A Comprehensive Sorting Algorithms Guide: Mastering the Art of Data Organization
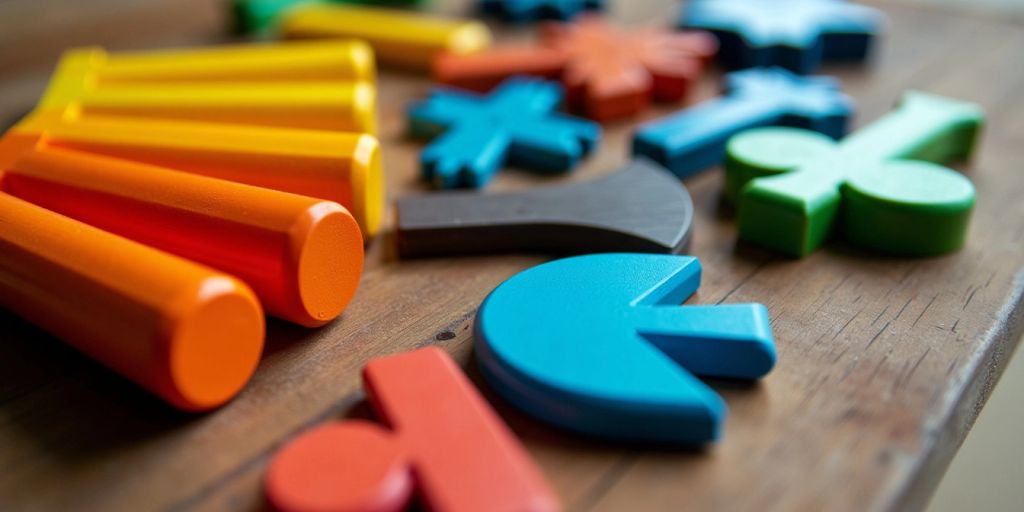
Sorting algorithms play a vital role in organizing data efficiently. They help us arrange lists or arrays in a specific order, making it easier to search and analyze information. From simple methods like Bubble Sort to more advanced techniques like Quick Sort, understanding these algorithms is crucial for anyone interested in programming and data science. In this guide, we’ll explore various sorting algorithms, their benefits, and when to use each one.
Key Takeaways
- Sorting algorithms help organize data, making it easier to work with.
- Different algorithms have unique strengths and weaknesses.
- Some algorithms are better for small datasets, while others excel with large ones.
- Understanding time complexity can guide you in choosing the right algorithm.
- Mastering sorting techniques is essential for effective programming.
Understanding the Basics of Sorting Algorithms
What Are Sorting Algorithms?
Sorting algorithms are essential methods in computer science that help to arrange elements in a list or array in a specific order. This order can be numerical or alphabetical, depending on the data type. A sorting algorithm is used to rearrange a given array or list of elements according to a comparison operator on the elements. Efficient sorting can significantly improve system performance, especially when handling large amounts of data.
Importance of Sorting in Data Organization
Sorting is crucial for several reasons:
- Easier Searching: When data is sorted, it becomes much simpler to find specific items.
- Better Analysis: Sorted data can reveal trends and patterns that might be missed in unsorted data.
- Improved Display: Presenting data in a sorted manner enhances readability and user experience.
Common Use Cases for Sorting Algorithms
Sorting algorithms are used in various real-world applications, including:
- Database Management: Organizing records for quick access.
- Search Engines: Ranking search results based on relevance.
- E-commerce: Sorting products by price, popularity, or ratings.
- Data Analysis: Preparing datasets for statistical analysis.
Understanding sorting algorithms is not just about sorting; it’s about learning how to solve problems efficiently.
In summary, sorting algorithms are foundational tools in data organization, making them vital for anyone working with data.
Bubble Sort: The Simplest Sorting Algorithm
How Bubble Sort Works
Bubble Sort is a straightforward sorting method. It works by repeatedly checking adjacent elements in a list and swapping them if they are in the wrong order. This process continues until the entire list is sorted. The bubble sort algorithm is easy to understand, making it a great choice for beginners.
Advantages and Disadvantages of Bubble Sort
Advantages:
- Simple to implement and understand.
