Understanding the Types of Sorting Algorithms: A Comprehensive Guide
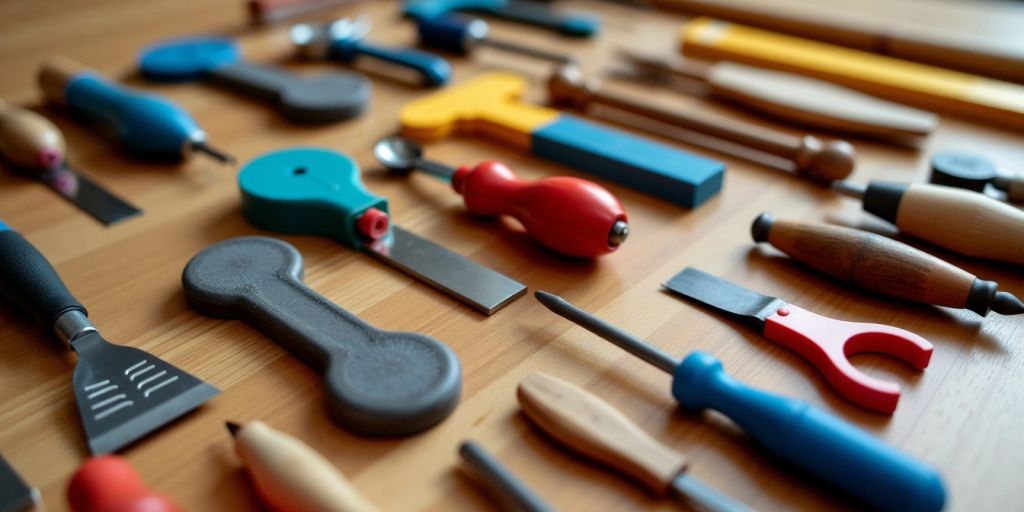
Sorting algorithms are essential tools in computer science, helping to organize data so we can find and use it more easily. In this guide, we will explore different types of sorting algorithms, their importance, and how they work. By understanding these algorithms, you can choose the right one for your needs, whether you’re coding a project or preparing for a job interview.
Key Takeaways
- Sorting algorithms help organize data for easier access and analysis.
- Different algorithms have unique strengths and weaknesses depending on the dataset size.
- Simple algorithms like Bubble Sort and Insertion Sort are good for small datasets.
- More complex algorithms like Quick Sort and Merge Sort are better for larger datasets.
- Choosing the right algorithm depends on factors like speed, memory use, and data characteristics.
Introduction to Sorting Algorithms
Sorting algorithms are essential tools in computer science. They help us organize data in a specific order, either from smallest to largest or vice versa. Understanding these algorithms is crucial because they make searching and analyzing data much easier.
Definition and Importance
Sorting refers to the rearrangement of a given array or list of elements according to a comparison operator on the elements. This process is vital for efficient data retrieval and presentation. For example, when you look up a name in a phone book, the names are sorted alphabetically, making it easier to find what you need.
Historical Background
The concept of sorting dates back to ancient times when people needed to organize information. Over the years, various sorting methods have been developed, each with its own strengths and weaknesses. Today, sorting algorithms are widely used in computer programming and data management.
Basic Concepts
Sorting algorithms can be divided into two main types: comparison-based and non-comparison-based. Here’s a quick overview:
- Comparison-Based Sorting: These algorithms sort data by comparing elements. Examples include Bubble Sort and Quick Sort.
- Non-Comparison-Based Sorting: These algorithms do not compare elements directly. Examples include Counting Sort and Radix Sort.
Type of Sorting Algorithm | Description |
---|---|
Comparison-Based | Sorts by comparing elements |
Non-Comparison-Based | Sorts without direct comparisons |
Understanding these basic concepts will help you choose the right sorting algorithm for your needs.
Sorting algorithms are the backbone of data organization and manipulation in the digital age. They play a vital role in making data more accessible and understandable.
Comparison-Based Sorting Algorithms
Overview of Comparison Sorts
Comparison-based sorting algorithms are methods that sort data by comparing elements to determine their order. These algorithms are fundamental in computer science and are widely used due to their simplicity and effectiveness. They generally operate on the principle of comparing pairs of elements and deciding their positions based on these comparisons.
