Mastering Recursion Coding Challenges: A Comprehensive Guide to Solving Complex Problems
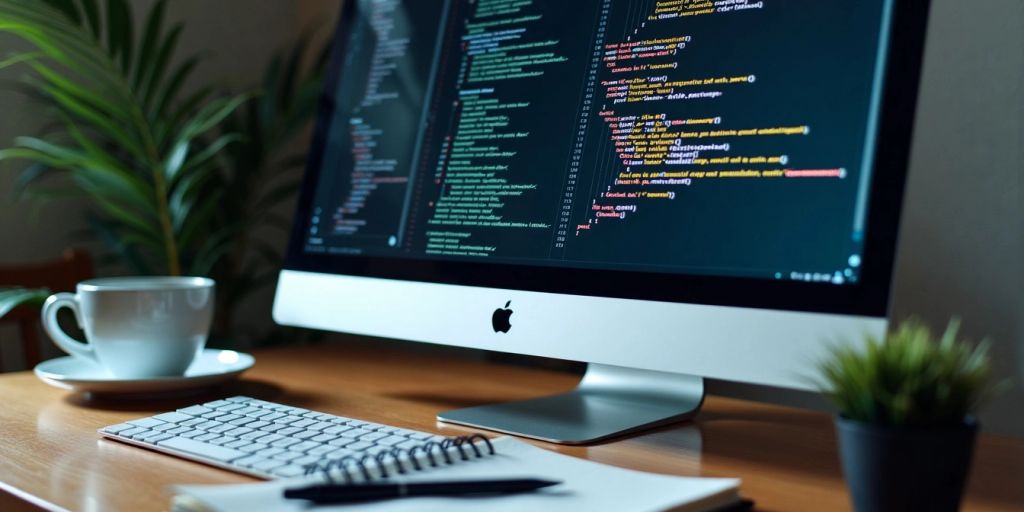
Recursion is a key concept in programming that allows functions to call themselves to solve problems. This guide will help you understand the basics of recursion, common patterns, and how to apply recursion in various situations. Whether you’re tackling math problems or working with data structures, mastering recursion can make your coding more efficient and elegant.
Key Takeaways
- Recursion involves a function calling itself to break down complex problems into simpler parts.
- It’s important to define a base case to prevent infinite loops in recursive functions.
- Common recursion patterns include tail recursion, memoization, and divide and conquer techniques.
- Recursion can be applied in various areas, such as mathematical calculations and traversing data structures like trees and graphs.
- Practicing recursion through coding challenges can help solidify your understanding and skills.
Understanding the Fundamentals of Recursion
Definition and Basics
Recursion is a programming technique where a function calls itself to solve a problem. This method allows us to break down complex issues into smaller, more manageable parts. For example, a recursive function can solve a problem by calling a copy of itself and tackling smaller subproblems of the original issue.
Importance of Base and Recursive Cases
In recursion, two key components are essential: the base case and the recursive case.
- Base Case: This is the condition that stops the recursion. Without it, the function would keep calling itself endlessly, leading to errors.
- Recursive Case: This is where the function continues to call itself, gradually moving towards the base case.
To illustrate, consider the factorial of a number, where the factorial of n (denoted as n!) is the product of all positive integers less than or equal to n. The recursive approach involves multiplying n by the factorial of n-1 until reaching the base case of 1.
Real-life Analogies
Understanding recursion can be easier with real-life examples:
- Russian Dolls: Each doll contains a smaller doll inside, similar to how a recursive function contains smaller calls.
- Family Trees: Each person can be traced back to their parents, just like how a recursive function can trace back to its base case.
- Matryoshka Dolls: These dolls fit inside one another, representing how problems can fit within smaller problems.
Recursion is a powerful tool in programming that allows us to solve complex problems with elegant solutions. By mastering its principles, you can tackle a wide range of challenges effectively.
Common Recursion Patterns and Techniques
Tail Recursion
Tail recursion is a special case where the recursive call is the last operation in the function. This can help optimize performance by allowing some programming languages to reuse stack frames, reducing memory usage. Tail recursion can be a game changer when dealing with large datasets.
Memoization
Memoization is a technique used to store the results of expensive function calls and return the cached result when the same inputs occur again. This can significantly speed up recursive functions by avoiding repeated calculations. Here’s a simple example:
memo = {}
def fibonacci(n):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci(n-1) + fibonacci(n-2)
return memo[n]
Divide and Conquer
Divide and conquer is a powerful strategy that breaks a problem into smaller subproblems, solves each subproblem independently, and combines their results. This approach is often used in algorithms like mergesort and quicksort. Here’s a quick overview of the steps:
- Divide the problem into smaller parts.
- Conquer each part recursively.
- Combine the results to get the final answer.
