Mastering Backend Development: A Comprehensive Guide to Tutorials
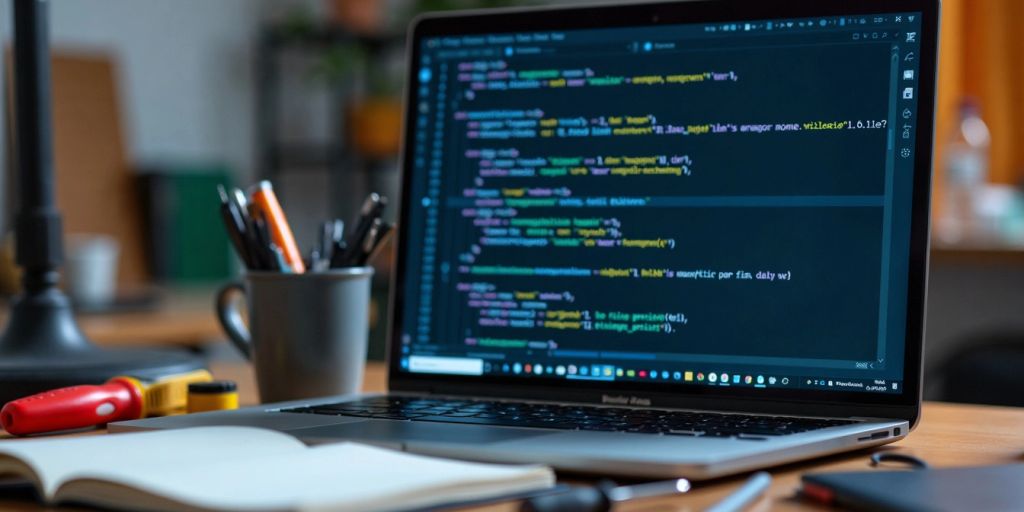
Backend development is essential for creating the services and logic behind web applications. This guide will help you learn the basics and advanced skills needed to become a successful backend developer. You’ll discover important concepts, programming languages, databases, and much more. Let’s dive into the world of backend development!
Key Takeaways
- Backend developers create the server-side logic of applications.
- Understanding programming languages like Python and JavaScript is crucial.
- Databases are essential for storing and managing data.
- APIs allow different software systems to communicate with each other.
- Learning security best practices helps protect applications from threats.
Understanding the Fundamentals of Backend Development
Backend development is crucial for creating web applications. It involves the server-side logic that users don’t see but is essential for functionality. Backend developers design and manage databases, create APIs, and ensure the application runs smoothly.
The Role of a Backend Developer
A backend developer’s job includes:
- Writing server-side code
- Managing databases
- Creating APIs for communication
- Ensuring security and performance
Core Concepts in Backend Development
To excel in backend development, you should understand:
- Client-Server Architecture: How clients and servers communicate.
- Databases: Storing and retrieving data efficiently.
- APIs: Allowing different software systems to interact.
Essential Tools and Technologies
Here are some key tools and technologies:
Tool/Technology | Purpose |
---|---|
Node.js | JavaScript runtime for server-side code |
Express.js | Web framework for building APIs |
SQL | Language for managing databases |
Git | Version control for code management |
Understanding these fundamentals is the first step to becoming a successful backend developer. With the right knowledge, you can build powerful applications that meet user needs.
In this guide, you will learn about key technologies, frameworks, and best practices for building web applications with our in-depth guide on backend development.
Mastering Server-Side Programming Languages
Backend development relies heavily on server-side programming languages. These languages are essential for creating the logic that powers web applications. Here are some key points to consider:
Popular Backend Languages
- JavaScript: Gaining popularity with Node.js, it allows for asynchronous programming, making it great for scalable applications.
- Python: Known for its simplicity, Python is widely used for backend development, especially with frameworks like Django.
- Ruby: Often used with Ruby on Rails, it’s favored for rapid application development.
- PHP: Commonly used for web development, especially in content management systems like WordPress.
- Java: A robust language often used in enterprise-level applications.
Choosing the Right Language
When selecting a language, consider:
- Your goals: What do you want to build?
- Job market: What languages are in demand in your area?
- Learning curve: Some languages are easier for beginners.
