Understanding What is REST: A Comprehensive Guide to RESTful Architecture
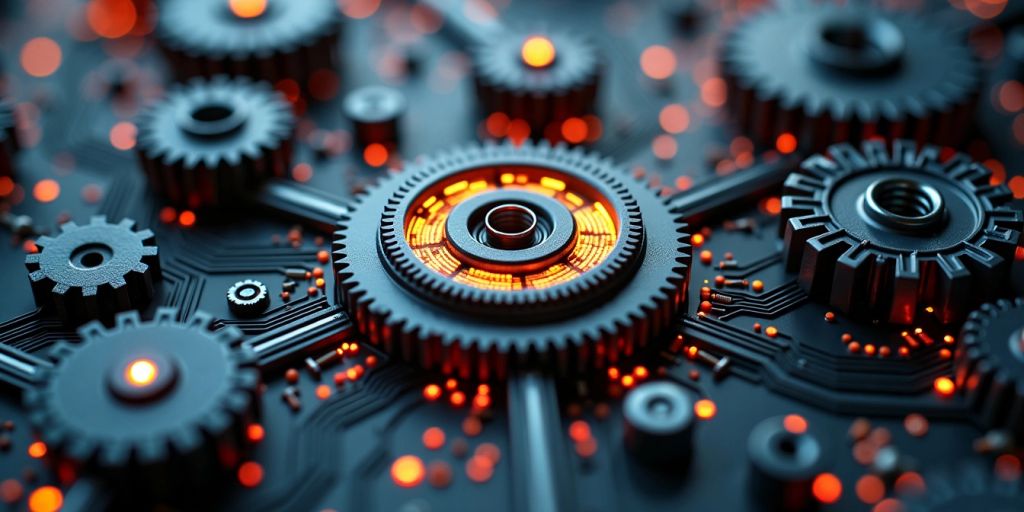
In today’s tech-driven world, understanding RESTful architecture is essential for anyone involved in web development. REST, or Representational State Transfer, simplifies how applications communicate over the internet. This guide will break down the key aspects of REST, its principles, and why it matters in modern software design.
Key Takeaways
- REST stands for Representational State Transfer, a way for apps to communicate over the web.
- It is built on principles like statelessness, meaning each request is independent.
- Resources in REST are identified by unique URLs, making them easy to locate.
- Common actions in REST are performed using HTTP methods like GET, POST, PUT, and DELETE.
- RESTful APIs are popular because they are simple, scalable, and flexible.
What is REST?
Definition and Origin
REST, which stands for Representational State Transfer, is a way to design networked applications. It was introduced by Roy Fielding in 2000. REST is not a protocol but an architectural style that helps developers create web services that are easy to use and understand. In REST, resources are identified using unique URLs, making it simple to access them.
Importance in Modern Web Development
REST has become essential in modern web development because it allows different applications to communicate easily. It promotes a clear separation between the client and server, which means that developers can work on each part independently. This flexibility is crucial for building scalable and maintainable applications.
Common Misconceptions
Many people confuse REST with RESTful APIs. While REST is a set of guidelines, a RESTful API is an API that follows these guidelines. Understanding this difference is important for developers to create effective web services.
RESTful architecture is about simplicity and efficiency, making it a popular choice for web services today.
Aspect | REST | RESTful API |
---|---|---|
Definition | Architectural style | API that follows REST |
Protocol | Not a protocol | Often uses HTTP |
State Management | Stateless | Can be stateful or stateless |
Resource Access | Via URIs | Via URIs |
In summary, REST is a powerful architectural style that has transformed how applications interact over the web. Understanding its principles is key to leveraging its full potential.
Core Principles of REST
Statelessness
In REST, statelessness means that each request from a client to a server is independent. This means the server does not keep any information about previous requests. Each request must contain all the information needed to understand and process it. This design makes REST APIs more scalable and reliable.
Client-Server Architecture
REST uses a client-server architecture where the client and server are separate. The client handles the user interface and user requests, while the server manages data and processes. This separation allows both sides to evolve independently, making it easier to update and maintain applications.
Cacheability
In REST, responses can be cached to improve performance. When a response is cacheable, clients can store it and reuse it for future requests. This reduces the need to contact the server every time, speeding up the process and reducing server load.
