Debug Like a Pro/ Solving 20 Common Coding Errors
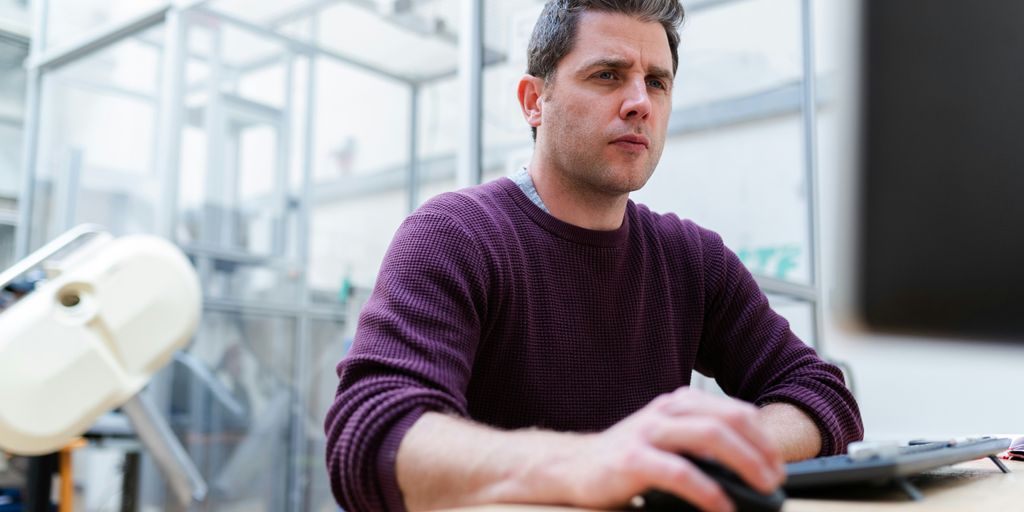
Every programmer, no matter how experienced, will face bugs. Debugging is a crucial skill that separates good developers from great ones. It’s not just about fixing errors—it’s about understanding your code deeply and improving your problem-solving skills. In this article, we’ll explore effective strategies to tackle 20 common coding errors and debug like a pro.
Key Takeaways
- Stay calm and don’t rush. A clear mind finds bugs faster.
- Reproduce the bug consistently to understand its cause.
- Use print statements wisely to track your code’s behavior.
- Learn to use a debugger for more efficient problem-solving.
- Keep track of common bugs to avoid repeating mistakes.
Stay Calm and Don’t Rush
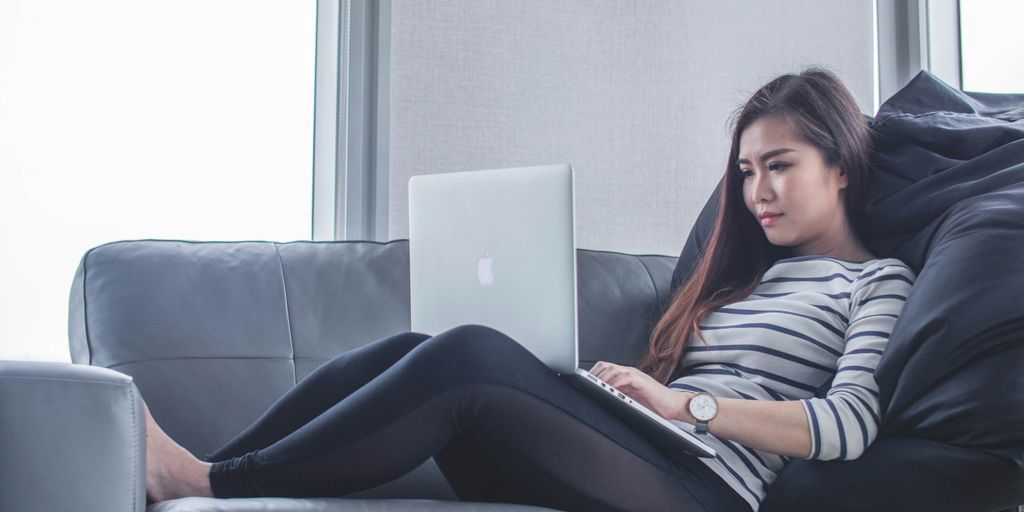
The first and most important rule of debugging is to stay calm. It’s easy to get frustrated when your code doesn’t work as expected, but jumping to conclusions or making rushed fixes often leads to more problems. Take a deep breath and approach debugging with a clear and methodical mindset. Bugs are puzzles to be solved, not roadblocks to be feared. By maintaining a calm attitude, you’re more likely to think clearly, spot patterns, and identify the root cause of the issue.
