Mastering C# Programming for Interviews: Essential Tips and Strategies
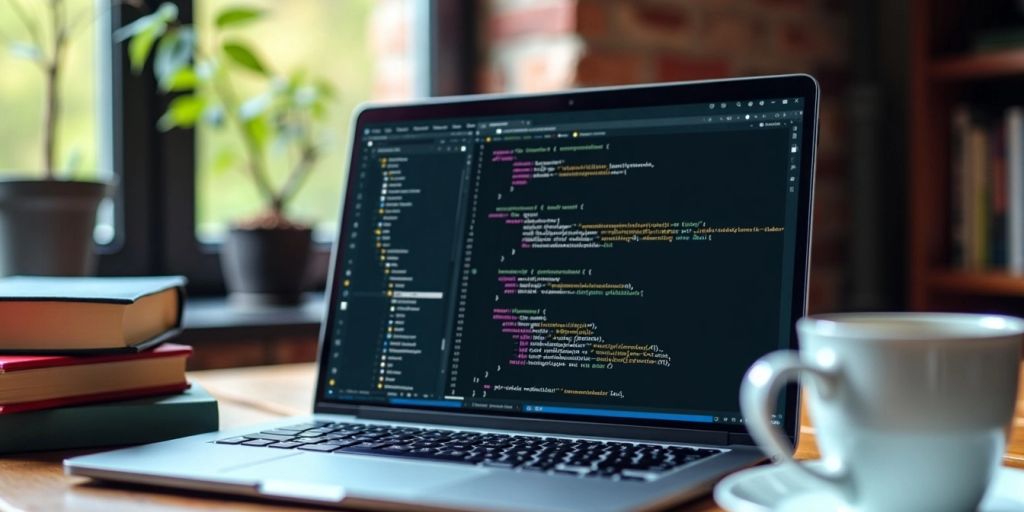
Getting ready for a C# job interview can be tough. This guide will help you understand the most important parts of preparing for interviews, from writing your resume to answering tricky questions. By following these tips and strategies, you’ll feel more confident and ready to impress your future employers.
Key Takeaways
- Craft a strong resume that highlights your C# skills and projects.
- Practice answering common behavioral questions to show your problem-solving abilities.
- Understand the basics of C# programming, including data types and control structures.
- Familiarize yourself with advanced topics like LINQ and asynchronous programming.
- Prepare for technical interviews by solving coding challenges and learning system design concepts.
Crafting a Compelling Resume and Cover Letter for C# Roles
Creating an Impactful Resume
Creating a strong resume is essential for getting noticed by employers. Your resume should highlight your experience, skills, and education. Here are some key points to consider:
- Use clear headings and bullet points.
- Tailor your resume for each job application.
- Include relevant C# projects and technologies.
Section | Description |
---|---|
Objective | A brief statement about your career goals. |
Skills | List of programming languages and tools. |
Experience | Previous jobs and responsibilities. |
Education | Your degrees and certifications. |
Drafting a Persuasive Cover Letter
A cover letter is your chance to explain why you are the best fit for the job. Make sure to:
- Address the hiring manager by name.
- Explain why you want to work for the company.
- Highlight your most relevant experiences.
Showcasing Your C# Projects
When applying for C# roles, showcasing your projects can set you apart. Consider these tips:
- Include links to your GitHub or portfolio.
- Describe your role in each project.
- Mention any challenges you overcame and the results achieved.
Remember, a well-crafted resume and cover letter can make a significant difference in your job search. They are your first chance to impress potential employers!
Mastering Behavioral Interview Questions
Understanding Different Types of Interviews
When preparing for interviews, it’s crucial to know the different types. Here are some common ones:
- Structured Interviews: These follow a set format with specific questions.
- Unstructured Interviews: More casual and open-ended, allowing for a free-flowing conversation.
- Behavioral Interviews: Focus on past experiences to predict future behavior.
Preparing for Common Behavioral Questions
To ace behavioral questions, consider these steps:
- Use the STAR Method: Structure your answers using Situation, Task, Action, and Result.
- Practice Common Questions: Familiarize yourself with questions like "Tell me about a time you faced a challenge."
- Reflect on Your Experiences: Think about your past roles and how they relate to the job you’re applying for.
Techniques for Answering Challenging Questions
Sometimes, you may face tough questions. Here are some techniques to handle them:
- Stay Calm: Take a moment to think before answering.
- Be Honest: If you don’t have a specific experience, it’s okay to say so and discuss how you would handle a similar situation.
- Focus on Learning: Highlight what you learned from past experiences, even if they were difficult.
