Mastering Binary Search Tree Tutorial: A Step-by-Step Guide for Beginners
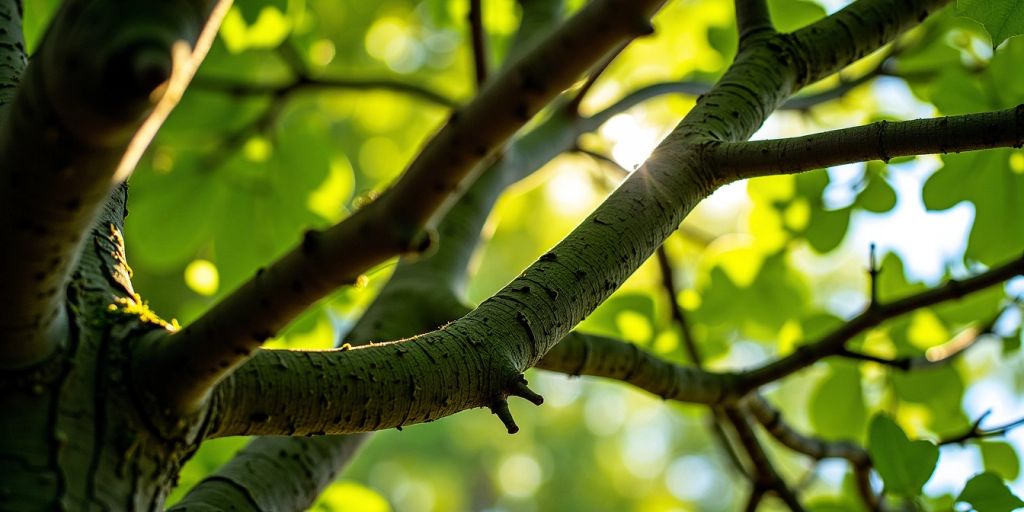
Binary Search Trees (BSTs) are a key concept in computer science, providing an efficient way to organize and manage data. This tutorial will guide you through the fundamentals of BSTs, from understanding their structure to implementing various operations. Whether you’re a beginner or looking to refresh your skills, this step-by-step guide will help you master the essentials of Binary Search Trees.
Key Takeaways
- A Binary Search Tree stores data in a way that allows for quick searching, adding, and removing of items.
- Each node has at most two children, with the left child always being smaller and the right child larger than the parent.
- Maintaining the BST property is crucial for performance; violating it can lead to inefficient operations.
- Common operations include inserting, searching, and deleting nodes, each with specific algorithms.
- Understanding BSTs can improve your coding skills and help in solving complex problems effectively.
Understanding the Basics of Binary Search Trees
Definition and Key Characteristics
A Binary Search Tree (BST) is a special kind of binary tree that organizes data in a way that makes searching, inserting, and deleting items very efficient. Here are some key features of a BST:
- Node Structure: Each node has a value, a left child, and a right child.
- Binary Tree Properties:
- Each node can have at most two children.
- The left child’s value is always less than its parent.
- The right child’s value is always greater than or equal to its parent.
- Recursive Definition: Both the left and right subtrees of a node are also BSTs.
Importance in Computer Science
Understanding BSTs is crucial because they are widely used in various applications. They help in:
- Fast data retrieval
- Efficient data organization
- Supporting complex data structures like AVL and Red-Black Trees
Real-World Applications
Binary Search Trees are used in many areas, including:
- Databases: For quick data searches.
- File Systems: To organize files efficiently.
- Computer Networks: For routing data packets.
Binary Search Trees are essential for managing ordered data effectively. They provide a systematic way to store and retrieve information quickly.
In summary, the tutorial covers all the aspects from definition to advantages and disadvantages of binary search trees in data structures. The binary search trees have multiple applications with multiple advantages. Therefore, refer to the tutorial until you get thorough with every detail of the binary search tree.
Setting Up Your Development Environment
Choosing the Right Tools
To start your journey with Binary Search Trees, you need to select the right tools. Here are some popular options:
- Text Editors: Visual Studio Code, Sublime Text, or Atom.
- IDEs: IntelliJ IDEA, Eclipse, or PyCharm.
- Version Control: Git for tracking changes in your code.
Installing Necessary Software
Once you’ve chosen your tools, it’s time to install the necessary software. Follow these steps:
- Download the installer for your chosen text editor or IDE.
- Follow the installation prompts to set it up on your computer.
- Install Git to manage your code versions.
