What is Programming: A Comprehensive Guide for Beginners
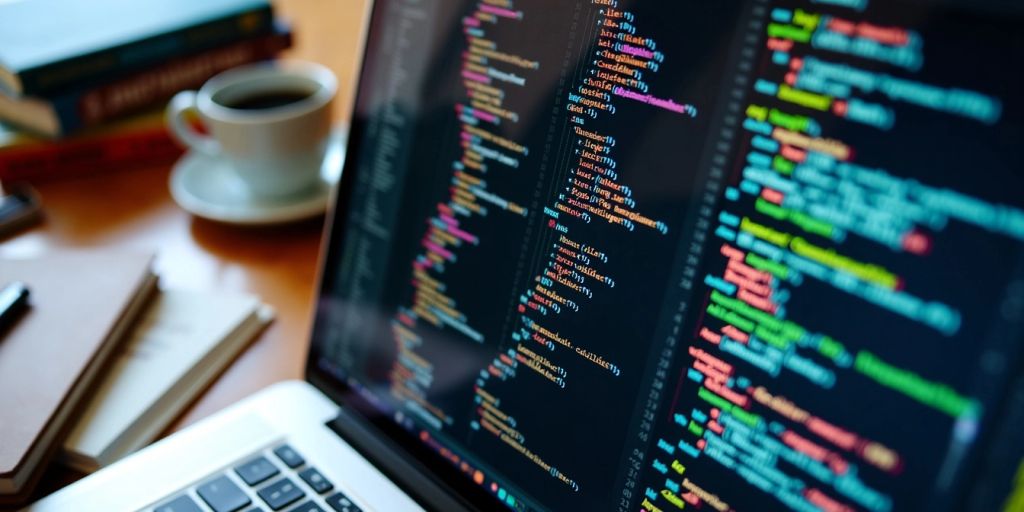
Programming is an essential skill in today’s tech-driven world. It involves writing instructions that tell computers how to perform specific tasks. This guide is designed to help beginners understand the basics of programming, its importance, and how to get started on their coding journey.
Key Takeaways
- Programming is about creating instructions for computers to follow.
- Learning programming can open doors to many career opportunities.
- Choosing the right programming language is crucial for beginners.
- Practice and patience are key to becoming a successful programmer.
- Joining a coding community can provide support and resources.
Understanding the Basics of Programming
What is Programming?
Programming is the process of creating instructions that tell a computer what to do. At its core, programming refers to a technological process for telling a computer which tasks to perform in order to solve problems. When you write a program, you are giving the computer a set of commands to follow. This means breaking down a big problem into smaller, manageable parts that the computer can understand and solve.
The Importance of Learning Programming
Learning programming is essential in today’s digital world. Here are some reasons why:
- Career Opportunities: Many jobs require programming skills.
- Problem-Solving Skills: It helps you think logically and solve problems.
- Creativity: You can create apps, websites, and games.
Common Misconceptions About Programming
Many people have misunderstandings about programming. Here are a few:
- You need to be a math genius: While math can help, it’s not always necessary.
- Programming is only for techies: Anyone can learn to code with practice.
- It’s too hard to learn: With the right resources, anyone can start coding.
Programming is not just about writing code; it’s about thinking differently and solving problems creatively.
Getting Started with Programming
Choosing Your First Programming Language
To begin your programming journey, selecting the right language is crucial. Here are some popular choices:
- Python: Great for beginners due to its simple syntax.
- JavaScript: Essential for web development.
- Java: Known for its versatility and use in large systems.
- C++: Good for understanding low-level programming.
Setting Up Your Development Environment
Once you’ve chosen a language, you need to set up your development environment. Follow these steps:
- Install an IDE: Integrated Development Environments like Visual Studio Code or PyCharm are user-friendly.
- Download the Language: Make sure to install the necessary software for your chosen language.
- Test Your Setup: Write a simple program to ensure everything is working.
Essential Tools for Beginners
Here are some tools that can help you as you start coding:
- Text Editors: Notepad++, Sublime Text, or Atom for writing code.
- Version Control: Git helps you manage changes to your code.
- Online Resources: Websites like Codecademy and freeCodeCamp offer interactive lessons.
Remember: Learning programming is a journey. Stay patient and keep practicing!
Core Concepts in Programming
Variables and Data Types
In programming, variables are like containers that hold information. For example, if you have a variable named x
, you might store the number 10
in it. This allows you to use x
later in your code without having to remember the number itself.
Here are some common data types you might encounter:
- Integer: Whole numbers (e.g., 1, 2, 3)
- Float: Decimal numbers (e.g., 1.5, 2.3)
- String: Text (e.g., "Hello, World!")
- Boolean: True or false values
Control Structures
Control structures help you decide how your program behaves. They allow you to control the flow of your code. Here are some key types:
- If statements: Make decisions based on conditions.
- Loops: Repeat actions until a condition is met.
- Switch statements: Choose between multiple options.
Functions and Methods
Functions are blocks of code that perform a specific task. You can define a function once and use it many times. For example:
def add(a, b):
return a + b
This function takes two numbers and returns their sum.
Methods are similar but are associated with objects in object-oriented programming.
Debugging and Error Handling
Debugging is the process of finding and fixing errors in your code. Here are some tips:
- Read error messages: They often tell you what went wrong.