Recursion is not just a coding technique; it’s a way to think about problems. By breaking them down into smaller pieces, you can tackle complex challenges more easily.
Summary Table
Technique | Description | Benefits |
---|---|---|
Tail Recursion | Last operation is a recursive call | Reduces memory usage |
Memoization | Caches results of function calls | Speeds up calculations |
Divide and Conquer | Breaks problems into smaller, manageable parts | Efficient problem-solving |
Mathematical Problems Solved by Recursion
Recursion is a powerful tool for solving various mathematical problems. Here are some common examples:
Factorials
Calculating the factorial of a number is a classic example of recursion. The factorial of a number n (denoted as n!) is the product of all positive integers up to n. The recursive formula is:
- Base Case: If n is 1, return 1.
- Recursive Case: Return n multiplied by the factorial of (n-1).
For example:
def factorial(n):
if n == 1:
return 1
return n * factorial(n - 1)
Fibonacci Sequence
The Fibonacci sequence is another great example. Each number in the sequence is the sum of the two preceding ones. The recursive approach is:
- Base Cases: If n is 0, return 0; if n is 1, return 1.
- Recursive Case: Return the sum of the Fibonacci of (n-1) and (n-2).
Example code:
def fibonacci(n):
if n == 0:
return 0
if n == 1:
return 1
return fibonacci(n - 1) + fibonacci(n - 2)
Greatest Common Divisor (GCD)
Finding the GCD of two numbers can also be done recursively using the Euclidean algorithm:
- Base Case: If b is 0, return a.
- Recursive Case: Return the GCD of b and the remainder of a divided by b.
Example:
def gcd(a, b):
if b == 0:
return a
return gcd(b, a % b)
Recursion allows us to break down complex problems into simpler ones, making it easier to find solutions. Using recursion can simplify your coding process and help you tackle challenging problems effectively.
In summary, recursion is a valuable technique for solving mathematical problems like factorials, Fibonacci sequences, and GCD calculations. By understanding these examples, you can apply recursion to various challenges in coding and computer science.
Summary Table
Problem | Recursive Formula |
---|---|
Factorial | n! = n * (n-1)! |
Fibonacci Sequence | F(n) = F(n-1) + F(n-2) |
Greatest Common Divisor | GCD(a, b) = GCD(b, a % b) |
Recursion in Data Structures
Tree Traversal
Recursion is a powerful tool for navigating trees. When you want to visit every node in a tree, recursion makes it easy. Here’s how it works:
- Start at the root node.
- Visit the left child recursively.
- Visit the right child recursively.
For example, in an in-order traversal, you would visit nodes in this order: left child, current node, right child. This method is simple and effective for tree structures.
Graph Traversal
When dealing with graphs, recursion shines through in Depth-First Search (DFS). This technique explores as far as possible along each branch before backtracking. Here’s a basic outline:
- Start from a node and mark it as visited.
- Recursively visit each unvisited neighbor.
- Backtrack when no unvisited neighbors are left.
This approach is efficient for exploring all connections in a graph.
Linked Lists
Recursion can also be used to traverse linked lists. The process is straightforward:
- Start at the head of the list.
- Process the current node.
- Call the function recursively for the next node.
This method allows you to handle each element without needing loops, making your code cleaner and easier to read.
Recursion in data structures is not just a technique; it’s a way to simplify complex problems by breaking them down into smaller, manageable parts. Recursion in the data structure can be defined as a method through which problems are broken down into smaller sub-problems to find a solution. Read on!
Optimizing Recursive Solutions
Avoiding Stack Overflow
To prevent stack overflow errors in recursion, follow these steps:
- Define a clear base case that will eventually be reached.
- Limit the depth of recursion by breaking down the problem into smaller parts.
- Consider using tail recursion where possible, as it can optimize memory usage.
Improving Time Complexity
To enhance the efficiency of your recursive solutions:
- Use memoization to store results of expensive function calls and reuse them when the same inputs occur again.
- Analyze the problem to see if it can be solved using iterative methods instead, which can be more efficient in some cases.
- Break down the problem into smaller subproblems that can be solved independently, reducing the overall complexity.
Using Iterative Alternatives
Sometimes, recursion can be replaced with iteration. Here are some benefits of using iterative solutions:
- Lower memory usage since they do not require maintaining a call stack.
- Faster execution for certain problems, especially those with deep recursion.
- Easier debugging since the flow of control is more straightforward.
Remember: While recursion is powerful, it’s essential to know when to use it and when to switch to iterative solutions for better performance.
In summary, optimizing recursive solutions involves understanding the problem, defining clear base cases, and considering alternatives like memoization and iteration. By mastering these techniques, you can tackle complex problems more effectively and efficiently. Mastering recursion is key to solving many coding challenges successfully.