Creating Your First Project
Now that your environment is ready, let’s create your first project:
- Open your text editor or IDE.
- Create a new folder for your project.
- Start a new file and save it as
main.py
(or any language you prefer).
Remember: Setting up your environment correctly is crucial to kickstart your programming career. It lays the foundation for mastering techniques for solving binary tree problems.
Constructing a Binary Search Tree
Node Structure and Properties
To build a Binary Search Tree (BST), we first need to understand the node structure. Each node in a BST contains:
- A unique value (key).
- A pointer to the left child node.
- A pointer to the right child node.
This structure ensures that the left child is always less than its parent, while the right child is greater or equal.
Inserting Nodes
Inserting a node into a BST is a crucial operation. Here’s how you can do it:
- Start at the root node.
- If the tree is empty, the new node becomes the root.
- If the tree is not empty, compare the new node’s value with the current node’s value:
- If it’s smaller, move to the left child.
- If it’s larger, move to the right child.
- Repeat until you find an empty spot to insert the new node.
Example of Insertion:
- If you insert the value
10
, it will be placed as the root if the tree is empty. - If you then insert
5
, it will go to the left of10
. - Inserting
15
will place it to the right of10
.
Visualizing the Tree
Visualizing a BST helps in understanding its structure. Here’s a simple representation:
10
/ \
5 15
This shows that 5
is less than 10
, and 15
is greater.
Understanding how to construct a BST is essential for performing operations like searching in binary search tree (BST) effectively.
By following these steps, you can successfully create a Binary Search Tree and prepare for further operations like searching and deleting nodes.
Basic Operations on Binary Search Trees
Binary Search Trees (BSTs) are powerful structures that allow for efficient data management. The main operations you can perform on a BST include searching, inserting, and deleting nodes. Understanding these operations is essential for working effectively with BSTs.
Searching for Nodes
To find a specific value in a BST, follow these steps:
- Start at the root node.
- Compare the target value with the current node’s value.
- If they match, you’ve found the value!
- If the target value is less, move to the left child.
- If it’s greater, move to the right child.
- Repeat until you find the value or reach a null node.
The search operation is efficient, with an average time complexity of O(log n).
Deleting Nodes
Deleting a node from a BST can be tricky. Here are the three main cases:
- Leaf Node: Simply remove it.
- One Child: Replace the node with its child.
- Two Children: Find the in-order successor (smallest value in the right subtree) to replace the node.
Traversing the Tree
Traversal allows you to visit all nodes in a specific order. The common methods include:
- In-order: Left, Root, Right (sorted order)
- Pre-order: Root, Left, Right
- Post-order: Left, Right, Root
Traversing a BST helps in understanding its structure and retrieving data in a sorted manner.
In summary, mastering these basic operations will help you effectively manage and utilize Binary Search Trees in various applications. Remember, the key to a successful BST is maintaining its properties during these operations!
Advanced Operations and Techniques
Balancing the Tree
Balancing a binary search tree (BST) is crucial for maintaining its efficiency. A balanced tree ensures that the height is minimized, which leads to faster operations. Here are some common methods to balance a BST:
- AVL Trees: Automatically balance themselves after insertions and deletions.
- Red-Black Trees: Maintain balance through color properties and rotations.
- Splay Trees: Move frequently accessed elements closer to the root.
Handling Duplicates
When working with BSTs, handling duplicates is important to maintain the integrity of the tree. Here are some strategies:
- Ignore Duplicates: Simply do not insert duplicate values.
- Count Duplicates: Store a count of how many times a value has been inserted.
- Store in a List: Keep duplicates in a linked list attached to the node.
Optimizing Performance
To ensure your BST operates efficiently, consider the following techniques:
- Use Iterative Methods: For searching and inserting, iterative methods can be faster than recursive ones.
- Limit Tree Height: Regularly balance the tree to keep its height low.
- Cache Frequently Accessed Nodes: Store pointers to frequently accessed nodes to speed up searches.
Remember: A well-maintained BST can significantly improve the performance of your applications.
Operation | Average Time Complexity | Worst Time Complexity |
---|---|---|
Search | O(log n) | O(n) |
Insert | O(log n) | O(n) |
Delete | O(log n) | O(n) |
Common Mistakes and How to Avoid Them
When working with Binary Search Trees (BSTs), it’s easy to make mistakes that can lead to problems. Here are some common pitfalls and how to avoid them:
Ignoring the BST Property
- Mistake: Forgetting to keep the BST property during insertions and deletions. Each node’s left subtree should only have nodes with values less than the node’s key, and the right subtree should only have nodes with values greater than the node’s key.
