Mastering Sorting Algorithms for Your Coding Interview
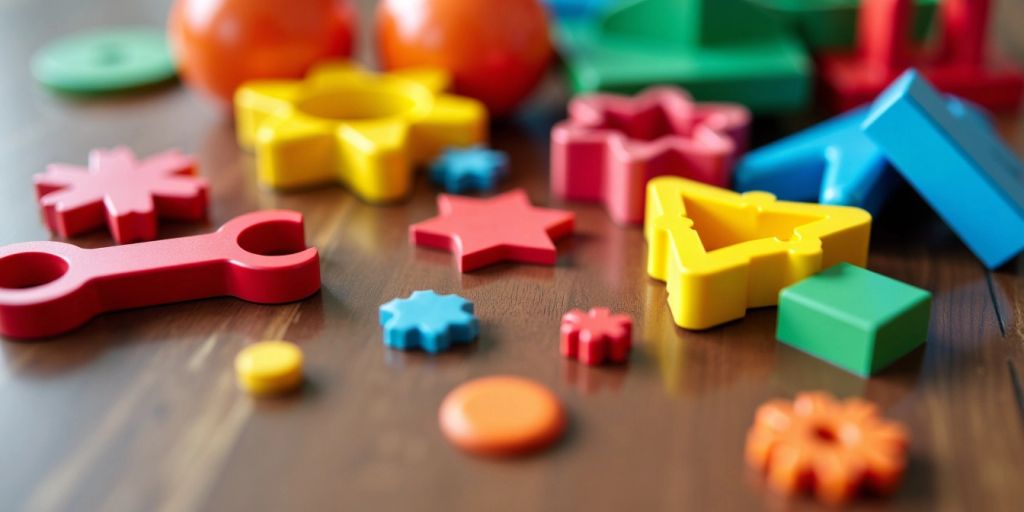
Sorting algorithms are essential tools in computer science, especially when preparing for coding interviews. They help organize data efficiently, which is crucial for problem-solving. Understanding these algorithms can not only improve your coding skills but also boost your chances of success in interviews. This article will guide you through the key sorting algorithms, their complexities, and common mistakes to avoid, helping you master this important topic.
Key Takeaways
- Sorting algorithms are vital for coding interviews and demonstrate problem-solving skills.
- Know at least one O(N log N) sorting algorithm, like Merge Sort or Quick Sort.
- Comparison-based sorts include Quick Sort, Merge Sort, and Heap Sort, while non-comparison sorts include Counting Sort and Radix Sort.
- Understanding time and space complexity is crucial for selecting the right sorting algorithm.
- Practice common sorting problems to sharpen your skills and prepare for interviews.
Understanding the Importance of Sorting Algorithms in Coding Interviews
Sorting algorithms are crucial in coding interviews because they help you demonstrate your problem-solving skills. Knowing how to sort data efficiently can set you apart from other candidates. Here’s why sorting algorithms matter:
Why Sorting Algorithms Matter
- Sorting is a fundamental operation in computer science.
- It helps in organizing data, making it easier to analyze and retrieve.
- Many real-world applications, like databases and search engines, rely on sorting.
Commonly Asked Sorting Algorithms
In interviews, you’ll often encounter:
- Quick Sort
- Merge Sort
- Heap Sort
These algorithms are popular because they represent efficient ways to sort data.
Impact on Problem-Solving Skills
Understanding sorting algorithms enhances your ability to tackle complex problems. It teaches you to break down issues into simpler parts and consider the trade-offs between time and space.
Mastering sorting algorithms equips you with essential tools for both practical applications and theoretical challenges in software engineering.
Sorting algorithms also play a vital role in data analysis: sorting helps in identifying patterns, trends, and outliers in datasets. It plays a vital role in statistical analysis, financial modeling, and more.
By grasping these concepts, you’ll be better prepared for your coding interviews and future programming tasks.
