Understanding What is CRUD: A Comprehensive Guide to Create, Read, Update, and Delete Operations
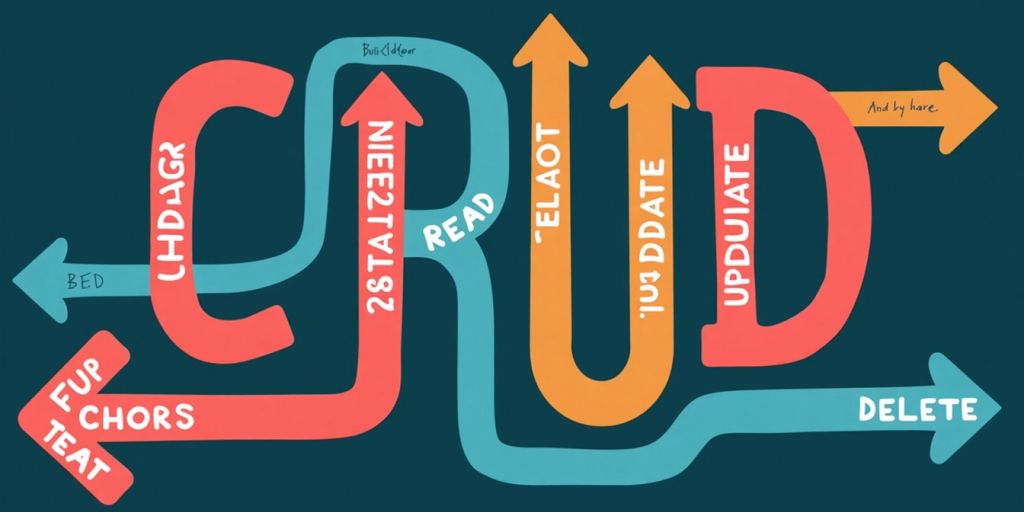
CRUD stands for Create, Read, Update, and Delete. These four actions are the basic building blocks for managing data in databases. Understanding CRUD is essential for anyone working with data, whether in software development, web applications, or even mobile apps. This guide will help you grasp the importance of CRUD operations and how they are applied in various systems.
Key Takeaways
- CRUD is an acronym for Create, Read, Update, and Delete, which are the main functions for managing data.
- These operations are essential for both SQL and NoSQL databases.
- Each CRUD operation corresponds to a specific action: creating new data, reading existing data, updating data, and deleting data.
- Implementing CRUD correctly is crucial for data integrity and application performance.
- Understanding CRUD is key for building user-friendly applications that interact seamlessly with databases.
Defining CRUD: The Backbone of Database Operations
CRUD is a simple acronym that stands for Create, Read, Update, and Delete. These four operations are the core functions that developers use to manage data in databases. Understanding CRUD is essential for anyone working with data, as it forms the foundation of how we interact with databases.
Origins and Meaning of CRUD
CRUD operations have been around since the early days of database management. They represent the basic actions that can be performed on data. Each operation plays a crucial role in how data is handled:
- Create: This operation adds new data.
- Read: This operation retrieves existing data.
- Update: This operation modifies existing data.
- Delete: This operation removes data.
The Role of CRUD in Databases
CRUD operations are vital for maintaining data integrity and ensuring that applications can effectively manage data. They allow users to:
- Add new records to a database.
- Retrieve and display information.
- Modify existing records as needed.
- Remove records that are no longer necessary.
CRUD in Different Database Systems
CRUD operations are used in various types of databases, including:
- Relational Databases: Such as MySQL and PostgreSQL, where data is organized in tables.
- NoSQL Databases: Such as MongoDB, which store data in a more flexible format.
Database Type | Example | CRUD Operations |
---|---|---|
Relational Database | MySQL | Yes |
NoSQL Database | MongoDB | Yes |
CRUD operations are the key principles that API developers and programmers follow while constructing robust APIs. They ensure that data is managed effectively and efficiently, making CRUD a fundamental concept in software development.
Breaking Down the CRUD Operations
CRUD operations are the essential actions that allow us to manage data in databases. Understanding these operations is crucial for anyone working with data. Let’s break down each operation:
Create Operation Explained
The Create operation is used to add new records to a database. This could mean creating a new user profile or adding a new product to a list. Here’s a simple example:
- Insert a new user into the database.
- Add a new product to the inventory.
Read Operation Explained
The Read operation allows us to fetch and view data from the database. This is often used to display information to users. For instance:
- Showing a list of products.
