Mastering the Art of Coding Task Problem Solving: Strategies for Success
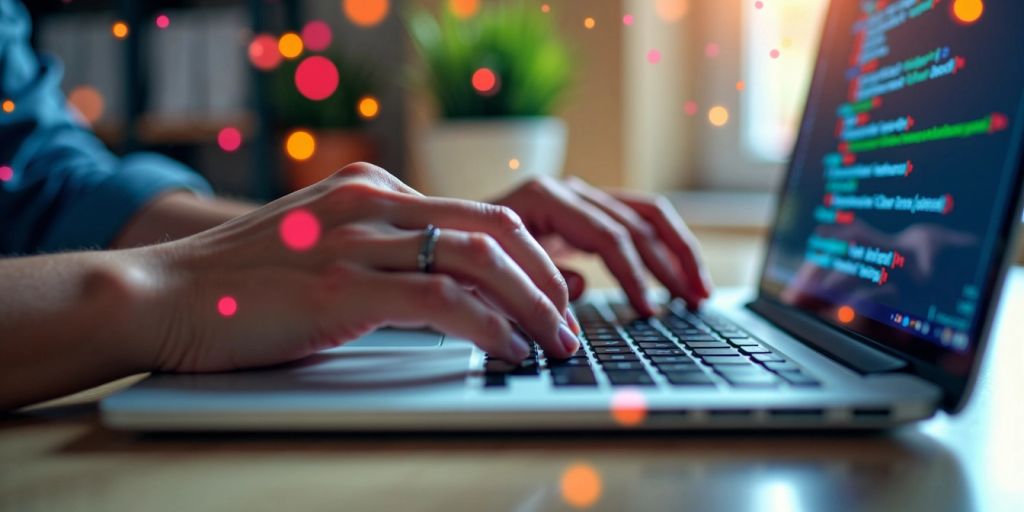
Coding is a vital skill in today’s world, and mastering problem-solving is key to becoming a great coder. This article will explore different strategies to help you tackle coding challenges effectively. From understanding the right mindset to implementing solutions, we’ll cover essential techniques that can make you a better programmer.
Key Takeaways
- Adopt a positive mindset to face challenges head-on.
- Break problems down into smaller parts for easier understanding.
- Plan your solution using pseudocode or diagrams.
- Practice regularly to improve your coding skills.
- Learn from others and seek feedback to grow.
Embracing the Problem-Solving Mindset
Understanding the Importance of Mindset
Having the right mindset is crucial for success in coding. Shift your focus from just writing code to solving problems. This change in perspective can make a big difference in how you approach challenges. Instead of asking, "How do I code this?" ask yourself, "What is the best way to solve this problem?"
Embracing Challenges and Failures
Coding is full of challenges. When you encounter a tough problem, remember that every issue has a solution. Embrace these challenges rather than avoiding them. Here are some key points to keep in mind:
- Every failure is a learning opportunity.
- Persistence is key; don’t give up easily.
- Celebrate small victories along the way.
Cultivating Patience and Persistence
Problem-solving takes time and effort. It’s important to be patient with yourself as you learn. Here are some strategies to help you stay persistent:
- Break problems into smaller parts.
- Take breaks when feeling stuck.
- Seek help from peers or mentors.
Remember, every great coder started as a beginner. With dedication and practice, you can improve your problem-solving skills and tackle any challenge that comes your way.
Breaking Down the Problem
Reading the Problem Statement Carefully
The first step in solving any coding problem is to read the problem statement carefully. This may seem simple, but it’s easy to miss important details that can guide your solution. Make sure you understand every requirement and constraint. If needed, read the statement multiple times to grasp it fully.
Identifying Key Requirements
After reading the problem, the next step is to identify the key requirements. Ask yourself:
- What is the problem asking for?
- What are the inputs?
- What are the expected outputs?
Breaking down the problem into these basic parts helps clarify what needs to be done. This process is often referred to as decomposition, where you divide the problem into smaller, manageable pieces.
Asking Clarifying Questions
If anything is unclear, don’t hesitate to ask questions. This is especially important in real-world scenarios where problem statements can be vague. Asking clarifying questions ensures you’re on the right track and helps prevent misunderstandings.
Understanding the problem is the first step to finding a solution. Without a clear grasp, you risk creating more issues.
By following these steps, you can effectively break down the problem and set yourself up for success in coding tasks.