Comparison-Based Sorting Algorithms
Sorting algorithms that rely on comparing elements are essential in programming. These algorithms help determine the order of elements in a list or array. Here, we will explore three popular comparison-based sorting algorithms: Quick Sort, Merge Sort, and Heap Sort.
Quick Sort
Quick Sort is a fast sorting method that uses a technique called "divide and conquer." It picks a ‘pivot’ element and divides the other elements into two groups: those less than the pivot and those greater than it. After that, it sorts the two groups recursively.
- Time Complexity:
- Average Case: O(n log n)
- Worst Case: O(n²) (when the pivot is the smallest or largest element)
- Space Complexity: O(log n) due to recursive calls.
Merge Sort
Merge Sort works by splitting the input array in half, sorting each half, and then merging the sorted halves back together. This method is reliable and often used in various applications.
- Time Complexity:
- Worst Case: O(n log n)
- Best Case: O(n log n)
- Average Case: O(n log n)
- Space Complexity: O(n) due to the temporary arrays used during merging.
Heap Sort
Heap Sort is similar to selection sort but uses a data structure called a heap to find the largest element more efficiently. It repeatedly selects the largest item and moves it to the end of the array.
- Time Complexity:
- Worst Case: O(n log n)
- Best Case: O(n log n)
- Average Case: O(n log n)
- Space Complexity: O(1) since it sorts in place.
Algorithm | Worst Case | Best Case | Average Case | Space Complexity |
---|---|---|---|---|
Quick Sort | O(n²) | O(n log n) | O(n log n) | O(log n) |
Merge Sort | O(n log n) | O(n log n) | O(n log n) | O(n) |
Heap Sort | O(n log n) | O(n log n) | O(n log n) | O(1) |
Understanding these algorithms is crucial for coding interviews. They not only showcase your problem-solving skills but also your ability to choose the right algorithm for a given situation.
In summary, mastering these comparison-based sorting algorithms will significantly enhance your coding interview performance and problem-solving capabilities.
Remember, while these algorithms are commonly asked in interviews, knowing when to apply them is just as important!
Non-Comparison Sorting Algorithms
Non-comparison sorting algorithms offer a fresh approach to organizing data. These methods, including Counting Sort, Radix Sort, and Bucket Sort, do not rely on comparing elements to determine their order. Instead, they use different strategies to sort data efficiently.
Counting Sort
Counting Sort is an integer sorting algorithm that counts the number of occurrences of each distinct key value. It works best when the range of potential items is small compared to the number of items. For example, if you have a large number of integers between 1 and 10, Counting Sort could be very efficient. However, if the integers range from 1 to 1,000,000, it may not be practical due to memory constraints.
Radix Sort
Radix Sort sorts numbers digit by digit, starting from the least significant digit to the most significant. This algorithm is effective when the numbers to be sorted have the same number of digits. If the numbers vary in length or are floating-point numbers, Radix Sort may not be suitable or efficient.
Bucket Sort
Bucket Sort divides an array into several buckets. Each bucket is then sorted individually, either using a different sorting algorithm or by recursively applying the bucket sort algorithm. This method is useful when the input is uniformly distributed over a range but less effective if the data is heavily skewed.
Understanding when to use these non-comparison sorts can greatly enhance your problem-solving skills in coding interviews. However, be mindful of their limitations and trade-offs, such as memory usage and specific conditions for efficiency.
Time and Space Complexity of Sorting Algorithms
Big O Notation Explained
Big O notation is a way to describe how the time or space requirements of an algorithm grow as the input size increases. It helps us understand the efficiency of sorting algorithms. For example, O(n log n) is a common time complexity for efficient sorting algorithms like Quick Sort and Merge Sort.