Reproduce the Bug Consistently
Before diving into the code, make sure you can reproduce the bug consistently. This is crucial for pinpointing the cause and verifying the fix later on. Ask yourself:
- What specific input or actions cause the bug to appear?
- Under what conditions does the bug not occur?
Reproducing the bug reliably gives you a concrete test case. Once you have that, the rest of the process becomes clearer.
Sometimes, just identifying the exact circumstances is enough to set off the lightbulb, especially if you’re familiar with the system and just wrote the offending code a few minutes ago.
Occasionally, bugs are intermittent, making them a developer’s worst nightmare. In such cases, use tools to simulate the load and conditions under which the bug was reported, such as JMeter for load testing or Chaos Monkey for introducing faults. This helps in reproducing those tricky, random bugs.
Use Print Statements Wisely
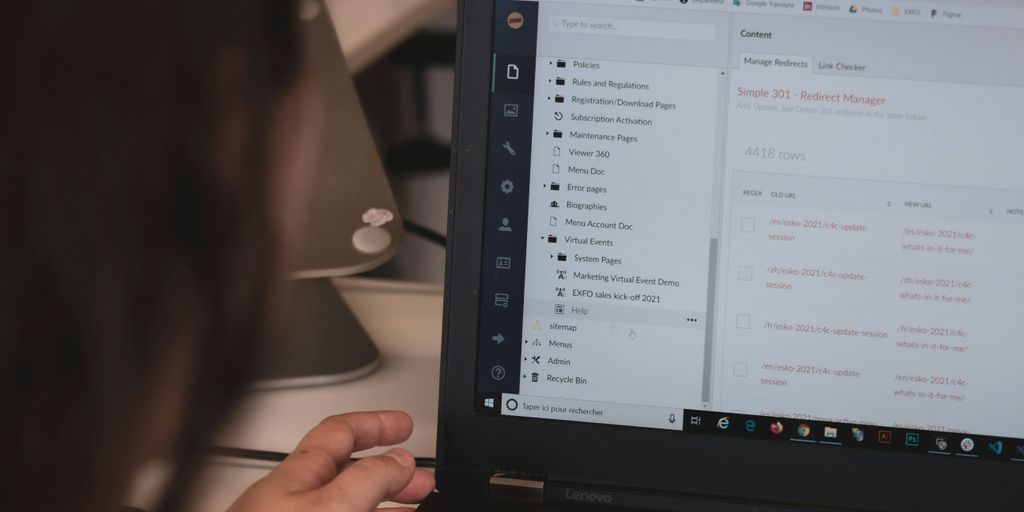
Using print statements is a classic yet effective way to debug your code. Print statement debugging helps you trace the behavior of a program to understand where an error occurred and how to fix it. However, it’s important to use them wisely.
Instead of scattering print statements randomly, place them strategically at critical points:
- Before and after complex functions or loops.
- At variable assignment points to verify that the right values are being passed.
A good practice is to print both the data and context, so you know what part of the code the output corresponds to. For example:
std::cout << "Entering function foo(int x) where x = " << x << std::endl;
A bad example would be:
std::cout << "Here" << std::endl;
While this approach may feel basic, it’s highly effective when combined with more sophisticated tools like debuggers.
Learn to Use a Debugger
Every programming environment offers debugging tools, but not every developer uses them to their full potential. Whether you’re coding in C++, Python, or JavaScript, learning to navigate the debugger in your chosen IDE can be a game-changer.
Mastering the debugger can provide far more detailed insights without altering your code.
Here are some features that can make your debugging process more efficient:
- Breakpoints: Pause code execution at specific lines to inspect variables and program state.
- Step-by-step execution: Move through your code one line at a time to understand exactly how it’s being executed.
- Variable watches: Monitor specific variables to see how their values change throughout the program.
Many developers rely on print statements for debugging because they feel quicker, but mastering the debugger can provide far more detailed insights without altering your code.
Check Your Assumptions
When debugging, it’s easy to overlook the basics. Check your assumptions about how your code should work. Often, we get stuck because we’ve marked the wrong spot as the problem area.
- Are you sure that function is returning the correct value?
- Is that variable initialized properly?
- Did you consider all edge cases?
A great debugging habit is to review your assumptions and validate them against actual behavior. Sometimes the bug isn’t where you’re looking — it could be a small oversight elsewhere in the code.
When debugging, take a step back and consider your strategy. This can help you see the problem from a new angle and find the real issue.
Simplify the Problem
When you’re facing a complex bug, try simplifying the code to isolate the issue. If you’re working with a large, complicated function or module, break it down into smaller components or comment out sections that aren’t immediately relevant to the bug.