Remember, mastering behavioral questions can significantly boost your confidence and help you stand out in interviews.
By preparing well, you can effectively showcase your skills and experiences, making a strong impression on your interviewers. This preparation is essential to catapult your C# journey and succeed in your career.
Fundamentals of C# Programming
Essential C# Concepts and Principles
Understanding the basics of C# is crucial for any developer. C# is a versatile language that supports various programming styles, including object-oriented programming (OOP). Here are some key concepts:
- Syntax: The rules that define the combinations of symbols that are considered to be correctly structured programs.
- Data Types: C# has several built-in data types, such as integers, floats, and strings.
- Control Structures: These include loops and conditionals that control the flow of the program.
Working with Data Types and Variables
In C#, data types are categorized into two main types: value types and reference types. Here’s a quick comparison:
Type | Description |
---|---|
Value Types | Store data directly (e.g., int, char) |
Reference Types | Store references to objects (e.g., string, arrays) |
To declare a variable, you simply specify the type followed by the variable name, like this:
int age = 25;
string name = "John";
Writing Control Structures and Loops
Control structures help manage the flow of your program. Here are some common types:
- If-Else Statements: Used for decision-making.
- Switch Statements: A cleaner alternative to multiple if-else statements.
- Loops: Such as
for
,while
, andforeach
, which allow you to repeat actions.
Remember, using loops effectively can help you avoid repetitive code and make your programs more efficient.
In summary, mastering these fundamentals will set a strong foundation for your C# programming journey. As you progress, you will find that these concepts are essential for tackling more advanced topics and challenges in C# development. Our free C# tutorials cover the basic and advanced concepts of C#, including fundamentals of C#, including syntax, data types, control structures, classes, and more.
Advanced C# Programming Techniques
Exploring LINQ and Collections
Language Integrated Query (LINQ) is a powerful feature in C# that allows you to query collections in a more readable way. Using LINQ can simplify your code significantly. Here are some key points to remember:
- LINQ can be used with various data sources, including arrays, lists, and databases.
- It provides a consistent way to query data regardless of the source.
- You can use LINQ methods like
Select
,Where
, andOrderBy
to manipulate data easily.
Asynchronous Programming with Async and Await
Asynchronous programming is essential for creating responsive applications. The async
and await
keywords in C# help manage tasks without blocking the main thread. Here’s how to use them effectively:
- Mark your method with the
async
keyword. - Use
await
before calling a task that takes time to complete. - Ensure that your method returns a
Task
orTask<T>
.
This approach allows your application to remain responsive while performing long-running operations.
Implementing Design Patterns in C#
Design patterns are proven solutions to common problems in software design. Here are a few popular patterns you might consider:
- Singleton: Ensures a class has only one instance and provides a global point of access to it.
- Factory: Creates objects without specifying the exact class of object that will be created.
- Observer: Defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified.
Understanding and applying design patterns can greatly enhance your coding skills and make your code more maintainable.
By mastering these advanced techniques, you can improve your C# programming skills and stand out in C# interview questions with example answers and tips. This knowledge will not only help you in interviews but also in real-world applications.
Effective Use of C# Development Tools
Introduction to Visual Studio and Alternatives
Visual Studio is a powerful IDE that many C# developers prefer. It offers a range of features that help streamline the coding process. However, there are alternatives like Visual Studio Code that are also popular. Here are some key points to consider:
- Visual Studio: Comprehensive features, great for large projects.
- Visual Studio Code: Lightweight, ideal for smaller tasks.
- JetBrains Rider: Excellent for cross-platform development.
Utilizing .NET and .NET Core Frameworks
.NET and .NET Core are essential for C# development. They provide libraries and tools that make coding easier. Here’s a quick comparison:
Feature | .NET Framework | .NET Core |
---|---|---|
Platform Support | Windows only | Cross-platform |
Performance | Good | Better |
Deployment | Complex | Simple |
Leveraging NuGet for Package Management
NuGet is a package manager that helps you manage libraries in your C# projects. Here’s how to use it effectively:
- Install Packages: Use the NuGet Package Manager to add libraries.
- Update Packages: Keep your libraries up to date for security and performance.
- Create Packages: Share your own libraries with the community.
Using the right tools can significantly enhance your productivity as a C# developer. Mastering these tools is key to success!
Writing Clean and Maintainable C# Code
Writing clean and maintainable code is crucial for any C# developer. Good code is easy to read and understand, which helps in long-term project success. Here are some key principles to follow:
Implementing SOLID Principles
- Single Responsibility Principle (SRP): Each class should have one reason to change. This makes your code easier to maintain.