Examples of Comparison Sorts
Here are some common comparison-based sorting algorithms:
- Bubble Sort: This algorithm repeatedly compares adjacent elements and swaps them if they are in the wrong order. It continues this process until the entire list is sorted.
- Insertion Sort: This method builds a sorted array one element at a time by taking an element from the unsorted part and placing it in the correct position in the sorted part.
- Selection Sort: This algorithm finds the minimum element from the unsorted part and swaps it with the first unsorted element, gradually building a sorted section.
- Merge Sort: A divide-and-conquer algorithm that splits the array into smaller parts, sorts them, and then merges them back together.
- Quick Sort: This algorithm selects a pivot element and partitions the array into two subarrays, sorting them recursively.
- Heap Sort: This method uses a binary heap data structure to sort elements by repeatedly extracting the largest element and placing it in the sorted section.
Advantages and Disadvantages
Algorithm | Time Complexity (Worst Case) | Space Complexity | Advantages | Disadvantages |
---|---|---|---|---|
Bubble Sort | O(n²) | O(1) | Simple to understand | Inefficient for large datasets |
Insertion Sort | O(n²) | O(1) | Efficient for small datasets | Still O(n²) in worst case |
Selection Sort | O(n²) | O(1) | Simple and easy to implement | Not suitable for large datasets |
Merge Sort | O(n log n) | O(n) | Efficient for large datasets | Requires additional space |
Quick Sort | O(n²) | O(log n) | Fast on average | Worst case can be slow |
Heap Sort | O(n log n) | O(1) | Good for memory-constrained systems | More complex to implement |
Comparison-based sorting algorithms are essential for organizing data efficiently, making them a crucial part of computer science education.
Bubble Sort: The Simplest Sorting Algorithm
How Bubble Sort Works
Bubble Sort is a straightforward sorting method. It works by repeatedly swapping adjacent elements if they are in the wrong order. This process continues until the entire list is sorted. The name comes from the way smaller elements seem to "bubble" to the top of the list.
Time and Space Complexity
The time complexity of Bubble Sort is O(n²) in the worst case, which means it can be quite slow for larger datasets. However, it is an in-place sorting algorithm, requiring only a small amount of additional memory (O(1)).
Use Cases and Limitations
Bubble Sort is mainly used for educational purposes due to its simplicity. Here are some points to consider:
- Best for small datasets: It works well when the dataset is small.
- Not efficient for large datasets: For larger datasets, other algorithms like Quick Sort or Merge Sort are much faster.
- Stable sorting: It maintains the relative order of equal elements, which can be important in some applications.
While Bubble Sort is easy to understand, it is not the best choice for sorting large amounts of data.
Feature | Bubble Sort |
---|---|
Time Complexity | O(n²) |
Space Complexity | O(1) |
Stability | Yes |
Best Use Case | Small datasets |
Insertion Sort: Efficient for Small Data Sets
Mechanism of Insertion Sort
Insertion Sort is a straightforward sorting method that organizes an array by building a sorted section one element at a time. It begins by assuming the first element is sorted. Then, it takes the next element from the unsorted section and places it in the correct position within the sorted section. This process continues until the entire array is sorted. This algorithm is particularly effective for small datasets.
Performance Analysis
The time complexity of Insertion Sort is O(n²) in the worst case, which means it can be slow for large datasets. However, it performs better when the data is already partially sorted. Here’s a quick comparison of its performance:
Data Condition | Time Complexity |
---|---|
Unsorted | O(n²) |
Partially Sorted | O(n) |
Nearly Sorted | O(n) |
Practical Applications
Insertion Sort is best used in scenarios such as:
- Sorting small lists
- Sorting data that is already partially sorted
- Situations where memory is limited, like embedded systems
Insertion Sort is a great choice for small to medium-sized datasets, especially when the data is nearly sorted. It’s easy to implement and requires minimal additional memory.
Summary
In conclusion, while Insertion Sort may not be the fastest option for large datasets, its simplicity and efficiency for small or partially sorted datasets make it a valuable tool in a programmer’s toolkit. Understanding its strengths and weaknesses can help you choose the right sorting algorithm for your needs.
Selection Sort: Finding the Minimum
Working Principle of Selection Sort
Selection Sort is a straightforward sorting method that works by repeatedly finding the minimum element from the unsorted portion of an array and moving it to the front. Here’s how it operates:
- Start with the first element of the array as the minimum.
- Compare this minimum with the other elements in the unsorted part.