By narrowing the focus, you reduce the surface area for errors, making it easier to spot the problem. Once you’ve identified the root cause, you can reintroduce the other parts and ensure everything works together seamlessly.
Use Version Control for Debugging
Version control systems like Git are not just for collaboration; they are also powerful tools for debugging. When you encounter a tricky bug, using version control can be a game-changer.
Git Bisect
One of the most useful commands in Git for debugging is git bisect
. This command helps you find out which commit introduced a bug by performing a binary search through your project history. This way, you can quickly identify the problematic code.
Create Debugging Branches
Instead of making changes directly to your main codebase, create a temporary branch where you can experiment and track your fixes. Once you solve the issue, you can merge the fix back into your main branch.
Using version control as part of your debugging process keeps your code organized and ensures that you always have a working version to fall back on.
Using a version control tool allows developers to find errors fast, roll back to a previous version, and correct the problem, mitigating the impact of bugs.
Get a Fresh Perspective
Sometimes, you can stare at code for hours and still miss something obvious. This is when getting a fresh pair of eyes on the problem can help. Don’t hesitate to ask a colleague or post a question online for a different perspective. Explaining the issue to someone else often clarifies your own thought process and may lead you to the solution.
Additionally, stepping away from the code for a while can work wonders. Once, when I had a difficult bug I decided to stop banging my head against the keyboard and go on a run. When I came back I had clarity and found the bug quickly! When you return with fresh eyes, you’ll often see the problem more clearly.
Keep Track of Common Bugs
Keeping a record of common bugs can save you a lot of time and effort in the long run. By documenting recurring issues, you can quickly identify and fix them when they appear again. Here are some tips to help you keep track of common bugs:
- Create a Bug Log: Maintain a log of all the bugs you encounter. Include details like the error message, the part of the code where it occurred, and how you fixed it.
- Categorize Bugs: Group similar bugs together. This can help you spot patterns and common causes.
- Use Bug Tracking Tools: Tools like Bugzilla can help you manage and track bugs efficiently. These tools allow you to assign bugs to team members, set priorities, and track the status of each bug.
- Review and Update Regularly: Regularly review your bug log and update it with new information. This will help you stay on top of recurring issues and prevent them from happening again.
Keeping a detailed record of bugs not only helps in fixing them faster but also improves your overall coding skills. By understanding the common issues in your code, you can write better and more robust code in the future.
Practice Debugging
Like any other skill, debugging improves with practice. Don’t wait until you encounter bugs in your own code — practice by solving debugging challenges or contributing to open-source projects where bugs are common. Another way is to leverage GPT, ask it to give you bad code for you to fix and have it grade your solution! By consistently practicing, you’ll build intuition and speed up your debugging process over time.
Debugging is a skill that gets better the more you do it. Keep practicing and you’ll find yourself solving problems faster and more efficiently.
Debugging is a crucial skill for any coder. It helps you find and fix errors in your code, making your programs run smoothly. If you want to get better at debugging, check out our interactive coding tutorials. They guide you step-by-step, showing you exactly where your code needs improvement. Start your journey to becoming a coding pro today!
Conclusion
Debugging is a crucial skill for any programmer, and mastering it can make your coding journey much smoother. Remember, every bug is an opportunity to learn and grow. By staying calm, methodically reproducing issues, and using the right tools and techniques, you can tackle even the most stubborn bugs. Keep practicing, stay curious, and don’t be afraid to ask for help when needed. With these strategies, you’ll not only fix errors more efficiently but also become a more proficient and confident developer. Happy debugging!
Frequently Asked Questions
Why is it important to stay calm while debugging?
Staying calm helps you think clearly and avoid making rushed fixes that might cause more problems.
How can I reproduce a bug consistently?
Identify the specific inputs or actions that trigger the bug and the conditions under which it does not occur.
When should I use print statements for debugging?
Use print statements to track the flow of execution and verify variable values at critical points in your code.
What are the benefits of using a debugger?
A debugger allows you to pause execution, inspect variables, and step through your code line by line, providing detailed insights without altering your code.
Why should I question my assumptions during debugging?
Bugs often arise from incorrect assumptions about how your code should work. Questioning these assumptions can help you identify the real issue.
How can simplifying the problem help in debugging?
Breaking down complex code into smaller parts can make it easier to isolate the issue and identify the root cause.
What is the role of version control in debugging?
Version control helps you keep track of changes, create debugging branches, and use tools like ‘git bisect’ to identify problematic code.
Why is it useful to keep a log of common bugs?
Keeping a log helps you recognize patterns, remember past solutions, and avoid making the same mistakes in the future.