- No additional memory is required, making it space-efficient.
Disadvantages:
- Not suitable for large datasets due to its inefficiency.
- The average and worst-case time complexity is O(n²), which can be slow.
When to Use Bubble Sort
Bubble Sort is best used in scenarios where:
- The dataset is small.
- The list is already partially sorted.
- You need a simple algorithm for educational purposes.
Bubble Sort is a great starting point for learning about sorting algorithms, but it’s not the best choice for performance in larger datasets.
Feature | Bubble Sort |
---|---|
Time Complexity (Best) | O(n) |
Time Complexity (Avg) | O(n²) |
Time Complexity (Worst) | O(n²) |
Space Complexity | O(1) |
Selection Sort: An Intuitive Approach
How Selection Sort Works
Selection sort is a straightforward sorting method. It works by repeatedly finding the smallest element from the unsorted part of the list and moving it to the beginning. Here’s how it goes:
- Start with the first element as the minimum.
- Compare it with the other elements to find the smallest one.
- Swap the smallest found element with the first element.
- Move to the next position and repeat until the list is sorted.
Advantages and Disadvantages of Selection Sort
Advantages:
- Easy to understand and implement.
- Performs well on small lists.
Disadvantages:
- Not efficient for large datasets.
- Has a time complexity of O(n²), making it slower than other algorithms for bigger lists.
When to Use Selection Sort
Selection sort is best used when:
- The dataset is small.
- Simplicity is more important than speed.
- Memory usage needs to be minimal, as it sorts in place without requiring additional storage.
Selection sort is a simple and efficient sorting algorithm that works by repeatedly selecting the smallest element from the unsorted portion of the list.
In summary, while selection sort may not be the fastest option, its intuitive approach makes it a great choice for beginners learning about sorting algorithms.
Insertion Sort: Efficient for Small Datasets
How Insertion Sort Works
Insertion Sort is a straightforward sorting method that builds a sorted list one item at a time. It works by taking an element from the unsorted part of the list and placing it in the correct position within the sorted section. This algorithm is particularly effective for small datasets.
Advantages and Disadvantages of Insertion Sort
Advantages:
- Simple to understand and implement.
- Efficient for small datasets or nearly sorted lists.
- Stable, meaning it maintains the relative order of equal elements.
Disadvantages:
- Not suitable for large datasets due to its O(n²) time complexity.
- Performance decreases significantly as the number of elements increases.
When to Use Insertion Sort
- When dealing with small datasets.
- When the data is already partially sorted.
- In situations where simplicity and ease of implementation are more important than speed.
Insertion Sort is a great choice for beginners learning about sorting algorithms, as it introduces fundamental concepts in a clear and manageable way.
Dataset Size | Time Complexity |
---|---|
Small | O(n) |
Medium | O(n²) |
Large | O(n²) |
Merge Sort: A Divide and Conquer Strategy
How Merge Sort Works
Merge Sort is a sorting method that uses a divide and conquer strategy. It works by breaking down the input array into smaller parts until each part has only one element. Then, it combines these parts back together in a sorted order. This process can be summarized in three main steps:
- Divide the array into two halves.
- Recursively sort each half.
- Merge the sorted halves back together.
Advantages and Disadvantages of Merge Sort
Advantages:
- Efficient for large datasets with a time complexity of O(n log n).
- It is a stable sort, meaning it keeps the order of equal elements.
Disadvantages:
- Requires additional space for temporary arrays, which can be a drawback for large datasets.
- Slower than some other algorithms like Quick Sort for smaller datasets.
When to Use Merge Sort
Merge Sort is best used when:
- You have a large dataset that needs sorting.
- Stability is important, such as when sorting records with multiple fields.
- You are working with linked lists, as it can be more efficient than other algorithms in this case.
Merge Sort is a powerful tool for organizing data, especially when dealing with large amounts of information. Its ability to efficiently sort while maintaining stability makes it a popular choice among programmers.