Learning Resources for Each Language
Here’s a quick table of resources:
Language | Resource |
---|---|
JavaScript | "Eloquent JavaScript" |
Python | "Learn Python the Hard Way" |
Ruby | "The Odin Project" |
PHP | "PHP: The Right Way" |
Java | "Head First Java" |
Remember: The key is to supplement theoretical learning by applying concepts through hands-on coding projects.
By mastering these languages, you’ll be well on your way to becoming a proficient backend developer. Understanding the fundamentals will help you build a strong foundation for your career in backend development.
Exploring Database Management Systems
Relational vs. Non-Relational Databases
When it comes to databases, there are two main types: relational and non-relational. Relational databases, like MySQL, use tables to organize data. Non-relational databases, such as MongoDB, are more flexible and can handle various data types. Here’s a quick comparison:
Feature | Relational Databases | Non-Relational Databases |
---|---|---|
Structure | Tables | Flexible documents |
Schema | Fixed | Dynamic |
Scalability | Vertical | Horizontal |
Use Cases | Transactional apps | Big data, real-time apps |
Popular Database Systems
Some of the most popular database systems include:
- MySQL: A widely used relational database.
- PostgreSQL: Known for its advanced features and standards compliance.
- MongoDB: A leading non-relational database that stores data in JSON-like documents.
Database Design and Optimization
Good database design is crucial for performance. Here are some tips:
- Normalize your data to reduce redundancy.
- Use indexes to speed up queries.
- Regularly back up your data to prevent loss.
A database management system (DBMS) is a software system that is designed to manage and organize data in a structured manner. This is essential for any application that needs to store and retrieve data efficiently.
By understanding these concepts, you can better manage data in your backend applications and ensure they run smoothly.
Building and Integrating APIs
Understanding RESTful APIs
APIs, or Application Programming Interfaces, are essential for connecting different software applications. The process of connecting two or more software applications or processes using APIs is referred to as API integration. This allows different systems to communicate and share data effectively.
API Development Best Practices
When developing APIs, consider the following steps:
- Choose a programming language and framework. Popular choices include:
- Node.js with Express.js
- Python with Flask or Django
- PHP with Laravel
- Ruby on Rails
- Java with Spring Boot
- Set up your development environment and create a starter project.
- Define the resources and endpoints your API will use. For example, if your API manages users, you might have endpoints like
/users
. - Implement CRUD (Create, Read, Update, Delete) operations for each resource.
- Add security measures, such as input validation and authentication.
- Connect your API to a database, like MongoDB or PostgreSQL.
- Test your API using tools like Postman.
- Document your API for other developers.
Tools for API Testing and Documentation
Using the right tools can make API development smoother. Here are some popular options:
- Postman: For testing API endpoints.
- Swagger: For documenting APIs.
- cURL: For making API requests from the command line.
Building a solid API is crucial for backend development. It allows different applications to work together seamlessly, enhancing user experience and functionality.
Enhancing Security in Backend Development
Common Security Threats
Backend developers face various security threats that can compromise applications. Some of the most common threats include:
- SQL Injection: Attackers can manipulate SQL queries to gain unauthorized access to data.
- Cross-Site Scripting (XSS): Malicious scripts can be injected into web pages viewed by users.
- Data Breaches: Sensitive information can be exposed due to poor security practices.
Implementing Authentication and Authorization
To protect applications, it’s crucial to implement strong authentication and authorization measures. Here are some key practices:
- Use multi-factor authentication to add an extra layer of security.
- Ensure that user roles are clearly defined to limit access to sensitive data.
- Regularly update passwords and encourage users to create strong ones.
Best Practices for Data Protection
Protecting data is essential for maintaining user trust. Here are some best practices:
- Data Encryption: Encrypt sensitive data both in transit and at rest to prevent unauthorized access.
- Regular Backups: Implement a backup strategy to recover data in case of loss or breach.
- Security Audits: Conduct regular audits to identify and fix vulnerabilities.
Security is not just a feature; it’s a fundamental part of backend development.
By following these guidelines, developers can significantly enhance the security of their backend applications and protect against common threats. Website security is vital to ensure that applications remain safe and reliable for users.