Layered System
REST supports a layered system architecture. This means that a client cannot tell whether it is connected directly to the end server or to an intermediary. This allows for scalability and flexibility, as different layers can be added or modified without affecting the overall system.
Understanding these core principles helps in designing effective RESTful APIs that are easy to use and maintain.
Principle | Description |
---|---|
Statelessness | Each request is independent and contains all necessary information. |
Client-Server | Clear separation between client and server roles. |
Cacheability | Responses can be stored and reused to improve performance. |
Layered System | Architecture can have multiple layers, enhancing scalability and flexibility. |
These principles are essential for creating RESTful APIs that are efficient and easy to work with.
Key Components of RESTful APIs
Resources and URIs
In RESTful APIs, resources are the key elements that clients interact with. Each resource is identified by a unique URI (Uniform Resource Identifier). For example, a collection of books might be accessed via /books
, while a specific book could be accessed at /books/123
. This clear identification helps in managing resources effectively.
HTTP Methods
RESTful APIs utilize standard HTTP methods to perform actions on resources. Here’s a quick overview of the main methods:
- GET: Retrieve a resource.
- POST: Create a new resource.
- PUT: Update an existing resource.
- DELETE: Remove a resource.
These methods allow clients to interact with resources in a straightforward manner.
Representation Formats
Resources can be represented in various formats, such as JSON or XML. Clients can specify their preferred format using HTTP headers like Accept
. This flexibility allows different systems to communicate effectively, regardless of their underlying technology.
Hypermedia as the Engine of Application State (HATEOAS)
HATEOAS is a key principle of REST that allows clients to navigate the API dynamically. Instead of hardcoding URLs, responses from the server include links to related resources. This means that clients can discover actions they can take based on the current state of the application, enhancing usability and flexibility.
In summary, RESTful APIs are designed to be simple and efficient, allowing for easy interaction between clients and servers. Understanding these key components is essential for anyone looking to work with RESTful architecture.
Benefits of RESTful Architecture
Scalability
RESTful architecture is designed to be highly scalable. This means that as the number of users grows, the system can handle more requests without slowing down. The stateless nature of REST allows servers to manage requests without keeping track of previous interactions, which helps in distributing the load efficiently.
Interoperability
One of the key advantages of RESTful APIs is their interoperability. They can work with various programming languages and platforms, making it easier for different systems to communicate. This flexibility allows developers to use the best tools for their projects without worrying about compatibility issues.
Flexibility
RESTful APIs offer great flexibility in how data is represented and accessed. Developers can choose from multiple formats like JSON or XML, depending on what works best for their application. This adaptability makes it easier to meet the specific needs of different clients.
Performance
The performance of RESTful APIs is often enhanced through caching. By storing frequently accessed data, the system can respond faster to requests. This reduces the load on servers and improves the overall user experience.
RESTful architecture provides a solid foundation for building modern web services, making it easier to create efficient and effective applications.
Benefit | Description |
---|---|
Scalability | Handles increased load without performance loss. |
Interoperability | Works across different platforms and languages. |
Flexibility | Supports various data formats for diverse client needs. |
Performance | Utilizes caching to speed up response times. |
Common Use Cases of RESTful APIs
RESTful APIs are widely used in various applications due to their flexibility and ease of integration. Here are some common scenarios where they shine:
Web Services Integration
- Communication Services: Platforms like Twilio utilize RESTful APIs to enable messaging and voice services.
- Payment Processing: Companies such as Stripe use REST APIs to handle online transactions securely.
- Location Services: Google Maps provides RESTful APIs for accessing geographical data and services.
Mobile Application Backend
Many mobile applications rely on RESTful APIs to connect to their backends. For instance:
- Ride-Sharing Apps: Services like Uber and Lyft use REST APIs to manage ride requests and access maps.
- Banking Apps: These apps access account information and facilitate transactions through RESTful APIs.
Cloud Services
RESTful APIs are essential in cloud computing, allowing applications to interact with cloud resources seamlessly. They enable:
- Resource Management: Users can manage cloud resources through simple API calls.
- Microservices Communication: Different services can communicate effectively using RESTful APIs.
IoT Applications
The Internet of Things (IoT) heavily relies on RESTful APIs for device communication. Examples include:
- Smart Home Devices: Devices like smart thermostats use REST APIs to send and receive data.