Time Complexity of Common Algorithms
Here’s a quick overview of the time complexities for some popular sorting algorithms:
Algorithm | Best Case | Average Case | Worst Case | Space Complexity |
---|---|---|---|---|
Quick Sort | O(n log n) | O(n log n) | O(n²) | O(log n) |
Merge Sort | O(n log n) | O(n log n) | O(n log n) | O(n) |
Heap Sort | O(n log n) | O(n log n) | O(n log n) | O(1) |
Insertion Sort | O(n) | O(n²) | O(n²) | O(1) |
Bubble Sort | O(n) | O(n²) | O(n²) | O(1) |
Selection Sort | O(n²) | O(n²) | O(n²) | O(1) |
Counting Sort | O(n + k) | O(n + k) | O(n + k) | O(n + k) |
Space Complexity Considerations
Space complexity refers to the amount of extra memory an algorithm needs. In-place sorting algorithms, like Quick Sort and Heap Sort, use minimal extra space, making them efficient for memory usage. On the other hand, algorithms like Merge Sort require more space, which can be a drawback in memory-limited environments.
Understanding the time and space complexities of sorting algorithms is crucial for selecting the right one for your coding challenges.
Summary
In summary, knowing the time and space complexities of sorting algorithms helps you choose the best one for your needs. Always consider the size and nature of your data when making your choice. This knowledge not only prepares you for coding interviews but also enhances your problem-solving skills in real-world scenarios.
Remember, we’ve covered the time and space complexities of 9 popular sorting algorithms: bubble sort, selection sort, insertion sort, merge sort, quicksort, heap sort, and more!
In-Place vs. Out-of-Place Sorting
Definitions and Differences
In sorting, algorithms can be classified as either in-place or out-of-place. An in-place sorting algorithm sorts the data within the original data structure, using a small amount of extra space. This means it doesn’t need a lot of additional memory, making it efficient for memory usage. On the other hand, out-of-place sorting algorithms require extra space proportional to the input size, which can be a drawback in memory-limited situations.
Examples of In-Place Sorting
Some common in-place sorting algorithms include:
- Quick Sort
- Heap Sort
- Insertion Sort
- Bubble Sort
These algorithms modify the original data structure directly, which can be beneficial when working with large datasets.
Examples of Out-of-Place Sorting
In contrast, out-of-place sorting algorithms like Merge Sort and Counting Sort create new data structures to hold the sorted data. This can lead to higher memory usage, especially with large inputs.
Algorithm | Type | Space Complexity |
---|---|---|
Quick Sort | In-Place | O(log n) |
Merge Sort | Out-of-Place | O(n) |
Heap Sort | In-Place | O(1) |
Counting Sort | Out-of-Place | O(n + k) |
Understanding the difference between in-place and out-of-place sorting algorithms is crucial for optimizing memory usage in your programs.
In summary, knowing when to use in-place versus out-of-place sorting can greatly impact the efficiency of your code. Choosing the right algorithm based on your specific needs is key to mastering sorting algorithms.
Stability in Sorting Algorithms
What is Stability?
In sorting, stability means that when two elements have the same value, their original order is kept in the sorted list. For example, if you have two books by the same author, a stable sort will keep them in the order they were before sorting.
Stable vs. Unstable Algorithms
Here are some examples of stable and unstable sorting algorithms:
- Stable Algorithms:
- Unstable Algorithms:
When Stability Matters
Stability is important in many real-world situations. For instance, when sorting a list of students by their grades, you might want to keep the order of students with the same grade. This can help in maintaining the original order of entries in a database, which is crucial for accurate data representation.
Understanding whether a sorting algorithm is stable can help you choose the right one for your task. For example, if you need to sort data while keeping the original order of equal elements, a stable algorithm is essential.
Real-World Applications of Sorting Algorithms
Sorting algorithms are not just theoretical concepts; they have real-life applications in various fields. Here are some key areas where sorting algorithms play a crucial role:
Sorting in Databases
- Data Retrieval: Sorting helps in quickly retrieving records based on specific criteria, improving the efficiency of database queries.
- Indexing: Sorted data allows for faster searching and indexing, which is essential for large databases.
