Unlocking the Power of Recursion Techniques Explained: A Comprehensive Guide
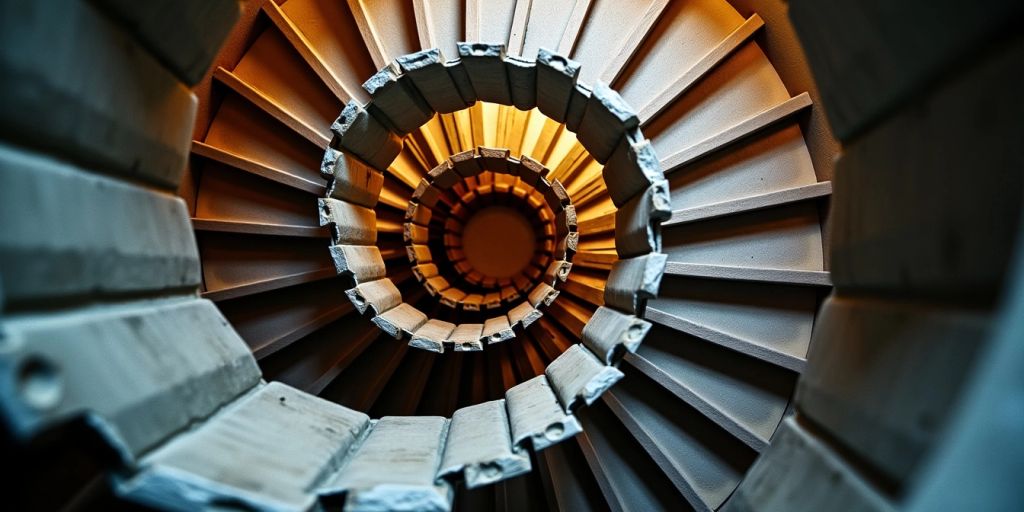
Recursion is a powerful programming tool that allows functions to call themselves to solve problems. It can seem tricky at first, but once you grasp the basics, it becomes a valuable skill. This guide will help you understand recursion from the ground up, covering its key concepts, applications, and techniques. Whether you’re a beginner or looking to enhance your coding skills, this comprehensive guide will unlock the secrets of recursion for you.
Key Takeaways
- Recursion is when a function calls itself to solve smaller parts of a problem.
- Every recursive function needs a base case to stop the calls and prevent infinite loops.
- Common uses of recursion include sorting, navigating trees, and solving math problems.
- Techniques like backtracking and memoization can make recursive functions more efficient.
- Visualizing recursion through call stacks can help understand how functions work.
Understanding the Fundamentals of Recursion
What is Recursion?
Recursion is a programming technique where a function calls itself to solve smaller parts of a problem. At its core, recursive lookup is a method used in programming to navigate through data structures that are layered or hierarchical, such as trees or graphs. This self-referencing can be tricky at first, but it’s a powerful tool once you get the hang of it.
Why Learn Recursion?
Learning recursion can help you tackle complex problems more easily. Here are some reasons to understand this concept:
- It simplifies problem-solving by breaking tasks into smaller, manageable parts.
- It enhances your coding skills and makes your code cleaner.
- It prepares you for coding interviews, where recursion is often tested.
Base Case and Recursive Case
Every recursive function has two main parts: the base case and the recursive case.
- Base Case: This is the simplest version of the problem that can be solved directly. For example, in a countdown function, when the number reaches zero, it stops calling itself.
- Recursive Case: This is where the function calls itself with a new argument that gets closer to the base case. For instance, in a factorial function, it keeps calling itself with a smaller number until it hits the base case.
Understanding these two parts is crucial for writing effective recursive functions. Without a base case, the function would keep calling itself forever, leading to errors.
By mastering these fundamentals, you’ll be well on your way to unlocking the full potential of recursion in programming!