Debugging Recursive Functions
Common Errors
Debugging recursive functions can be tricky. Here are some common mistakes to watch out for:
- Missing Base Case: Without a base case, the function may run forever.
- Incorrect Base Case: If the base case is wrong, the function might not stop when it should.
- Infinite Recursion: This happens when the function keeps calling itself without reaching the base case.
Testing Strategies
To ensure your recursive function works correctly, consider these testing methods:
- Use Print Statements: One of the simplest ways to debug a recursive function is to insert
print()
statements within the function. This allows you to track the flow of execution and see how the values change. - Test with Simple Cases: Start with small inputs to see if the function behaves as expected.
- Check Edge Cases: Make sure to test the function with edge cases, like zero or negative numbers, to ensure it handles all scenarios.
Visualization Tools
Visualizing recursion can help you understand how the function operates. Here are some tools you can use:
- Call Stack Visualizers: These tools show how functions are called and returned, helping you see the flow of execution.
- Debuggers: Use a debugger to step through your code line by line, observing how variables change.
- Flowcharts: Create flowcharts to map out the logic of your recursive function, making it easier to spot errors.
Debugging recursion requires patience and practice. Understanding how your function behaves at each step is key to finding and fixing issues.
Advanced Recursion Challenges
Dynamic Programming
Dynamic programming is a powerful technique that uses recursion to solve problems by breaking them down into simpler subproblems. It stores the results of these subproblems to avoid redundant calculations. Here are some key points:
- Overlapping Subproblems: Identify when the same subproblem is solved multiple times.
- Optimal Substructure: Ensure that the optimal solution can be constructed from optimal solutions of its subproblems.
- Memoization: Store results of expensive function calls and reuse them when the same inputs occur again.
Backtracking
Backtracking is a method for solving problems incrementally, trying partial solutions and then abandoning them if they are not valid. This technique is often used in:
- Puzzle Solving: Like Sudoku or N-Queens.
- Pathfinding: Finding paths in mazes or graphs.
- Combinatorial Problems: Generating combinations or permutations of a set.
Game Theory
In game theory, recursion helps analyze strategies in competitive situations. It can be used to:
- Minimax Algorithm: Determine the best move for a player assuming the opponent also plays optimally.
- Alpha-Beta Pruning: Optimize the minimax algorithm by eliminating branches that won’t be selected.
- Recursive State Evaluation: Evaluate game states recursively to decide the best course of action.
Recursion is a vital tool in programming, especially for complex problems. It allows you to break down challenges into manageable parts, making solutions easier to find.
By mastering these advanced recursion challenges, you can tackle a wide range of problems effectively and efficiently. Remember, practice is key to becoming proficient in these techniques!
Practical Applications of Recursion
Recursion is a powerful tool in programming that can simplify complex problems. Here are some practical applications where recursion shines:
Sorting Algorithms
- Quick Sort: This algorithm divides the array into smaller parts and sorts them recursively.
- Merge Sort: It splits the array into halves, sorts each half, and then merges them back together.
- Heap Sort: Recursion is used to maintain the heap property while sorting.
Search Algorithms
- Binary Search: This method divides the search interval in half, making it efficient for sorted arrays.
- Depth-First Search (DFS): Used in tree and graph traversal, it explores as far as possible along each branch before backtracking.
- Breadth-First Search (BFS): While typically iterative, recursion can be used to explore nodes level by level.
Parsing Expressions
- Expression Trees: Recursion helps in evaluating and simplifying expressions represented as trees.
- Syntax Trees: Used in compilers, recursion can parse and analyze the structure of programming languages.
- Regular Expressions: Recursion can be applied to match complex patterns in strings.
Recursion is especially useful for navigating tree structures such as hierarchical data stored in a database. It allows for elegant solutions to complex problems by breaking them down into smaller, manageable parts.
In summary, recursion is not just a coding technique; it’s a way to think about problems. By mastering its applications, you can tackle a wide range of challenges effectively.
Learning and Practicing Recursion
Interactive Tutorials
- Engage with online platforms that offer interactive coding environments.
- Follow step-by-step guides to understand recursion better.
- Utilize visual aids to see how recursion works in real-time.
Coding Challenges
- Participate in coding competitions that focus on recursion.
- Solve practice questions for recursion to enhance your skills. For example, consider the following recursive C function: let len be the length of the string s and num be the number of characters printed on the screen.
- Join coding communities to share and discuss solutions.
Analyzing Example Problems
- Study existing recursive solutions to learn different approaches.
- Break down complex problems into simpler recursive steps.
- Compare recursive and iterative solutions to understand their differences.
Mastering recursion takes time and practice. The more you practice, the better you will become.