- Consequence: This can turn your BST into a general binary tree, losing the efficiency of BST operations like search, insert, and delete.
Not Handling Duplicates Properly
- Mistake: Failing to decide how to handle duplicate values when inserting nodes.
- Consequence: This can lead to an unbalanced tree or undefined behavior. Typically, duplicates are either not allowed or placed consistently in the left or right subtree.
Dealing with Edge Cases
- Mistake: Overlooking edge cases such as inserting into an empty tree or deleting the root node.
- Consequence: Ignoring these cases can cause the program to crash or produce incorrect results.
Summary of Common Mistakes
Mistake | Consequence |
---|---|
Ignoring the BST Property | Loss of efficiency in operations |
Not Handling Duplicates Properly | Unbalanced tree or undefined behavior |
Forgetting Edge Cases | Program crashes or incorrect results |
Avoiding these common mistakes requires a solid understanding of how BSTs work. Always test your implementation with various edge cases and think about how to handle duplicates and keep the tree balanced. Understanding these pitfalls is key to mastering binary search trees.
Analyzing the Performance of Binary Search Trees
Time Complexity
The performance of binary search trees (BSTs) can vary based on their structure. Here’s a quick overview of the time complexity for common operations:
Operation | Best Case | Average Case | Worst Case |
---|---|---|---|
Insertion | O(log n) | O(log n) | O(n) |
Deletion | O(log n) | O(log n) | O(n) |
Search | O(log n) | O(log n) | O(n) |
In the worst case, if the tree becomes unbalanced, the time complexity can degrade to O(n). This happens when the tree resembles a linked list.
Space Complexity
The space complexity for operations in a BST is generally O(n) because, in the worst case, all nodes may need to be stored in memory. Here’s a breakdown:
- Insertion: O(n)
- Deletion: O(n)
- Search: O(n)
Comparing with Other Data Structures
When analyzing BSTs, it’s essential to compare them with other data structures:
- Arrays: Searching in a sorted array is O(log n) using binary search, but insertion and deletion are O(n).
- Linked Lists: Searching is O(n), while insertion and deletion are O(1) if the position is known.
- Hash Tables: Average-case search, insertion, and deletion are O(1), but they can have high space overhead.
Understanding the performance of binary search trees is crucial for selecting the right data structure for your needs. A well-balanced BST can provide efficient operations, making it a valuable tool in computer science.
Conclusion
In summary, while binary search trees offer efficient searching and sorting capabilities, their performance can be significantly affected by their structure. Keeping the tree balanced is key to maintaining optimal performance.
Applications of Binary Search Trees
Binary Search Trees (BSTs) are widely used in various fields due to their efficiency and structure. They help in organizing data in a sorted manner, making it easier to search and retrieve information quickly. Here are some key applications:
Databases and File Systems
- Databases: Many databases, like MySQL and SQLite, utilize BSTs to store and search for data efficiently. They help in speeding up queries by maintaining indexes.
- File Systems: Operating systems use BSTs to manage files. For instance, the Windows NTFS file system employs BSTs to organize the Master File Table (MFT), which contains details about all files on the hard drive.
Computer Networks
- Routing Tables: In computer networks, BSTs can store routing tables, allowing routers to quickly determine the best path for data packets. This enhances the speed and efficiency of data transmission.
Machine Learning and AI
- Decision Trees: BSTs are also used in machine learning algorithms, particularly in decision trees, where they help in making decisions based on input features.
Application Area | Description |
---|---|
Databases | Store and search data efficiently |
File Systems | Organize files on hard drives |
Computer Networks | Manage routing tables for data packets |
Machine Learning | Implement decision trees for predictive modeling |
BSTs are essential in many applications, providing a structured way to manage and retrieve data efficiently.
In summary, the versatility of Binary Search Trees makes them a valuable tool in various domains, from databases to machine learning. Their ability to maintain sorted data allows for quick access and manipulation, which is crucial in today’s data-driven world.
Exploring Variants of Binary Search Trees
Binary Search Trees (BSTs) have different types that help fix some problems with the basic BST. These variants make the tree work better in situations where a regular BST might not perform well. Here are some common types of Binary Search Trees:
AVL Trees
AVL Trees are a kind of self-balancing BST. They keep the heights of the left and right subtrees close to each other. If they get too different, the tree will adjust itself. This helps keep operations fast.