Common Applications of Recursion
Recursion is a powerful technique used in various programming scenarios. It allows us to solve complex problems by breaking them down into smaller, more manageable parts. Here are some common applications:
Sorting Algorithms
- Quick Sort: This algorithm divides the array into smaller parts and sorts them recursively.
- Merge Sort: It splits the array into halves, sorts each half, and then merges them back together.
- Heap Sort: This method uses a binary heap to sort elements recursively.
Tree and Graph Traversal
Recursive algorithms are powerful tools for traversing and manipulating tree structures. They offer elegant solutions for:
- Preorder Traversal: Visit the root, then the left subtree, followed by the right subtree.
- Inorder Traversal: Visit the left subtree, then the root, and finally the right subtree.
- Postorder Traversal: Visit the left subtree, the right subtree, and then the root.
Mathematical Computations
Recursion is also useful in solving mathematical problems, such as:
- Factorial Calculation: Finding the product of all positive integers up to a given number.
- Fibonacci Sequence: Generating a sequence where each number is the sum of the two preceding ones.
- Power Calculation: Calculating the result of raising a number to a certain exponent.
Recursion simplifies complex problems by breaking them down into smaller, easier-to-solve parts. This approach not only makes the code cleaner but also enhances efficiency in many cases.
Exploring Recursive Algorithms
Factorial Calculation
Calculating the factorial of a number is a classic example of recursion. The factorial of a number n (denoted as n!) is the product of all positive integers up to n. The recursive formula is:
- Base Case: If n is 0, return 1.
- Recursive Case: Return n multiplied by the factorial of (n-1).
Here’s how it looks in code:
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n - 1)
Fibonacci Sequence
The Fibonacci sequence is another popular example of recursion. Each number in the sequence is the sum of the two preceding ones. The recursive formula is:
- Base Cases: If n is 0, return 0; if n is 1, return 1.
- Recursive Case: Return the sum of the Fibonacci of (n-1) and (n-2).
Here’s the code:
def fibonacci(n):
if n == 0:
return 0
elif n == 1:
return 1
else:
return fibonacci(n - 1) + fibonacci(n - 2)
Tower of Hanoi
The Tower of Hanoi is a classic puzzle that illustrates recursion beautifully. The goal is to move a stack of disks from one pole to another, following these rules:
- Only one disk can be moved at a time.
- A disk can only be placed on top of a larger disk.
- All disks start on one pole.
The recursive solution involves moving smaller stacks of disks between poles. Here’s the code:
def tower_of_hanoi(n, source, destination, auxiliary):
if n == 1:
print(f"Move disk 1 from {source} to {destination}")
return
tower_of_hanoi(n - 1, source, auxiliary, destination)
print(f"Move disk {n} from {source} to {destination}")
tower_of_hanoi(n - 1, auxiliary, destination, source)
Recursion is a powerful tool that simplifies complex problems by breaking them down into smaller, manageable tasks. Understanding these algorithms is essential for mastering recursion.
In summary, recursive algorithms like factorial calculation, Fibonacci sequence, and Tower of Hanoi showcase the elegance and power of recursion. They help in solving problems that can be divided into smaller, similar problems, making them easier to tackle.
Summary Table of Recursive Algorithms
Algorithm | Description |
---|---|
Factorial | Product of all positive integers up to n. |
Fibonacci | Sum of the two preceding numbers. |
Tower of Hanoi | Move disks between poles following specific rules. |
Advanced Recursion Techniques
Backtracking
Backtracking is a powerful technique used to solve problems incrementally. It builds candidates for solutions and abandons them if they are not valid. This method is often used in puzzles and games. Here are some common applications:
- Solving mazes
- N-Queens problem
- Sudoku puzzles
Memoization
Memoization is an optimization technique that stores the results of expensive function calls and returns the cached result when the same inputs occur again. This can significantly speed up recursive algorithms. For example:
- Fibonacci sequence: Instead of recalculating values, store them in a list.
- Dynamic programming: Use memoization to solve complex problems efficiently.