- Retrieving a user’s details.
Update Operation Explained
The Update operation is for modifying existing records. This could involve changing a user’s password or updating a product’s price. Here’s how it works:
- Change a user’s email address.
- Update the price of a product.
Delete Operation Explained
The Delete operation removes records from the database. This is used when we no longer need certain data. For example:
- Deleting a user account.
- Removing a product from the inventory.
CRUD operations are vital for maintaining data integrity and ensuring smooth interactions with databases.
In summary, CRUD operations are the backbone of database management, allowing us to create, read, update, and delete data effectively. Understanding these operations helps in building robust applications that can handle data efficiently.
Implementing CRUD in SQL
SQL Syntax for CRUD Operations
CRUD operations are essential for managing data in databases. In SQL, these operations are performed using specific commands:
- Create: To add new data, you use the
INSERT INTO
command. For example: - Read: To retrieve data, the
SELECT
command is used. For instance: - Update: To change existing data, you can use the
UPDATE
command: - Delete: To remove data, the
DELETE
command is applied:
Examples of CRUD in SQL
Here’s a quick summary of how CRUD operations look in SQL:
Operation | SQL Command |
---|---|
Create | INSERT INTO users (name, email, password) VALUES ('John Doe', 'john@example.com', 'securepassword'); |
Read | SELECT * FROM users WHERE email = 'john@example.com'; |
Update | UPDATE users SET password = 'newpassword' WHERE email = 'john@example.com'; |
Delete | DELETE FROM users WHERE email = 'john@example.com'; |
Best Practices for SQL CRUD Operations
To ensure smooth and secure CRUD operations, consider these best practices:
- Validation: Always check data before adding it to the database.
- Security: Use safe coding practices to prevent attacks like SQL injection.
- Performance: Optimize your queries for faster results.
- Transactional Integrity: Use transactions to keep data safe during multiple operations.
Understanding CRUD operations is crucial for anyone working with databases. CRUD operations refer to four essential functions — create, read, update, and delete — that manage data in databases or other storage systems.
By mastering these commands, you can effectively manage data in SQL databases, making your applications more efficient and reliable.
CRUD Operations in NoSQL Databases
CRUD operations in NoSQL databases can vary based on the specific database system being used. Understanding these operations is crucial for effective data management. Here’s a breakdown of how CRUD works in NoSQL databases:
Understanding NoSQL Databases
NoSQL databases are designed to handle large volumes of data and provide flexibility in data storage. They differ from traditional SQL databases in several ways:
- Schema-less: NoSQL databases often do not require a fixed schema, allowing for more dynamic data structures.
- Scalability: They can easily scale horizontally, making them suitable for big data applications.
- Variety of Data Models: NoSQL databases can be document-oriented, key-value stores, column-family stores, or graph databases.
CRUD in Document-Oriented Databases
In document-oriented databases like MongoDB, CRUD operations are performed using specific commands:
Operation | Command | Description |
---|---|---|
Create | db.collection.insertOne() or db.collection.insertMany() |
Adds new documents to a collection |
Read | db.collection.find() or db.collection.findOne() |
Retrieves documents from a collection |
Update | db.collection.updateOne() , db.collection.updateMany() , or db.collection.replaceOne() |
Modifies existing documents |
Delete | db.collection.deleteOne() or db.collection.deleteMany() |
Removes documents from a collection |
CRUD in Key-Value Stores
Key-value stores, such as Redis, use a simpler approach:
- Create: Add a new key-value pair.
- Read: Retrieve the value associated with a key.
- Update: Change the value of an existing key.
- Delete: Remove a key-value pair from the store.
In NoSQL databases, CRUD operations are often embedded within application code, allowing for dynamic data handling.
Understanding how CRUD works in NoSQL databases is essential for developers and database administrators. By mastering these operations, you can effectively manage and manipulate data in various applications.
CRUD in RESTful APIs
Mapping CRUD to HTTP Methods
In RESTful APIs, the CRUD operations are often linked to specific HTTP methods. Here’s how they typically match up:
CRUD Operation | HTTP Method | Description |
---|---|---|
Create | POST | Adds a new resource |
Read | GET | Retrieves existing resources |
Update | PUT | Modifies an existing resource |
Delete | DELETE | Removes a resource |
Understanding this mapping is crucial for building effective APIs. Each operation corresponds to a specific action that can be performed on the data.
Building RESTful APIs with CRUD
When creating a RESTful API, you will implement CRUD operations to manage resources. Here’s a simple outline of how to do this:
- Define your resources: Identify what data you will manage (e.g., users, products).
