Mastering the Art of Understanding Recursion: A Comprehensive Guide
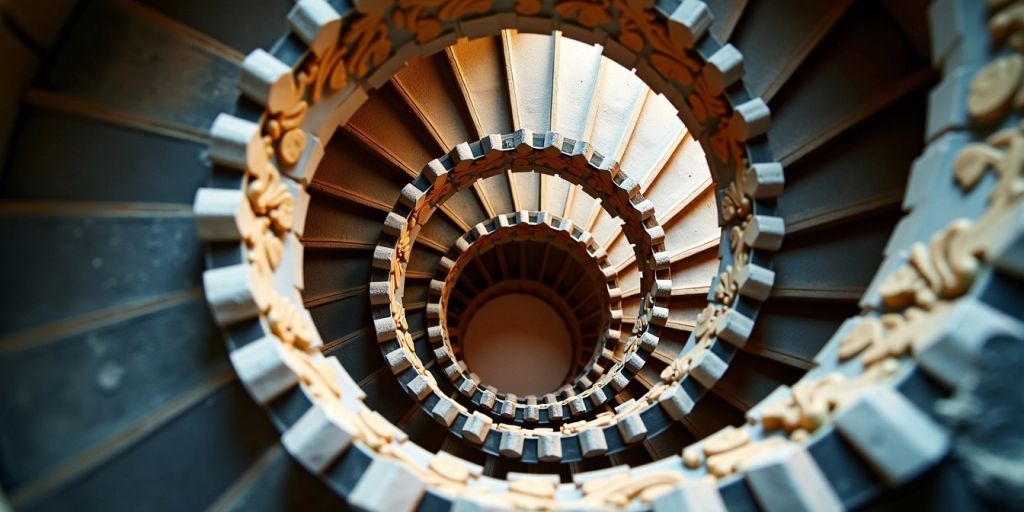
Recursion is a powerful concept in programming that allows functions to call themselves to solve problems. This guide will help you understand recursion, its principles, and how to apply it effectively in various programming scenarios. Whether you’re new to coding or looking to sharpen your skills, this comprehensive guide will make recursion easier to grasp and use in your projects.
Key Takeaways
- Recursion involves a function calling itself to break down complex problems into simpler parts.
- Every recursive function needs a base case to stop the recursion and a recursive case to continue it.
- Recursive algorithms are often more elegant but can be less efficient than iterative solutions.
- Common recursive techniques include backtracking and divide-and-conquer strategies.
- Understanding recursion is essential for solving problems in coding interviews and real-world applications.
The Fundamentals of Understanding Recursion
Defining Recursion in Programming
Recursion is a method where a function calls itself to solve smaller parts of the same problem. This technique allows programmers to break down complex tasks into simpler ones. For example, when calculating a factorial, the function will call itself with a smaller number until it reaches the base case.
The Base Case and Recursive Case
Every recursive function needs two main parts:
- Base Case: This is the condition that stops the recursion. Without it, the function would keep calling itself forever.
- Recursive Case: This is where the function calls itself with a modified argument, moving it closer to the base case.
Component | Description |
---|---|
Base Case | Stops the recursion |
Recursive Case | Calls the function again with a smaller input |
Common Misconceptions About Recursion
Many people think recursion is only for advanced programmers. However, it can be quite simple once you understand the basics. Here are some common myths:
- Recursion is always slower than iteration: This isn’t true; sometimes recursion can be more efficient.
- You need to know everything about recursion to use it: You just need to understand the base and recursive cases.
- Recursion is only for specific problems: In reality, it can be applied to many different types of problems.
Recursion is a powerful tool that can simplify complex problems, making them easier to solve. Understanding its fundamentals is the first step to mastering it.
The Principles Behind Recursive Algorithms
Divide and Conquer Strategy
Recursion often uses a method called divide and conquer. This means breaking a big problem into smaller, easier parts. Here’s how it works:
- Identify the main problem.
- Split it into smaller problems.
- Solve each smaller problem.
- Combine the results.
Self-Similarity in Problems
In recursion, smaller problems often look like the original problem. This is known as self-similarity. For example, when calculating the factorial of a number, the function calls itself with a smaller number until it reaches the base case.
Termination Criteria
Every recursive function needs a termination criterion. This is a condition that tells the function when to stop calling itself. Without it, the function could run forever, leading to errors like stack overflow. The base case is the simplest form of the problem, which can be solved directly without further recursion.
Understanding these principles is crucial for mastering recursion. They help in breaking down complex problems into manageable parts, making it easier to find solutions.
Summary Table
Principle | Description |
---|---|
Divide and Conquer | Breaks problems into smaller parts |
Self-Similarity | Smaller problems resemble the original problem |
Termination Criteria | Condition to stop recursion |
Comparing Recursion and Iteration
When to Use Recursion
Recursion is often a great choice when:
- The problem can be broken down into smaller, similar problems.
