Understanding the Differences: Binary Tree vs Binary Search Tree
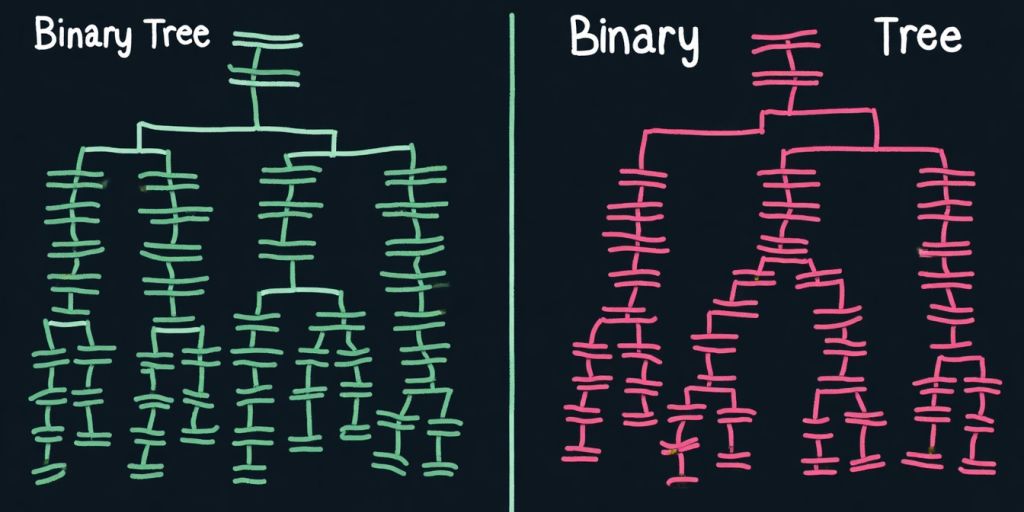
When it comes to organizing data in computer science, two important structures are the binary tree and the binary search tree (BST). Both play crucial roles in data management, but they have distinct characteristics and uses. Understanding these differences can help in choosing the right structure for specific applications. This article will break down these concepts in a simple way, making it easier for anyone to grasp their importance and functionality.
Key Takeaways
- A binary tree can have up to two children per node, while a binary search tree arranges nodes in a specific order.
- In a binary search tree, the left child is always less than the parent, and the right child is always greater.
- Binary trees are used for various applications like expression trees and decision trees, while binary search trees are ideal for fast searching and sorting.
- Insertion and deletion operations in binary trees do not follow a specific order, unlike in binary search trees.
- Traversing a binary tree can take longer since there is no order, while a binary search tree allows for quicker searches due to its structure.
- Different types of binary trees include full, complete, and perfect trees, whereas binary search trees have types like AVL and Red-Black trees.
- Balancing is crucial in binary search trees to maintain efficiency, while binary trees can become unbalanced easily.
- Understanding these structures helps in selecting the right one for coding challenges and real-world applications.
Definition and Basic Concepts
What Is a Binary Tree?
A binary tree is a type of data structure where each node can have at most two children, referred to as the left and right children. The top node is called the root, while nodes without children are known as leaf nodes. This structure is useful in various applications, such as expression trees and decision trees.
What Is a Binary Search Tree?
A binary search tree (BST) is a special kind of binary tree where the nodes are arranged in a specific order. In a BST:
- All nodes in the left subtree have values less than the parent node.
- All nodes in the right subtree have values greater than the parent node.
This arrangement allows for efficient searching, insertion, and deletion operations.
Key Differences Between Binary Tree and Binary Search Tree
Feature | Binary Tree | Binary Search Tree |
---|---|---|
Structure | No specific order | Ordered structure |
Searching | Slower, O(n) | Faster, O(log n) |
Insertion/Deletion | More complex | More efficient |
Common Terminologies in Binary Trees
- Root Node: The topmost node in the tree.
- Leaf Node: A node with no children.
- Height: The longest path from the root to a leaf.
Common Terminologies in Binary Search Trees
- Node: An element in the tree.
- Subtree: A tree formed by a node and its descendants.
- BST Characteristics: The rules that define the order of nodes in a BST.
Importance of Understanding These Structures
Understanding binary trees and binary search trees is crucial for efficient data management. They are foundational concepts in computer science that help in organizing data for quick access and manipulation.
Mastering these structures can significantly improve your programming skills and problem-solving abilities.
