem type, the next step is to break it down into smaller parts. This involves:
- Dividing the main problem into smaller, independent subproblems.
- Storing solutions: Solve each subproblem and store the solution in a table or array for future reference.
- Building the solution: Use the stored solutions to construct the final answer to the main problem.
Formulating the Recursive Solution
The final step is to create a recursive solution. This includes:
- Writing a function that calls itself to solve the subproblems.
- Ensuring that you check if a subproblem has already been solved to avoid unnecessary calculations.
- Using memoization to store results of subproblems, which can significantly improve efficiency.
By following these steps, you can systematically approach dynamic programming problems and enhance your problem-solving skills. Remember, practice makes perfect!
Advanced Techniques in Dynamic Programming
Dynamic programming (DP) is a powerful method for solving complex problems by breaking them down into simpler subproblems. Here, we will explore some advanced techniques that can enhance your DP skills.
State Compression
State compression is a technique used to reduce the amount of memory needed for storing states in DP problems. By representing states in a more compact form, you can often solve problems that would otherwise be too large to handle. For example:
- Use bitmasks to represent subsets.
- Store only necessary states instead of all possible states.
- Apply mathematical transformations to reduce dimensions.
Bitmasking
Bitmasking is a specific form of state compression that allows you to efficiently represent sets of items. This technique is particularly useful in problems involving subsets or combinations. Here are some key points:
- Each bit in an integer can represent whether an item is included in a subset.
- Operations like union and intersection can be performed using bitwise operations.
- It can significantly speed up the solution for problems like the Traveling Salesman Problem.
Divide and Conquer with DP
This technique combines the principles of divide and conquer with dynamic programming. Instead of solving the problem in a single pass, you break it down into smaller parts, solve each part, and then combine the results. This approach can be particularly effective for:
- Problems with a clear recursive structure.
- Situations where overlapping subproblems can be reused.
- Enhancing the efficiency of naive recursive solutions.
In summary, mastering these advanced techniques can greatly improve your ability to tackle complex dynamic programming problems.
Dynamic Programming in Coding Interviews
Common Interview Questions
Dynamic programming (DP) is a popular topic in coding interviews. Here are some common questions you might encounter:
- The Knapsack Problem: How do you maximize the value of items in a knapsack?
- Coin Change Problem: How can you make change for a given amount using the least number of coins?
- Longest Common Subsequence: How do you find the longest sequence that appears in the same order in two strings?
How to Explain Your Solution
When discussing your DP solution in an interview, consider these steps:
- Define the Problem: Clearly state what the problem is asking.
- Identify Subproblems: Explain how the problem can be broken down into smaller parts.
- Optimal Substructure: Discuss how solving these smaller parts leads to the overall solution.
- Choose a Method: Decide between memoization or tabulation based on the problem’s needs.
Tips for Success
To excel in dynamic programming interviews, keep these tips in mind:
- Practice Regularly: Work on various DP problems to build your skills.
- Understand Time Complexity: Be ready to discuss the efficiency of your solution.
- Communicate Clearly: Explain your thought process as you work through the problem.
Dynamic programming is a powerful tool that can significantly improve your problem-solving skills.
In summary, mastering dynamic programming can set you apart in coding interviews. By understanding common questions, explaining your solutions clearly, and practicing regularly, you can unlock the secrets of algorithmic efficiency. Remember, a dynamic programming cheatsheet for coding interviews can be a great resource, including practice questions, techniques, time complexity, and recommended resources.
Real-World Applications of Dynamic Programming
Dynamic programming (DP) is not just a theoretical concept; it has many real-world applications that can significantly improve efficiency in various fields. Here are some key areas where DP shines:
Resource Allocation
- Optimizing resources in projects to ensure maximum efficiency.
- Scheduling tasks in a way that minimizes costs and maximizes output.
- Distributing resources among competing needs effectively.
Sequence Alignment in Bioinformatics
- Comparing DNA sequences to find similarities and differences.
- Aligning protein sequences to understand evolutionary relationships.
- Identifying mutations that may lead to diseases.
Network Optimization
- Routing data in networks to minimize delays and maximize throughput.
- Managing bandwidth to ensure fair distribution among users.
- Designing efficient networks that can handle large amounts of data.
