10 Essential Tips to Learn Programming Effectively
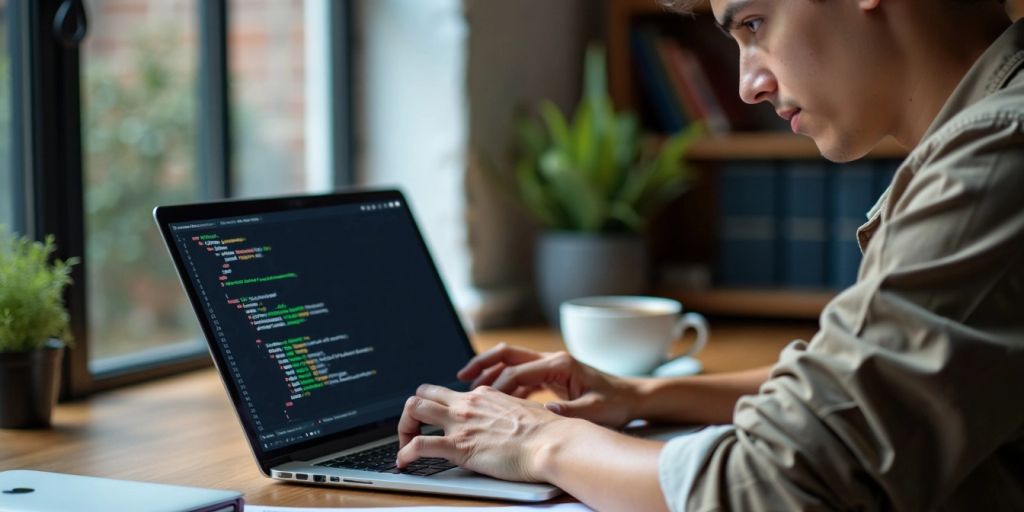
Learning to program can feel overwhelming, especially if you’re just starting out. However, with the right approach and mindset, you can make the process smoother and more enjoyable. Here are ten essential tips to help you learn programming effectively and build a strong foundation for your coding journey.
Key Takeaways
- Always plan your project before you start coding to avoid mistakes.
- Select tools and languages that fit your project and skill level.
- Write clean and easy-to-read code to help yourself and others.
- Understand data structures and algorithms to improve your coding skills.
- Practice coding regularly to become a better programmer.
1. Plan Before You Code
Before you start typing away at your keyboard, it’s important to have a clear plan. Many new programmers make the mistake of jumping straight into coding without thinking things through. Here’s why planning is crucial:
- Understand the Problem: Take time to figure out what you are trying to solve. Write down the problem in simple terms.
- Break It Down: Divide the problem into smaller, manageable parts. This makes it easier to tackle each piece one at a time.
- Design a Solution: Sketch out how you want your program to work. This could be in the form of flowcharts or simple diagrams.
Benefits of Planning
Benefit | Description |
---|---|
Saves Time | Planning helps you avoid mistakes that waste time later. |
Reduces Errors | A clear plan can help you spot potential issues before coding. |
Improves Focus | Knowing what to do next keeps you on track. |
Taking the time to plan can make your coding journey smoother and more enjoyable.
By following these steps, you’ll set yourself up for success and make your coding experience much more effective. Remember, a little planning goes a long way!
2. Choose The Right Tools
Choosing the right tools for programming is essential for your success. Using the right programming language and software can make a big difference in how efficiently you work. Here are some key points to consider when selecting your tools:
- Project Requirements: Understand what your project needs. Some languages are better suited for specific tasks.
- Community Support: A strong community can help you solve problems and learn faster. Look for languages with active forums and resources.
- Familiarity: If you already know a language, it might be easier to stick with it for new projects.
Popular Programming Languages and Their Uses
Language | Best For | Community Support |
---|---|---|
Python | Data Science, Web Development | High |
JavaScript | Web Development | Very High |
Java | Mobile Apps, Enterprise | High |
C++ | Game Development | Moderate |
Remember, the tools you choose can shape your learning experience. Take the time to explore different options and find what works best for you.
In addition to programming languages, consider using tools like code editors, version control systems, and online resources. These can enhance your coding experience and help you learn more effectively. For example, there are 15 free tools to learn coding basics that cover languages like HTML, CSS, Python, and more. Using these tools can provide a solid foundation for your programming journey.