Sorting in Search Engines
- Ranking Results: Search engines use sorting algorithms to rank web pages based on relevance, ensuring users get the best results first.
- Data Organization: Sorting helps in organizing vast amounts of data, making it easier to access and analyze.
Sorting in E-Commerce
- Product Listings: E-commerce platforms sort products based on price, popularity, or ratings, enhancing user experience.
- Inventory Management: Sorting algorithms assist in managing stock levels and optimizing supply chains.
In many cases, the real-life applications of data structures and algorithms extend beyond computing, influencing everyday tasks and decisions.
Understanding these applications can help you appreciate the importance of sorting algorithms in both technical and non-technical fields.
Advanced Sorting Techniques
Tim Sort
Tim Sort is a hybrid sorting algorithm derived from Merge Sort and Insertion Sort. It is designed to perform well on many kinds of real-world data. This algorithm is used in Python’s built-in sort function, making it a practical choice for developers. Tim Sort has a time complexity of O(n log n) in the worst case and is stable, meaning it keeps the original order of equal elements.
Intro Sort
Intro Sort begins with Quick Sort and switches to Heap Sort when the recursion depth exceeds a certain level. This approach combines the fast average performance of Quick Sort with the worst-case performance of Heap Sort, ensuring efficiency across various data sets. It is particularly useful when you want to avoid the worst-case scenario of Quick Sort.
Dual-Pivot Quick Sort
Dual-Pivot Quick Sort is an optimized version of the traditional Quick Sort. Instead of using a single pivot, it uses two pivots to partition the array into three parts. This method can lead to better performance on average, especially with large datasets. It is the default sorting algorithm in Java for primitive types, showcasing its effectiveness in practical applications.
Understanding these advanced sorting techniques can significantly enhance your problem-solving skills in coding interviews. They not only improve your efficiency but also demonstrate your depth of knowledge in algorithms.
Algorithm | Best Case | Average Case | Worst Case | Space Complexity | When to Use |
---|---|---|---|---|---|
Tim Sort | O(n log n) | O(n log n) | O(n log n) | O(n) | When handling real-world data in Python. |
Intro Sort | O(n log n) | O(n log n) | O(n log n) | O(log n) | When you want to avoid Quick Sort’s worst case. |
Dual-Pivot Quick Sort | O(n log n) | O(n log n) | O(n^2) | O(log n) | When sorting large datasets in Java. |
Common Mistakes in Sorting Algorithm Interviews
Overlooking Time Complexity
One of the biggest mistakes candidates make is not paying attention to time complexity. When asked to sort data, many jump straight into sorting the entire array, which takes O(n log n) time. Instead, they should consider if a more efficient method exists, like Quick Select, which can find the kth smallest or largest element in O(n) average time. This is especially important for large datasets.
Not Using Heap Sort When Appropriate
Another common error is ignoring the benefits of Heap Sort. For example, if you’re tasked with finding the ‘k’ largest elements in an unsorted array, using a min heap of size k can help you achieve this in O(n log k) time. This is much better than sorting the entire array, which would take O(n log n) time.
Not Realizing the Patterns Emerging from a Sorted Array
Candidates often fail to see the patterns that arise from sorted arrays. Recognizing these patterns can simplify problems significantly. For instance, the two-pointer technique is very effective when working with sorted data. In problems like the "two-sum" challenge, using two pointers can lead to a solution in linear time, making it much faster than other methods.
Understanding these common mistakes can greatly improve your performance in coding interviews. By focusing on efficiency and recognizing patterns, you can demonstrate your problem-solving skills effectively.
Demonstrating Mastery in Interviews
Communicating Complexity
When discussing sorting algorithms in an interview, clarity is key. You should be able to explain the time and space complexities of the algorithms you choose. Use Big O notation to describe how the algorithm performs as the input size grows. This shows that you understand not just how to implement the algorithm, but also its efficiency.
