Unlocking the Power of Python Scripting: A Beginner’s Guide to Automation and Efficiency
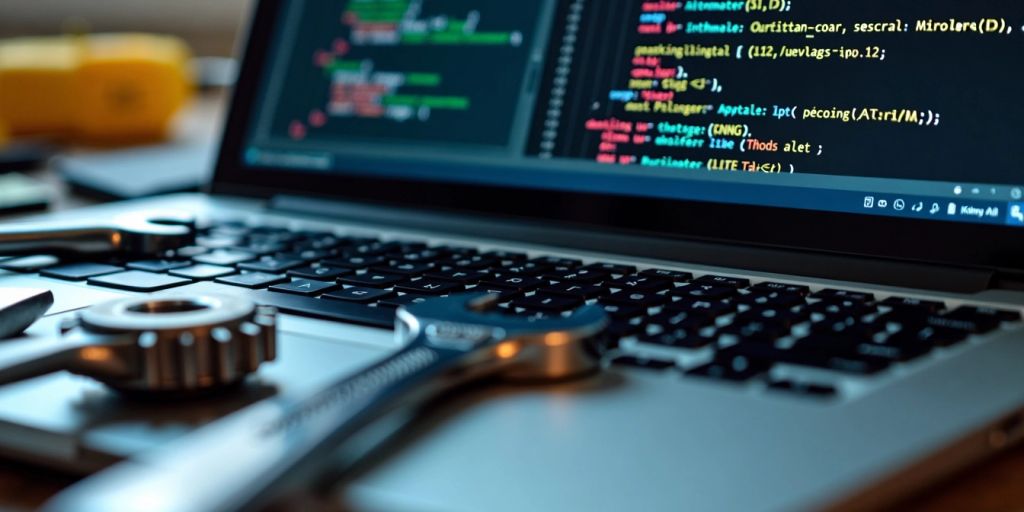
Python scripting is a powerful tool that can help you automate tasks and improve your efficiency. Whether you are just starting out or looking to enhance your skills, understanding the basics of Python can open up a world of possibilities. This guide will introduce you to essential concepts, tools, and techniques that can make your scripting journey smoother and more effective.
Key Takeaways
- Python scripting helps automate repetitive tasks, saving you time and effort.
- Setting up your Python environment is the first step to start scripting effectively.
- Popular libraries like Pandas and BeautifulSoup can simplify data handling and web scraping.
- Error handling is crucial; using try-except blocks can help catch and fix mistakes in your scripts.
- Best practices like writing clear code and documenting your work can make your scripts easier to understand and maintain.
Understanding the Basics of Python Scripting
What is Python Scripting?
Python scripting is a way to write small programs that automate tasks. Python is a powerful language that allows you to create scripts to perform various functions quickly and easily. It’s widely used for everything from simple tasks to complex applications.
Why Use Python for Scripting?
There are many reasons to choose Python for scripting:
- Easy to Learn: Python has a simple syntax that makes it beginner-friendly.
- Versatile: You can use Python for web development, data analysis, automation, and more.
- Large Community: There are many resources and libraries available, making it easier to find help.
Setting Up Your Python Environment
To start scripting in Python, you need to set up your environment. Here’s how:
- Download Python: Go to the official Python website and download the latest version.
- Install an IDE: Choose an Integrated Development Environment (IDE) like PyCharm or Visual Studio Code.
- Test Your Setup: Open your IDE and write a simple script to ensure everything is working.
Setting up your environment correctly is crucial for a smooth scripting experience.
Step | Description |
---|---|
1 | Download Python from the official site |
2 | Install an IDE for coding |
3 | Write a test script to check your setup |
By understanding these basics, you’ll be well on your way to how to write scripts in python and automate your tasks effectively!
Essential Python Libraries for Scripting
Introduction to Python Libraries
Python libraries are collections of pre-written code that help you perform specific tasks without having to write everything from scratch. They save time and make coding easier. Using libraries can greatly enhance your scripting capabilities.
Popular Libraries for Automation
Here are some of the most popular libraries you might want to use:
- TensorFlow: This library was developed by Google and is great for machine learning tasks.
- Matplotlib: This library helps you create graphs and plots to visualize data.
- Requests: A simple library for making HTTP requests, perfect for web scraping.