- Use print statements: Check the values of variables at different points.
- Test your code: Run small parts of your program to see if they work as expected.
Remember, every programmer makes mistakes. The key is to learn from them and keep improving your skills!
In summary, understanding these core concepts is essential for anyone looking to start coding. They form the foundation of how to write effective programs and solve problems. As you learn, keep practicing and experimenting with these ideas!
Exploring Popular Programming Languages
Introduction to Python
Python is a versatile language that is great for beginners. It is known for its easy-to-read syntax and can be used for many things like web development, data analysis, and even artificial intelligence. Its extensive libraries and community support make it a top choice for new programmers.
JavaScript for Web Development
JavaScript is essential for making websites interactive. It allows you to create dynamic content that responds to user actions. If you want to dive into web development, learning JavaScript is a must after mastering HTML and CSS. Here’s a quick look at its features:
- Dynamic Interactivity: Makes web pages lively.
- Frontend and Backend: Works on both sides of web development.
- Frameworks: Supports tools like React and Node.js.
The Versatility of Java
Java is a widely-used programming language known for its reliability. It is often used in building large applications and Android apps. Its syntax is similar to Python, making it easier to learn if you already know Python. Here are some key points about Java:
- Platform Independence: Write once, run anywhere.
- Object-Oriented: Focuses on objects, making it easier to manage complex programs.
Getting to Know C++
C++ is a powerful language that combines low-level and high-level programming features. It is often used in game development and applications where performance is critical. Here’s why you might want to learn C++:
- System-Level Programming: Great for understanding how computers work.
- Game Development: Many games are built using C++.
- Performance: Ideal for applications that need speed.
Learning these languages can open many doors in the tech world. Some of the best programming languages to help you get a job include Python, JavaScript, Java, and HTML.
Practical Tips for New Programmers
Practice Regularly
To become a good programmer, practice is key. Here’s a simple plan to follow:
- Learn the basics of a programming language.
- Start writing small programs.
- Pick a specialization that interests you.
- Learn the important tools in that area.
- Keep practicing!
- Prepare for job interviews.
Join a Coding Community
Being part of a coding community can help you a lot. You can:
- Share your projects.
- Get feedback from others.
- Ask questions when you’re stuck.
- Learn from experienced programmers.
Utilize Online Resources
There are many online resources available. Some useful ones include:
- Coding tutorials on YouTube.
- Free coding platforms like Codecademy.
- Forums like Stack Overflow for asking questions.
Stay Patient and Persistent
Learning to code can be tough. Remember:
"Every expert was once a beginner."
If you find something hard, don’t give up! Keep reviewing until it makes sense. Be willing to learn new things and try different methods to solve problems. This will help you improve your coding skills over time.
Advanced Topics for Future Learning
Understanding Algorithms and Data Structures
Algorithms and data structures are essential for solving complex problems efficiently. Mastering these concepts can significantly improve your programming skills. Here are some key areas to focus on:
- Sorting Algorithms: Learn techniques like bubble sort, insertion sort, and quicksort.
- Data Structures: Familiarize yourself with arrays, linked lists, stacks, queues, and trees.
- Algorithmic Complexity: Understand time and space complexity to evaluate how efficient your algorithms are.
Introduction to Frameworks and Libraries
Frameworks and libraries can make programming easier by providing pre-written code. Here are some popular ones:
Framework/Library | Language | Purpose |
---|---|---|
React | JavaScript | Building user interfaces |
Django | Python | Web development |
Spring | Java | Enterprise applications |
TensorFlow | Python | Machine learning |
Exploring Software Development Methodologies
Learning about different software development methodologies can help you work better in teams. Here are a few:
- Agile: Focuses on iterative development and collaboration.
- Waterfall: A linear approach where each phase must be completed before the next begins.
- DevOps: Combines development and operations for faster delivery.
Understanding these methodologies can help you adapt to various work environments and improve your teamwork skills.
If you’re eager to dive deeper into coding and enhance your skills, now is the perfect time to take action! Visit our website to explore a variety of resources designed to help you succeed in coding interviews and beyond. Don’t wait—start your journey today!
Conclusion
In summary, programming is a vital skill in our tech-driven world. It opens doors to many career paths and allows you to create amazing things, from apps to websites. As you start your coding journey, remember to be patient and practice regularly. Don’t hesitate to seek help from online communities or resources. With time and effort, you can become a confident programmer. So, dive in and enjoy the learning process!
Frequently Asked Questions
Can I learn programming without a degree in computer science?
Yes! Many successful programmers are self-taught. With hard work, practice, and good resources, anyone can learn to code.
Which programming language should I start with?
Python is a great choice for beginners because it is simple and easy to read. It has a lot of resources to help you learn.
How long will it take to learn programming?
It depends on how much time you can dedicate. With regular practice, you can build a strong foundation in a few months.
Are there many job opportunities in programming?
Absolutely! There is a growing demand for programmers in many industries as technology keeps advancing.
Can beginners create their own software or apps?
Starting with simple projects is a good idea. As you learn the basics, you can gradually take on bigger challenges.
What if I get stuck while learning to code?
It’s normal to feel stuck sometimes. Take breaks, ask for help, and keep practicing. Everyone faces challenges when learning.