Planning Your Approach
Using Pseudocode for Planning
Planning your coding approach is crucial. Pseudocode helps you outline your solution in simple terms, making it easier to translate into actual code later. It allows you to focus on the logic without worrying about syntax errors. Here’s how to effectively use pseudocode:
- Write down the main steps of your solution.
- Use simple language to describe what each part does.
- Review your pseudocode to ensure it covers all requirements.
Diagramming and Visualizing
Visual aids can make complex problems easier to understand. Creating diagrams or flowcharts helps you see the relationships between different parts of the problem. Here are some benefits of diagramming:
- Clarifies the Path Forward: It shows the steps needed to solve the problem.
- Identifies Potential Issues Early: You can spot challenges before coding.
- Saves Time and Effort: Planning upfront can prevent wasted time later.
Writing Down the Problem
Writing the problem in your own words can reveal gaps in your understanding. This technique is helpful because:
- It forces you to think critically about the problem.
- It helps you identify key requirements and constraints.
- It prepares you to explain the problem to others, fostering collaboration.
Remember, breaking down the problem into smaller parts makes it easier to tackle each component one at a time. This approach is known as decomposition, and it’s a key strategy in effective problem-solving.
By planning your approach carefully, you set yourself up for success in coding tasks. Always take the time to understand the problem fully before jumping into coding!
Choosing the Right Tools and Techniques
Selecting Appropriate Data Structures
Choosing the right data structure is crucial for solving coding problems efficiently. Here are some common data structures and their uses:
- Arrays: Good for storing a fixed-size sequence of elements.
- Linked Lists: Useful for dynamic data where size can change.
- Stacks: Ideal for problems that require last-in, first-out access.
- Queues: Best for first-in, first-out scenarios.
Understanding Algorithm Efficiency
Efficiency is key in coding. You should always consider the time and space complexity of your algorithms. Here’s a quick overview:
Algorithm Type | Time Complexity | Space Complexity |
---|---|---|
Sorting (e.g., Quick) | O(n log n) | O(log n) |
Searching (e.g., Binary) | O(log n) | O(1) |
Dynamic Programming | O(n^2) | O(n) |
Leveraging Libraries and Frameworks
Using libraries can save you time and effort. Here are some popular ones:
- NumPy: Great for numerical computations.
- Pandas: Excellent for data manipulation and analysis.
- React: Useful for building user interfaces.
Remember, the right tools can make a big difference in your coding journey. They help you focus on solving the problem rather than getting stuck on implementation details.
By mastering these tools and techniques, you can enhance your problem-solving skills and tackle coding challenges with confidence!
Implementing the Solution
Writing Clean and Maintainable Code
When you start coding, focus on writing clean code. This means using clear names for your variables and functions, and keeping your code organized. Here are some tips:
- Use meaningful names for variables and functions.
- Keep your code DRY (Don’t Repeat Yourself).
- Add comments to explain complex parts.
Handling Edge Cases
Edge cases are situations that might not happen often but can cause your program to fail. To handle them:
- Think about unusual inputs.
- Test your code with these inputs.
- Make sure your code can handle them without crashing.
Iterating and Refining Solutions
After your initial implementation, it’s important to refine your solution. This involves:
- Reviewing your code for improvements.
- Testing it thoroughly to find any bugs.
- Making adjustments based on feedback or new insights.
Remember, coding is a journey. Each step you take helps you grow as a problem solver. Embrace the process and learn from every experience!
Testing and Debugging
Testing is a vital part of coding that ensures your program works correctly. It helps find mistakes, checks if your solution meets the needs, and improves the overall quality of your software. Here are some key points about testing:
Developing a Testing Strategy
- Verification: Testing confirms that your code meets the required tasks.
- Reliability: Good testing makes your software more dependable by finding and fixing problems early.
- Maintenance: Well-tested code is easier to manage later on, acting as a guide for future developers.
- Cost-Effectiveness: Fixing bugs during development is cheaper than after the software is released.
Using Debugging Tools Effectively
Debugging is the process of finding and fixing errors in your code. Here are some effective techniques:
- Print Statements: Use print statements to see how your code is running and where it might be going wrong.
- Using Debuggers: Debuggers let you go through your code step by step, checking variables and flow.
- Understanding Common Errors: Learn about common mistakes like syntax errors and logical errors to fix them quickly.
Debugging is not just about fixing errors; it’s about understanding your code better.
Learning from Bugs and Errors
When you encounter bugs, take the time to analyze them. Here are some steps to follow:
- Identify the specific error.
- Gather information on how to reproduce the issue.