Deploying and Scaling Backend Applications
Introduction to Deployment
Deploying a backend application means making it available for users. Choosing the right platform is crucial for a successful deployment. Here are some popular options:
- Cloud Providers: AWS, Google Cloud, Azure
- Platform as a Service (PaaS): Heroku, Vercel
- Containerization: Docker, Kubernetes
Scaling Applications Effectively
Scaling is about handling more users without slowing down. Here are some strategies:
- Load Balancing: Distributing traffic across multiple servers.
- Horizontal Scaling: Adding more machines to handle increased load.
- Vertical Scaling: Upgrading existing machines with more resources.
Strategy | Description |
---|---|
Load Balancing | Distributes traffic to multiple servers |
Horizontal Scaling | Adds more machines to handle increased load |
Vertical Scaling | Upgrades existing machines with more resources |
Monitoring and Maintenance
Once deployed, it’s important to keep an eye on the application. Regular monitoring helps catch issues early. Here are some tools to consider:
- Monitoring Tools: New Relic, Datadog
- Logging Tools: ELK Stack, Splunk
- Alerting Tools: PagerDuty, Opsgenie
Regular maintenance ensures that your application runs smoothly and efficiently. Always be prepared to make updates and improvements as needed.
Conclusion
Deploying and scaling backend applications is a vital part of development. By understanding the right tools and strategies, developers can ensure their applications are robust and ready for users. Effective scaling not only improves performance but also enhances user satisfaction.
Adopting DevOps Practices
DevOps is a software development approach that focuses on collaboration, automation, and continuous delivery. This helps teams create high-quality products efficiently. Here are some key practices to adopt:
Continuous Integration and Continuous Deployment (CI/CD)
- Continuous Integration (CI) involves regularly merging code changes into a central repository. This helps catch issues early.
- Continuous Deployment (CD) automates the release of code to production, ensuring that updates are delivered quickly and reliably.
Infrastructure as Code
- This practice allows developers to manage and provision infrastructure using code. It makes setups reproducible and reduces manual errors.
- Tools like Terraform and Ansible are popular for implementing Infrastructure as Code.
Popular DevOps Tools
Tool | Purpose |
---|---|
Jenkins | Automates CI/CD processes |
Docker | Containerization of applications |
Kubernetes | Orchestration of containerized apps |
Git | Version control for code |
Terraform | Infrastructure management |
Adopting DevOps practices can significantly improve the speed and quality of software delivery. By focusing on collaboration and automation, teams can respond to changes more effectively.
Implementing these practices will not only enhance your backend development skills but also prepare you for the evolving tech landscape.
Engaging in Hands-On Projects
Getting practical experience is crucial for mastering backend development. Working on real projects helps solidify your understanding of concepts. Here are some beginner project ideas to get you started:
Beginner Projects to Try
- Build a simple CMS blog using a framework like Django or Ruby on Rails.
- Create a REST API to connect with a database like MongoDB or MySQL.
- Make a weather application that uses server-side APIs to show data on a front-end site.
- Automate tasks by writing Python scripts to process Excel data or send emails.
- Develop a user login system with encrypted passwords and sessions.
Tips for Project Success
- Start with simple projects and gradually increase complexity.
- Use version control to track changes in your code.
- Document your code thoroughly to make it easier to understand later.
Engaging in hands-on projects not only enhances your skills but also builds your confidence as a developer.
By applying what you learn through these projects, you’ll accelerate your journey in backend development. Remember, practice makes perfect!
Staying Updated with Industry Trends
Following Influential Blogs and Newsletters
To keep up with the fast-paced world of backend development, following influential blogs and newsletters is essential. Here are some popular sources:
- Daily.dev: A platform that curates the latest developer news.
- Smashing Magazine: Offers articles on web development and design.
- Dev.to: A community of developers sharing insights and tutorials.
Participating in Developer Communities
Engaging with other developers can provide valuable insights and support. Consider:
- Joining forums like Stack Overflow.
- Participating in local meetups or online groups.
- Contributing to open-source projects to learn from others.
Attending Conferences and Workshops
Conferences and workshops are great for networking and learning about the latest trends. Some notable events include:
- Google I/O: Focuses on Google technologies.
- AWS re:Invent: Covers cloud computing and services.
- PyCon: A conference for Python enthusiasts.
Staying updated is not just about reading; it’s about engaging with the community and sharing knowledge. Embrace the journey of continuous learning to thrive in backend development.