- If a smaller element is found, update the minimum.
- Swap the minimum element with the first element of the unsorted part.
- Move to the next element and repeat until the array is sorted.
Efficiency Considerations
Selection Sort has a time complexity of O(n²), which means it can be slow for large datasets. Here’s a quick comparison of its performance:
Dataset Size | Time Complexity |
---|---|
Small | O(n) |
Medium | O(n²) |
Large | O(n²) |
Selection Sort is effective for sorting small datasets where the overhead of more complex algorithms isn’t justified.
Real-World Examples
Selection Sort is often used in educational settings to teach sorting concepts. It can also be useful in scenarios where simplicity is more important than speed, such as:
- Small lists of items
- Situations where memory usage is a concern
- Teaching basic algorithm principles
Selection Sort is a great way to understand the basics of sorting algorithms, even if it’s not the most efficient choice for larger datasets.
Merge Sort: Divide and Conquer
Steps in Merge Sort
Merge Sort is a popular sorting algorithm known for its efficiency and stability. It follows a divide-and-conquer approach to sort a given array of elements. Here’s how it works:
- Divide: Split the array into two halves until each subarray contains a single element.
- Conquer: Recursively sort each half.
- Merge: Combine the sorted halves back into a single sorted array.
Complexity Analysis
Merge Sort has a time complexity of O(n log n) in all cases (best, average, and worst). This makes it faster than simpler algorithms like Bubble Sort and Insertion Sort, which have a time complexity of O(n²). However, it requires additional space for temporary storage during the merge process, leading to a space complexity of O(n).
When to Use Merge Sort
Merge Sort is particularly useful when:
- You need a stable sort (it keeps the order of equal elements).
- You are working with large datasets.
- You have enough memory to handle the additional space required.
Merge Sort is a highly efficient sorting algorithm that is great for large datasets, but it does require extra memory for merging.
In summary, Merge Sort is a reliable choice for sorting tasks that demand efficiency and stability, especially with larger datasets.
Quick Sort: Fast and Efficient
Quick Sort Algorithm Explained
Quick Sort is a divide-and-conquer algorithm that sorts an array by selecting a pivot element. It then partitions the array into two parts: elements less than the pivot and elements greater than the pivot. This process is repeated recursively on the sub-arrays until the entire array is sorted. The steps are as follows:
- Choose a pivot element from the array.
- Partition the array into two groups: elements less than the pivot and elements greater than the pivot.
- Recursively sort the two groups.
- Combine the sorted groups and the pivot to get the final sorted array.
Average and Worst-Case Scenarios
Quick Sort is known for its efficiency. It has an average time complexity of O(n log n), making it suitable for large datasets. However, in the worst-case scenario, where the pivot is the smallest or largest element, the time complexity can degrade to O(n²). Here’s a quick comparison:
Scenario | Time Complexity |
---|---|
Best Case | O(n log n) |
Average Case | O(n log n) |
Worst Case | O(n²) |
Practical Implementations
Quick Sort is widely used in various applications due to its speed and efficiency. It is particularly effective for:
- Sorting large datasets
- In-place sorting where memory usage is a concern
- Situations where average performance is more critical than worst-case performance
Quick Sort is a popular choice for sorting because it is fast and requires minimal extra memory.
Summary
In summary, Quick Sort is a powerful sorting algorithm that efficiently handles large datasets. Its average-case performance is impressive, but it’s essential to be aware of its worst-case scenarios. Understanding when to use Quick Sort can greatly enhance your sorting capabilities.
Heap Sort: Using Binary Heaps
Understanding Heap Sort
Heap Sort is a comparison-based sorting technique that uses a binary heap data structure. It organizes the data in a way that allows for efficient sorting. The process begins by building a binary heap from the input array. A binary heap is a special tree structure where each parent node is greater than or equal to its children. This property helps in sorting the data effectively.
Steps in Heap Sort
The steps involved in Heap Sort are:
- Build a binary heap from the input array.
- Extract the largest element from the root and swap it with the last element of the unsorted region.
- Heapify the remaining unsorted region to maintain the binary heap property.
- Repeat the above steps until the entire array is sorted.