- Document your findings to help with future problems.
By mastering testing and debugging, you can improve your coding skills and create better software.
Practicing Regularly
Engaging with Coding Challenges
Regular practice is essential for improving your coding skills. Here are some effective ways to practice:
- Solve coding challenges on platforms like LeetCode and HackerRank.
- Participate in coding competitions to test your skills against others.
- Contribute to open-source projects to gain real-world experience.
Diversifying Your Problem-Solving Portfolio
It’s important to tackle a variety of problems. This helps you develop a well-rounded skill set. Consider the following:
- Explore different types of problems such as algorithms, data structures, and system design.
- Work on projects that interest you to keep your motivation high.
- Join coding communities to learn from others and share your experiences.
Seeking Feedback and Continuous Improvement
After practicing, always look for ways to improve:
- Ask peers for feedback on your solutions.
- Review your code for efficiency and clarity.
- Learn from mistakes to avoid repeating them in the future.
Remember, consistent practice is key to mastering coding. By regularly engaging with challenges and learning from your experiences, you will enhance your problem-solving abilities and become a more effective coder. It is important to practice making observations on your own, and you should be solving problems in the range more and more often as you go down the problem list.
Learning from Others
Collaborating with Peers
Programming is a team effort. Working with others can help you learn faster and gain new insights. Here are some ways to collaborate:
- Join coding meetups to meet fellow developers.
- Participate in online forums and communities.
- Engage in code reviews to get feedback on your work.
Seeking Feedback and Mentorship
Finding a mentor can be a game-changer. A mentor can guide you through challenges and help you grow. Consider these steps:
- Reach out to experienced developers in your network.
- Ask for constructive criticism on your projects.
- Be open to learning from their experiences.
Studying Other People’s Code
Reading code written by others is a great way to improve your skills. Here’s how to do it effectively:
- Explore open-source projects on platforms like GitHub.
- Analyze well-documented libraries to understand best practices.
- Review code snippets shared in online communities.
Learning from others not only enhances your skills but also helps you avoid common mistakes. Embrace the knowledge shared by the community to become a better coder.
By engaging with others, you can boost your coding skills and build a supportive network. Remember, programming is not just about writing code; it’s about sharing knowledge and growing together!
Staying Updated with Industry Trends
Following Technology Blogs and Webinars
Staying informed about the latest developments in technology is crucial for any coder. Here are some effective ways to keep up:
- Subscribe to newsletters from reputable tech blogs.
- Attend webinars hosted by industry experts.
- Follow influential developers on social media for real-time updates.
Participating in Coding Communities
Engaging with others in the coding community can provide valuable insights. Consider:
- Joining online forums like Stack Overflow.
- Participating in local coding meetups.
- Contributing to discussions in open-source projects.
Exploring New Tools and Frameworks
The tech landscape is always changing. To stay relevant, you should:
- Experiment with new programming languages.
- Learn about emerging frameworks.
- Understand the latest software development trends. For instance, embracing automation in production processes is a key trend to watch in 2024.
Staying updated is not just about learning new skills; it’s about adapting to the ever-evolving tech world. Continuous learning is essential for success in coding.
Preparing for Coding Interviews
Understanding Common Interview Questions
Coding interviews often focus on a few key areas. Here are some common topics you should be familiar with:
- Data Structures: Arrays, linked lists, trees, and graphs.
- Algorithms: Sorting, searching, and dynamic programming.
- System Design: For senior roles, understanding how to design scalable systems is crucial.
Practicing Mock Interviews
Mock interviews are a great way to prepare. Here’s how to make the most of them:
- Simulate Real Conditions: Set a timer and solve problems without looking up solutions.
- Engage with Peers: Practice with friends or use platforms like Pramp.
- Seek Feedback: After each mock interview, ask for constructive criticism to improve.
Handling Time Constraints Effectively
In coding interviews, time management is key. Here are some tips:
- Set Time Limits: Practice solving problems within a set time.
- Prioritize Tasks: Focus on the most important parts of the problem first.
- Stay Calm: If you get stuck, take a deep breath and think through the problem logically.
Remember, coding interviews are not just about finding the right answer; they are about showing your thought process and problem-solving skills. Embrace each opportunity to learn and grow!
Advanced Problem-Solving Techniques
Dynamic Programming
Dynamic programming is a powerful method used to solve complex problems by breaking them down into simpler sub-problems. This technique is especially useful when problems have overlapping subproblems. Instead of solving the same sub-problem multiple times, you solve it once and store the result for future reference. This can save a lot of time and effort.
