Essential Strategies for Effective Java Interview Preparation in 2024
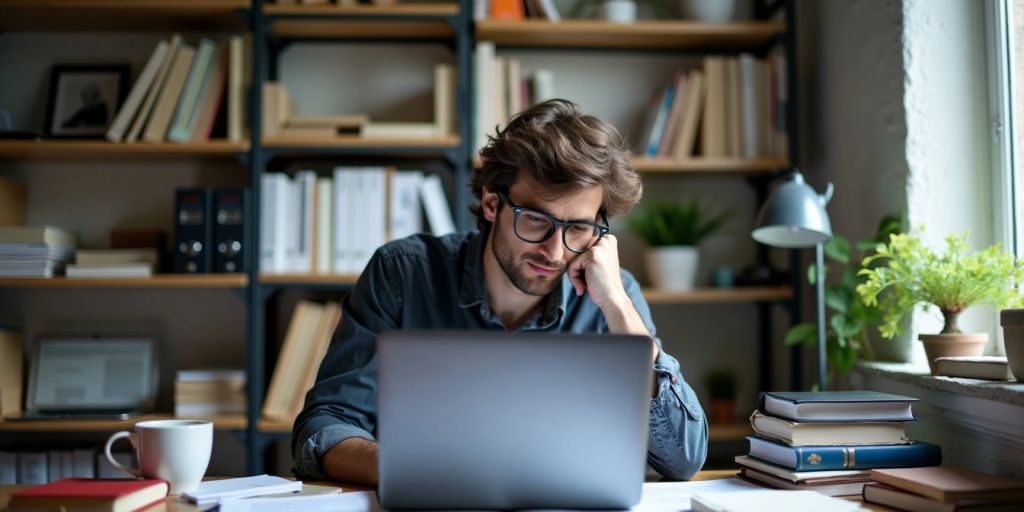
Preparing for a Java interview can be a bit overwhelming, especially with all the different topics you need to study. This guide will help you navigate the essential strategies for effective interview preparation in 2024. From understanding the interview process to mastering Java fundamentals, we’ll cover everything you need to know to feel confident and ready for your next interview.
Key Takeaways
- Start with the basics: Make sure you understand core Java concepts like OOP and syntax.
- Practice coding: Use online platforms to solve coding problems and improve your skills.
- Prepare for behavioral questions: Use the STAR method to answer questions about your past experiences.
- Conduct mock interviews: This helps you get comfortable with the interview format and receive feedback.
- Stay updated: Keep an eye on the latest Java trends and features to impress your interviewers.
Understanding the Core Java Interview Process
The Java interview process is designed to assess both your technical skills and your fit within a company. It usually consists of several stages that help employers evaluate candidates thoroughly.
Technical Screening and Initial Assessments
In this first stage, candidates often undergo a technical screening, which may include:
- Phone or video interviews focusing on basic Java concepts.
- Questions about object-oriented programming principles, exception handling, and collections.
- A brief assessment of your coding skills through a simple coding challenge.
Coding Challenges and Problem-Solving
Coding challenges are a crucial part of the interview process. They typically involve:
- Take-home assignments or online tests on platforms like HackerRank.
- Tasks that test your problem-solving abilities and coding skills.
- Questions that may include core Java interview questions and answers (2024) to help you prepare effectively.
Behavioral and Cultural Fit Interviews
Behavioral interviews assess how well you fit into the company culture. Key aspects include:
- Evaluating your teamwork and leadership skills.
- Discussing how you handle pressure and failures.
- Understanding your problem-solving approach in real-world scenarios.
Preparing for a Java interview requires a mix of solid knowledge, practice, and understanding of common questions.
By following these strategies, candidates can enhance their chances of success in the Java interview process.
Mastering Java Fundamentals
Key Concepts in Object-Oriented Programming
Understanding Object-Oriented Programming (OOP) is crucial for Java developers. Here are the four main principles:
- Encapsulation: This means keeping data safe by bundling it with methods that operate on it. It hides the details from the outside world.
- Inheritance: This allows a new class to take on properties and methods from an existing class, making code reuse easier.
- Polymorphism: This lets one interface be used for different actions, depending on the situation. It includes method overloading and overriding.
- Abstraction: This hides complex details and shows only the necessary parts, often using abstract classes and interfaces.
Understanding Java Syntax and Semantics
Java has a specific way of writing code, known as syntax. Here are some key points:
- Data Types: Java has two main types: primitive (like
int
,double
,char
) and non-primitive (likeString
, arrays). - Variables: These are used to store data. Each variable must have a data type.
- Operators: Special symbols that perform operations on variables, such as arithmetic and logical operations.
Common Java Libraries and Frameworks
Familiarity with popular libraries and frameworks can enhance your Java skills. Here are a few:
- Java Standard Library: Provides essential classes and methods for basic programming tasks.