3. Keep Your Code Clean And Readable
Readable code is crucial for maintaining and updating your projects. Following best practices for keeping your code clean can make a big difference. Here are some key points to consider:
- Use consistent naming conventions: This helps others understand your code better.
- Write meaningful names: Choose names that describe what the variable or function does.
- Declare variables appropriately: Make sure to use the right type for your variables.
Proper indentation and comments are also important. They help you and others read the code easily. Remember, you will spend more time reading code than writing it!
Keeping your code clean not only helps you but also makes it easier for others to work with your code in the future.
4. Learn Data Structures And Algorithms
Understanding data structures and algorithms (DSA) is crucial for writing efficient code. They help you organize and manage data effectively, which is essential for creating optimized software solutions. Here are some key points to consider:
Why Learn DSA?
- Improves problem-solving skills: Knowing DSA helps you tackle complex problems more easily.
- Enhances coding efficiency: You can write faster and more efficient code.
- Prepares for interviews: Many tech interviews focus on DSA questions.
Key Data Structures
Data Structure | Description |
---|---|
Arrays | A collection of items stored at contiguous memory locations. |
Linked List | A sequence of nodes where each node points to the next. |
Stack | A collection of elements that follows the Last In First Out (LIFO) principle. |
Queue | A collection that follows the First In First Out (FIFO) principle. |
Tree | A hierarchical structure with nodes connected by edges. |
Graph | A collection of nodes connected by edges, useful for representing networks. |
Important Algorithms
- Sorting Algorithms: Organize data in a specific order (e.g., Bubble Sort, Quick Sort).
- Searching Algorithms: Find specific data within a structure (e.g., Linear Search, Binary Search).
- Dynamic Programming: Break down problems into simpler subproblems to optimize performance.
Learning DSA is not just about memorizing; it’s about understanding how to apply these concepts in real-world scenarios.
By mastering data structures and algorithms, you will be better equipped to write efficient code and solve complex problems effectively.
5. Embrace Version Control
Version control is a crucial part of programming. Using tools like Git helps you manage your code effectively. With version control, you can track changes, collaborate with others, and easily go back to earlier versions if needed. This is especially important when working in teams, as it helps to organize workflows and keep your codebase secure.
Benefits of Version Control
- Collaboration: Multiple people can work on the same project without overwriting each other’s changes.
- Backup: You can restore previous versions of your code if something goes wrong.
- History: You can see the history of changes made to your code, which helps in understanding how it evolved.
Common Version Control Systems
System | Description |
---|---|
Git | A widely used system that allows for local and remote repositories. |
SVN | A centralized version control system that is less common today. |
Mercurial | A distributed version control system similar to Git. |
Embracing version control not only improves your coding skills but also prepares you for real-world programming challenges.
In summary, learning version control is essential for any programmer. It enhances collaboration, keeps your work organized, and provides a safety net for your code.
6. Test Early And Often
Testing your code is a crucial step in programming. Catching bugs early can save you a lot of time and effort later. Here are some key points to remember:
- Run tests frequently: Don’t wait until the end of your project to test your code. Testing often helps you find issues sooner.
- Automate your tests: Use tools that can run tests automatically. This makes it easier to check your code regularly.
- Follow best practices: Here are some unit testing best practices for an effective QA strategy:
- Run tests early and often
- Remove redundancy in your tests
- Name your tests effectively
- Use deterministic testing
- Follow the AAA (Arrange, Act, Assert) pattern
- Automate your testing process
Testing is not just a final step; it’s part of the coding process. By integrating testing into your workflow, you can improve the quality of your code and your overall programming skills.
7. Document Your Code
Documentation is often overlooked, but it plays a vital role in understanding your code. Writing clear comments and creating user-friendly README files can save you and others a lot of time later. Here are some key points to consider:
- Use clear comments: Explain what your code does, especially for complex sections.
- Create README files: Provide an overview of your project, how to set it up, and how to use it.
- Generate documentation: Use tools to create structured documentation automatically.
Documentation Type | Purpose | Tools |
---|---|---|
Comments | Explain code logic | Inline comments |
README files | Project overview | Markdown, GitHub |
Generated docs | Structured info | Doxygen, Sphinx |
Remember, good documentation helps others understand your work and makes it easier for you to revisit your code later. Master code documentation with essential steps, examples, best practices, and tools to streamline your process effectively.