Greedy Algorithms
Greedy algorithms work by making the best choice at each step, hoping that these local optimums will lead to a global optimum. This approach is effective when the problem has an optimal substructure, meaning that an optimal solution can be built from optimal solutions of its subproblems. Here are some key points about greedy algorithms:
- They are often easier to implement than other methods.
- They can be faster in terms of execution time.
- However, they do not always guarantee the best solution.
Divide and Conquer
Divide and conquer is another effective strategy. This technique involves dividing the problem into smaller parts, solving each part independently, and then combining the results. This method is particularly useful for problems that can be broken down into similar sub-problems. Here’s how it works:
- Divide the problem into smaller sub-problems.
- Conquer each sub-problem recursively.
- Combine the solutions of the sub-problems to get the final solution.
By mastering these advanced techniques, you can tackle a wide range of coding challenges more effectively. Embrace these strategies to enhance your problem-solving skills and become a more proficient coder!
Building a Diverse Problem-Solving Portfolio
Tackling Different Types of Problems
Building a diverse problem-solving portfolio is essential for any developer. It helps you gain experience and confidence. Here are some ways to tackle various problems:
- Work on different projects: Engage in personal, open-source, or team projects.
- Explore various domains: Try problems in web development, data science, or mobile apps.
- Challenge yourself: Solve problems that push your limits and expand your skills.
Balancing Breadth and Depth
It’s important to have a mix of skills. Here’s how to balance your portfolio:
- Focus on core skills: Master key programming languages and frameworks.
- Learn new technologies: Stay updated with the latest tools and trends.
- Reflect on your experiences: Regularly review what you’ve learned and how you can improve.
Reflecting on Past Solutions
Learning from your past work is crucial. Consider these points:
- Document your solutions: Keep a record of how you solved problems.
- Analyze your mistakes: Understand what went wrong and how to avoid it next time.
- Seek feedback: Share your solutions with peers to gain insights.
Building a diverse portfolio not only enhances your skills but also prepares you for real-world challenges.
By following these strategies, you can create a strong problem-solving portfolio that showcases your abilities and readiness for any coding challenge.
Creating a varied problem-solving portfolio is essential for anyone looking to excel in coding. By practicing different types of challenges, you can sharpen your skills and boost your confidence. Don’t wait any longer—visit our website to start your coding journey today!
Conclusion
In conclusion, becoming skilled at solving coding problems is essential for every programmer. This journey involves understanding the problem well, planning your approach, picking the right tools, and creating effective solutions. It’s not just about knowing how to code; it’s also about being creative and sticking with it, even when things get tough. By practicing regularly and learning from your mistakes, you can turn tricky problems into simple solutions, which helps you grow as a coder. Remember, every expert started as a beginner. With hard work and a love for coding, you can become a confident programmer ready to face any challenge. Keep practicing, keep learning, and enjoy the process!
Frequently Asked Questions
How can I get better at solving coding problems?
To improve your coding problem-solving skills, practice regularly. Websites like LeetCode and HackerRank offer many challenges to help you sharpen your skills. Also, learning about different algorithms and data structures can provide you with various methods to tackle problems.
What programming language should I use for coding challenges?
The best language for coding challenges depends on what you are comfortable with. Many beginners find Python helpful because it is easy to understand, but you can use any language you like.
What should I do if I can’t solve a problem?
If you get stuck, break the problem down into smaller parts and try to solve those first. If you’re still having trouble, look for help online or take a break. Sometimes, stepping away can help you see the solution more clearly.
Are there common mistakes beginners make in coding?
Yes, some common mistakes include not fully understanding the problem before coding, neglecting edge cases, and not testing their code thoroughly. It’s important to have a clear plan before you start coding.
How crucial is it to understand algorithms for coding?
Understanding algorithms is very important. An algorithm is a step-by-step guide to solving a problem. Knowing various algorithms helps you choose the best one for the task at hand.
Is there a single best way to solve coding problems?
Not really. There are often multiple solutions to a coding problem. Some may be more efficient or easier to read than others, but the best solution can depend on different factors, like the problem’s requirements.
How can I learn from my coding mistakes?
When you make a mistake, take the time to understand what went wrong. Debugging is a valuable learning experience. Each error teaches you something new and helps you improve your skills.
How can I stay updated with coding trends?
To stay current, read technology blogs, attend webinars, and join coding communities. Engaging with others in the field can help you learn about new tools and best practices.