Structure and Properties
Node Structure in Binary Trees
A binary tree consists of nodes, where each node can have up to two children: a left child and a right child. The structure can be visualized as follows:
- Root Node: The topmost node.
- Leaf Nodes: Nodes without children.
- Internal Nodes: Nodes with at least one child.
Node Structure in Binary Search Trees
In a binary search tree (BST), each node contains one key and can have up to two children. The arrangement is such that:
- All nodes in the left subtree have values less than the node’s value.
- All nodes in the right subtree have values greater than the node’s value.
Properties of Binary Trees
- Each node can have zero, one, or two children.
- The height is the longest path from the root to a leaf node.
- Supports various traversal methods like in-order, pre-order, and post-order.
Properties of Binary Search Trees
- Nodes are arranged in a specific order for efficient searching.
- No duplicate nodes are allowed.
- Both left and right subtrees must also be binary search trees.
Height and Depth in Binary Trees
- Height: The number of edges in the longest path from the root to a leaf.
- Depth: The number of edges from the root to a specific node.
Height and Depth in Binary Search Trees
- The height of a BST affects its performance. A balanced BST has a height of log(n), while an unbalanced one can have a height of n.
- Depth is calculated similarly to binary trees, impacting search efficiency.
Understanding the structure and properties of these trees is crucial for effective data management and retrieval.
Summary Table
Feature | Binary Tree | Binary Search Tree |
---|---|---|
Node Structure | Up to 2 children per node | Ordered nodes with 2 children |
Height | Varies | Log(n) for balanced trees |
Search Efficiency | O(n) | O(log n) for balanced trees |
Types of Binary Trees
Full Binary Tree
A full binary tree is a type of binary tree where every node has either zero or two children. This means that no node can have just one child. In a full binary tree, all leaf nodes are at the same level.
Complete Binary Tree
A complete binary tree is a binary tree in which all levels are fully filled except possibly for the last level, which is filled from left to right. This structure ensures that the tree is as compact as possible.
Perfect Binary Tree
A perfect binary tree is a special type of complete binary tree where all internal nodes have exactly two children, and all leaf nodes are at the same level. This means that every level of the tree is fully filled.
Extended Binary Tree
An extended binary tree is a binary tree where every node has either two children or is a leaf node. If a node does not have a child, it is represented by a null pointer. This helps in maintaining the structure of the tree.
Balanced Binary Tree
A balanced binary tree is a binary tree where the height of the left and right subtrees of any node differ by at most one. This balance helps in maintaining efficient operations like insertion and deletion.
Skewed Binary Tree
A skewed binary tree is a type of binary tree where each parent node has only one child. This can either be a left-skewed tree (all nodes have left children) or a right-skewed tree (all nodes have right children). This structure can lead to inefficiencies in operations.
Understanding the different types of binary trees is crucial for selecting the right structure for specific applications. Each type has its own advantages and disadvantages, making them suitable for various scenarios.
Type of Binary Tree | Description |
---|---|
Full Binary Tree | Every node has 0 or 2 children. |
Complete Binary Tree | All levels are fully filled except possibly the last level. |
Perfect Binary Tree | All internal nodes have 2 children, and all leaf nodes are at the same level. |
Extended Binary Tree | Every node has 2 children or is a leaf node. |
Balanced Binary Tree | Height of left and right subtrees differ by at most one. |
Skewed Binary Tree | Each parent node has only one child. |
Types of Binary Search Trees
AVL Trees
AVL trees are a type of self-balancing binary search tree. In an AVL tree, the heights of the two child subtrees of any node differ by at most one. This property ensures that the tree remains balanced, leading to efficient operations. AVL trees provide fast search, insertion, and deletion.
Red-Black Trees
Red-black trees are another type of self-balancing binary search tree. Each node has an extra bit for denoting the color of the node, either red or black. This coloring helps maintain balance during insertions and deletions. The properties of red-black trees ensure that the longest path from the root to a leaf is no more than twice as long as the shortest path.
Splay Trees
Splay trees are a type of binary search tree that performs a splay operation on access. This means that when a node is accessed, it is moved to the root of the tree. This can improve the time complexity for frequently accessed nodes, making them faster to reach in the future.
Tango Trees
Tango trees are a hybrid of binary search trees and splay trees. They maintain balance by using a combination of splaying and a secondary structure to keep track of the tree’s shape. This allows for efficient operations while keeping the tree balanced.