Divide and Conquer
Divide and conquer is a strategy that breaks a problem into smaller subproblems, solves each subproblem independently, and combines their results. This technique is widely used in algorithms like:
- Merge Sort
- Quick Sort
- Binary Search
Mastering these advanced techniques can greatly enhance your problem-solving skills in programming. They allow you to tackle complex challenges with ease and efficiency.
In summary, understanding these advanced recursion techniques can help you unlock the full potential of recursion in your coding journey. Each technique has its unique applications and can be a game-changer in solving intricate problems.
Visualizing Recursion
To truly grasp the concept of recursion, it’s essential to visualize the process. This can be done through various methods that help illustrate how recursive functions operate. Here are some key techniques:
Call Stack Representation
- Call Stack: This is a structure that keeps track of active function calls. Each time a function calls itself, a new layer is added to the stack.
- Visualizing Layers: Imagine each function call as a layer in a cake. The top layer is the most recent call, while the bottom layer is the first.
- Unwinding: When the base case is reached, the stack starts to unwind, returning values back through the layers.
Tracing Recursive Calls
- Step-by-Step Tracing: Write down each function call and its parameters. This helps in understanding how the function progresses.
- Example: For a function that calculates the factorial, you can trace how it calls itself with decreasing values until it reaches 1.
- Flowchart: Create a flowchart to visualize the decision-making process of the recursive function.
Debugging Recursive Functions
- Print Statements: Use print statements to show when a function is called and when it returns. This can clarify the flow of execution.
- Visual Debuggers: Many programming environments have tools that allow you to step through code, showing the call stack in real-time.
- Common Pitfalls: Be aware of infinite loops or missing base cases, which can lead to stack overflow errors.
Understanding recursion is not just about coding; it’s about developing a mindset that embraces problem-solving through self-referential techniques. By visualizing recursion, you can enhance your ability to tackle complex programming challenges.
By employing these visualization techniques, you can demystify recursion and improve your coding skills significantly. Visual aids can make a big difference in understanding how recursive functions work and how to implement them effectively.
Handling Multiple Recursive Calls
Understanding Multiple Calls
Some recursive functions involve more than one recursive call. This is often seen in problems like the Fibonacci sequence, where each number is the sum of the two previous numbers. For example:
def fibonacci(n):
if n == 0:
return 0
elif n == 1:
return 1
else:
return fibonacci(n-1) + fibonacci(n-2)
print(fibonacci(5))
In this function, when n
is greater than 1, it calls itself twice, creating multiple branches of recursion.
Examples of Multiple Calls
Here are some common scenarios where multiple recursive calls are used:
- Fibonacci Sequence: Each number is derived from the sum of the two preceding numbers.
- Permutations: Generating all possible arrangements of a set of items.
- Tree Traversals: Navigating through nodes in a tree structure.
Optimizing Multiple Calls
To make recursive functions more efficient, consider these strategies:
- Memoization: Store results of expensive function calls and reuse them when the same inputs occur again.
- Dynamic Programming: Break down problems into simpler subproblems and solve them just once.
- Tail Recursion: Optimize recursive calls to avoid stack overflow by ensuring the recursive call is the last operation in the function.
Understanding how to handle multiple recursive calls is crucial for solving complex problems efficiently.
Recursion in Data Structures
Binary Trees
Binary trees are a common data structure where recursion shines. Each node in a binary tree can have up to two children, making it a perfect candidate for recursive functions. Traversing a binary tree can be done using recursion in various ways:
- In-order Traversal: Visit the left child, then the node, and finally the right child.
- Pre-order Traversal: Visit the node first, then the left child, followed by the right child.
- Post-order Traversal: Visit the left child, then the right child, and finally the node.
Linked Lists
Linked lists are another structure where recursion is useful. Each element points to the next, allowing for recursive operations like:
- Reversing a Linked List: You can reverse the list by recursively changing the pointers.
- Finding an Element: Search for an element by checking each node recursively.