Dynamic programming is a powerful tool that can help you determine the optimal strategy in various scenarios, from the Fibonacci sequence to complex mapping problems.
In summary, dynamic programming is a versatile technique that can be applied across different domains, making it an essential skill for anyone looking to enhance their problem-solving abilities in technology and science.
Challenges and Pitfalls in Dynamic Programming
Dynamic programming (DP) is a powerful tool, but it comes with its own set of challenges. Understanding these can help you avoid common mistakes and improve your problem-solving skills.
Common Mistakes to Avoid
- Ignoring the Problem Type: Not every problem is suitable for DP. Make sure to identify if the problem has overlapping subproblems and optimal substructure.
- Overcomplicating Solutions: Sometimes, a simple recursive solution is better. Don’t force DP if it’s not needed.
- Neglecting Base Cases: Always define your base cases clearly. Missing these can lead to incorrect results.
Understanding Time and Space Complexity
Dynamic programming often improves time complexity at the cost of increased space complexity. Here’s a quick comparison:
Approach | Time Complexity | Space Complexity |
---|---|---|
Brute Force | Exponential | O(1) |
Memoization | O(n) | O(n) |
Tabulation | O(n) | O(n) |
Debugging Dynamic Programming Solutions
Debugging DP can be tricky. Here are some tips:
- Print Intermediate Results: This helps you see how values are being computed.
- Check Recursive Calls: Ensure that you’re not recalculating values unnecessarily.
- Use Visual Aids: Drawing out the problem can clarify how the solution is structured.
Remember: Dynamic programming is a tool for improving a pre-existing solution. Understanding its challenges will help you use it effectively.
Learning Resources and Further Reading
Books and Tutorials
- Introduction to Algorithms by Thomas H. Cormen, Charles E. Leiserson, and Ronald L. Rivest: This book is a great starting point for understanding algorithms, including dynamic programming. It covers both theory and practical applications.
- Dynamic Programming for Coding Interviews by Meenakshi: This book focuses on common dynamic programming problems and provides clear explanations and solutions.
- The Algorithm Design Manual by Steven S. Skiena: This book offers insights into algorithm design and includes a section on dynamic programming.
Online Courses and Videos
- Coursera: Offers courses on algorithms that include sections on dynamic programming.
- edX: Provides various computer science courses that cover dynamic programming techniques.
- YouTube: Channels like "CS Dojo" and "mycodeschool" have excellent video tutorials on dynamic programming.
Practice Problems and Platforms
- LeetCode: A popular platform with many dynamic programming problems to practice.
- HackerRank: Offers challenges specifically focused on dynamic programming.
- Codewars: A fun way to solve dynamic programming problems and improve your skills.
Dynamic programming is a powerful technique that helps break down complex problems into smaller, manageable parts. By learning these resources, you can master the art of solving dynamic programming problems effectively.
Summary Table of Resources
Type | Resource Name | Description |
---|---|---|
Book | Introduction to Algorithms | Comprehensive guide on algorithms and DP. |
Book | Dynamic Programming for Coding Interviews | Focuses on common DP problems. |
Online Course | Coursera | Courses on algorithms including DP. |
Online Course | edX | Various CS courses covering DP techniques. |
Practice Platform | LeetCode | Many DP problems to practice. |
Comparing Dynamic Programming with Other Techniques
Greedy Algorithms
Greedy algorithms make the best choice at each step, hoping to find the global optimum. They are often faster but can miss the best overall solution. Here are some key points:
- Local vs. Global: Greedy focuses on local optimums, while dynamic programming looks for global optimums.
- Efficiency: Greedy algorithms are usually more efficient in terms of time complexity.
- Use Cases: Problems like minimum spanning trees can be solved using greedy methods, while others, like the Knapsack problem, require dynamic programming.
Divide and Conquer
Divide and conquer breaks a problem into smaller parts, solves them independently, and combines the results. In contrast, dynamic programming solves subproblems and stores their solutions to avoid recalculating them. Here’s how they differ:
- Problem Breakdown: Divide and conquer splits problems into independent subproblems, while dynamic programming handles overlapping subproblems.
- Storage: Dynamic programming saves solutions to subproblems, making it more efficient for certain types of problems.
