Mastering Binary Trees for Beginners: A Comprehensive Guide to Understanding and Implementing Data Structures
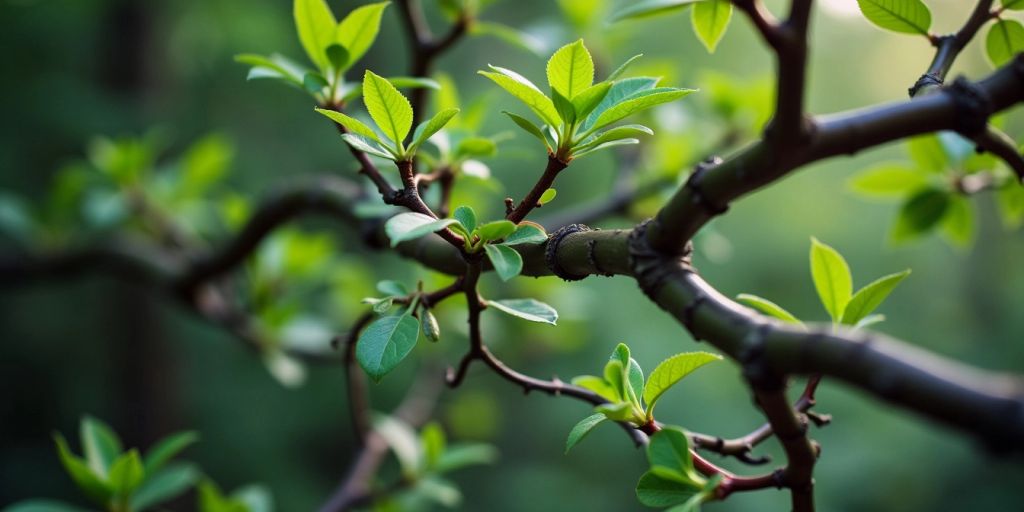
Binary trees are essential structures in computer science, acting as the backbone for many algorithms and applications. This guide aims to demystify binary trees for beginners, breaking down their types, traversal methods, and practical implementations in Python. By understanding these concepts, you’ll be well-equipped to tackle more complex data structures and algorithms.
Key Takeaways
- Binary trees consist of nodes, where each node can have up to two children.
- Different types of binary trees include full, complete, and balanced trees, each serving unique purposes.
- Traversal methods like pre-order, in-order, and post-order allow us to visit nodes in specific sequences.
- Implementing binary trees in Python involves creating classes for nodes and trees and learning how to insert and delete nodes.
- Binary trees have various applications, such as in search algorithms, expression trees, and heaps used in priority queues.
Introduction to Binary Trees for Beginners
What Are Binary Trees?
A binary tree is a special kind of data structure that organizes information in a hierarchical way. In this structure, each node can have at most two children, known as the left child and the right child. This makes it easy to manage and access data efficiently.
Importance of Binary Trees in Computer Science
Binary trees are crucial in computer science for several reasons:
- They help in organizing data in a way that makes searching faster.
- They are used in various algorithms, such as sorting and searching.
- They form the basis for more complex data structures like binary search trees and heaps.
Basic Terminology and Concepts
Understanding binary trees involves knowing some key terms:
- Node: The basic unit of a binary tree, which contains data and links to its children.
- Leaf: A node that does not have any children.
- Height: The length of the longest path from the root to a leaf.
Term | Definition |
---|---|
Node | A unit containing data and links to children. |
Leaf | A node with no children. |
Height | The longest path from the root to a leaf. |
Understanding these basic concepts is essential for mastering binary trees and their applications in programming and algorithms.
In summary, binary trees are a fundamental data structure that plays a vital role in various computer science applications. By grasping the basic terminology and concepts, beginners can build a strong foundation for further learning in data structures and algorithms.
Types of Binary Trees
Binary trees come in various forms, each with unique characteristics and uses. Understanding these types is essential for mastering binary trees.
