Demystifying Data Structures and Algorithms for Coding Newbies
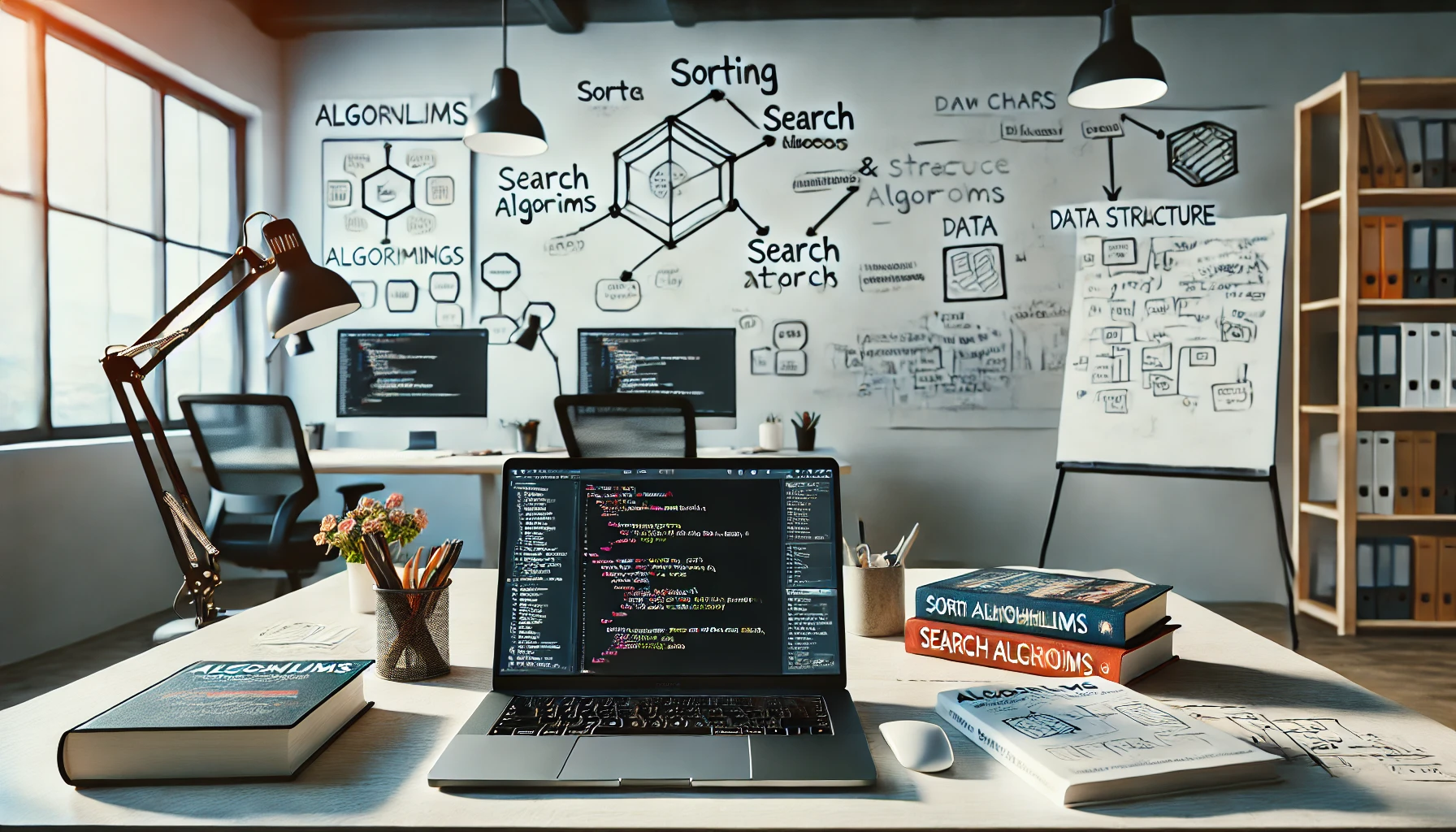
Starting with programming can feel like solving a big puzzle. Data structures and algorithms are key pieces of this puzzle. They help you store, organize, and use data efficiently. This guide will break down these concepts, making them easier to understand for beginners.
Key Takeaways
- Data structures help in organizing and managing data efficiently.
- Algorithms are step-by-step instructions to solve problems.
- Choosing the right data structure and algorithm can improve program performance.
- Understanding Big O notation is crucial for analyzing algorithm efficiency.
- Practice and real-world applications are essential for mastering these concepts.
Understanding the Basics of Data Structures
Defining Data Structures
Data structures are the fundamental building blocks of computer programming. They define how data is organized, stored, and manipulated within a program. Think of them as the architects of your code, dictating how information is stored, retrieved, and manipulated.