- Set up routes: Create endpoints for each CRUD operation (e.g.,
/users
,/products
). - Implement logic: Write the code to handle requests and perform the corresponding CRUD actions.
- Test your API: Ensure that each operation works as expected.
Security Considerations for CRUD in APIs
Security is vital when dealing with CRUD operations in APIs. Here are some key points to consider:
- Authentication: Ensure that only authorized users can access or modify resources.
- Data Validation: Always validate input data to prevent errors and security issues.
- Rate Limiting: Protect your API from abuse by limiting the number of requests a user can make.
Implementing these security measures helps protect your data and maintain the integrity of your application.
By understanding how CRUD operations fit into RESTful APIs, you can create robust and secure applications that effectively manage data.
Best Practices for Efficient CRUD Operations
To ensure that CRUD operations are both effective and secure, consider the following best practices:
1. Data Validation Techniques
- Always validate input data before performing any CRUD operations. This helps prevent invalid data from entering your database.
- Use built-in validation methods or libraries to streamline this process.
2. Ensuring Transactional Integrity
- Utilize transactions to maintain data integrity, especially when multiple related operations are involved. This ensures that either all operations succeed or none do.
- For example, if a user creates an order and updates inventory, both actions should either complete successfully or roll back if one fails.
3. Optimizing Performance
- Optimize your database queries and indexes to enhance the speed of CRUD operations. This can significantly improve user experience.
- Regularly analyze query performance and adjust as necessary.
4. Security Measures for CRUD Operations
- Protect against common vulnerabilities like SQL injection and XSS by using parameterized queries and input sanitization.
- Improved collaboration efficiency: Standardized CRUD practices and clear code structures enhance team collaboration efficiency.
Following these best practices not only improves the performance of your CRUD operations but also enhances the overall security and reliability of your applications.
Real-World Applications of CRUD
CRUD operations are everywhere in our daily tech interactions. They help manage data in various applications, making them essential for developers. Here are some key areas where CRUD is applied:
CRUD in Web Development
- User Accounts: When a user creates an account, the system uses the Create operation. Users can then read their profiles, update their information, or delete their accounts.
- E-commerce: In online stores, CRUD helps manage product listings. For example, when a new product is added, it’s created in the database. Customers can read product details, update their shopping carts, or delete items they no longer want.
CRUD in Mobile Applications
- Social Media: Users create posts, read others’ posts, update their status, and delete unwanted content. This interaction relies heavily on CRUD operations.
- Fitness Apps: Users can create workout plans, read their progress, update their goals, and delete old records.
CRUD in Enterprise Systems
- Customer Relationship Management (CRM): Businesses use CRUD to manage customer data. They create new customer records, read existing data, update contact information, and delete outdated records.
- Inventory Management: Companies track their stock using CRUD. They create new inventory entries, read stock levels, update quantities, and delete items that are no longer available.
Application Area | CRUD Operations Involved |
---|---|
Web Development | Create accounts, read profiles, update info, delete accounts |
Mobile Applications | Create posts, read updates, update goals, delete records |
Enterprise Systems | Create customer records, read data, update info, delete records |
Understanding CRUD operations is crucial for building effective applications. They form the backbone of data management in various systems, ensuring smooth user experiences and efficient data handling.
CRUD operations are essential in many real-life situations, from managing your favorite apps to organizing data in businesses. They help you create, read, update, and delete information easily. If you want to learn more about how to apply these skills in your coding journey, visit our website today!
Conclusion
In summary, CRUD operations are key to working with databases in software development. Knowing how to create, read, update, and delete data is essential for building strong and effective applications. By mastering these operations, developers can keep their data safe, ensure it works well, and provide a better experience for users. Understanding CRUD not only helps in managing data but also lays the groundwork for more advanced programming concepts.
Frequently Asked Questions
What does CRUD stand for?
CRUD stands for Create, Read, Update, and Delete. These are the main actions you can do with data in a database.
Why are CRUD operations important?
CRUD operations are important because they help manage data in a way that keeps it organized and easy to use.
How do you perform a Create operation?
To perform a Create operation, you add new data to the database, like creating a new user profile.
What is the Read operation?
The Read operation is when you look at or retrieve data from the database, like checking a list of users.
How can you update data in a database?
You can update data by changing existing information, like updating a user’s email address.
What does the Delete operation do?
The Delete operation removes data from the database, such as deleting a user account.