Full Binary Trees
A full binary tree is a type of binary tree where every node has either 0 or 2 children. This means that no node has only one child. Here are some key points about full binary trees:
- All leaf nodes are at the same level.
- The number of nodes at each level doubles as you go down the tree.
- They are often used in applications where a complete structure is needed.
Complete Binary Trees
A complete binary tree is similar to a full binary tree, but it allows for the last level to be filled from left to right. Here are some features:
- All levels, except possibly the last, are fully filled.
- The last level has all nodes as far left as possible.
- This structure is useful in implementing heaps.
Balanced Binary Trees
A balanced binary tree maintains a height difference of no more than one between the left and right subtrees of any node. This balance ensures efficient operations. Key points include:
- They provide better performance for search operations.
- Common types include AVL trees and Red-Black trees.
- Balancing helps in keeping the tree height minimal, which is crucial for performance.
Type of Binary Tree | Characteristics |
---|---|
Full Binary Tree | Every node has 0 or 2 children |
Complete Binary Tree | All levels are fully filled except possibly the last |
Balanced Binary Tree | Height difference between subtrees is at most 1 |
Understanding the different types of binary trees is crucial for selecting the right structure for your data needs. Each type serves specific purposes and optimizes various operations in computer science.
In summary, knowing the types of binary trees helps in choosing the right one for your application, ensuring efficiency and effectiveness in data handling. Binary trees are fundamental in data structure design, making them a key topic for beginners to grasp.
Binary Tree Traversal Methods
Tree traversal refers to the process of visiting or accessing each node of the tree exactly once in a certain order. Understanding these traversal methods is essential for working with binary trees effectively. Here, we will explore three main traversal techniques: pre-order, in-order, and post-order.
Pre-order Traversal
In pre-order traversal, the order of visiting nodes is: root, left subtree, and then right subtree. This method is useful when you want to process the root before its children. For example, it can be used to create a copy of the tree.
Steps for Pre-order Traversal:
- Visit the root node.
- Recursively traverse the left subtree.
- Recursively traverse the right subtree.
In-order Traversal
In-order traversal visits nodes in the following order: left subtree, root, and then right subtree. This method is particularly important in binary search trees (BSTs) because it retrieves elements in sorted order.
Benefits of In-order Traversal:
- Yields sorted output for BSTs.
- Validates if a tree is a BST.
- Helps find the kth smallest or largest element efficiently.
Post-order Traversal
Post-order traversal visits nodes in this order: left subtree, right subtree, and then root. This method is useful for operations that require processing children before their parent, such as deleting a tree.
Applications of Post-order Traversal:
- Safely deleting a tree.
- Evaluating postfix expressions.
- Resolving dependencies in tasks.
Understanding these traversal methods is crucial for mastering binary trees and their applications in various algorithms and data structures.
Implementing Binary Trees in Python
Setting Up the Environment
To start working with binary trees in Python, you need to set up your environment. Here’s how:
- Install Python: Make sure you have Python installed on your computer. You can download it from the official website.
- Choose an IDE: Use an Integrated Development Environment (IDE) like PyCharm, VSCode, or even Jupyter Notebook for coding.
- Create a New Project: Start a new project where you will write your binary tree code.
Creating Node and Tree Classes
In Python, we can represent a binary tree using classes. Here’s a simple way to create a node and a tree:
class Node:
def __init__(self, value):
self.value = value # The value of the node
self.left = None # Left child
self.right = None # Right child
class BinaryTree:
def __init__(self):
self.root = None # The root of the tree
Inserting and Deleting Nodes
To manage nodes in a binary tree, we need methods for inserting and deleting nodes. Here’s a basic example:
Inserting a Node:
- If the tree is empty, the new node becomes the root.
- If the tree is not empty, compare the value of the new node with the current node and decide to go left or right.
Deleting a Node:
- Find the node to delete.
- If it has no children, simply remove it.
- If it has one child, replace it with its child.
- If it has two children, find the in-order successor to replace it.
Note: Understanding how to insert and delete nodes is crucial for mastering binary trees. These operations help maintain the structure and integrity of the tree.