Importance in Programming
Data structures are crucial in programming because they provide a way to manage large amounts of data efficiently. They help in optimizing performance and ensuring that your code runs smoothly. Without proper data structures, even simple tasks can become complex and slow.
Common Types of Data Structures
There are several common types of data structures, each with its own unique characteristics and uses:
- Arrays: Simple and efficient for accessing elements by index, ideal for static collections.
- Linked Lists: Useful for dynamic data sets where frequent insertions and deletions occur.
- Stacks and Queues: Essential for LIFO (Last In, First Out) and FIFO (First In, First Out) operations, respectively.
- Trees: Hierarchical structures like binary trees and AVL trees, vital for efficient searching and sorting.
- Graphs: Represent networks of nodes and edges, crucial for algorithms in networking, social networks, and more.
- Hash Tables: Provide fast access to data through key-value pairs, commonly used in database indexing.
Understanding these basic data structures is the first step in becoming a proficient programmer. They are the tools that will help you solve complex problems and write efficient code.
Introduction to Algorithms
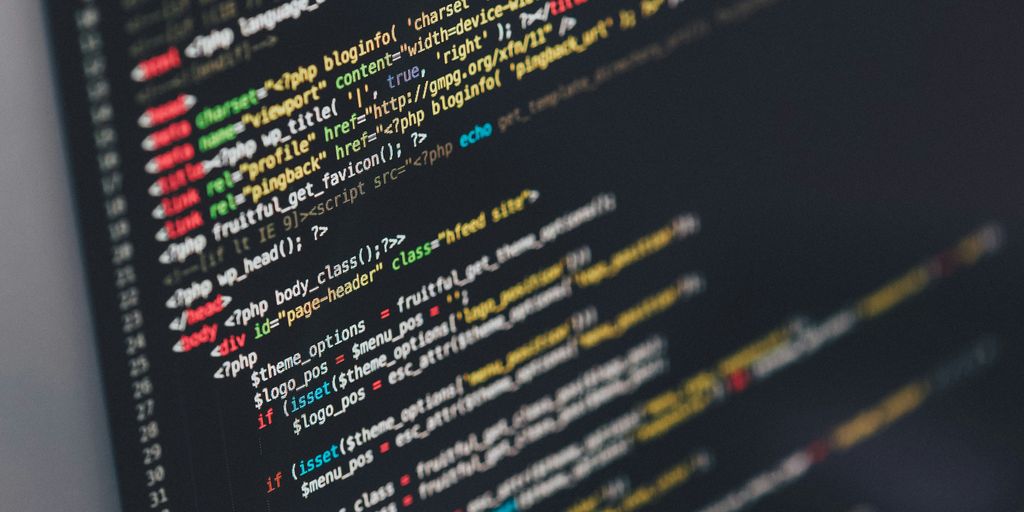
What Are Algorithms?
At its core, an algorithm is just a set of instructions that tells a computer how to perform a task. Think of it like a recipe in a cookbook. The recipe (algorithm) tells you the steps to follow to bake a cake (solve a problem). You gather your ingredients (data), follow the instructions (process), and voila! You have your cake (solution). An algorithm is a set of commands that must be followed for a computer to perform calculations or other problem-solving operations.
Types of Algorithms
Algorithms come in many types, each suited for different tasks. Here are a few common ones:
- Sorting Algorithms: These arrange data in a particular order. Examples include Bubble Sort, Merge Sort, and Quick Sort.
- Searching Algorithms: These help find specific data within a structure. Examples include Linear Search and Binary Search.
- Graph Algorithms: These work on graph data structures. Examples include Depth-First Search (DFS) and Breadth-First Search (BFS).
- Dynamic Programming Algorithms: These solve problems by breaking them down into simpler subproblems. Examples include the Fibonacci sequence and the Knapsack problem.
Role in Problem Solving
Algorithms are essential for solving computational problems efficiently. They help in optimizing performance and making the best use of resources. By choosing the right algorithm for a given problem, you can achieve optimal performance and solve complex tasks with ease.
Understanding algorithms is like learning the secret to solving puzzles. Once you know the steps, even the most complex problems become manageable.
Exploring Arrays and Linked Lists
Characteristics of Arrays
Arrays are like a row of boxes, each holding a piece of data. They are great for storing linear data of similar types. Arrays are efficient for accessing elements by their index. However, they have a fixed size, which means you can’t add more elements once it’s full.
Advantages of Linked Lists
Linked lists are like a chain of pearls, where each pearl is a node holding data. Unlike arrays, linked lists can grow and shrink as needed. This makes them flexible for dynamic data sets. They also make it easy to insert and delete elements without shifting other elements around.
Use Cases in Programming
- Arrays are ideal for tasks where you need quick access to elements, like in lookup tables.
- Linked lists are useful when you need a data structure that can grow and shrink, like in a playlist of songs.
Both arrays and linked lists can be used to store linear data of similar types, but they both have some advantages and disadvantages.