Conclusion
By following these steps, you can stay informed about the latest trends and technologies in backend development. Remember, the field is always changing, and being proactive will help you succeed!
Contributing to Open Source
Contributing to open source is a fantastic way to improve your skills as a developer while also building your portfolio. Starting small is key to making a positive impact. Here are some important points to consider:
Benefits of Open Source Contribution
- Skill Development: You can learn new technologies and improve your coding skills.
- Networking: Meet other developers and build connections in the tech community.
- Portfolio Building: Showcase your contributions to potential employers.
Finding Projects to Contribute To
Here are some easy ways to find open source projects:
- Identify your interests.
- Explore open source platforms.
- Join open source communities.
- Check GitHub for projects needing help.
Best Practices for Contributing
- Start Small: Begin with fixing bugs or improving documentation.
- Communicate Clearly: Discuss your changes with project maintainers.
- Test Your Code: Make sure everything works before submitting.
Contributing to open source can be a rewarding experience. It allows you to learn, grow, and connect with others in the field.
Highlighting Your Contributions
Make sure to include your open source work in your portfolio. This can show your ability to collaborate and adapt to different codebases.
By following these steps, you can effectively contribute to open source projects and enhance your skills as a backend developer.
Preparing for Backend Development Interviews
Common Interview Questions
When preparing for a backend developer interview, it’s important to know the key topics that are often covered. Here are some common areas to focus on:
- Backend programming languages: Understand the languages you will be using, such as Python, Java, or Node.js.
- APIs: Be ready to discuss how APIs work and how to create them.
- Data structures: Know the different types of data structures and when to use them.
- Servers: Understand how servers operate and how to manage them.
- Algorithms: Be prepared to solve problems using algorithms.
Technical Assessment Tips
To excel in your technical assessment, consider these tips:
- Practice coding: Use platforms like LeetCode or HackerRank to sharpen your skills.
- Understand the fundamentals: Make sure you have a solid grasp of core concepts.
- Mock interviews: Conduct mock interviews with friends or use online services.
- Review past projects: Be ready to discuss your previous work and the technologies you used.
Building a Strong Portfolio
A strong portfolio can set you apart from other candidates. Here’s how to build one:
- Include projects: Showcase your best work, especially those that demonstrate your backend skills.
- Explain your role: Clearly describe what you did in each project.
- Highlight technologies: Mention the tools and languages you used.
- Keep it updated: Regularly add new projects and experiences.
Preparing for interviews can be stressful, but with the right preparation, you can boost your confidence and improve your chances of success. Focus on the key areas and practice regularly to feel ready for any question that comes your way!
Getting ready for backend development interviews can be tough, but it doesn’t have to be! Start your journey with us at AlgoCademy, where we offer interactive coding tutorials and resources to help you ace your interviews. Don’t wait—visit our website and start coding for free today!
Conclusion: Starting Your Backend Development Adventure
Learning backend development takes time and effort, but it’s worth it. By following a clear plan that focuses on the basics and practicing what you learn, you can build the skills you need to succeed. Remember, the best way to learn is by doing. Start with small projects and gradually take on bigger challenges. Stay curious, ask questions, and keep improving your skills. With dedication, you can become a skilled backend developer ready to tackle real-world problems.
Frequently Asked Questions
What is backend development?
Backend development is the part of web development that deals with the server side. It involves creating the logic, managing databases, and ensuring everything works smoothly behind the scenes.
What programming languages should I learn for backend development?
Some popular languages for backend development include Python, JavaScript (especially with Node.js), Ruby, and PHP. You can choose one to start with based on your interests.
How do I start learning backend development?
Begin with the basics of programming, understand how servers work, and then gradually move on to building simple projects like APIs or databases.
What tools do I need for backend development?
You’ll need a good code editor, a version control system like Git, and tools for managing databases, such as MySQL or MongoDB.
What is an API and why is it important?
An API (Application Programming Interface) allows different software applications to communicate with each other. It’s important because it enables integration between different systems.
How can I make my backend applications secure?
To secure your applications, implement authentication and authorization, keep software updated, and regularly check for vulnerabilities.
What are some common challenges in backend development?
Common challenges include managing databases, ensuring security, optimizing performance, and debugging issues that arise.
How do I prepare for a backend developer interview?
Study common interview questions, practice coding problems, and build a portfolio of projects to showcase your skills.