Installing and Managing Libraries
To use these libraries, you need to install them first. Here’s how:
- Open your command line or terminal.
- Type
pip install library_name
(replacelibrary_name
with the name of the library). - Press Enter and wait for the installation to finish.
You can also manage your libraries using a requirements file, which lists all the libraries your project needs. This makes it easier to share your project with others.
Using libraries not only speeds up your work but also allows you to focus on solving problems rather than getting stuck on coding details.
Writing Your First Python Script
Choosing an IDE or Text Editor
To start scripting in Python, you need a good IDE (Integrated Development Environment) or a text editor. Here are some popular options:
- PyCharm: Great for beginners and has many features.
- Visual Studio Code: Lightweight and customizable.
- Jupyter Notebook: Perfect for data analysis and visualization.
Basic Syntax and Structure
Python is known for its simple syntax. Here are some key points to remember:
- Indentation: Use spaces or tabs to define blocks of code.
- Comments: Use
#
to add comments in your code. - Variables: Store data using variables, like
name = "John"
.
Running Your Script
Once you have written your script, it’s time to run it. Here’s how:
- Open your IDE or text editor.
- Write your code and save the file with a
.py
extension. - Open a terminal or command prompt.
- Navigate to the folder where your script is saved.
- Type
python your_script.py
and hit enter.
Remember: Always test your script to ensure it works as expected. This is the best way to learn and improve your skills!
By following these steps, you can easily create and run your first project. Enjoy your journey into Python scripting!
Automating File Operations with Python
Reading and Writing Files
Python makes it easy to read and write files. You can open a file, read its contents, and even write new data to it. Here’s how you can do it:
- Open the file using
open()
. - Read the contents with
read()
orreadlines()
. - Write to the file using
write()
.
Here’s a simple example:
with open('example.txt', 'r') as file:
content = file.read()
print(content)
Organizing and Managing Directories
Managing files is not just about reading and writing; it’s also about keeping things organized. You can create, delete, and rename directories using Python. Here are some common tasks:
- Create a new directory with
os.mkdir()
. - Remove a directory with
os.rmdir()
. - List all files in a directory using
os.listdir()
.
Automating File Backups
Automating file backups is a great way to protect your data. You can write a script that copies important files to a backup folder. Here’s a simple way to do it:
- Identify the files you want to back up.
- Use
shutil.copy()
to copy files to the backup location. - Schedule the script to run regularly using Task Scheduler or Cron.
Automating file operations can save you a lot of time and effort. With Python, you can handle files quickly and efficiently.
By using Python for file automation, you can streamline your workflow and reduce the chances of errors. Whether it’s reading, writing, or organizing files, Python has the tools you need to make it easy!
Web Scraping with Python
Introduction to Web Scraping
Web scraping is a method used to extract data from websites. Python is a great choice for this task because it has many libraries that make scraping easier. In this section, we will look at how to use Python for web scraping and why it is so popular.
Using BeautifulSoup for HTML Parsing
BeautifulSoup is one of the most popular libraries for web scraping. It helps you parse HTML and XML documents. Here are some key features of BeautifulSoup:
- Easy to use: It has a simple interface.
- Flexible: It can handle different types of HTML.
- Powerful: It can search and modify the parse tree.
Automating Data Extraction
Once you have the data, you can automate the extraction process. Here’s how you can do it:
- Install BeautifulSoup: Use pip to install the library.
- Write a script: Create a Python script that uses BeautifulSoup to scrape data.
- Run the script: Execute your script to collect the data automatically.
Web scraping can save you a lot of time by automating data collection tasks. It allows you to gather information quickly and efficiently.
Feature | Description |
---|---|
Easy to Learn | Simple syntax and structure |
Community Support | Large community for help and resources |
Versatile | Works with many types of websites |
Automating Data Analysis with Python
Introduction to Data Analysis
Data analysis is the process of inspecting, cleaning, and modeling data to discover useful information. Python makes this process easier and faster. With its powerful libraries, you can handle large datasets and perform complex calculations without much hassle.
Using Pandas for Data Manipulation
Pandas is a popular library in Python that helps you work with data. Here are some key features of Pandas:
- DataFrames: These are like tables that hold your data in rows and columns.
- Data Cleaning: You can easily remove or fill missing values.
- Data Filtering: Quickly find specific data points based on conditions.