- Key Features:
- Heights of child subtrees differ by at most one.
- Automatically rebalances when needed.
- Ensures O(log n) time for operations.
Red-Black Trees
Red-Black Trees are another type of self-balancing BST. They use colors (red and black) to help keep the tree balanced. The rules about colors help maintain balance during insertions and deletions.
- Key Features:
- Each node is either red or black.
- The tree maintains balance through specific color rules.
- Guarantees O(log n) time for insertions and deletions.
Balanced Binary Search Trees (BBSTs)
Balanced BSTs make sure that the left and right sides of the tree are about the same height. This prevents the tree from becoming too tall and skinny, which can slow down operations.
- Key Features:
- Keeps the tree balanced.
- Prevents worst-case scenarios where the tree acts like a linked list.
- Useful for applications needing consistent performance.
Type of Tree | Key Feature | Use Case |
---|---|---|
AVL Trees | Self-balancing | Real-time systems |
Red-Black Trees | Color-based balancing | Frequent insertions and deletions |
Balanced BSTs | Height balance | Database indexing and file systems |
Understanding these variants is crucial for optimizing performance in various applications. They help ensure that operations remain efficient, even as data changes.
By learning about these different types of Binary Search Trees, you can choose the right one for your needs and improve your programming skills!
Resources for Further Learning
Books and Textbooks
- Understanding Data Structures: A great starting point for beginners.
- Algorithms Unlocked: This book simplifies complex concepts.
- Introduction to Algorithms: A more advanced read for deeper insights.
Online Courses and Tutorials
- Data Structures and Algorithms: Comprehensive courses available on platforms like Coursera and Udemy.
- Interactive Coding Platforms: Websites like Codecademy and LeetCode offer hands-on practice.
- YouTube Channels: Channels like freeCodeCamp and The Coding Train provide free tutorials.
Code Practice Platforms
- HackerRank: Great for practicing coding challenges.
- Codewars: Offers a fun way to improve your skills through challenges.
- GeeksforGeeks: A resourceful site for learning and practicing data structures.
By completing this tutorial, you will understand the technical fundamentals of binary search trees with all the necessary details and practical examples.
If you’re eager to dive deeper into coding, check out our website for more resources! We offer a variety of interactive tutorials and helpful guides that can boost your skills and prepare you for coding interviews. Don’t wait—start your journey today!
Conclusion
In wrapping up our journey through Binary Search Trees (BSTs), it’s clear that mastering this data structure is essential for anyone looking to improve their coding skills. We explored how BSTs work, their key features, and the basic operations like adding, searching, and removing nodes. Understanding these concepts not only helps in coding interviews but also enhances your ability to solve real-world problems efficiently. As you continue to practice and apply what you’ve learned, you’ll find that BSTs can be a powerful tool in your programming toolkit. Keep experimenting and coding, and you’ll become more confident in using Binary Search Trees in your projects!
Frequently Asked Questions
What exactly is a Binary Search Tree (BST)?
A Binary Search Tree (BST) is a special kind of binary tree where each node has a value, and all values in the left part are smaller, while all values in the right part are larger. This setup helps in quickly finding, adding, or removing values.
What are some common uses for Binary Search Trees?
Binary Search Trees are often used in databases, file systems, and applications that require fast searching and sorting of data.
How do I add a new value to a Binary Search Tree?
To add a value, you start at the root and compare it to the value you want to add. If it’s smaller, you go left; if it’s larger, you go right. You keep doing this until you find an empty spot where you can place the new value.
What happens if I try to add a duplicate value?
In a typical Binary Search Tree, duplicates are usually not allowed. If you try to add a duplicate, you can either ignore it or choose to handle it in a specific way, like counting occurrences.
Can a Binary Search Tree become unbalanced?
Yes, if you keep adding values in a sorted order, it can become unbalanced, resembling a linked list. This makes searching slower.
What is the time complexity for searching in a Binary Search Tree?
In the best case, searching in a BST takes O(log n) time, but in the worst case (like an unbalanced tree), it can take O(n) time.
How do I delete a value from a Binary Search Tree?
To delete a value, you find it first. If it has no children, you simply remove it. If it has one child, you replace it with that child. If it has two children, you find the smallest value in its right subtree, replace the value to be deleted with that, and then delete the smallest value.
What are some common mistakes when working with Binary Search Trees?
Common mistakes include forgetting the BST rules when adding or removing nodes, not handling duplicates correctly, and failing to keep the tree balanced.