Treap
A treap is a combination of a binary search tree and a heap. Each node has a key and a priority. The binary search tree property is maintained based on the keys, while the heap property is maintained based on the priorities. This structure allows for efficient search and insertion operations.
Scapegoat Trees
Scapegoat trees are a type of binary search tree that maintains balance by occasionally rebuilding the tree. When a node is inserted and the tree becomes unbalanced, a scapegoat node is identified, and the tree is rebuilt to restore balance. This method ensures that the tree remains efficient for operations.
Understanding the different types of binary search trees is crucial for selecting the right structure for specific applications. Each type has its own strengths and weaknesses, making them suitable for various scenarios.
Summary of Types of Binary Search Trees
Type | Key Feature |
---|---|
AVL Trees | Self-balancing with height difference ≤ 1 |
Red-Black Trees | Color-coded for balance |
Splay Trees | Moves accessed nodes to the root |
Tango Trees | Hybrid of splay and binary search trees |
Treap | Combines binary search and heap properties |
Scapegoat Trees | Rebuilds tree to maintain balance |
Insertion Operations
Inserting Nodes in Binary Trees
In a binary tree, nodes can be added in any order. This means that when you insert a new node, it can go anywhere in the tree without following a specific rule. This flexibility allows for easy insertion but can lead to an unbalanced tree.
Inserting Nodes in Binary Search Trees
In contrast, when inserting nodes in a binary search tree (BST), nodes are inserted according to their values, maintaining the BST property. This means that for any given node, all values in the left subtree are smaller, and all values in the right subtree are larger. This structure allows for efficient searching and retrieval of data.
Complexity of Insertion in Binary Trees
The time complexity for inserting a node in a binary tree is generally O(1) if you are adding it at the end. However, if you need to maintain a specific structure, it can take longer.
Complexity of Insertion in Binary Search Trees
For a balanced BST, the time complexity for insertion is O(log n). However, in the worst case, if the tree becomes unbalanced, it can degrade to O(n).
Common Mistakes During Insertion
- Ignoring the BST property: When inserting into a BST, always check the values to maintain the structure.
- Inserting duplicates: Decide how to handle duplicates before starting the insertion process.
- Not balancing the tree: Regularly check and balance the tree to maintain efficiency.
Best Practices for Insertion
- Always validate the value before inserting.
- Use a recursive approach for cleaner code.
- Consider using self-balancing trees like AVL or Red-Black trees for better performance.
Understanding the differences in insertion methods is crucial for optimizing performance in data structures. Binary trees allow for flexible insertion, while binary search trees require order to maintain efficiency.
Deletion Operations
Deleting Nodes in Binary Trees
Deleting a node in a binary tree can be straightforward or complex, depending on the node’s position. Here are the main steps:
- Find the node to be deleted.
- If the node has no children, simply remove it.
- If the node has one child, replace it with its child.
- If the node has two children, find its inorder predecessor or inorder successor, copy its value to the node, and then delete the predecessor or successor.
Deleting Nodes in Binary Search Trees
In a binary search tree (BST), deletion is a bit more structured due to the properties of the tree. The steps are similar:
- Locate the node to delete.
- If it has no children, remove it directly.
- If it has one child, link its parent to its child.
- If it has two children, the trick is to find the inorder successor of the node. Copy contents of the inorder successor to the node, and delete the inorder successor.
Complexity of Deletion in Binary Trees
The time complexity for deleting a node in a binary tree is generally O(n) in the worst case, as you may need to traverse the entire tree to find the node.
Complexity of Deletion in Binary Search Trees
For a balanced BST, the deletion operation has a time complexity of O(log n). However, in the worst case (for an unbalanced tree), it can degrade to O(n).
Common Mistakes During Deletion
- Forgetting to update the parent node’s link.
- Not handling the case of two children correctly.
- Failing to maintain the BST properties after deletion.
Best Practices for Deletion
- Always check the structure of the tree after deletion to ensure it remains a valid BST.
- Use iterative methods for deletion to avoid stack overflow in deep trees.
- Consider balancing the tree after multiple deletions to maintain efficiency.
Traversal Techniques
In-Order Traversal in Binary Trees
In in-order traversal, the nodes are visited in the following order: left child, current node, and then right child. This method is particularly useful for binary search trees as it retrieves the nodes in sorted order.
Pre-Order Traversal in Binary Trees
In pre-order traversal, the sequence is: current node, left child, and then right child. This technique is often used to create a copy of the tree or to get a prefix expression of the tree.