- Wearable Technology: Fitness trackers often communicate with mobile apps via RESTful APIs.
RESTful APIs are a key component in modern software development, enabling diverse applications to communicate effectively and efficiently.
In summary, RESTful APIs are integral to various sectors, enhancing functionality and user experience across platforms. Their ability to facilitate communication between different systems makes them a preferred choice for developers.
Best Practices for Designing RESTful APIs
Using Nouns for Resource URLs
When creating URLs for your resources, always use nouns. For example, instead of using verbs, structure your URLs like /books
for a collection of books or /books/123
for a specific book. This makes it easier for users to understand what they are accessing.
Versioning
Including a version number in your API URL is a smart practice. For instance, use /v1/books
to indicate the first version of your API. This helps you make changes without breaking existing applications that rely on your API.
Error Handling
It’s crucial to implement graceful error handling. Always use standard HTTP status codes to indicate the result of an API request. For example, a 200 status code means success, while a 404 indicates that the resource was not found. This helps users quickly understand what went wrong.
Security Considerations
Security should be a top priority. Always use authentication and encryption to protect your API. This is especially important for public APIs that handle sensitive data.
Caching
Utilizing caching can significantly improve performance. Both server-side and client-side caching can speed up response times. For example, if a client frequently requests the same data, caching that data can reduce the need for repeated API calls.
Summary Table of Best Practices
Practice | Description |
---|---|
Use Nouns for URLs | Structure URLs with nouns for clarity. |
Versioning | Include version numbers in URLs for backward compatibility. |
Error Handling | Use standard HTTP status codes for clear error reporting. |
Security Considerations | Implement authentication and encryption for data protection. |
Caching | Use caching to improve performance and reduce server load. |
Following these best practices will help you design robust and scalable RESTful APIs that are easy to use and maintain. A well-designed API can greatly enhance user experience and application performance.
Challenges and Limitations of REST
RESTful APIs, while popular, come with their own set of challenges. Here are some key issues:
Handling Complex Transactions
- Complex transactions can be difficult to manage in REST. Since each request is independent, maintaining a sequence of operations can be tricky. This can lead to inconsistencies if one part of a transaction fails while others succeed.
Performance Overhead
- REST APIs can experience performance overhead due to the stateless nature of requests. Each request must carry all necessary information, which can lead to larger payloads and longer response times.
Security Risks
- Security is a major concern. REST APIs must handle various security issues, such as:
- Using HTTPS to encrypt data
- Validating user inputs to prevent attacks
- Monitoring for unusual activity
- Implementing proper authentication methods like OAuth 2.0
REST APIs are not a one-size-fits-all solution. They require careful planning and management to address these challenges effectively.
In summary, while RESTful architecture offers many benefits, it also presents challenges that developers must navigate to ensure robust and secure applications. Understanding these limitations is crucial for effective API design.
When using REST, there are some bumps in the road. It can be tricky to manage state, and sometimes it doesn’t fit well with real-time needs. If you’re curious about how to tackle these issues and improve your coding skills, visit our website for free resources and interactive tutorials!
Conclusion
In conclusion, understanding REST is essential for anyone looking to work with web services today. REST, which stands for Representational State Transfer, provides a simple and effective way for different software systems to communicate. Its key principles, like statelessness and resource-based design, make it easy to build and maintain APIs. As we’ve seen, many popular platforms, such as Amazon and Twitter, rely on RESTful APIs to connect their services. By grasping the basics of REST, developers can create more efficient and flexible applications, making it a vital skill in the tech world.
Frequently Asked Questions
What does REST stand for?
REST stands for Representational State Transfer. It’s a way to design networked applications.
Why is REST important?
REST is important because it helps different software systems communicate easily over the internet.
What are the main parts of a RESTful API?
The main parts of a RESTful API include resources, HTTP methods, and response formats.
How does REST improve performance?
REST improves performance by being stateless, which means each request is independent and doesn’t require the server to remember past requests.
What are some common uses for RESTful APIs?
Common uses for RESTful APIs include connecting web services, mobile apps, and cloud services.
What should I keep in mind when designing a RESTful API?
When designing a RESTful API, use clear resource names, proper HTTP methods, and include versioning.