- Counting Nodes: Count the number of nodes by recursively traversing the list.
Graphs
Graphs can be complex, but recursion helps simplify many operations. For example:
- Depth-First Search (DFS): This algorithm explores as far as possible along each branch before backtracking, using recursion to visit nodes.
- Finding Connected Components: You can use recursion to explore all nodes connected to a starting node.
- Cycle Detection: Recursion can help determine if a cycle exists in a graph.
Recursion is a powerful tool in programming, especially when dealing with complex data structures. It allows us to break down problems into smaller, manageable parts, making it easier to solve them.
In summary, recursion plays a vital role in working with data structures like binary trees, linked lists, and graphs. By understanding how to apply recursion effectively, you can tackle a variety of programming challenges with confidence. Mastering recursion not only enhances your coding skills but also improves your problem-solving abilities.
Practical Examples of Recursion
Inverting a Binary Tree
Inverting a binary tree is a classic example of recursion. The idea is to swap the left and right children of each node. Here’s how it works:
- Check if the node is null. If it is, return null.
- Swap the left and right children of the current node.
- Recursively call the function on the left and right children.
class TreeNode:
def __init__(self, x):
self.val = x
self.left = None
self.right = None
def invert_tree(node):
if node is None:
return None
node.left, node.right = invert_tree(node.right), invert_tree(node.left)
return node
Generating Subsets
Generating all subsets of a set is another great example of recursion. The process involves:
- Including the current element in the subset.
- Excluding the current element from the subset.
- Recursively call the function for the next element.
Here’s a simple implementation:
def generate_subsets(s, current=[], index=0):
if index == len(s):
print(current)
return
generate_subsets(s, current + [s[index]], index + 1)
generate_subsets(s, current, index + 1)
Permutations of a String
Permutations of a string can also be solved using recursion. The steps are:
- Swap each character with the first character.
- Recursively call the function for the remaining characters.
- Backtrack to restore the original string.
Here’s how it looks in code:
def permute_string(s, l, r):
if l == r:
print(''.join(s))
else:
for i in range(l, r + 1):
s[l], s[i] = s[i], s[l]
permute_string(s, l + 1, r)
s[l], s[i] = s[i], s[l]
Recursion is a powerful tool that allows us to break down complex problems into simpler ones. By practicing these examples, you can enhance your understanding of how recursion works and its applications in programming.
These examples show how recursion can be applied in various scenarios, making it a versatile technique in programming. Understanding these concepts will help you tackle more complex problems with confidence.
Optimizing Recursive Functions
Tail Recursion
Tail recursion is a special case where the recursive call is the last operation in the function. This allows some programming languages to optimize the call stack, preventing stack overflow. Using tail recursion can significantly enhance performance.
Avoiding Stack Overflow
To prevent stack overflow errors, consider these strategies:
- Limit recursion depth: Ensure your base case is reached quickly.
- Use iterative solutions: Sometimes, converting recursion to iteration can be more efficient.
- Increase stack size: In some programming environments, you can adjust the stack size to accommodate deeper recursion.
Improving Efficiency
To improve the efficiency of recursive functions, we can use techniques like memoization and dynamic programming. For example, in the Fibonacci sequence, instead of recalculating values, we store them:
Fibonacci Number | Value |
---|---|
0 | 0 |
1 | 1 |
2 | 1 |
3 | 2 |
4 | 3 |
5 | 5 |
6 | 8 |
7 | 13 |
8 | 21 |
9 | 34 |
10 | 55 |
By understanding and applying these optimization techniques, you can unlock the full potential of recursion in your programming tasks.
Challenges and Pitfalls of Recursion
Common Mistakes
Recursion can be tricky, and many beginners make mistakes. Here are some common pitfalls:
- Missing Base Case: Without a base case, the function will keep calling itself forever, leading to a crash.
- Incorrect Recursive Case: If the recursive case doesn’t bring you closer to the base case, it can also cause infinite loops.