Performance Metrics
Heap Sort has a time complexity of O(n log n), making it efficient for large datasets. Here’s a quick comparison of Heap Sort with other algorithms:
Algorithm | Time Complexity (Best) | Time Complexity (Average) | Time Complexity (Worst) | Space Complexity |
---|---|---|---|---|
Heap Sort | O(n log n) | O(n log n) | O(n log n) | O(1) |
Quick Sort | O(n log n) | O(n log n) | O(n²) | O(log n) |
Merge Sort | O(n log n) | O(n log n) | O(n log n) | O(n) |
Advantages and Disadvantages
Advantages:
- Heap Sort is an in-place sorting algorithm, meaning it doesn’t require extra memory for sorting.
- It is stable, maintaining the order of equal elements.
Disadvantages:
- It can be slower than other algorithms like Quick Sort for smaller datasets.
- The construction of the binary heap requires additional space, which can be a drawback for large datasets.
In summary, Heap Sort is a powerful sorting algorithm that is particularly useful when memory efficiency is a priority. It balances speed and space, making it a solid choice for many applications.
Non-Comparison Sorting Algorithms
Introduction to Non-Comparison Sorts
Non-comparison sorting algorithms offer a fresh approach to organizing data. These methods, including counting sort, radix sort, and bucket sort, do not rely on comparing elements to sort them. Instead, they use other techniques to achieve sorting more efficiently in certain scenarios.
Types of Non-Comparison Sorts
- Counting Sort: This algorithm counts the number of occurrences of each unique element. It then calculates the position of each element in the sorted array based on these counts.
- Radix Sort: Radix sort processes the digits of the numbers from the least significant to the most significant. It uses counting sort as a subroutine to sort the numbers based on each digit.
- Bucket Sort: This method distributes elements into several "buckets". Each bucket is then sorted individually, either using a different sorting algorithm or recursively applying bucket sort.
Benefits and Drawbacks
- Benefits:
- Drawbacks:
Non-comparison sorting algorithms can be very effective, especially when dealing with large datasets or specific types of data. They provide a unique way to sort without the need for direct comparisons, making them valuable tools in a programmer’s toolkit.
Radix Sort: Sorting by Digits
Radix Sort Mechanism
Radix sort is a unique sorting method that organizes numbers by their digits. It starts with the least significant digit and works its way to the most significant. This means that it sorts the numbers based on each digit, one at a time. For example, if we have the numbers [170, 45, 75, 90, 802, 24, 2, 66], the sorting process would look like this:
- Sort by the least significant digit: [170, 90, 802, 2, 24, 45, 75, 66]
- Sort by the second least significant digit: [802, 2, 24, 45, 66, 170, 75, 90]
- Sort by the most significant digit: [2, 24, 45, 66, 75, 90, 170, 802]
This method allows radix sort to achieve the final sorted order by repeatedly sorting the elements by their significant digits.
Efficiency and Use Cases
The time complexity of radix sort is O(d*(n+k)), where:
- d is the number of digits,
- n is the number of elements,
- k is the range of the digits.
This makes radix sort faster than many comparison-based algorithms, especially when dealing with large datasets. However, it does require more memory, which can be a downside.
Comparison with Other Algorithms
Radix sort is particularly effective for sorting integers or strings with a fixed length. Here’s a quick comparison:
Algorithm | Time Complexity | Space Complexity | Best Use Case |
---|---|---|---|
Radix Sort | O(d*(n+k)) | O(n+k) | Large datasets of integers |
Quick Sort | O(n log n) | O(log n) | General-purpose sorting |
Merge Sort | O(n log n) | O(n) | Stable sorting for large data |
Bubble Sort | O(n²) | O(1) | Small datasets |
Radix sort is a powerful tool for sorting large amounts of data quickly, but it’s important to consider the specific needs of your application before choosing it as your sorting method.
Counting Sort: Efficient for Specific Data
How Counting Sort Works
Counting sort is a special sorting method that works best with whole numbers. It counts how many times each number appears in the list. For example, if you have the numbers 1, 2, 2, and 3, counting sort will count:
Number | Count |
---|---|
1 | 1 |
2 | 2 |
3 | 1 |
Using this count, it can then place each number in the correct order in a new list. This method is very fast for certain types of data, especially when the range of numbers is not too large.
Time and Space Efficiency
Counting sort is known for its speed. In fact, it generally performs faster than all comparison-based sorting algorithms, such as merge sort and quicksort, if the range of numbers is small. Its time complexity is O(n + k), where n is the number of elements and k is the range of the input values. However, it does require extra space for the count array, which can be a downside if the range of numbers is very large.