- Spring Framework: A powerful framework for building Java applications, especially for web development.
- Hibernate: A library for managing database operations in Java applications.
Mastering these fundamentals is essential for success in Java interviews. Prepare for your next job with these top Java interview questions to ace your Java interview.
Advanced Java Topics to Focus On
Multithreading and Concurrency
Multithreading is a key concept in Java that allows multiple threads to run simultaneously. Understanding how to manage threads effectively is crucial for building responsive applications. Here are some important points to consider:
- Thread Lifecycle: Know the different states of a thread (New, Runnable, Blocked, Waiting, Timed Waiting, and Terminated).
- Synchronization: Learn how to use synchronized blocks and methods to prevent thread interference.
- Concurrency Utilities: Familiarize yourself with classes from the
java.util.concurrent
package, such asExecutorService
andCountDownLatch
.
Java Collections Framework
The Java Collections Framework provides a set of classes and interfaces for storing and manipulating groups of objects. Here’s what you should focus on:
- List, Set, and Map Interfaces: Understand the differences between these collections and when to use each.
- Common Implementations: Get to know
ArrayList
,HashSet
, andHashMap
and their performance characteristics. - Iterators: Learn how to use iterators for traversing collections efficiently.
Java Memory Management
Memory management is vital for optimizing application performance. Here are some key aspects:
- Garbage Collection: Understand how Java automatically manages memory and the different garbage collection algorithms. For example, know the difference between soft and weak references.
- Heap vs. Stack: Learn the differences between heap memory and stack memory, and how they affect performance.
- Memory Leaks: Be aware of common causes of memory leaks and how to avoid them.
Mastering these advanced topics will not only prepare you for interviews but also enhance your overall programming skills in Java.
By focusing on these areas, you can significantly improve your chances of success in Java interviews and become a more proficient developer.
Effective Problem-Solving Techniques
In Java interviews, demonstrating strong problem-solving skills is crucial. These skills help you tackle coding challenges effectively. Here are some techniques to enhance your problem-solving abilities:
Approaching Coding Challenges
- Understand the Problem: Read the problem statement carefully and clarify any doubts.
- Break It Down: Divide the problem into smaller, manageable parts.
- Plan Your Solution: Outline your approach before coding.
Debugging and Optimizing Java Code
- Use IDE Features: Modern IDEs like IntelliJ IDEA offer tools for debugging, such as breakpoints and variable inspection.
- Implement Logging: Use logging frameworks to track runtime information, which helps in identifying issues.
- Optimize Algorithms: Focus on improving the efficiency of your code by refining algorithms and reducing memory usage.
Utilizing Design Patterns
- Familiarize with Common Patterns: Understand patterns like Singleton, Factory, and Observer to solve problems more effectively.
- Apply Patterns Appropriately: Use design patterns that fit the problem context to create robust solutions.
- Practice with Examples: Work on coding problems that require the application of design patterns to reinforce your understanding.
By mastering these techniques, you can improve your coding skills and stand out in interviews. Remember, practice is key to becoming proficient in problem-solving!
Preparing for Coding Interviews
Practicing with Mock Interviews
Mock interviews are a great way to prepare for the real thing. They help you get used to the interview format and improve your confidence. Here are some tips for effective mock interviews:
- Choose a partner: Find a friend or colleague to practice with.
- Use online platforms: Websites like Pramp and Interviewing.io offer mock interviews with real interviewers.
- Record your sessions: Watching your performance can help you identify areas for improvement.
Leveraging Online Coding Platforms
Using online coding platforms can significantly enhance your preparation. Here are some popular options:
- LeetCode: Offers a wide range of coding problems categorized by difficulty.
- HackerRank: Focuses on Java-specific challenges to sharpen your skills.
- CodeSignal: Provides a fun way to practice coding through games and challenges.
Time Management During Coding Tests
Managing your time effectively during coding tests is crucial. Here are some strategies:
- Read the problem carefully: Make sure you understand what is being asked before you start coding.
- Plan your approach: Spend a few minutes outlining your solution before diving in.
- Keep track of time: Set a timer to ensure you don’t spend too long on any one question.
Preparing for coding interviews is not just about knowing Java; it’s about practicing and refining your skills. Mock interviews and coding platforms are essential tools to help you succeed.
Behavioral Interview Preparation
Common Behavioral Questions
When preparing for behavioral interviews, it’s important to anticipate the most commonly asked behavioral questions. Here are some examples:
- Why do you want to work for this company?
- Can you describe a challenging situation you faced at work?
- How do you handle conflicts within a team?
Using the STAR Method
The STAR method is a great way to structure your answers. It stands for:
- Situation: Describe the context within which you performed a task or faced a challenge.