- Open/Closed Principle (OCP): Code should be open for extension but closed for modification. This helps avoid breaking existing functionality.
- Liskov Substitution Principle (LSP): Subtypes must be substitutable for their base types without altering the correctness of the program.
Using Common Design Patterns
- Factory Pattern: Helps in creating objects without specifying the exact class of object that will be created.
- Observer Pattern: Useful for creating a subscription mechanism to allow multiple objects to listen and react to events.
- Strategy Pattern: Enables selecting an algorithm’s behavior at runtime.
Best Practices for Code Readability
- Use meaningful names for variables and methods to convey their purpose clearly.
- Keep methods short and focused on a single task to enhance readability.
- Comment wisely: Use comments to explain why something is done, not what is done.
Writing clean code is not just about following rules; it’s about creating a codebase that is easy to work with and understand.
By following these guidelines, you can ensure that your C# code remains clean and maintainable, making it easier for you and others to work on it in the future. Remember, achieving clean code is a continuous process that involves regular review and improvement.
Preparing for Technical C# Interviews
Reviewing Common C# Interview Questions
To get ready for your C# interviews, it’s important to review common questions that you might face. Here are some key areas to focus on:
- Basic C# concepts: Understand the fundamentals of the language.
- Object-oriented programming: Be ready to explain OOP principles.
- Data structures: Know how to use arrays, lists, and dictionaries.
Solving Coding Challenges
Practicing coding challenges is essential. Here are some tips:
- Pick a good programming language: C# is a great choice for coding interviews.
- Plan your time: Tackle questions in order of importance.
- Practice regularly: Use platforms like LeetCode or HackerRank to sharpen your skills.
Understanding System Design Concepts
System design questions can be tricky. Here’s how to prepare:
- Learn the basics: Understand how to design scalable systems.
- Study common architectures: Familiarize yourself with microservices and monolithic designs.
- Practice explaining your designs: Be ready to discuss your thought process clearly.
Preparing for technical interviews requires dedication and practice. Focus on your C# skills and stay confident!
Building a Strong C# Portfolio
Selecting Projects to Showcase
When building your C# portfolio, it’s important to choose projects that highlight your skills and creativity. Here are some tips for selecting the right projects:
- Focus on variety: Include different types of projects, such as web applications, desktop apps, and games.
- Showcase complexity: Choose projects that demonstrate your ability to solve complex problems.
- Highlight collaboration: If you worked on team projects, mention your role and contributions.
Documenting Your Code
Good documentation is key to a strong portfolio. Here’s how to effectively document your projects:
- Use clear comments: Explain your code with comments that are easy to understand.
- Create a README file: Include a summary of the project, how to run it, and any dependencies.
- Organize your code: Keep your code clean and structured for easy navigation.
Creating an Online Portfolio
An online portfolio is essential for showcasing your work. Here are steps to create one:
- Choose a platform: Use GitHub, personal websites, or portfolio builders.
- Include a professional bio: Write a short introduction about yourself and your skills.
- Link to your projects: Make sure to provide links to your showcased projects.
A strong portfolio not only displays your work but also reflects your growth as a developer. It’s a powerful tool to impress potential employers.
In summary, building a strong C# portfolio involves selecting diverse projects, documenting your code well, and creating an accessible online presence. This will help you stand out in the competitive job market.
Negotiating Salary and Evaluating Job Offers
Researching Market Salaries
Before you start negotiating, it’s important to know the average salary for C# developers in your area. This helps you set realistic expectations. Here are some steps to follow:
- Check online resources like salary websites.
- Ask peers in the industry about their experiences.
- Look at job postings to see salary ranges.
Understanding Job Offer Components
When you receive a job offer, it’s not just about the salary. Consider these components:
- Base salary: The main amount you will earn.
- Benefits: Health insurance, retirement plans, etc.
- Bonuses: Performance bonuses or signing bonuses.
- Work-life balance: Flexible hours or remote work options.
Strategies for Effective Negotiation
Negotiating your salary can be tricky, but here are some tips:
- Don’t name a figure first: Let the employer make the first offer.
- Be ready to compromise: Know what you can give up.
- Highlight your skills: Show how your experience adds value to the company.
Remember, negotiating is a normal part of the hiring process. Being prepared can make a big difference in the outcome.
Summary
Negotiating your salary and evaluating job offers is crucial for your career. By doing your research and understanding the components of an offer, you can make informed decisions that benefit your future. Always approach negotiations with confidence and clarity, and don’t hesitate to ask for what you deserve!