Post-Order Traversal in Binary Trees
In post-order traversal, the order is: left child, right child, and then the current node. This method is useful for deleting the tree or evaluating postfix expressions.
In-Order Traversal in Binary Search Trees
In binary search trees, in-order traversal guarantees that the nodes are accessed in ascending order. This is a key feature that differentiates binary search trees from regular binary trees.
Pre-Order Traversal in Binary Search Trees
Pre-order traversal in binary search trees can be used to create a copy of the tree structure, maintaining the order of nodes as they are inserted.
Post-Order Traversal in Binary Search Trees
Post-order traversal is useful for operations like deleting nodes, as it ensures that child nodes are processed before their parent nodes.
Summary of Traversal Techniques
Traversal Type | Order of Visit | Use Case |
---|---|---|
In-Order | Left, Node, Right | Sorted output for BST |
Pre-Order | Node, Left, Right | Copying tree, prefix expression |
Post-Order | Left, Right, Node | Deleting tree, postfix expression |
Tree traversal techniques are essential for accessing each node of the tree exactly once in a certain order. Understanding these methods is crucial for effective tree manipulation and data retrieval.
Search Operations
Searching in Binary Trees
Searching in a binary tree can be quite simple but also inefficient. You typically have to check each node one by one. This means that the time it takes to find a value can be quite long, especially if the tree is large. Here are some key points to remember:
- No specific order: Unlike binary search trees, binary trees do not have a specific order for their nodes.
- Traversal needed: You may need to use traversal methods like in-order, pre-order, or post-order to find a value.
- Time complexity: The worst-case time complexity can be O(n), where n is the number of nodes.
Searching in Binary Search Trees
Searching in a binary search tree (BST) is much more efficient. For searching a value in a BST, consider it as a sorted array. This allows us to use the binary search algorithm, which is faster. Here’s how it works:
- Start at the root node.
- Compare the value you are searching for with the current node’s value.
- If the value is smaller, move to the left child; if larger, move to the right child.
- Repeat until you find the value or reach a leaf node.
Complexity of Search in Binary Trees
The complexity of searching in binary trees can vary:
- Best case: O(1) if the root node is the target.
- Average case: O(n) if the tree is unbalanced.
- Worst case: O(n) if the tree is a straight line.
Complexity of Search in Binary Search Trees
In a binary search tree, the search complexity is generally better:
- Best case: O(1) if the root node is the target.
- Average case: O(log n) if the tree is balanced.
- Worst case: O(n) if the tree is unbalanced.
Binary Search Algorithm
The binary search algorithm is a method used to find a specific value in a sorted array. It works by repeatedly dividing the search interval in half. If the value is less than the middle element, it narrows the interval to the lower half; otherwise, it narrows it to the upper half. This method is efficient and reduces the number of comparisons needed.
Common Mistakes During Search
When searching in binary trees or binary search trees, some common mistakes include:
- Not checking both children: In binary trees, you might miss a value if you only check one side.
- Assuming order in binary trees: Remember, binary trees do not have a specific order.
- Ignoring tree balance: In binary search trees, an unbalanced tree can lead to poor performance.
Understanding how to search effectively in these structures is crucial for optimizing performance in various applications.
Applications of Binary Trees
Expression Trees
Expression trees are a type of binary tree used to represent expressions. Each internal node corresponds to an operator, and each leaf node corresponds to an operand. This structure allows for easy evaluation of expressions by traversing the tree.
Decision Trees
Decision trees are used in machine learning and statistics for making decisions based on certain conditions. Each node represents a decision point, and the branches represent the possible outcomes. They help in making informed choices based on data.
Hierarchical Representations
Binary trees can represent hierarchical data structures, such as organizational charts or file systems. Each node can represent an entity, and the connections show relationships between them.
Binary Heaps
Binary heaps are a special type of binary tree used to implement priority queues. They allow for efficient retrieval of the highest (or lowest) priority element, making them useful in algorithms like Dijkstra’s.
Priority Queues
Priority queues are abstract data types where each element has a priority. Binary trees can efficiently manage these priorities, allowing for quick access to the highest priority element.
Huffman Coding
Huffman coding is a compression algorithm that uses binary trees to represent variable-length codes for characters. This method reduces the overall size of data, making it efficient for storage and transmission.