Ideal Scenarios for Counting Sort
Counting sort is best used when:
- The input consists of non-negative integers.
- The range of the input values (k) is not significantly larger than the number of elements (n).
- You need a stable sort, meaning that equal elements maintain their original order.
Counting sort is a great choice when you have specific data types and need a quick solution. It shines in scenarios where other sorting methods might struggle.
In summary, counting sort is a powerful tool for sorting specific types of data quickly and efficiently, making it a valuable addition to any programmer’s toolkit.
Choosing the Right Sorting Algorithm
When it comes to choosing a sorting algorithm, there are several important factors to consider. The right choice can greatly affect the performance of your application. Here are some key points to keep in mind:
Factors to Consider
- Input Size: The size of your dataset matters. For small datasets, simpler algorithms like Bubble Sort or Insertion Sort may work well. For larger datasets, more efficient algorithms like Merge Sort or Quick Sort are usually better.
- Time Complexity: Different algorithms have different time complexities. For example, Quick Sort has an average time complexity of O(n log n), while Bubble Sort has O(n²). Understanding these differences can help you choose wisely.
- Space Complexity: Some algorithms require more memory than others. If memory is limited, you might prefer algorithms like Heap Sort, which is more memory-efficient.
- Stability: Stability refers to whether equal elements maintain their relative order after sorting. If this is important for your application, consider stable algorithms like Merge Sort.
Comparative Analysis
Here’s a quick comparison of some common sorting algorithms:
Algorithm | Time Complexity (Worst) | Space Complexity | Stability |
---|---|---|---|
Bubble Sort | O(n²) | O(1) | Yes |
Insertion Sort | O(n²) | O(1) | Yes |
Selection Sort | O(n²) | O(1) | No |
Merge Sort | O(n log n) | O(n) | Yes |
Quick Sort | O(n²) | O(log n) | No |
Heap Sort | O(n log n) | O(1) | No |
Radix Sort | O(k * n) | O(n + k) | Yes |
Counting Sort | O(n + k) | O(k) | Yes |
Best Practices
- Understand Your Data: Before selecting an algorithm, analyze the characteristics of your data. This includes its size, type, and whether it’s already partially sorted.
- Test Different Algorithms: If possible, implement and test multiple algorithms on your dataset to see which performs best.
- Keep Learning: Sorting algorithms are a fundamental part of computer science. Continuously learning about them will help you make better decisions in the future.
Choosing the right sorting algorithm is crucial for optimizing performance. Consider the running time, space complexity, and the expected format of your data to make an informed decision.
When picking a sorting method, it’s important to think about what you need. Different algorithms work better for different tasks. For example, if you have a lot of data, some methods will be faster than others. To learn more about sorting algorithms and how they can help you in coding interviews, visit our website and start coding for free!
Conclusion
In summary, sorting algorithms are essential tools that help us organize data efficiently. They make it easier to find and use information, whether it’s in a phone book or a computer database. Each sorting method has its own strengths and weaknesses, making some better for small lists and others for larger ones. By understanding these algorithms, you can choose the best one for your needs, improving both speed and performance in your projects. As technology continues to grow, knowing how to sort data effectively will remain a key skill for anyone working with information.
Frequently Asked Questions
What is a sorting algorithm?
A sorting algorithm is a method used to arrange items in a specific order, like from smallest to largest or alphabetically.
Why are sorting algorithms important?
Sorting algorithms help in organizing data, making it easier to find and use. They speed up searching and improve data presentation.
What are some common types of sorting algorithms?
Some well-known sorting algorithms include Bubble Sort, Insertion Sort, Quick Sort, and Merge Sort.
How does Bubble Sort work?
Bubble Sort compares adjacent items in a list and swaps them if they are in the wrong order. It keeps doing this until the entire list is sorted.
What is the best sorting algorithm for small datasets?
For small datasets, simple algorithms like Bubble Sort or Insertion Sort usually work well.
What is the difference between comparison-based and non-comparison sorting algorithms?
Comparison-based algorithms sort items by comparing them, while non-comparison algorithms, like Counting Sort, use counting techniques to sort.
When should I use Merge Sort?
Use Merge Sort when you have a large dataset that needs to be sorted efficiently, as it works well with big lists.
Are sorting algorithms used in real life?
Yes, sorting algorithms are used in everyday applications like organizing contacts in a phone or sorting search results online.