Top 10 TypeScript Interview Questions You Must Prepare For
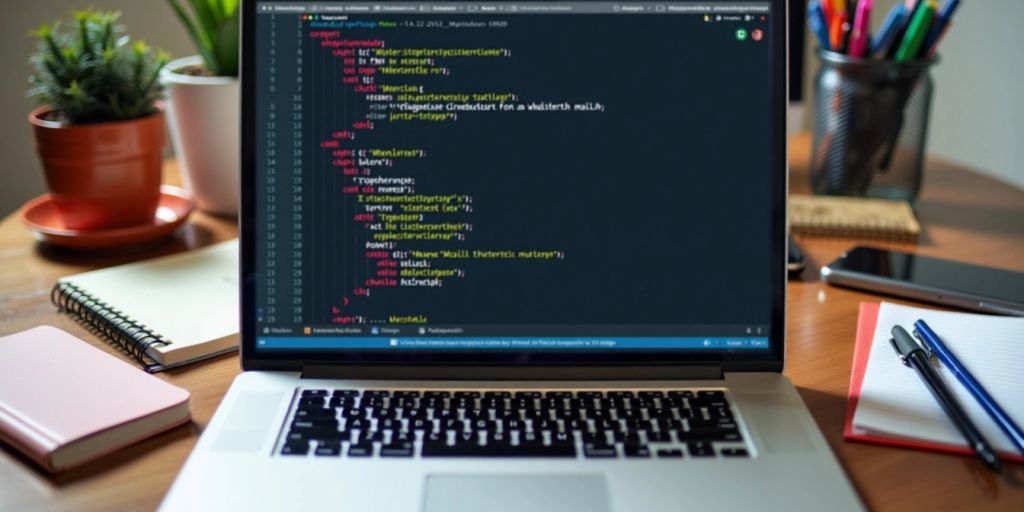
If you’re preparing for a TypeScript interview, it’s essential to understand the key concepts that often come up in discussions. TypeScript is a powerful tool that enhances JavaScript by adding types, making your code safer and easier to read. This article covers the top 10 TypeScript interview questions to help you get ready and boost your confidence.
Key Takeaways
- TypeScript adds types to JavaScript, helping to catch errors early.
- Understanding primitive types is fundamental to using TypeScript effectively.
- Arrays in TypeScript can hold multiple types, making them flexible.
- The ‘any’ type allows you to bypass type checking when necessary.
- Optional properties let you define object fields that may or may not be present.
1. Primitive Types
In TypeScript, there are three main primitive types that you should know: string, number, and boolean. These types are similar to those in JavaScript and are essential for defining variables.
Types Overview
Type | Description |
---|---|
string | Represents text values like "hello" or "TypeScript". |
number | Represents numeric values such as 1, 2, or 3. |
boolean | Represents a value that can be either true or false. |
Key Points
- String: Used for text. For example,
let name: string = "Alice";
- Number: Used for numbers. For example,
let age: number = 30;
- Boolean: Used for true/false values. For example,
let isStudent: boolean = true;
Understanding these primitive types is crucial for any TypeScript developer. They form the foundation of how you work with data in your applications.
When preparing for your interview, remember that these types are the building blocks of TypeScript. Make sure you can explain how to use them effectively!
2. Arrays
In TypeScript, arrays are used to store multiple values of the same type. They are ordered collections, meaning each item has a specific position, starting from index 0. For example, if you have an array of numbers, the first number is at index 0, the second at index 1, and so on.
Declaring Arrays
You can declare an array in TypeScript in a couple of ways:
- Using the square brackets:
let numbers: number[] = [10, 20, 30];
- Using the
Array
type:let numbers: Array<number> = [10, 20, 30];
Accessing Array Elements
To access elements in an array, you use their index. For example:
numbers[0]
gives you10
numbers[1]
gives you20
Important Points
- Arrays can hold any type of data, but it’s best to keep them consistent.
- You can add or remove items from an array easily.
Arrays in TypeScript are different from JavaScript. They have additional features that help manage data better.
Example of Using Arrays
Here’s a simple example of how to use arrays in TypeScript:
let fruits: string[] = ["apple", "banana", "cherry"];
console.log(fruits[1]); // Output: banana
Understanding how to work with arrays is crucial for TypeScript programming, especially when handling collections of data.
3. Any Type
In TypeScript, the any type is a special type that allows you to store values of any kind. This is useful when you don’t know the type of a value ahead of time, such as when data comes from an API or user input. For example:
let person: any = "Foo";
When to Use Any Type
- Flexibility: Use it when you need to handle different types of data without strict rules.
- Temporary Solution: It can be a quick fix while developing, but it’s better to replace it with specific types later.