Aspect | Merge Sort |
---|---|
Time Complexity | O(n log n) |
Space Complexity | O(n) |
Stability | Yes |
Best Use Case | Large datasets |
Quick Sort: The Fastest Sorting Algorithm
How Quick Sort Works
Quick Sort is a popular sorting method that uses a technique called divide and conquer. It starts by selecting a special element known as the pivot. The array is then divided into two parts: elements less than the pivot and elements greater than the pivot. This process is repeated for each part until the entire array is sorted.
Advantages and Disadvantages of Quick Sort
Advantages:
- Speed: Quick Sort is generally faster than many other sorting algorithms, especially for large datasets.
- Efficiency: It has an average time complexity of O(n log n), making it suitable for various applications.
Disadvantages:
- Unstable: Quick Sort can change the order of equal elements, which may not be desirable in some cases.
- Worst-case performance: In rare situations, its performance can drop to O(n²), especially if the pivot is poorly chosen.
When to Use Quick Sort
Quick Sort is best used when:
- You have a large dataset to sort.
- Speed is a priority.
- You do not need to maintain the order of equal elements.
Quick Sort is a favorite among programmers due to its speed and efficiency. It’s a great choice for many sorting tasks!
Heap Sort: Efficient Memory Management
How Heap Sort Works
Heap Sort is a comparison-based sorting algorithm that uses a binary heap data structure. It starts by building a max-heap from the input data. In a max-heap, each parent node is greater than or equal to its child nodes. Once the heap is built, the largest element (the root) is repeatedly removed and placed at the end of the list. The remaining elements are then re-heapified until the entire list is sorted.
Advantages and Disadvantages of Heap Sort
Advantages:
- Efficient Memory Management: Heap Sort does not require additional storage for sorting, making it memory efficient.
- Time Complexity: It has a time complexity of O(n log n), which is suitable for large datasets.
Disadvantages:
- Not Stable: Heap Sort does not maintain the relative order of equal elements.
- Complex Implementation: Compared to simpler algorithms like Bubble Sort, Heap Sort can be more complex to implement.
When to Use Heap Sort
Heap Sort is ideal when you need to sort large datasets and want to manage memory efficiently. It is also useful in scenarios where stability is not a concern, such as in priority queues.
Feature | Heap Sort | Bubble Sort |
---|---|---|
Time Complexity | O(n log n) | O(n^2) |
Space Complexity | O(1) | O(1) |
Stability | No | Yes |
Best Use Case | Large datasets | Small datasets |
Counting Sort and Radix Sort: Specialized Techniques
How Counting Sort Works
Counting Sort is a unique algorithm that sorts elements by counting the number of occurrences of each unique value. It works best when the range of input values is not significantly larger than the number of elements to be sorted. Here’s a simple breakdown of how it operates:
- Count the occurrences of each unique element in the input array.
- Calculate the cumulative count to determine the position of each element in the sorted array.
- Place each element in its correct position in the output array based on the cumulative counts.
This method is particularly effective for sorting integers or small ranges of numbers.
How Radix Sort Works
Radix Sort is another specialized sorting technique that sorts numbers by processing their digits. It sorts the input numbers digit by digit, starting from the least significant digit to the most significant. Here’s how it works:
- Group the numbers based on the least significant digit.
- Sort these groups using a stable sorting algorithm (like Counting Sort).
- Repeat the process for each digit until all digits have been processed.
Radix Sort is efficient for sorting integers or strings and can handle large datasets effectively.
When to Use Counting and Radix Sort
- Counting Sort is ideal when:
- Radix Sort is suitable when:
Both Counting Sort and Radix Sort are powerful tools in the sorting arsenal, especially when dealing with specific types of data. Understanding when to use these algorithms can greatly enhance your data organization skills.
Understanding Time Complexity in Sorting Algorithms
Big O Notation and Its Importance
Time complexity is a way to describe how the time to complete an algorithm changes as the size of the input increases. Big O notation helps us understand the worst-case scenario for an algorithm’s performance. It gives us a way to compare different algorithms based on how quickly they run as the input size grows.