- You want a solution that is easier to read and understand.
- The task involves structures like trees or graphs.
Recursion can simplify complex problems.
When to Use Iteration
Iteration is usually preferred when:
- The problem can be solved with loops.
- You need better performance and efficiency.
- The task involves a large number of repetitions.
Performance Considerations
Here’s a quick comparison of recursion and iteration:
Aspect | Recursion | Iteration |
---|---|---|
Memory Usage | Higher (due to call stack) | Lower (uses loops) |
Readability | Often clearer for complex tasks | Can be less clear for complex tasks |
Speed | Slower due to function calls | Generally faster |
In many cases, recursion is simpler to code and understand than iteration, but it can be less efficient.
Understanding when to use each method is key to effective programming. By weighing the pros and cons, you can choose the best approach for your specific problem.
Classic Recursive Algorithms
Recursion is a powerful tool in programming, and several classic algorithms showcase its effectiveness. Here, we will explore three well-known recursive algorithms: the Tower of Hanoi, the Flood Fill Algorithm, and Binary Search.
The Tower of Hanoi
The Tower of Hanoi is a classic puzzle that involves moving disks from one peg to another, following specific rules. The recursive solution can be summarized in three steps:
- Move the top n-1 disks from the source peg to an auxiliary peg.
- Move the nth disk directly to the destination peg.
- Move the n-1 disks from the auxiliary peg to the destination peg.
Key Insight: This algorithm beautifully illustrates how recursion can simplify complex problems by breaking them down into smaller tasks.
Flood Fill Algorithm
The Flood Fill Algorithm is commonly used in graphics applications, such as paint bucket tools. It works by filling a connected area with a specific color. The recursive approach involves:
- Checking if the current pixel matches the target color.
- Changing the pixel’s color to the new color.
- Recursively applying the same process to adjacent pixels (up, down, left, right).
Binary Search
The binary search algorithm is an efficient way to find an item in a sorted list. The recursive version works as follows:
- Compare the target value to the middle element of the list.
- If the target is equal to the middle element, return its index.
- If the target is less than the middle element, search the left half.
- If the target is greater, search the right half.
This method significantly reduces the number of comparisons needed, making it much faster than a linear search.
Recursion allows us to solve problems in a more elegant way, often leading to clearer and more concise code.
In summary, classic recursive algorithms like the Tower of Hanoi, Flood Fill, and Binary Search demonstrate the versatility and power of recursion in programming. Understanding these algorithms can help you master the art of recursion and apply it effectively in your coding endeavors.
Advanced Recursive Techniques
Backtracking Algorithms
Backtracking is a powerful technique used to solve problems incrementally. It explores all possible solutions and abandons those that fail to satisfy the conditions. Here are some key points about backtracking:
- Explores all options: It tries every possible path until it finds a solution.
- Prunes paths: If a path doesn’t lead to a solution, it stops exploring that route.
- Commonly used in puzzles: Problems like Sudoku and the N-Queens problem utilize backtracking.
Tree Traversal Methods
Tree traversal is essential for processing data structures like trees. There are several methods to traverse trees:
- In-order: Visit the left subtree, the node, and then the right subtree.
- Pre-order: Visit the node first, then the left and right subtrees.
- Post-order: Visit the left and right subtrees before the node.
These methods help in various applications, such as searching and sorting.
Divide-and-Conquer Algorithms
Divide-and-conquer is a strategy that breaks a problem into smaller sub-problems, solves each one, and combines the results. This technique is effective for:
- Sorting: Algorithms like Merge Sort and Quick Sort.
- Searching: Binary Search is a classic example.
- Complex problems: It simplifies problems by reducing their size at each step.
Recursion is not just a programming technique; it reflects patterns in nature and problem-solving.
In conclusion, mastering these advanced recursive techniques can significantly enhance your programming skills and problem-solving abilities. Understanding how to apply backtracking, tree traversal, and divide-and-conquer strategies will make you a more effective programmer. Remember, most algorithms that utilize recursion can also be implemented iteratively, but recursion often provides a clearer and more elegant solution.
Practical Applications of Recursion
Recursion is not just a theoretical concept; it has many real-world uses that make it a valuable tool in programming. Here are some practical applications:
File System Search
- Recursion can be used to navigate through directories and files.
- It allows for searching through nested folders efficiently.
- Each folder can be treated as a smaller problem, making it easier to find files.
Drawing Fractals
- Fractals are complex patterns that can be created using recursive functions.
- They are found in nature, like snowflakes and trees.
- Recursive algorithms can generate these patterns by repeating simple shapes.
Maze Generation
- Recursion helps in creating mazes by dividing spaces into smaller sections.
- It can also be used to find paths through the maze.