Summary
Implementing binary trees in Python involves setting up your environment, creating classes for nodes and trees, and writing methods for inserting and deleting nodes. Mastering these basics will set a strong foundation for more advanced tree operations.
Common Operations on Binary Trees
Searching for a Node
Searching for a node in a binary tree is a fundamental operation. Here’s how you can do it:
- Start at the root node.
- Compare the target value with the current node’s value.
- If they match, you’ve found the node!
- If the target value is smaller, search the left subtree.
- If it’s larger, search the right subtree.
- Repeat until you find the node or reach a leaf node.
Searching efficiently is crucial for performance.
Finding the Height of a Tree
The height of a binary tree is the length of the longest path from the root to a leaf. To find it:
- If the tree is empty, the height is -1.
- If it has only one node, the height is 0.
- For other cases, use the formula:
- Height = 1 + max(height of left subtree, height of right subtree)
Counting the Number of Nodes
Counting nodes in a binary tree can be done using a simple recursive method:
- If the tree is empty, return 0.
- Otherwise, return 1 (for the current node) + count of left subtree + count of right subtree.
Operation | Description |
---|---|
Searching for a Node | Find a specific node in the tree. |
Finding the Height | Determine the longest path from root to leaf. |
Counting Nodes | Count all nodes in the tree. |
Understanding these operations is essential for working with binary trees effectively. They form the basis for more complex operations and algorithms.
Applications of Binary Trees
Binary trees are not just theoretical concepts; they have real-world applications that make them essential in various fields of computer science. Here are some key areas where binary trees are utilized:
Binary Search Trees
- Sorted Data Storage: A binary search tree (BST) is a data structure used to store data in a sorted manner. Each node in a BST has at most two children, which allows for efficient searching, insertion, and deletion operations.
- Fast Lookups: Searching for a value in a BST can be done in O(log n) time on average, making it much faster than linear search methods.
- Dynamic Data Handling: BSTs can easily adapt to changes in data, allowing for efficient updates.
Expression Trees
- Mathematical Expressions: Expression trees represent expressions in a tree format, where each leaf node is an operand and each internal node is an operator. This structure is useful for evaluating expressions and converting between different notations (like infix to postfix).
- Compiler Design: Compilers use expression trees to parse and evaluate expressions during code compilation.
- Simplifying Calculations: They help in simplifying complex expressions by breaking them down into manageable parts.
Heaps and Priority Queues
- Task Scheduling: Heaps are a special type of binary tree used to implement priority queues, which are essential in task scheduling systems.
- Efficient Sorting: Heaps can be used to sort data efficiently using heap sort, which has a time complexity of O(n log n).
- Resource Management: In operating systems, heaps help manage resources by prioritizing tasks based on their importance.
Binary trees are versatile structures that enhance the efficiency of various algorithms and applications. Their ability to organize data hierarchically makes them invaluable in computer science.
In summary, binary trees play a crucial role in many applications, from data storage and retrieval to expression evaluation and resource management. Understanding these applications helps in grasping the importance of binary trees in the broader context of data structures and algorithms.
Balancing Binary Trees
Understanding Tree Rotations
Balancing a binary tree is crucial for maintaining its efficiency. A balanced binary tree ensures that operations like insertion, deletion, and searching can be performed quickly. The main technique used to balance trees is through rotations. Here are the types of rotations:
- Left Rotation: This is used when a right-heavy tree needs balancing.
- Right Rotation: This is applied to left-heavy trees.
- Left-Right Rotation: A combination of left and right rotations for specific cases.
Implementing AVL Trees
AVL trees are a type of self-balancing binary search tree. They maintain a height difference of at most 1 between the left and right subtrees. This means that a binary tree is balanced if the height of the tree is O(log n) where n is the number of nodes. For example, the AVL tree maintains O(log n) height. Here’s how to implement an AVL tree:
- Insert a Node: Add the node like in a regular binary search tree.
- Check Balance: After insertion, check the balance factor of each node.