Using Built-In Sort Functions
Many programming languages offer built-in sort functions. While it’s important to know how to implement sorting algorithms from scratch, using these built-in functions can save time during an interview. Just be ready to explain how they work under the hood and when to use them effectively.
Asking the Right Questions
During the interview, don’t hesitate to ask clarifying questions. This can help you understand the problem better and show your thought process. For example, you might ask:
- What is the size of the data set?
- Are there any constraints on the input?
- Should the sorting be stable?
Understanding sorting algorithms is crucial for coding interviews. It not only demonstrates your technical skills but also your ability to think critically about problem-solving.
Summary Table of Key Points
Key Aspect | Description |
---|---|
Clarity | Explain algorithms clearly using Big O notation. |
Built-In Functions | Know when to use built-in sort functions and their workings. |
Questions | Ask clarifying questions to understand the problem better. |
By mastering these aspects, you can effectively demonstrate your knowledge and skills in sorting algorithms during coding interviews. Remember, practice makes perfect!
Practice Problems for Sorting Algorithms
Easy Level Problems
- Sort an array of 0s, 1s and 2s: The task is to sort the array, i.e., put all 0s first, then all 1s, and all 2s last. This problem is the same as the famous "dutch national flag problem".
- Reverse the words in a string: Given a string, reverse the order of the words in it.
Medium Level Problems
- Kth Smallest Element: Given an integer array and an integer k, return the kth smallest element in the array.
- Subarray Sum Equals K: Given an unsorted array of integers and an integer k, find the number of subarrays whose sum equals k.
- Three Sum: Given an array of integers, return an array of triplets such that i != j != k and nums[i] + nums[j] + nums[k] = 0.
Hard Level Problems
- Alien Dictionary: You are given a list of lexicographically sorted words from an alien language. This language has a unique order. Return the alphabetical order of all the letters found in the list of words.
- Container with the Most Water: Given n non-negative integers, find two lines that form a container that can hold the most amount of water.
Practicing these problems will help you understand sorting algorithms better and prepare you for coding interviews. Focus on the logic behind each problem and how sorting can simplify your approach.
If you’re looking to sharpen your skills in sorting algorithms, check out our practice problems! They are designed to help you understand and apply what you’ve learned. Don’t miss out on the chance to boost your coding abilities—visit our website today and start coding for free!
Conclusion
In summary, mastering sorting algorithms is key for success in coding interviews. While it might seem overwhelming at first, focusing on a few essential algorithms like Merge Sort and Quick Sort can make a big difference. These algorithms not only help you solve problems efficiently but also show your understanding of important concepts in computer science. Remember, it’s not just about memorizing; it’s about knowing when and how to use these algorithms effectively. Good luck with your interview prep, and keep practicing!
Frequently Asked Questions
What are sorting algorithms?
Sorting algorithms are methods used to arrange data in a certain order, like from smallest to largest or alphabetically.
Why are sorting algorithms important for coding interviews?
Understanding sorting algorithms helps show your problem-solving skills and knowledge of how to optimize solutions.
What is the difference between comparison-based and non-comparison sorting algorithms?
Comparison-based sorting algorithms decide order by comparing elements, while non-comparison algorithms sort based on item distribution.
Can you name a few commonly used sorting algorithms?
Yes, some common sorting algorithms include Quick Sort, Merge Sort, and Heap Sort.
What does ‘in-place’ mean in sorting?
In-place sorting means sorting the data without needing extra space for another copy of the data.
What is the time complexity of Quick Sort?
The average time complexity of Quick Sort is O(n log n), but in the worst case, it can be O(n²).
What is a stable sorting algorithm?
A stable sorting algorithm maintains the relative order of records with equal keys, meaning it doesn’t change their positions.
How can I practice sorting algorithms for interviews?
You can practice sorting algorithms by solving problems on platforms like AlgoCademy or coding challenge websites.