- Stack Overflow: Too many recursive calls can fill up the call stack, causing a program to crash.
Performance Issues
Recursion can be expensive in terms of memory and time. Here are some factors to consider:
- Memory Usage: Each recursive call takes up space in memory, which can lead to high usage.
- Time Complexity: Some recursive algorithms, like the naive Fibonacci sequence, can be slow because they repeat calculations.
- Debugging Difficulty: Recursive functions can be hard to debug due to their complex flow.
Debugging Tips
When debugging recursive functions, keep these tips in mind:
- Print Statements: Use print statements to track the flow of the function.
- Visualize the Call Stack: Draw the call stack to see how the function calls itself.
- Test with Simple Cases: Start with simple inputs to ensure the function behaves as expected.
Recursion is a powerful tool, but it requires careful handling to avoid common pitfalls. Understanding these challenges can help you write better recursive functions.
Recursion in Real-World Applications
File System Navigation
Recursion is often used in file systems to navigate through directories and subdirectories. When searching for a file, a recursive function can check each folder and its subfolders until it finds the target file. This method is efficient and simplifies the search process.
Network Protocols
In networking, recursion helps in resolving domain names. When you type a URL, recursive lookup is used to find the corresponding IP address. This process involves multiple steps, where each step may require looking up additional information, making recursion a vital tool in network communication.
Game Development
In game development, recursion can be used for various tasks, such as pathfinding algorithms. For example, when a character needs to find the shortest route to a destination, recursive techniques can help explore all possible paths efficiently.
Recursion is a powerful tool that simplifies complex problems by breaking them down into smaller, manageable parts. Here are some key applications:
- File System Navigation: Searching through directories.
- Network Protocols: Resolving domain names.
- Game Development: Pathfinding algorithms.
Recursion allows programmers to tackle complex problems by dividing them into simpler tasks, making it easier to manage and solve them effectively.
Summary Table of Applications
Application Area | Description |
---|---|
File System Navigation | Searching for files in nested directories. |
Network Protocols | Resolving domain names to IP addresses. |
Game Development | Finding paths in game environments. |
Recursion is a powerful tool used in many real-life situations, from solving puzzles to managing complex data. It helps break down big problems into smaller, easier parts. If you want to learn how to use recursion effectively and improve your coding skills, visit our website today!
Conclusion
In summary, recursion is a key tool in programming that helps break down tough problems into smaller, easier parts. By using recursion, you can solve complex tasks more simply and clearly. This method is not just useful for coding interviews; it also helps you think better about how to tackle problems. Whether you’re just starting out or have been coding for a while, getting the hang of recursion can open up new ways to solve challenges. So, as you continue your coding journey, remember that mastering recursion can make you a better programmer and help you create more efficient solutions.
Frequently Asked Questions
What is recursion in simple terms?
Recursion is when a function calls itself to solve a problem. It’s like a task that can be broken down into smaller, similar tasks.
Why is recursion important to learn?
Learning recursion helps you understand how to break down complex problems into easier parts. It’s a valuable skill for coding interviews and improves your problem-solving abilities.
What is a base case in recursion?
The base case is the simplest part of a problem that can be solved without further recursion. It helps stop the function from calling itself forever.
Can you give an example of recursion?
Sure! A common example is calculating the factorial of a number, where the function keeps calling itself with a smaller number until it reaches 1.
What are some common uses of recursion?
Recursion is often used in sorting data, navigating trees or graphs, and solving mathematical problems like the Fibonacci sequence.
How can I visualize recursion?
You can visualize recursion by thinking of a call stack, which shows how functions call each other. You can also trace the steps of the function to see how it works.
What are the challenges of using recursion?
Some challenges include running into performance issues if the recursion goes too deep, which can lead to stack overflow errors. It’s also easy to make mistakes if you don’t set the base case correctly.
How can I optimize recursive functions?
You can optimize them by using techniques like tail recursion, which helps reduce the amount of memory used, or memoization, which remembers previous results to speed up calculations.