- Perform Rotations: If the tree is unbalanced, perform the necessary rotations to restore balance.
Red-Black Trees
Red-black trees are another type of self-balancing binary tree. They ensure that the tree remains approximately balanced during insertions and deletions. The properties of red-black trees include:
- Each node is either red or black.
- The root is always black.
- Red nodes cannot have red children.
- Every path from a node to its descendant leaves must have the same number of black nodes.
Balancing binary trees is essential for ensuring efficient data operations. Without balance, performance can degrade significantly, leading to slower operations.
By understanding these concepts, you can effectively manage and implement balanced binary trees in your projects.
Advanced Binary Tree Concepts
Threaded Binary Trees
Threaded binary trees are a special type of binary tree where the null pointers are replaced with pointers to the next node in the in-order traversal. This allows for faster traversal without using a stack or recursion. This structure is particularly useful for in-order traversal as it makes it easier to navigate through the tree.
Binary Space Partitioning
Binary space partitioning (BSP) is a method for recursively subdividing a space into convex sets by hyperplanes. This technique is widely used in computer graphics for rendering scenes. It helps in efficiently organizing objects in a scene, allowing for quick visibility determination. Here’s a simple breakdown of its advantages:
- Efficient Rendering: Reduces the number of objects to be rendered.
- Collision Detection: Helps in determining which objects intersect.
- Scene Management: Organizes complex scenes into manageable parts.
Segment Trees
Segment trees are a powerful data structure used for storing intervals or segments. They allow querying which segments overlap with a given point efficiently. This is particularly useful in scenarios like:
- Range Queries: Quickly find the sum or minimum in a range of values.
- Dynamic Updates: Efficiently update values in the segments.
- Interval Overlap: Determine if a point lies within any segment.
Understanding these advanced concepts can significantly enhance your ability to work with binary trees and their applications in various fields. They provide a deeper insight into how data can be structured and manipulated effectively.
Summary
In summary, mastering advanced binary tree concepts like threaded binary trees, binary space partitioning, and segment trees can greatly improve your programming skills. These structures not only optimize performance but also open up new possibilities for solving complex problems in computer science.
Highlighted Concept
The concept of phylo2vec is an example of how binary trees can be utilized in biological data analysis, showcasing their versatility in different domains.
Troubleshooting and Debugging Binary Trees
Common Errors and Their Fixes
When working with binary trees, you might encounter several common issues. Here are some typical errors and how to fix them:
- Null Pointer Exceptions: Ensure that you check for null nodes before accessing their properties.
- Infinite Loops: Make sure your traversal methods have proper base cases to prevent endless recursion.
- Incorrect Node Connections: Double-check that you are linking child nodes correctly when inserting or deleting nodes.
Debugging Techniques
Debugging binary trees can be tricky, but here are some effective strategies:
- Print Statements: Use print statements to display the current node and its children during traversal.
- Visual Representation: Draw the tree structure on paper or use a tool to visualize the tree at different stages.
- Unit Tests: Write tests for each function to ensure they work as expected, especially for insertion and deletion.
Optimizing Performance
To enhance the performance of your binary tree operations, consider the following tips:
- Use Iterative Methods: For large trees, iterative methods can help avoid stack overflow issues.
- Balance the Tree: Implement balancing techniques to keep the tree height minimal, improving search times.
- Profile Your Code: Use profiling tools to identify bottlenecks in your tree operations.
Debugging binary trees requires patience and careful observation. By following systematic approaches, you can identify and fix issues effectively.
Summary
Understanding common errors, employing debugging techniques, and optimizing performance are crucial for mastering binary trees. With practice, you will become more adept at troubleshooting and ensuring your binary tree implementations run smoothly.
Highlighted Techniques
- Model debugging strategies can be applied to improve your understanding of binary trees. Learning techniques for visualizing data can significantly enhance your debugging process.
Practical Projects Using Binary Trees
Building a Simple Database Index
Creating a simple database index using binary trees can help you understand how data is organized and retrieved efficiently. Here’s how you can approach this project:
- Define the structure of your binary tree nodes to hold data and pointers to left and right children.