Automating Data Visualization
Visualizing data helps you understand it better. You can use libraries like Matplotlib and Seaborn to create graphs and charts. Here’s how to automate this process:
- Load your data into a DataFrame using Pandas.
- Create visualizations using Matplotlib or Seaborn.
- Save your graphs as images or display them directly.
Automating data analysis can save you a lot of time and effort, allowing you to focus on making decisions based on your findings.
In summary, automating data analysis with Python not only speeds up the process but also enhances accuracy. By using libraries like Pandas, you can manipulate data easily and visualize it effectively. This is especially useful for automating data extraction from SQL databases and preparing it for Python analysis.
Scheduling and Running Python Scripts
Using Task Scheduler on Windows
To schedule your Python scripts on Windows, you can use the built-in Task Scheduler. Here’s how to do it:
- Open Task Scheduler from the Start menu.
- Click on "Create Basic Task".
- Follow the prompts to name your task and set the trigger (when you want it to run).
- Choose "Start a Program" and browse to your Python executable and script.
- Finish the setup and your script will run automatically at the scheduled time.
Using Cron Jobs on Linux
On Linux, you can use cron jobs to run your scripts at specific times. Here’s a simple guide:
- Open the terminal.
- Type
crontab -e
to edit your cron jobs. - Add a line in the format
* * * * * /path/to/python /path/to/your_script.py
to schedule your script. - Save and exit the editor.
Time Field | Description |
---|---|
* | Minute (0-59) |
* | Hour (0-23) |
* | Day of Month (1-31) |
* | Month (1-12) |
* | Day of Week (0-7) |
Automating Script Execution
To ensure your scripts run smoothly, consider these tips:
- Test your scripts before scheduling them.
- Log outputs to a file for easy troubleshooting.
- Check permissions to ensure your script can run without issues.
Automating your Python scripts can save you a lot of time and effort. With the right setup, you can let your scripts run on their own!
By following these steps, you can easily set up your Python scripts to run automatically, whether you’re using Windows or Linux. This will help you manage your tasks more efficiently and free up your time for other activities.
Error Handling and Debugging in Python Scripts
Common Errors in Python Scripting
Errors can happen for many reasons when writing Python scripts. Here are some common types:
- Syntax Errors: Mistakes in the code structure, like missing colons or parentheses.
- Runtime Errors: Problems that occur while the program is running, such as dividing by zero.
- Logical Errors: The code runs without crashing, but it doesn’t give the right results.
Using Try-Except Blocks
To manage errors, Python uses try-except blocks. This is how it works:
- Try: Write the code that might cause an error inside the
try
block. - Except: If an error happens, the code in the
except
block runs instead. - Finally: You can also add a
finally
block that runs no matter what, even if there was an error.
Here’s a simple example:
try:
result = 10 / 0 # This will cause a runtime error
except ZeroDivisionError:
print("You can't divide by zero!")
finally:
print("This will always run.")
Debugging Tools and Techniques
Debugging is the process of finding and fixing errors in your code. Here are some helpful tools and techniques:
- Print Statements: Use
print()
to check values at different points in your code. - Python Debugger (pdb): A built-in tool that lets you step through your code line by line.
- Integrated Development Environment (IDE): Many IDEs have built-in debugging tools that make it easier to find errors.
Debugging is an important skill for any programmer. Learning how to find and fix errors will make you a better coder.
Summary
Understanding how to handle errors and debug your scripts is crucial for writing effective Python code. By using try-except blocks and debugging tools, you can create scripts that run smoothly and efficiently. Remember, every programmer makes mistakes; the key is to learn from them!
Best Practices for Writing Efficient Python Scripts
Writing Clean and Readable Code
To make your Python scripts easy to understand, follow these tips:
- Use meaningful names for variables and functions.
- Keep your code organized with proper indentation. In Python, indentation is crucial; it helps define the structure of your code. Following the PEP 8 style guide is a good idea, which suggests using 4 spaces for each level of indentation.
- Add comments to explain complex parts of your code. This helps others (and your future self) understand what you were thinking.
Optimizing Script Performance
To ensure your scripts run quickly and efficiently, consider these strategies:
- Avoid using unnecessary loops. Instead, try to use built-in functions that are faster.
- Use data structures like lists and dictionaries wisely. Choose the right one for your needs.