In summary, binary trees are versatile structures that find applications in various fields, from computer science to data management. Their ability to represent hierarchical data makes them essential in many algorithms and systems.
Application | Description |
---|---|
Expression Trees | Represent mathematical expressions for evaluation. |
Decision Trees | Aid in decision-making processes based on conditions. |
Hierarchical Data | Represent structures like organizational charts or file systems. |
Binary Heaps | Implement priority queues for efficient element retrieval. |
Huffman Coding | Use variable-length codes for data compression. |
Applications of Binary Search Trees
Database Indexing
Binary search trees are widely used in databases to maintain sorted data. This allows for quick retrieval of records. Efficient searching is crucial in database management systems.
Dictionary Implementations
In programming, binary search trees can be used to implement dictionaries. Each word can be stored as a node, making it easy to search for definitions or synonyms.
Range Queries
Binary search trees allow for efficient range queries. For example, you can quickly find all numbers between two values. This is useful in applications like statistical analysis.
Auto-Completion
When typing in search bars, binary search trees can help suggest words based on the letters typed so far. This enhances user experience by providing quick suggestions.
Data Retrieval
Binary search trees enable fast data retrieval. When data is organized in a BST, finding specific items becomes much quicker compared to other structures.
Sorting
Binary search trees can also be used for sorting data. By performing an in-order traversal, you can retrieve data in sorted order. This is a simple yet effective way to sort items.
In summary, a binary search tree (BST) is a data structure used for storing data in a sorted manner. Each node in a BST has at most two children, which helps in maintaining order and efficiency in various applications.
Advantages of Binary Trees
Simplicity of Structure
Binary trees are easy to understand and implement. Their structure is straightforward, making them a great choice for beginners learning about data structures. This simplicity allows for quick learning and application.
Ease of Implementation
Implementing binary trees is generally simpler than other data structures. You can create a binary tree with just a few lines of code, which is beneficial for quick projects or prototypes.
Versatility in Applications
Binary trees can be used in various applications, such as:
- Expression Trees: Used in compilers to represent expressions.
- Decision Trees: Useful in machine learning for making decisions.
- Hierarchical Representations: Ideal for representing data in a structured way.
Efficient Traversal Methods
Binary trees support different traversal methods, including:
- In-Order Traversal
- Pre-Order Traversal
- Post-Order Traversal
These methods allow for flexible data access and manipulation.
Memory Usage
Binary trees can be memory efficient, especially when compared to other data structures that may require more overhead. They can dynamically adjust to the amount of data being stored.
Flexibility
Binary trees can easily adapt to various types of data and can be modified to suit specific needs. This flexibility makes them suitable for a wide range of applications.
Binary trees are versatile enough to represent virtually any hierarchical relationship. They can be applied in virtually any real-world setting, such as file systems, databases, and more.
Advantages of Binary Search Trees
Efficient Search Operations
Binary search trees (BSTs) are designed for quick data retrieval. They allow you to find values faster than in regular binary trees. This is because you can skip large parts of the tree based on comparisons. For example:
- If the value you are looking for is less than the current node, you only check the left subtree.
- If it’s greater, you check the right subtree.
Ordered Data Storage
BSTs keep data in a specific order. This means:
- The left child is always less than the parent node.
- The right child is always greater than the parent node.
This organization makes it easier to manage and understand the data.
Dynamic Data Management
BSTs can grow and shrink as needed. You can easily add or remove nodes without needing to reorganize the entire structure. This flexibility is important for applications that require frequent updates.
Scalability
As your data grows, BSTs can handle larger datasets efficiently. They maintain their performance even as more nodes are added, making them suitable for applications that need to scale.
Memory Usage
BSTs are generally efficient in terms of memory. They only use as much space as needed for the nodes, unlike some other data structures that may require extra space for pointers or links.
In summary, binary search trees provide a systematic way of storing data that allows for quick retrieval, making them a crucial tool in many applications such as databases, search engines, and more.
Disadvantages of Binary Trees
Potential for Imbalance
Binary trees can become unbalanced, which means that one side of the tree can grow taller than the other. This can lead to inefficient operations. An unbalanced tree can degrade performance significantly, making it harder to search for elements.
Complexity in Operations
Operations like insertion and deletion can become complicated in binary trees. If the tree is not balanced, these operations may require traversing many nodes, leading to longer processing times.
Memory Overhead
Binary trees can consume more memory than necessary. Each node requires additional space for pointers to its children, which can lead to higher memory usage compared to other data structures like arrays.