Post-Interview Follow-Up Strategies
Sending Thank You Notes
After your interview, it’s important to send a thank you note. This shows your appreciation for the interviewers’ time. In your message, mention something interesting you discussed during the interview. Keep it short and sweet; you don’t need to write a long explanation about why you should be hired.
Addressing Interview Shortcomings
Take a moment to think about how the interview went. Did you struggle with any questions? Write down what you could improve for next time. This reflection helps you learn and grow for future interviews.
Maintaining Professional Contact
Staying in touch with your interviewers can be beneficial. Here are some ways to keep the connection alive:
- Follow up on any updates regarding your application.
- Share relevant articles or resources that might interest them.
- Connect on professional networks like LinkedIn.
Following up after an interview is not just about getting the job; it’s about building relationships and showing your professionalism.
Summary
Following up after an interview is a crucial step. It helps you express gratitude, reflect on your performance, and maintain connections. Remember, every interview is a chance to learn and improve your skills for the future!
Developing Soft Skills for C# Developers
Effective Communication Techniques
Effective communication is key in any job, especially in C# development. Here are some tips to improve your communication skills:
- Listen actively to understand others better.
- Use clear and concise language when explaining technical concepts.
- Practice non-verbal communication to convey confidence.
Building Professional Relationships
Building strong relationships can help you in your career. Consider these strategies:
- Network with other developers and professionals.
- Seek mentorship from experienced colleagues.
- Participate in team activities to strengthen bonds.
Time Management and Productivity
Managing your time well is crucial for success. Here are some techniques:
- Set specific goals for each day.
- Use tools like calendars and to-do lists to stay organized.
- Take regular breaks to maintain focus and energy.
Developing soft skills is just as important as technical skills. They can greatly impact your career growth and job satisfaction.
In summary, mastering soft skills like communication, relationship-building, and time management can enhance your effectiveness as a C# developer. These skills are essential for navigating the complexities of the tech industry and succeeding in interviews.
Continuous Learning and Professional Development
Staying Updated with C# Trends
To be a successful C# developer, keeping up with the latest trends is crucial. Here are some effective ways to stay informed:
- Follow tech blogs and newsletters.
- Join developer communities online.
- Attend conferences and workshops.
Participating in Coding Communities
Engaging with others can enhance your skills. Consider:
- Joining forums like Stack Overflow.
- Contributing to open-source projects.
- Participating in hackathons.
Pursuing Advanced Certifications
Certifications can boost your career. Here are some popular options:
Certification Name | Focus Area |
---|---|
Microsoft Certified: C# Developer | C# fundamentals and advanced topics |
.NET Foundation Certification | .NET technologies and frameworks |
Azure Developer Certification | Cloud development with C# |
Continuous learning is essential for growth. Embrace new challenges and keep evolving your skills.
By following these strategies, you can ensure that your knowledge remains relevant and that you are well-prepared for future opportunities in the C# programming field.
Continuous learning is key to growing in your career. By constantly improving your skills, you can stay ahead in the fast-paced tech world. Don’t wait to take the next step in your journey. Visit our website to start coding for free and unlock your potential today!
Final Thoughts on C# Interview Success
In conclusion, mastering C# programming is a vital step for anyone aiming to succeed in technical interviews. By focusing on the key concepts, practicing coding challenges, and preparing for behavioral questions, you can boost your confidence and skills. Remember, it’s not just about knowing the code; it’s also about how you present yourself and communicate your ideas. Keep learning and practicing, and don’t hesitate to seek help from resources like AlgoCademy. With dedication and the right strategies, you’ll be well on your way to landing that dream job in C# development.
Frequently Asked Questions
What should I include in my C# resume?
Make sure to add your skills, work experience, and any projects you’ve done in C#. Highlight what makes you stand out.
How can I prepare for C# technical interviews?
Practice common C# questions, work on coding challenges, and understand the basics of system design.
What are some key C# concepts I should know?
Focus on object-oriented programming, data types, and control structures. These are essential for any C# role.
How important is it to showcase my C# projects?
Very important! Showcasing your projects can demonstrate your skills and creativity to potential employers.
What are behavioral interview questions?
These questions assess how you’ve handled situations in the past. They often start with ‘Tell me about a time when…’
How can I improve my coding skills in C#?
Practice regularly, join coding communities, and consider taking online courses or tutorials.
What tools should I use for C# development?
Visual Studio is a popular choice, but you can also explore alternatives like JetBrains Rider or Visual Studio Code.
How do I follow up after an interview?
Send a thank-you note, express your appreciation, and reiterate your interest in the position.