Time Complexity of Common Sorting Algorithms
Here’s a quick look at the time complexities of some popular sorting algorithms:
Algorithm | Best Case | Average Case | Worst Case |
---|---|---|---|
Bubble Sort | O(n) | O(n²) | O(n²) |
Selection Sort | O(n²) | O(n²) | O(n²) |
Insertion Sort | O(n) | O(n²) | O(n²) |
Merge Sort | O(n log n) | O(n log n) | O(n log n) |
Quick Sort | O(n log n) | O(n log n) | O(n²) |
Heap Sort | O(n log n) | O(n log n) | O(n log n) |
Counting Sort | O(n + k) | O(n + k) | O(n + k) |
Radix Sort | O(nk) | O(nk) | O(nk) |
Choosing the Right Algorithm Based on Time Complexity
When selecting a sorting algorithm, consider the size of your data and the specific needs of your application. For example:
- Use Bubble Sort for small datasets or educational purposes.
- Choose Merge Sort for larger datasets where stability is important.
- Opt for Quick Sort when speed is crucial, but be aware of its worst-case performance.
Understanding time complexity is essential for making informed decisions about which sorting algorithm to use. The time complexity of an algorithm is not equal to the actual time required to execute a particular code, but the number of times a statement executes.
By grasping these concepts, you can better navigate the world of sorting algorithms and choose the right one for your needs.
Practical Applications of Sorting Algorithms
Sorting algorithms play a crucial role in many real-life applications. They help organize data efficiently, making it easier to access and analyze. Here are some key areas where sorting algorithms are commonly used:
Sorting in Real-World Applications
- Search Engines: Sorting algorithms help rank search results based on relevance.
- E-commerce: Online stores use sorting to display products by price, popularity, or ratings.
- Data Analysis: Analysts sort data to find trends and insights quickly.
Optimizing Sorting for Performance
To ensure efficient data handling, different sorting algorithms are chosen based on the situation. Here’s a quick comparison of some common algorithms:
Algorithm | Best Case | Average Case | Worst Case |
---|---|---|---|
Bubble Sort | O(n) | O(n²) | O(n²) |
Quick Sort | O(n log n) | O(n log n) | O(n²) |
Merge Sort | O(n log n) | O(n log n) | O(n log n) |
Insertion Sort | O(n) | O(n²) | O(n²) |
Case Studies of Sorting Algorithms in Action
- Database Management: Backend databases often use Merge Sort for efficient data retrieval.
- Gaming: Sorting algorithms can be used to arrange player scores or levels.
- Card Games: When playing cards with friends, Insertion Sort can help organize your hand quickly.
Sorting algorithms are essential for organizing data effectively, making them invaluable in various fields. Understanding their applications can greatly enhance your problem-solving skills in programming.
Sorting algorithms are not just theoretical concepts; they have real-world uses that can make a big difference. From organizing data in apps to optimizing search results, these algorithms help improve efficiency in many areas. If you want to dive deeper into coding and learn how to apply these skills, visit our website and start coding for free today!
Conclusion
Sorting algorithms are key to organizing data in today’s digital world. Knowing how to pick the right sorting method for your needs can make a big difference in your programming or data science work. Whether you’re just starting out or have been coding for a while, understanding these algorithms is essential. It’s like learning the basics of reading and writing in computer science, opening up many opportunities for solving problems. So, jump in, try different methods, and keep learning! Happy sorting!
Frequently Asked Questions
What are sorting algorithms?
Sorting algorithms are methods used in computer science to arrange data in a specific order, like numbers from smallest to largest.
Why is sorting important?
Sorting helps organize data, making it easier to find, analyze, and use. It’s essential in many applications, like searching for information.
What is Bubble Sort?
Bubble Sort is a simple sorting method that compares adjacent items and swaps them if they are in the wrong order.
When should I use Selection Sort?
Selection Sort is good for small lists where simplicity is more important than speed.
How does Insertion Sort work?
Insertion Sort builds a sorted list one item at a time by taking each new item and placing it in the correct position.
What is Merge Sort?
Merge Sort is an efficient algorithm that splits a list into smaller parts, sorts them, and then merges them back together.
Why is Quick Sort so popular?
Quick Sort is fast and efficient, making it a favorite for sorting large datasets.
What is time complexity in sorting?
Time complexity refers to how the time to sort data increases with the size of the data. It helps choose the right algorithm for the task.