Traversal Inefficiencies
Traversing a binary tree can be inefficient, especially if the tree is unbalanced. In the worst case, you may need to visit every node to find a specific value, which can be time-consuming.
Insertion and Deletion Complexities
Inserting or deleting nodes in a binary tree can be tricky. If not done carefully, it can lead to an unbalanced tree, which further complicates future operations.
Limited Use Cases
While binary trees are versatile, they may not be suitable for all applications. For example, they are not the best choice for scenarios requiring frequent searching, as their performance can lag behind that of binary search trees.
In summary, while binary trees are useful, they come with several disadvantages that can impact their efficiency and effectiveness in certain situations. Understanding these limitations is crucial for choosing the right data structure for your needs.
Disadvantages of Binary Search Trees
Need for Balancing
Binary search trees can become unbalanced, which leads to poor performance. When a BST is unbalanced, it can behave like a linked list, making operations slow. This can happen when data is inserted in a sorted order.
Complex Implementation
Implementing a binary search tree can be tricky. You need to ensure that the tree remains balanced after every insertion and deletion. This complexity can lead to mistakes, especially for beginners.
Memory Overhead
Binary search trees require extra memory for pointers. Each node has pointers to its children, which can add up, especially in large trees. This can be a concern in memory-limited environments.
Potential for Degeneration
If not managed properly, a binary search tree can degenerate into a linear structure. This means that the time complexity for search, insertion, and deletion can degrade to O(n), which is inefficient.
Insertion and Deletion Complexities
The time taken for insertion and deletion can vary significantly based on the tree’s structure. In the worst case, these operations can take longer than expected, especially in unbalanced trees.
Limited Use Cases
While binary search trees are useful, they are not always the best choice for every application. In some scenarios, other data structures may perform better.
In summary, while binary search trees offer efficient searching, they come with challenges that can affect performance and implementation. Understanding these disadvantages is crucial for effective data management.
Balancing Techniques in Binary Search Trees
Importance of Balancing
Balancing a binary search tree is crucial for maintaining its efficiency. A balanced binary tree ensures that the height of the tree is approximately O(log n), where n is the number of nodes. This balance allows for faster search, insertion, and deletion operations.
Rotations in AVL Trees
AVL trees are a type of self-balancing binary search tree. They use rotations to maintain balance. Here are the types of rotations:
- Single Right Rotation: Used when a left-heavy tree needs balancing.
- Single Left Rotation: Used for a right-heavy tree.
- Double Rotations: A combination of left and right rotations to fix more complex imbalances.
Coloring Rules in Red-Black Trees
Red-Black trees maintain balance through specific coloring rules:
- Each node is either red or black.
- The root is always black.
- Red nodes cannot have red children.
- Every path from a node to its descendant leaves must have the same number of black nodes.
Splaying in Splay Trees
Splay trees are another type of self-adjusting binary search tree. They move frequently accessed elements closer to the root through a process called splaying. This can improve access times for recently accessed nodes.
Balancing in Tango Trees
Tango trees use a unique approach by maintaining a balance between the tree structure and the access frequency of nodes. They adjust the tree based on how often nodes are accessed, ensuring that frequently accessed nodes are quicker to reach.
Scapegoat Tree Adjustments
Scapegoat trees are a type of binary search tree that rebalances itself after certain operations. If a node becomes too unbalanced, the tree will rebuild itself to restore balance, ensuring that the height remains manageable.
Balancing techniques are essential for maintaining the efficiency of binary search trees. Without them, operations can degrade to linear time, making the tree less effective for large datasets.
Comparative Analysis
Performance Comparison
When comparing binary trees and binary search trees (BSTs), it’s essential to consider their performance in various operations:
- Binary Trees:
- Binary Search Trees:
Use Case Scenarios
Both structures have their unique applications:
- Binary Trees are often used in:
- Binary Search Trees are preferred for:
Complexity Analysis
The complexity of operations varies significantly:
- Binary Trees:
- Simpler structure but can lead to inefficiencies due to lack of order.
- Binary Search Trees:
- More complex but provide faster search, insert, and delete operations due to their ordered nature.
Memory Usage Comparison
Memory usage can also differ:
- Binary Trees: Generally use less memory per node since they don’t require additional pointers for balancing.
- Binary Search Trees: May require more memory due to balancing techniques, but they optimize search operations.
Scalability
- Binary Trees: Can become inefficient as they grow larger without a specific structure.