- Implement insertion methods to add new records while maintaining the binary search tree properties.
- Create search functions to retrieve records based on keys, showcasing the efficiency of binary trees.
Creating a Huffman Tree for Data Compression
Huffman coding is a popular method for data compression. You can implement this using binary trees:
- Build a frequency table of characters from the input data.
- Create a priority queue to build the Huffman tree based on character frequencies.
- Generate codes for each character by traversing the tree, where left edges represent 0 and right edges represent 1.
Developing a Decision Tree for Machine Learning
Decision trees are widely used in machine learning for classification tasks. Here’s a simple way to create one:
- Select a dataset that you want to classify.
- Choose a splitting criterion (like Gini impurity or information gain) to decide how to split the data at each node.
- Recursively build the tree by splitting the dataset until you reach a stopping condition (like a maximum depth or minimum samples per leaf).
These projects are tailored to offer hands-on learning experiences, allowing beginners to explore various data structures while honing their programming skills.
By working on these projects, you will gain practical experience with binary trees and understand their applications in real-world scenarios.
Further Reading and Resources
Recommended Books and Articles
Here are some great books and articles to help you dive deeper into binary trees and data structures:
- "Data Structures and Algorithms in Python" by Michael T. Goodrich et al.
- "Introduction to Algorithms" by Thomas H. Cormen et al.
- "Data Structures and Algorithms in Java" by Robert Lafore
Online Courses and Tutorials
Consider these online resources for structured learning:
- Coursera – Offers various courses on data structures.
- edX – Provides free courses from top universities.
- Udacity – Features nanodegree programs focused on data structures.
Practice Problems and Challenges
To sharpen your skills, try these exercises:
- Implement a binary search tree and perform basic operations like insertion and deletion.
- Write a program to find the height of a binary tree.
- Create a function to count the number of nodes in a tree.
Mastering data structures is essential for any aspiring programmer. Practice regularly to build your confidence and skills!
If you’re eager to dive deeper into coding and enhance your skills, check out our website! We offer a variety of resources and interactive tutorials designed to help you succeed in coding interviews. Don’t wait—start your journey today!
Conclusion
In summary, getting to know and using binary trees is a key part of learning computer science. These trees are important for many tasks, like evaluating expressions and managing data. Each way to go through a binary tree—whether it’s in-order for sorting, pre-order for copying, or post-order for safely removing nodes—has its own special use. Both the recursive and iterative methods have their benefits: recursion makes the code easier to read, while iterative methods can help avoid problems with memory limits. This guide aims to give you a solid grasp of binary tree traversal techniques and shows how to choose the right method based on what you need. By mastering these skills, you’ll be better equipped to tackle various coding challenges.
Frequently Asked Questions
What is a binary tree?
A binary tree is a type of data structure that has nodes, where each node can have up to two children. It helps organize data in a way that makes it easier to search and manage.
Why are binary trees important?
Binary trees are important because they are used in many areas of computer science, like databases and search algorithms, to store and retrieve data efficiently.
What are the different types of binary trees?
There are several types of binary trees, including full binary trees, complete binary trees, and balanced binary trees, each with its own specific properties.
How do you traverse a binary tree?
You can traverse a binary tree using different methods, such as pre-order, in-order, and post-order traversal, which determine the order in which you visit the nodes.
Can I implement a binary tree in Python?
Yes, you can implement a binary tree in Python by creating classes for the nodes and the tree itself, allowing you to insert, delete, and manage nodes.
What common operations can I perform on binary trees?
Common operations on binary trees include searching for a node, finding the height of the tree, and counting the total number of nodes.
What are some real-world applications of binary trees?
Binary trees are used in various applications, like binary search trees for fast searching, expression trees for evaluating mathematical expressions, and heaps for managing priority queues.
How can I balance a binary tree?
You can balance a binary tree using techniques like tree rotations and by implementing specific types of trees, such as AVL trees or red-black trees.