8. Stay Updated
In the fast-changing world of technology, keeping your skills fresh is crucial. Here are some ways to stay informed:
- Read Blogs and Articles: Follow popular programming blogs and websites to learn about the latest trends.
- Join Online Communities: Engage with other programmers on platforms like Reddit or Stack Overflow.
- Take Online Courses: Websites like Coursera and Udemy offer courses on new technologies.
- Attend Conferences: Participate in tech conferences to network and learn from experts.
Resource Type | Examples |
---|---|
Blogs | Medium, Dev.to |
Online Courses | Coursera, Udemy |
Communities | Reddit, Stack Overflow |
Conferences | PyCon, JavaOne |
Staying curious and exploring new resources will help you grow as a programmer. By following blogs, joining communities, taking courses, and staying curious, you can stay informed and continually improve your skills.
9. Seek Feedback And Collaborate
Getting input from others is a key part of learning programming. Working with others can help you improve your skills. Here are some ways to seek feedback and collaborate effectively:
- Ask for Code Reviews: Share your code with peers or mentors and ask for their thoughts. They might spot mistakes you missed or suggest better ways to do things.
- Pair Programming: This is a method where two programmers work together at one computer. One writes the code while the other reviews it in real-time. This can lead to better solutions and faster learning.
- Join Coding Communities: Online forums and local meetups are great places to connect with other programmers. You can share your work, ask questions, and learn from others’ experiences.
Method | Benefits |
---|---|
Code Reviews | Get constructive feedback on your code. |
Pair Programming | Learn from each other in real-time. |
Coding Communities | Network and share knowledge with others. |
Collaborating with others not only helps you learn faster but also makes coding more enjoyable.
By seeking feedback and working with others, you can grow as a programmer and build a strong network in the tech community.
10. Practice, Practice, Practice
When it comes to learning programming, the key is to practice regularly. Many beginners make the mistake of only reading or watching tutorials without actually coding. Just like in math, where you can’t solve problems by just memorizing formulas, programming requires hands-on experience.
Why Practice is Important
- Builds Confidence: The more you code, the more comfortable you become.
- Improves Problem-Solving Skills: Regular practice helps you think critically and solve problems faster.
- Familiarizes You with Syntax: Coding often means remembering specific rules and commands. Practicing helps you remember them better.
Tips for Effective Practice
- Start Small: Begin with simple projects or coding challenges.
- Set Goals: Aim to complete a certain number of coding problems each week.
- Use Online Platforms: Websites like LeetCode and HackerRank offer great challenges to improve your skills.
- Work on Personal Projects: Think of a project you’d like to create and start coding it.
Remember, consistent practice is essential. It’s not just about quantity but also about quality. Focus on understanding the logic behind your code.
By making practice a regular part of your learning, you’ll find that programming becomes easier and more enjoyable. Don’t skip the exercises, even if they seem simple. Every bit of practice counts!
To truly get better at coding, you need to practice a lot. The more you code, the more confident you’ll become. Don’t wait any longer! Visit our website to start your coding journey for free and unlock your potential today!
Conclusion
Learning to program can be tough, but it’s also very rewarding. By using these ten important tips, you can improve your coding skills and write better code. Remember, becoming a great programmer takes time and practice. Stay patient, keep trying, and be open to new ideas. Enjoy your coding journey!
Frequently Asked Questions
What should I do before I start coding?
It’s important to plan first. Understand the problem, break it into smaller parts, and create a solution before you start coding.
How do I pick the best programming tools?
Choose tools based on your project needs, community support, and how comfortable you are with them.
Why is clean code important?
Clean code is easier to read and maintain. Use clear names for variables and functions, and keep your code tidy.
What are data structures and algorithms?
They are ways to organize and process data. Knowing them helps you write better and faster code.
What is version control?
It’s a system that helps you keep track of changes in your code. Git is a popular tool for this.
Why should I test my code?
Testing helps find bugs and ensures that your code works as it should. It’s good to test often.
How can I document my code?
Write comments to explain what your code does and create user-friendly guides so others can understand it.
How do I keep my skills up to date?
Read blogs, books, and attend events related to programming to stay informed about new trends and tools.