- Profile your code to find slow parts and improve them.
Documenting Your Scripts
Good documentation is key to maintaining your scripts. Here’s how to do it:
- Write a clear README file that explains what your script does.
- Use docstrings to describe functions and classes. This helps anyone who reads your code understand its purpose.
- Keep your documentation up to date as you make changes to your script.
Remember, writing clean and efficient code not only helps you but also makes it easier for others to collaborate with you.
By following these best practices, you can create Python scripts that are not only effective but also easy to read and maintain. Good coding habits lead to better programming!
Advanced Scripting Techniques in Python
Using Regular Expressions
Regular expressions (regex) are powerful tools for searching and manipulating text. They allow you to find patterns in strings, making tasks like data validation and extraction easier. Here are some common uses:
- Validating email addresses
- Searching for specific words
- Replacing text patterns
Working with APIs
APIs (Application Programming Interfaces) let your Python scripts communicate with other software. This is useful for getting data from websites or services. To work with APIs, follow these steps:
- Understand the API documentation.
- Use the
requests
library to make calls. - Handle the data returned.
Multithreading and Multiprocessing
When you want your scripts to run faster, you can use multithreading or multiprocessing. These techniques allow your program to do many things at once. Here’s a quick comparison:
Feature | Multithreading | Multiprocessing |
---|---|---|
Best for | I/O-bound tasks | CPU-bound tasks |
Memory usage | Lower | Higher |
Complexity | Easier to implement | More complex |
Mastering these advanced techniques can greatly enhance your Python skills. In this advanced python topics tutorial, learn about various advanced python concepts with additional resources and master python programming language.
Real-World Applications of Python Scripting
Python is a versatile language that can be used in many areas. Here are some common applications:
Automating System Administration Tasks
- Managing servers: Python scripts can help automate tasks like starting or stopping services.
- Monitoring system performance: You can write scripts to check CPU usage or memory.
- User management: Automate adding or removing users in a system.
Building Simple Web Applications
- Creating web apps: Use frameworks like Flask or Django to build web applications.
- Handling user input: Python can process forms and store data in databases.
- Displaying data: You can show information dynamically on web pages.
Creating Custom Automation Tools
- Task automation: Write scripts to automate repetitive tasks like sending emails.
- Data processing: Use Python to clean and analyze data from various sources.
- Integration with other tools: Python can connect with APIs to pull or push data.
Python is a powerful language that can be used for many tasks, making it a great choice for automation.
Application Type | Description |
---|---|
System Administration | Automate server management and monitoring |
Web Development | Build and manage web applications |
Custom Tools | Create scripts for specific automation needs |
Python scripting is used in many real-life situations, from automating tasks to analyzing data. It’s a powerful tool that can help you solve problems quickly and efficiently. If you want to learn how to use Python effectively, visit our website to start your coding journey today!
Conclusion
In summary, Python scripting is a powerful tool that can help you automate tasks and work more efficiently. By learning the basics, you can save time and make your life easier. Whether you want to automate simple chores or tackle more complex projects, Python gives you the skills to do it. Remember, practice is key! The more you code, the better you’ll get. So, dive in and start exploring the world of Python. With platforms like AlgoCademy, you can find great resources to help you along the way. Happy coding!
Frequently Asked Questions
What is Python scripting?
Python scripting is using the Python programming language to write small programs that automate tasks. It can help you do things faster and easier.
Why should I learn Python for scripting?
Python is popular because it’s easy to read and write. It has many libraries that help you automate different tasks without much effort.
How do I set up Python on my computer?
You can download Python from the official website. After installing it, you can use a code editor or an IDE to write your scripts.
What are some libraries I should know about?
Some popular libraries for automation include ‘os’ for file operations and ‘requests’ for web tasks. These can make your work much simpler.
How can I write my first script?
Start by picking a simple task you want to automate. Write the code in your editor, save it, and run it to see how it works.
What is web scraping?
Web scraping is collecting data from websites. You can use Python libraries like BeautifulSoup to help you get the information you need.
How do I schedule my scripts to run automatically?
You can use tools like Task Scheduler on Windows or Cron Jobs on Linux to set a time for your scripts to run without you having to start them.
What should I do if my script has errors?
If you see errors, check your code for mistakes. You can use ‘try-except’ blocks to handle errors and make debugging easier.