Recursion in Different Programming Languages
Python
Recursion in Python is a method where a function calls itself to solve smaller parts of a problem. This technique is useful for tasks like calculating factorials or navigating tree structures. Here are some key points:
- Base Case: This is essential to stop the recursion. Without it, the function would keep calling itself indefinitely.
- Stack Overflow: If the recursion goes too deep, it can lead to a crash due to too many function calls.
- Example: A simple recursive function to calculate factorial:
def factorial(n): if n == 0: return 1 else: return n * factorial(n - 1)
JavaScript
In JavaScript, recursion works similarly. Functions can call themselves to break down problems. Here are some important aspects:
- Closure: JavaScript allows functions to maintain their own scope, which can be useful in recursive functions.
- Tail Call Optimization: Some JavaScript engines optimize tail calls, making recursion more efficient.
- Example: A recursive function to find the Fibonacci sequence:
function fibonacci(n) { if (n <= 1) return n; return fibonacci(n - 1) + fibonacci(n - 2); }
C++
In C++, recursion is also a common technique. It allows functions to call themselves, similar to Python and JavaScript. Here are some points to consider:
- Memory Management: C++ requires careful management of memory, especially with deep recursion.
- Performance: Recursive functions can be less efficient than iterative solutions in C++ due to function call overhead.
- Example: A recursive function to calculate the greatest common divisor (GCD):
int gcd(int a, int b) { if (b == 0) return a; return gcd(b, a % b); }
Language | Key Feature | Example Use Case |
---|---|---|
Python | Easy syntax | Factorial calculation |
JavaScript | Closure and optimization | Fibonacci sequence |
C++ | Memory management | GCD calculation |
Recursion is a powerful tool in programming, but it requires careful handling to avoid issues like stack overflow. Understanding the language’s features can help you use recursion effectively.
Comparing Recursion and Iteration
Memory Usage
- Recursion uses more memory because each function call adds a new layer to the stack. This can lead to stack overflow if the recursion is too deep.
- Iteration is generally more memory-efficient since it uses a single stack frame for the loop.
Performance Considerations
- Speed: Iteration is often faster because it avoids the overhead of multiple function calls.
- Complexity: Recursion can be simpler and more elegant for problems that naturally fit its structure, like tree traversals.
- Overhead: Recursion has higher overhead due to function calls, while iteration has lower overhead.
Readability and Maintainability
- Recursion can make code easier to read and understand for problems that are recursive in nature.
- Iteration may lead to more complex code for the same problems, making it harder to follow.
Aspect | Recursion | Iteration |
---|---|---|
Memory Overhead | High (due to stack frames) | Low |
Processing Overhead | High (function calls) | Low |
Time Complexity | Can be exponential | Generally lesser |
Risk of Infinite Loop | CPU crash (stack overflow) | Memory exhaustion |
In summary, understanding the difference between recursion and iteration is crucial for choosing the right approach for your coding challenges. Each method has its strengths and weaknesses, and the best choice often depends on the specific problem you are trying to solve.
When we look at recursion and iteration, we see two different ways to solve problems in coding. Recursion is like a puzzle where a problem is solved by breaking it down into smaller pieces, while iteration is more straightforward, repeating steps until a goal is reached. Both methods have their strengths and weaknesses, and knowing when to use each can make you a better coder. Want to dive deeper into coding? Visit our website to start coding for free!
Final Thoughts on Recursion
In summary, mastering recursion is essential for anyone serious about coding. This technique allows you to break down tough problems into smaller, easier parts, making solutions clearer and more elegant. Whether you’re working with trees, sorting lists, or exploring complex data, recursion is a powerful tool in your programming kit. So, embrace recursion, practice regularly, and watch your problem-solving skills soar. With dedication, you can tackle any coding challenge that comes your way!
Frequently Asked Questions
What is recursion?
Recursion is when a function calls itself to solve a problem. It breaks the problem into smaller parts until it reaches a simple case that can be solved easily.
When should I use recursion?
Use recursion for problems that have repeating structures, like trees or math problems. It’s great when you can split the problem into similar smaller problems.
What is a base case in recursion?
A base case is a specific condition that tells the recursion when to stop. It prevents the function from running forever.
Is recursion better than iteration?
Not always. Recursion can be simpler and cleaner for some problems, but it can also use more memory and be slower than iteration.
How can I avoid errors in recursion?
Make sure to define a clear base case and test your function with different inputs to catch mistakes.
What are some common problems solved with recursion?
Common problems include calculating factorials, generating Fibonacci numbers, and traversing trees.
Can recursion lead to performance issues?
Yes, if not managed well, recursion can use a lot of memory and may cause stack overflow errors with deep recursion.
How can I practice recursion?
You can practice recursion by working on coding challenges on websites like LeetCode or HackerRank.