- Task: Explain your responsibilities in that situation.
- Action: Detail the specific actions you took to address the situation.
- Result: Share the outcomes of your actions, emphasizing what you learned.
Showcasing Teamwork and Leadership
In interviews, demonstrating your teamwork and leadership skills is crucial. Here are some tips:
- Highlight your role in team projects.
- Discuss how you motivated others during challenging times.
- Provide examples of how you resolved conflicts or facilitated collaboration.
Remember, preparation is key. Practicing your responses can help you feel more confident and articulate during the interview.
Resources for Java Interview Preparation
Recommended Books and Guides
When preparing for Java interviews, having the right materials is crucial. Here are some top books to consider:
- "Effective Java" by Joshua Bloch: A must-read for understanding best practices in Java.
- "Java: The Complete Reference" by Herbert Schildt: Comprehensive coverage of Java concepts.
- "Core Java Volume I & II" by Cay S. Horstmann: Great for both beginners and experienced developers.
Online Courses and Tutorials
In addition to books, online resources can enhance your learning:
- Oracle’s official Java documentation: The go-to source for Java standards and practices.
- GeeksforGeeks: Offers numerous Java programming examples and tutorials.
- Udemy and Coursera: Platforms with various Java courses tailored to different skill levels.
Practice Problems and Coding Drills
To excel in coding interviews, practice is essential. Here are some platforms to help you:
- LeetCode: A vast collection of coding problems categorized by difficulty.
- HackerRank: Focuses on Java-specific challenges to sharpen your skills.
- Interviewing.io: Provides mock interviews with real engineers from top tech companies.
Remember, consistent practice is key to mastering Java interview questions.
Summary
Utilizing these resources will help you prepare effectively for your Java interviews. The right materials can make a significant difference in your confidence and performance during the interview process.
Best Practices for Java Interviews
Effective Communication Skills
Clear communication is key. During your interview, make sure to express your thoughts clearly. This helps the interviewer understand your problem-solving process. Here are some tips:
- Speak slowly and clearly.
- Use technical terms appropriately.
- Ask for clarification if you don’t understand a question.
Highlighting Personal Projects
Showcasing your personal projects can set you apart. Discussing your work demonstrates your passion for Java. Consider these points:
- Explain the project’s purpose and your role.
- Highlight any challenges you faced and how you overcame them.
- Mention any technologies or frameworks you used.
Asking Insightful Questions
At the end of the interview, asking questions shows your interest in the role and the company. Here are some examples:
- What does a typical day look like for a Java developer here?
- Can you tell me about the team I would be working with?
- What are the biggest challenges the team is currently facing?
Preparing for a Java interview in 2024 means brushing up on your core Java concepts and being ready to discuss your experiences confidently.
By following these best practices, you can enhance your performance and make a lasting impression during your Java interviews.
Common Java Interview Questions
In this section, we will look at some frequently asked Java interview questions to help you understand what to expect. As you gain more experience, the questions may become tougher, but it’s important to remember the basics. You can expect a variety of questions that test your knowledge of the Java API.
Core Java Concepts
Some common questions include:
- What are the differences between abstract classes and interfaces in Java?
- How does multithreading work in Java, and what are its benefits?
- What is the difference between the
equals()
method and the==
operator in Java? - Explain method overloading and method overriding in Java.
- How do you handle exceptions in Java? Describe the try-catch-finally block.
Advanced Java Topics
As you advance, you might face questions like:
- How does the Java Collections Framework work?
- What are Generics, and why are they useful?
- Can you explain the concept of Garbage Collection in Java?
Scenario-Based Questions
You may also encounter scenario-based questions, such as:
- How would you design a multi-threaded application?
- Describe a situation where you had to optimize Java code for performance.
- How would you handle a memory leak in a Java application?
Being well-prepared for both basic and advanced Java topics will significantly improve your chances of success in interviews.
Remember, practice is key! Familiarize yourself with these questions and try to articulate your answers clearly during the interview.
Utilizing Mock Interviews for Practice
Mock interviews are a great way to prepare for real Java interviews. They help you get comfortable with the interview format and improve your confidence. Practicing with mock interviews can significantly boost your performance.
Benefits of Mock Interviews
- Realistic Experience: Simulates the actual interview environment.
- Feedback: Provides constructive criticism to help you improve.
- Confidence Building: Reduces anxiety by familiarizing you with the process.
Finding Mock Interview Platforms
- LeetCode: Offers a variety of coding problems and mock interview options.
- HackerRank: Great for practicing Java-specific questions.
- Interviewing.io: Allows you to practice anonymously with experienced engineers.
Analyzing and Learning from Feedback
- Review Performance: After each mock interview, take time to review your performance.
- Identify Weaknesses: Focus on areas where you struggled.