- This method allows for complex maze designs with less code.
Recursion simplifies complex problems by breaking them down into smaller, manageable parts. This approach not only makes coding easier but also enhances understanding of the problem at hand.
In summary, recursion is a powerful technique that can be applied in various fields, especially in data structures. Recursive algorithms are powerful tools for traversing and manipulating tree structures. They offer elegant solutions for preorder, inorder, and postorder traversals, making them essential in programming.
Optimizing Recursive Functions
Memoization Techniques
Memoization is a powerful optimization technique that stores the results of expensive function calls and returns the cached result when the same inputs occur again. This can significantly reduce the time complexity of recursive functions. Here are some key points about memoization:
- Store results: Keep a record of previously computed results.
- Check cache first: Before performing calculations, check if the result is already available.
- Use data structures: Commonly, a dictionary or hash map is used for storing results.
Tail Call Optimization
Tail call optimization (TCO) is a technique that allows recursive functions to avoid stack overflows by unwinding the call stack and starting from the global context. This is particularly useful in languages that support it, such as R. Here’s how it works:
- Identify tail calls: A tail call is a function call that is the last action in a function.
- Optimize the call: The compiler can optimize the call to reuse the current function’s stack frame.
- Prevent stack overflow: This optimization helps in handling deep recursion without running out of stack space.
Dynamic Programming
Dynamic programming is another method to optimize recursive functions by breaking down problems into simpler subproblems and storing their solutions. Here’s a simple approach:
- Identify overlapping subproblems: Recognize when the same subproblem is solved multiple times.
- Store solutions: Use a table to store the results of subproblems.
- Build up solutions: Solve larger problems using the stored solutions of smaller problems.
Optimizing recursive functions can lead to significant performance improvements, making them more efficient and practical for real-world applications.
Testing Recursive Functions
Using Unit Tests
Testing recursive functions is crucial to ensure they work correctly. Here are some steps to follow:
- Identify the base case: Make sure your tests cover the simplest scenario where the function should return a result without further recursion.
- Test the recursive case: Create tests that check if the function correctly calls itself and processes the input as expected.
- Check edge cases: Consider unusual inputs, like empty arrays or negative numbers, to see how the function behaves.
Common Testing Frameworks
Several frameworks can help you test recursive functions effectively:
- Jest: A popular JavaScript testing library that makes it easy to write tests for recursive logic.
- JUnit: A widely used framework for testing Java applications, suitable for testing recursive methods.
- PyTest: A powerful testing tool for Python that can handle recursive functions well.
Debugging Recursive Code
Debugging recursion can be tricky. Here are some tips:
- Use print statements: Add print statements to track the function’s progress and see how many times it calls itself.
- Visualize the call stack: Understanding how the function calls itself can help identify where things go wrong.
- Limit recursion depth: Temporarily restrict the depth of recursion to make debugging easier.
Remember, testing is essential! It helps catch errors early and ensures your recursive functions perform as intended.
Example Testing with Jest
Here’s a simple example of how to test a recursive function using Jest:
const factorial = require('./factorial');
test('Factorial of 5 equals 120', () => {
expect(factorial(5)).toBe(120);
});
This test checks if the factorial function correctly calculates the factorial of 5.
Highlighted Concept
In Java, recursion is a process in which a function calls itself directly or indirectly. This is essential for understanding how recursive functions operate and how to test them effectively.
Recursion in Different Programming Languages
Recursion is a powerful concept that appears in many programming languages. It allows functions to call themselves to solve problems. Here, we will explore how recursion is implemented in three popular languages: Python, JavaScript, and functional languages.
Recursion in Python
Python is known for its simplicity and readability, making it a great choice for learning recursion. Here are some key points about recursion in Python:
- Base Case: This is the condition that stops the recursion.
- Recursive Case: This is where the function calls itself with a modified argument.
Example: Calculating the factorial of a number:
def factorial(n):
if n <= 1:
return 1 # Base case
return n * factorial(n - 1) # Recursive call
Recursion in JavaScript
JavaScript also supports recursion, especially for tasks that involve nested structures. Here are some important aspects:
- Base Case: The condition that ends the recursion.
- Recursive Case: The part where the function calls itself.
Example: Finding the Fibonacci sequence:
function fibonacci(n) {
if (n <= 1) return n; // Base cases
return fibonacci(n - 1) + fibonacci(n - 2); // Recursive call
}
Recursion in Functional Languages
Functional languages like Haskell and Lisp use recursion as a primary method for iteration. Here are some features:
- Tail Recursion: A special case where the recursive call is the last operation in the function, allowing for optimization.
- Immutable Data: Functional languages often use immutable data structures, which can make recursion more efficient.
Example: A simple recursive function in Haskell:
factorial n = if n <= 1 then 1 else n * factorial (n - 1)
Recursion is a fundamental concept in programming, allowing for elegant solutions to complex problems.