- Examples: Merge sort is a classic divide and conquer algorithm, while Fibonacci sequence calculation can be optimized using dynamic programming.
Backtracking
Backtracking is a method for solving problems incrementally, trying partial solutions and removing those that fail to satisfy the conditions. Here’s how it compares:
- Exploration: Backtracking explores all possibilities, while dynamic programming focuses on optimal solutions.
- Efficiency: Dynamic programming is generally more efficient for problems with overlapping subproblems.
- Use Cases: Backtracking is often used in puzzles and games, while dynamic programming is used in optimization problems.
Technique | Key Feature | Best Use Case |
---|---|---|
Greedy Algorithms | Local optimums | Minimum spanning trees |
Divide and Conquer | Independent subproblems | Merge sort |
Dynamic Programming | Overlapping subproblems | Knapsack problem |
Backtracking | Incremental exploration | Puzzles and games |
Understanding the differences between these techniques helps in choosing the right approach for a problem. Each method has its strengths and weaknesses, and knowing when to use each can greatly enhance your problem-solving skills.
Future Directions in Dynamic Programming
Dynamic Programming in Machine Learning
Dynamic programming (DP) is making waves in the field of machine learning. It helps in optimizing algorithms that require decision-making over time. Here are some areas where DP is being applied:
- Reinforcement learning
- Sequence prediction
- Neural network training
Quantum Computing and DP
As quantum computing evolves, it opens new doors for dynamic programming. Quantum algorithms can potentially solve DP problems faster than classical methods. This could lead to breakthroughs in:
- Complex optimization problems
- Cryptography
- Large-scale simulations
Ethical Considerations in Algorithm Design
With the rise of DP in various fields, ethical considerations are becoming crucial. It’s important to ensure that algorithms are designed responsibly. Key points include:
- Fairness in decision-making
- Transparency in algorithmic processes
- Accountability for algorithmic outcomes
The future of dynamic programming is bright, but it comes with responsibilities that we must not overlook. Understanding these implications is key to harnessing DP effectively.
As we look ahead in the world of dynamic programming, there are exciting opportunities to explore. Whether you’re just starting or looking to sharpen your skills, now is the perfect time to dive in. Don’t miss out on the chance to enhance your coding abilities and prepare for your dream job. Visit our website to start coding for free and unlock your potential!
Conclusion
In summary, dynamic programming is a powerful tool that can help you solve complex problems more easily. By breaking down a problem into smaller parts and solving each part only once, you can save time and effort. This method not only makes your code run faster but also helps you understand the problem better. Remember, the key to mastering dynamic programming is practice. The more you work on different problems, the more comfortable you will become with the concepts. So, keep coding, stay curious, and don’t hesitate to ask for help when you need it. Happy coding!
Frequently Asked Questions
What is dynamic programming?
Dynamic programming is a method used in computer science to solve problems by breaking them down into smaller parts. It helps to find the best solution by storing results of smaller problems so they don’t have to be solved again.
Why is dynamic programming important?
Dynamic programming is important because it makes algorithms faster and more efficient. By reusing solutions to smaller problems, it saves time and resources when solving bigger problems.
Can you give an example of a dynamic programming problem?
Sure! One common example is the ‘Knapsack Problem,’ where you have to decide which items to carry in a bag to maximize the total value without exceeding the bag’s weight limit.
What is memoization?
Memoization is a technique used in dynamic programming where you store the results of expensive function calls and reuse them when the same inputs occur again.
What are overlapping subproblems?
Overlapping subproblems are smaller problems that are solved multiple times in the process of solving a bigger problem. Dynamic programming helps avoid solving these again.
How does dynamic programming differ from recursion?
Dynamic programming is a more efficient way to solve problems compared to simple recursion. While recursion solves problems step by step, dynamic programming saves solutions to avoid repeating work.
What is the coin change problem?
The coin change problem involves finding the number of ways to make a certain amount of money using a set of coins. It’s a classic example of a problem that can be solved using dynamic programming.
How can I improve my skills in dynamic programming?
To improve your skills in dynamic programming, practice solving different problems, watch tutorials, and read books on algorithms. Websites like LeetCode and HackerRank offer many practice problems.