- Binary Search Trees: More scalable due to their ordered nature, allowing for efficient operations even as the dataset grows.
Understanding the difference between binary tree and binary search tree is crucial for selecting the right data structure for your needs. A visual comparison of binary trees and binary search trees (BST) can help clarify their structural differences and how their properties affect data management.
Common Mistakes and How to Avoid Them
Mistakes in Insertion
When inserting nodes into binary trees or binary search trees, common mistakes can lead to incorrect structures. Here are some frequent errors:
- Not maintaining the binary search tree property: Ensure that for each node, the left child is smaller and the right child is larger.
- Inserting duplicates: Decide on a strategy for handling duplicates, as they can disrupt the tree’s structure.
- Forgetting to update pointers: Always check that pointers are correctly assigned after insertion.
Mistakes in Deletion
Deleting nodes can be tricky. Here are some common pitfalls:
- Not considering all cases: Deleting a node with two children requires special handling.
- Failing to update parent pointers: Ensure that the parent node’s pointer is updated after deletion.
- Memory leaks: Always free memory for deleted nodes to avoid leaks.
Mistakes in Traversal
Traversal methods can be confusing. Common mistakes include:
- Incorrect order of traversal: Make sure to follow the correct order for in-order, pre-order, or post-order traversals.
- Not visiting all nodes: Ensure that your traversal method visits every node in the tree.
- Using the wrong data structure: Ensure you are using the right stack or queue for the traversal method.
Mistakes in Search
Searching in trees can lead to errors if not done correctly. Here are some common mistakes:
- Not checking both subtrees: When searching, always check both left and right subtrees.
- Assuming the tree is balanced: Be aware that unbalanced trees can lead to inefficient searches.
- Ignoring the base case: Always check if the current node is null before proceeding.
Avoiding these mistakes is crucial for maintaining the integrity of your binary trees and binary search trees.
Avoiding Imbalance
To prevent imbalance in binary search trees, consider the following:
- Use self-balancing trees: Implement AVL or Red-Black trees to maintain balance automatically.
- Regularly check balance: After insertions and deletions, check the balance of the tree.
- Rebalance when necessary: If the tree becomes unbalanced, perform rotations to restore balance.
Best Practices
To ensure smooth operations with binary trees and binary search trees, follow these best practices:
- Understand the properties of each structure: Knowing the differences helps in choosing the right tree for your needs.
- Practice coding tree operations: Regular practice can help solidify your understanding.
- Review and debug your code: Always check your code for logical errors and test with various cases.
Future Trends and Developments
Advancements in Tree Structures
The future of tree data structures looks promising with ongoing innovations. Researchers are focusing on enhancing the efficiency and flexibility of these structures. Some key advancements include:
- Dynamic balancing techniques to maintain optimal performance.
- Hybrid structures that combine features of different tree types.
- Integration with machine learning for smarter data handling.
Innovations in Search Algorithms
As technology evolves, search algorithms are becoming more sophisticated. The focus is on:
- Faster search times through improved algorithms.
- Adaptive algorithms that learn from user behavior.
- Parallel processing to handle large datasets efficiently.
Impact of Machine Learning
Machine learning is set to revolutionize how we use tree structures. It can:
- Enhance data retrieval processes.
- Improve decision-making in applications like AI.
- Enable predictive analytics for better insights.
Integration with Big Data
With the rise of big data, tree structures are being adapted to manage vast amounts of information. This includes:
- Scalable tree designs that can grow with data.
- Optimized storage solutions to reduce memory usage.
- Real-time processing capabilities for immediate data access.
Future Applications
The potential applications of advanced tree structures are vast. They include:
- Smart cities where data is managed efficiently.
- Healthcare systems for better patient data management.
- Financial services for real-time transaction processing.
The future of tree data structures is bright, with endless possibilities for innovation and application. Understanding these trends is crucial for anyone interested in technology and data management.
Research Directions
Ongoing research is crucial for the evolution of tree structures. Key areas include:
- Exploring new algorithms for better performance.
- Investigating cross-disciplinary applications in various fields.
- Developing educational resources to teach these concepts effectively.
In summary, the future of tree data structures is filled with exciting possibilities, driven by advancements in technology and the need for efficient data management.
Expert Opinions and Case Studies
Insights from Computer Scientists
Computer scientists often emphasize the importance of understanding data structures. They note that binary trees and binary search trees are foundational concepts in computer science. Here are some key insights:
- Real-life applications of data structures and algorithms are crucial for efficient programming.