In summary, recursion is a versatile tool that can be used across various programming languages. Understanding how it works in different contexts can help you become a better programmer.
Language | Key Feature |
---|---|
Python | Simple syntax |
JavaScript | Dynamic capabilities |
Functional | Emphasis on immutability |
Real-World Examples of Recursion
Recursion is not just a programming concept; it appears in many aspects of our world. Here are some notable examples:
Factorial Calculation
The factorial of a number is a classic example of recursion. It is calculated by multiplying the number by the factorial of the number minus one. For instance:
- Factorial of 5: 5! = 5 × 4 × 3 × 2 × 1 = 120
- Factorial of 3: 3! = 3 × 2 × 1 = 6
- Factorial of 0: 0! = 1 (by definition)
Fibonacci Sequence
The Fibonacci sequence is another famous example where each number is the sum of the two preceding ones. The sequence starts as follows:
- 0, 1, 1, 2, 3, 5, 8, 13, …
- The formula is: F(n) = F(n-1) + F(n-2)
Depth-First Search (DFS)
In computer science, DFS is a method for traversing tree or graph structures. It explores as far as possible along each branch before backtracking. Here’s a simple breakdown:
- Start at the root node.
- Explore each branch before moving to the next sibling.
- Backtrack when you reach a leaf node.
Recursion helps simplify complex problems by breaking them down into smaller, manageable parts. This makes it easier to solve intricate issues.
Other Examples of Recursion in Nature
- Fractals: Patterns that repeat at different scales, like snowflakes or tree branches.
- Russian Dolls: Each doll contains a smaller version of itself, showcasing a physical representation of recursion.
- Storytelling: Some tales have stories within stories, creating a recursive narrative structure.
Recognizing these patterns helps emphasize that recursion is not just a programming paradigm. Instead, it’s a universal concept, reflecting the inherent structures and patterns in the world around us.
Challenges and Pitfalls of Recursion
Stack Overflow Issues
Recursion can lead to stack overflow errors if the recursion goes too deep. This happens when a function calls itself too many times without reaching a base case. Each call uses up memory, and if it exceeds the limit, the program crashes. To avoid this, always ensure that your recursive function has a clear base case.
Infinite Recursion
Another common problem is infinite recursion. This occurs when the base case is never met, causing the function to call itself endlessly. For example, if a function is supposed to reduce a number but mistakenly keeps it the same, it will never stop. To prevent this, double-check your logic to ensure that the function will eventually reach the base case.
Handling Large Inputs
Recursive functions can struggle with large inputs. They may take a lot of memory and time, making them inefficient. For instance, calculating Fibonacci numbers recursively can be slow for large values. In such cases, consider using iterative methods or optimizing with techniques like memoization.
Summary of Challenges
Here’s a quick summary of the main challenges:
- Stack Overflow: Too many recursive calls.
- Infinite Recursion: No base case reached.
- Large Inputs: High memory and time consumption.
Recursion is a powerful tool, but it requires careful handling to avoid common pitfalls. Always test your functions thoroughly to ensure they work as intended!
Recursion can be tricky! It’s easy to get lost in loops and endless calls, which can lead to mistakes and confusion. If you want to learn how to tackle these challenges and improve your coding skills, visit our website today! We offer free resources to help you get started on your coding journey!
Final Thoughts on Recursion
In conclusion, understanding recursion is like learning a new language in programming. It may seem tricky at first, but with practice, it becomes clearer. Recursion helps break down big problems into smaller, easier parts, making it a powerful tool for programmers. Remember, it’s important to have a base case to stop the function from running forever. As you continue to explore coding, keep recursion in mind; it can be very useful for certain tasks, especially when dealing with complex data structures like trees. With time and experience, you’ll find that recursion can be a valuable addition to your coding skills.
Frequently Asked Questions
What is recursion in programming?
Recursion is when a function calls itself to solve a problem. It breaks a big problem into smaller parts that are easier to handle.
Why do we need a base case in recursion?
A base case stops the recursion from going on forever. It tells the function when to stop calling itself.
What are some common examples of recursion?
Some common examples include calculating factorials, generating Fibonacci numbers, and searching through trees.
How does recursion differ from iteration?
Recursion uses functions calling themselves, while iteration uses loops to repeat actions. Recursion can be clearer for some problems.
Can recursion cause errors?
Yes, if not set up correctly, it can lead to stack overflow errors, where the program runs out of memory.
When should I use recursion?
Use recursion for problems that can be broken down into smaller, similar problems, like tree traversal or certain algorithms.
What is memoization in recursion?
Memoization is a technique to store results of expensive function calls and reuse them when the same inputs occur again.
Is recursion used in all programming languages?
Yes, most programming languages support recursion, but the way it’s implemented can vary.