Example of Any Type
Here’s a simple example:
const employeeData: string = `{"name": "John Doe", "salary": 60000}`;
const employee: any = JSON.parse(employeeData);
console.log(employee.name);
console.log(employee.salary);
Important Note
Using the any type can lead to runtime errors because it skips type-checking. It’s best to use it sparingly to keep your code safe and reliable.
Remember, while the any type offers flexibility, it can also make your code less safe. Always try to use more specific types when possible.
Summary
The any type is a powerful feature in TypeScript, but it should be used wisely. It allows for flexibility but can introduce risks if overused. Always aim for type safety to avoid potential issues in your code.
4. Void Type
In TypeScript, the void type is used to indicate that a function does not return a value. This is particularly useful for functions that perform actions but do not need to send any data back to the caller. For example:
function notify(): void {
alert("The user has been notified.");
}
When a function is declared with a return type of void, it means that it can only return null
or undefined
. Here’s a simple breakdown of the void type:
- Purpose: To signify that a function does not return a value.
- Usage: Commonly used in event handlers or functions that perform side effects.
Key Points:
- A void function can’t return any value other than
null
orundefined
. - It is the opposite of the
any
type, which can hold any value.
The void type is essential for functions that are meant to perform actions without needing to return data.
Example:
Here’s a quick example of a void function:
function logMessage(message: string): void {
console.log(message);
}
In this example, logMessage
takes a string and logs it to the console, but it does not return any value. Understanding the void type is crucial for TypeScript interview questions as it helps clarify how functions operate in TypeScript.
5. Unknown Type
The unknown type in TypeScript is a safer alternative to the any type. It allows you to assign any value to it, but you cannot use that value until you check its type. This means you must perform some type-checking before using it, making your code safer.
Why Use Unknown?
- Type Safety: Unlike any, unknown requires you to check the type before using it.
- Flexibility: You can still assign any value to it, but you must ensure it’s the right type before using it.
Example of Unknown Type
Here’s a simple example:
let input: unknown = "Hello, TypeScript!";
if (typeof input === "string") {
console.log(input.toUpperCase()); // Safe to use as a string
}
Comparison with Any Type
Feature | Any Type | Unknown Type |
---|---|---|
Type Checking | No type checking required | Requires type checking |
Assignability | Can assign any type | Can assign any type, but must check before use |
Safety | Less safe | More safe |
Using the unknown type helps you write more reliable code by enforcing type checks, especially when dealing with user input or data from APIs. It’s a great way to ensure that your code behaves as expected without unexpected errors.
6. Variable Declarations
In TypeScript, variables are essential for storing data. You can create variables using three main keywords: var
, let
, and const
. Each has its own rules and uses.
1. Using var
- Declares a variable that can be accessed globally or within a function.
- Example:
var name = "Alice";
2. Using let
- Creates a variable that is limited to the block it is defined in.
- Example:
let age = 30; if (true) { let age = 25; // This age is different } console.log(age); // Outputs: 30
3. Using const
- Declares a constant variable that cannot be changed after it is set.
- Example:
const pi = 3.14;
Summary of Variable Declarations
Keyword | Scope | Reassignable | Example |
---|---|---|---|
var | Function/Global | Yes | var x = 10; |
let | Block | Yes | let y = 20; |
const | Block | No | const z = 30; |
Remember, using let and const is generally preferred over var to avoid unexpected behavior due to scoping issues.
Understanding how to declare variables correctly is crucial for writing effective TypeScript code. It helps in managing data and ensuring that your code runs smoothly.
7. Arrow Function Syntax
Arrow functions in TypeScript provide a concise way to define functions. They are also known as lambdas in other programming languages. Here’s a simple breakdown of how they work:
Key Features:
- Shorter Syntax: Arrow functions are shorter and easier to read compared to traditional function expressions.
- Lexical
this
: They inherit thethis
value from their surrounding context, which is helpful when using callbacks.
Example:
Here’s how you can define a function that adds two numbers:
let add = (x: number, y: number): number => x + y;
This is much simpler than the traditional way:
function add(x: number, y: number): number {
return x + y;
}
Usage in Arrays:
Arrow functions are often used with array methods. For example, to filter an array for multiples of five:
let numbers = [3, 5, 9, 15, 34, 35];
let fiveMultiples = numbers.filter(num => (num % 5) === 0);
console.log(fiveMultiples); // Output: [5, 15, 35]
Arrow functions make your code cleaner and easier to understand. They are a great tool for modern TypeScript programming!
8. Function Type Annotations
In TypeScript, function type annotations help you specify what types of values a function can accept and return. This makes your code clearer and safer. Here’s how you can define a function with type annotations:
function greet(name: string): string {
return `Hello, ${name}`;
}
In this example, the function greet
takes a parameter name
of type string
and returns a value of type string
. This means you can only pass a string to this function.