- Understanding these structures helps in optimizing search and retrieval processes.
- They are essential for various applications, including databases and AI.
Case Study: Binary Trees in AI
In artificial intelligence, binary trees are used for decision-making processes. For example, they can help in:
- Classifying data based on certain features.
- Making predictions by traversing the tree.
- Optimizing search in large datasets.
Case Study: Binary Search Trees in Databases
Binary search trees play a vital role in database indexing. They allow for:
- Faster search operations compared to linear search methods.
- Dynamic data management, enabling easy insertions and deletions.
- Efficient range queries, which are essential for data retrieval.
Understanding the real-life applications of data structures and algorithms can significantly enhance programming skills and efficiency.
Industry Applications
Many industries utilize binary trees and binary search trees for various purposes:
- Finance: For managing hierarchical data.
- Healthcare: In decision support systems.
- E-commerce: For product categorization and search optimization.
These insights and case studies highlight the relevance of binary trees and binary search trees in both theoretical and practical applications, showcasing their significance in the tech world.
Resources for Further Learning
Books on Data Structures
- "Data Structures and Algorithms Made Easy" by Narasimha Karumanchi
- "Introduction to Algorithms" by Thomas H. Cormen
- "Algorithms Unlocked" by Thomas H. Cormen
Online Courses
- Coursera: Data Structures and Algorithms Specialization
- edX: Data Structures Fundamentals
- Udacity: Data Structures and Algorithms Nanodegree
Tutorials and Guides
- GeeksforGeeks: Comprehensive tutorials on various data structures
- Khan Academy: Interactive lessons on algorithms and data structures
- Codecademy: Hands-on coding exercises for beginners
Understanding data structures is crucial for efficient programming. A binary tree data structure is a hierarchical data structure in which each node has at most two children, referred to as the left child and the right child.
Coding Practice Platforms
- LeetCode: Practice problems on data structures and algorithms
- HackerRank: Coding challenges to improve your skills
- Codewars: Community-driven coding challenges
Research Papers
- Explore recent studies on binary trees and search trees in academic journals
- Look for papers discussing advancements in tree structures and algorithms
Community Forums
- Stack Overflow: Ask questions and share knowledge with other programmers
- Reddit: Join subreddits focused on programming and data structures
- Discord: Engage with communities for real-time discussions
If you’re eager to dive deeper into coding, check out our website for more resources! We offer a variety of interactive tutorials and helpful guides to boost your skills. Don’t wait—start your coding journey today!
Conclusion
In summary, understanding the differences between a binary tree and a binary search tree (BST) is essential for anyone learning about data structures. A binary tree is a general structure where each node can have up to two children, while a binary search tree is a special type of binary tree that keeps its nodes in a specific order. This order allows for quicker searching, adding, and removing of nodes. Knowing these differences helps in choosing the right structure for your coding tasks, making your programs more efficient.
Frequently Asked Questions
What is a binary tree?
A binary tree is a type of data structure where each node can have at most two children, known as the left and right child.
What defines a binary search tree?
A binary search tree is a special kind of binary tree where the left child has a smaller value than the parent, and the right child has a larger value.
How do binary trees and binary search trees differ?
The main difference is that binary trees do not have any specific order for their nodes, while binary search trees are organized in a way that allows for efficient searching.
What are some common terms related to binary trees?
Common terms include root node, leaf node, height, depth, and parent node.
What are the key properties of binary search trees?
In a binary search tree, each left child is smaller than its parent, and each right child is larger, which helps in efficient searching.
Can you name some types of binary trees?
Yes! Types of binary trees include full binary trees, complete binary trees, and perfect binary trees.
What types of binary search trees exist?
There are several types, including AVL trees, red-black trees, and splay trees.
How do you insert a node in a binary tree?
In a binary tree, you can insert a node in any position without following a specific order.
What is the process for inserting a node in a binary search tree?
In a binary search tree, you must place the node in the correct position according to its value, maintaining the order.
Why is balancing important in binary search trees?
Balancing helps to keep the tree efficient for searching, inserting, and deleting operations.
What are some common mistakes people make with binary trees?
Common mistakes include improper insertion, deletion errors, and failing to maintain the tree’s balance.
Where can I learn more about binary trees and binary search trees?
You can find resources like coding tutorials, online courses, and books on data structures to learn more.