- Adjust Strategies: Change your approach based on feedback to improve for the next time.
Mock interviews are not just practice; they are a vital part of your preparation strategy. They help you understand what to expect and how to respond effectively.
By utilizing mock interviews, you can enhance your skills and be better prepared for the challenges of a real Java interview. Remember, the more you practice, the more confident you will become!
Technical Deep Dive Interviews
System Design Questions
In technical deep dive interviews, system design questions are crucial. These questions assess your ability to create scalable and efficient systems. Here are some common areas to focus on:
- Understanding requirements: Clearly define what the system needs to do.
- Choosing the right architecture: Decide between microservices, monoliths, etc.
- Scalability considerations: Plan for future growth and user load.
In-Depth Framework Discussions
Interviewers often dive into specific frameworks you’ve used. Be prepared to discuss:
- Framework features: Know the key functionalities.
- Use cases: Explain when and why you used them.
- Limitations: Be honest about any challenges faced.
Performance and Scalability Considerations
Understanding how to optimize performance is vital. Here are some tips:
- Profiling tools: Familiarize yourself with tools that help identify bottlenecks.
- Caching strategies: Learn how to implement caching to improve speed.
- Load testing: Know how to simulate user load to test system performance.
Mastering these topics can significantly boost your confidence and performance in interviews.
By preparing for these areas, you can demonstrate your technical depth and problem-solving skills effectively. Remember, technical deep dives are your chance to shine and show your expertise!
Staying Updated with Java Trends
Latest Java Features and Updates
Keeping up with the latest Java features is essential for developers. Here are some key updates to watch for in 2024:
- DevOps Integration: Enhancing collaboration between development and operations.
- Serverless Architecture: Simplifying deployment and scaling of applications.
- Big Data Technologies: Leveraging Java for handling large datasets.
Industry Best Practices
To stay relevant in the Java community, consider these best practices:
- Continuous Learning: Regularly update your skills through courses and tutorials.
- Networking: Connect with other Java professionals to share knowledge and experiences.
- Contributing to Open Source: Engage in open-source projects to gain practical experience.
Networking with Java Professionals
Building a strong network can open doors to new opportunities. Here are ways to connect:
- Attend Java meetups and conferences.
- Join online forums and discussion groups.
- Follow influential Java developers on social media.
Staying updated with Java trends is crucial for career growth. Embrace change and adapt to new technologies to remain competitive in the job market.
Trend | Description |
---|---|
DevOps | Integration of development and operations. |
Serverless Architecture | Simplified deployment and scaling. |
Big Data | Handling large datasets efficiently. |
Remote Access Solutions | Enhancing accessibility for developers. |
Spring Framework | Continued popularity for building applications. |
By following these strategies, you can ensure that you are well-prepared for the evolving landscape of Java development in 2024.
To keep up with the latest in Java, it’s important to stay informed about new features and trends. Regularly checking resources and communities can help you learn and grow as a programmer. Don’t miss out on the chance to enhance your skills! Visit our website to start coding for free and explore our interactive tutorials that will guide you through the latest Java developments.
Conclusion
As you gear up for your Core Java interviews in 2024, it’s important to have a solid understanding of the basics and a good grasp of advanced topics. This guide has provided you with key insights, from the structure of interviews to important Java features and effective study methods. To further boost your Java skills and connect with top tech companies, check out Weekday. There, you can find great job opportunities and expand your professional network. Dive deeper into your Java preparation and explore exciting career paths with Weekday.
Frequently Asked Questions
What should I study to prepare for a Java interview?
To get ready for a Java interview, focus on the basics of Java, data structures, and algorithms. Also, practice coding problems and review common interview questions.
How can I practice coding for my Java interview?
You can practice coding by using online platforms like LeetCode or HackerRank. These sites offer many coding problems and mock interviews to help you get ready.
What kind of questions are asked in Java interviews?
Java interviews often include questions about Java concepts, coding challenges, and behavioral questions to see how you work with others.
How do I handle coding challenges during the interview?
Take your time to understand the problem, plan your solution, and speak your thoughts aloud. This helps the interviewer follow your logic.
What is the STAR method for interviews?
The STAR method stands for Situation, Task, Action, and Result. It helps you structure your answers to behavioral questions by telling a clear story.
Why is it important to know Java libraries and frameworks?
Knowing Java libraries and frameworks can help you solve problems faster and show the interviewer that you are familiar with tools used in the industry.
How can I improve my problem-solving skills in Java?
Practice regularly by solving coding problems, participating in coding competitions, and reviewing algorithms to sharpen your skills.
What should I do if I get stuck during a coding interview?
If you get stuck, don’t panic. Take a moment to think, ask clarifying questions, and explain your thought process to the interviewer.