Benefits of Function Type Annotations
- Clarity: It’s clear what types are expected.
- Safety: Helps catch errors during development.
- Documentation: Acts as a form of documentation for other developers.
Example of Function Type Annotations
Here’s another example:
function add(a: number, b: number): number {
return a + b;
}
This function add
takes two numbers and returns their sum, which is also a number.
Summary
Using function type annotations is a great way to enhance your TypeScript code. They allow you to define the expected return type of a function, enhancing code clarity and type safety.
Type annotations are essential for writing robust TypeScript code. They help prevent bugs and make your code easier to understand.
9. Object Creation
Creating objects in TypeScript is straightforward and can be done in several ways. Understanding how to create objects is essential for effective programming. Here are some common methods:
1. Using Object Literals
You can create an object using curly braces. For example:
let person = {
name: "John",
age: 30
};
2. Using Classes
Classes serve as blueprints for creating objects. Here’s a simple example:
class Car {
make: string;
model: string;
constructor(make: string, model: string) {
this.make = make;
this.model = model;
}
}
let myCar = new Car("Toyota", "Corolla");
3. Using Interfaces
Interfaces define the structure of an object. Here’s how you can use them:
interface Animal {
species: string;
sound: string;
}
let dog: Animal = {
species: "Dog",
sound: "Bark"
};
4. Using the new
Keyword
You can also create objects using the new
keyword with a constructor function:
function Person(name: string, age: number) {
this.name = name;
this.age = age;
}
let person1 = new Person("Alice", 25);
Summary Table
Method | Description |
---|---|
Object Literals | Simple and quick object creation. |
Classes | Use for structured object creation. |
Interfaces | Define object structure. |
Constructor | Create objects with custom logic. |
Object creation is a fundamental skill in TypeScript that helps you build complex applications efficiently.
By mastering these methods, you can effectively manage and utilize objects in your TypeScript projects. Remember, practice makes perfect!
10. Optional Properties
In TypeScript, optional properties allow you to define properties in an object that may or may not be present. This is useful when you want to create flexible data structures. You can indicate an optional property by adding a question mark (?) after the property name.
Example of Optional Properties
Here’s a simple example:
interface User {
name: string;
age?: number; // age is optional
}
const user1: User = { name: "Alice" }; // valid
const user2: User = { name: "Bob", age: 30 }; // also valid
Benefits of Using Optional Properties
- Flexibility: You can create objects without needing to specify every property.
- Clarity: It makes it clear which properties are required and which are not.
Important Points
- Optional properties can be used in both interfaces and type aliases.
- When accessing optional properties, you should check if they exist to avoid errors.
Optional properties help in creating more adaptable and clear data structures, making your code easier to manage.
Summary
In summary, optional properties in TypeScript are a powerful feature that allows developers to create more flexible and understandable code. By using the question mark (?) syntax, you can easily define which properties are required and which are optional, enhancing the overall structure of your applications.
In this section, we explore optional properties that can enhance your coding experience. If you’re eager to improve your skills and land that dream job, visit our website today! We offer free coding lessons to get you started on your journey.
Conclusion
Preparing for a TypeScript interview can feel overwhelming, but it doesn’t have to be. By focusing on the key questions we’ve discussed, you can build a strong foundation in TypeScript. Remember, understanding the basics and practicing coding problems will help you feel more confident. Whether you’re just starting out or have some experience, these questions will guide you in the right direction. Good luck, and don’t forget to keep learning and practicing!
Frequently Asked Questions
What are the basic types in TypeScript?
TypeScript has several basic types like ‘number’ for numbers, ‘string’ for text, and ‘boolean’ for true or false values.
How do arrays work in TypeScript?
In TypeScript, arrays are used to store multiple values in a single variable. You can create an array of any type, like numbers or strings.
What does ‘any’ type mean?
The ‘any’ type in TypeScript means that a variable can hold any kind of value. It’s useful when you are not sure what type you will have.
What is the void type?
The void type is used for functions that do not return any value. It tells us that the function doesn’t give back anything.
What is an unknown type?
The unknown type is a safer version of ‘any’. It means you don’t know what type it is yet, but you must check it before using it.
How can I declare variables in TypeScript?
You can declare variables using ‘let’, ‘const’, or ‘var’. ‘let’ and ‘const’ are the most common in modern TypeScript.
What is arrow function syntax?
Arrow functions are a shorter way to write functions in TypeScript. They use the ‘=>’ symbol and are great for keeping code clean.
How do I create objects in TypeScript?
You can create objects using curly braces {}. You define properties and their types inside the object.