Diving into Stacks and Queues
Understanding Stacks
A stack is a linear data structure that follows a particular order in which the operations are performed. The order may be Last-In-First-Out (LIFO). Imagine a stack of plates; the last plate you put on top is the first one you take off. This structure is useful for tasks like managing function calls or undo operations in software.
Exploring Queues
Queues, on the other hand, follow the First-In-First-Out (FIFO) principle. Think of a line at a grocery store: the first person in line is the first to be served. Queues are great for handling tasks in sequence, like printing documents or processing tasks in a multi-threaded environment.
Applications in Real-World Scenarios
Both stacks and queues are essential for bringing order to chaos. They streamline processes in various applications:
- Function Call Management: Stacks help manage function calls in programming languages.
- Task Scheduling: Queues are used in operating systems to manage tasks.
- Undo Mechanisms: Stacks are used to implement undo features in software.
- Print Queue Management: Queues manage the order of print jobs in printers.
Understanding these structures can make your code more efficient and easier to manage.
Tree Data Structures Explained
Binary Trees
A tree data structure is a hierarchical structure that is used to represent and organize data in a way that is easy to navigate and search. Binary trees are a type of tree where each node has at most two children, often referred to as the left and right child. This structure is particularly useful for searching and sorting operations.
Balanced Trees
Balanced trees, such as AVL trees and Red-Black trees, maintain their height to ensure operations like insertion, deletion, and lookup are efficient. These trees automatically adjust their structure to remain balanced, which helps in keeping the operations fast.
Use Cases and Applications
Tree structures are widely used in various applications:
- File Systems: Organize files and directories in a hierarchical manner.
- Databases: Implement indexing mechanisms to speed up data retrieval.
- Networking: Manage routing information efficiently.
Trees are vital for file systems and databases in the tech realm, nurturing efficient data organization.
Graph Data Structures and Their Uses
Graphs are like intricate networks, similar to social media connections or city maps. Each node represents a point of interest, linked by edges that show relationships. Graphs are essential for representing complex relationships between different entities.
Understanding Graphs
A graph data structure is a collection of nodes connected by edges. It’s used to represent relationships between different entities. Graphs can be either directed, with one-way connections, or undirected, with two-way connections.
Types of Graphs
Graphs come in two main types:
- Directed Graphs: These have edges with a direction, like one-way streets.
- Undirected Graphs: These have edges without direction, like two-way streets.
Real-World Applications
Graphs are used in many real-world scenarios, such as:
- Social Networks: Representing connections between people.
- Navigation Systems: Mapping routes and locations.
- Recommendation Engines: Suggesting products or content based on user preferences.
Graph algorithms, like Breadth-First Search (BFS) and Depth-First Search (DFS), help in exploring and analyzing these networks efficiently.
Hash Tables and Their Importance
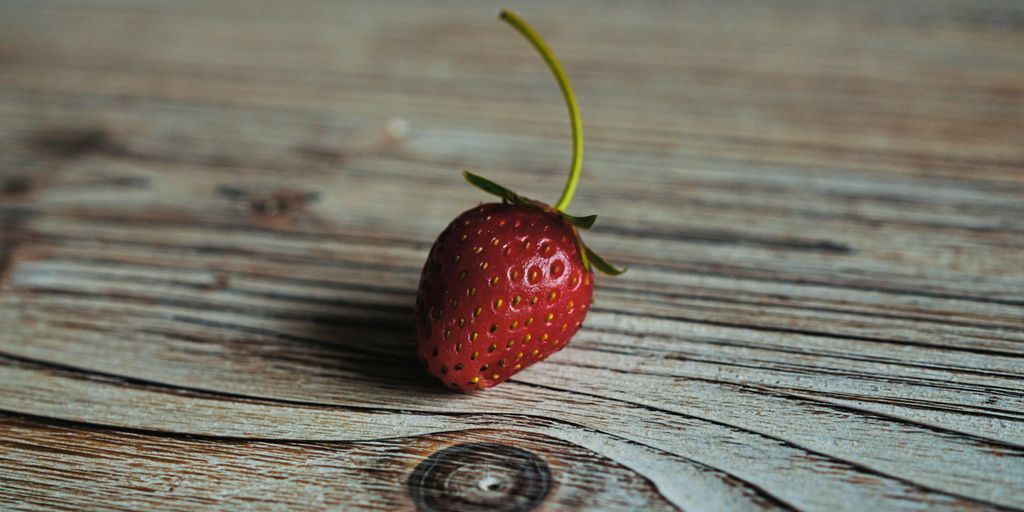
What Are Hash Tables?
A hash table is a data structure that stores data in key-value pairs. It uses a hash function to compute an index, also called a hash code, into an array of buckets or slots, from which the desired value can be found. This makes data retrieval very fast.
How Hash Tables Work
Hash tables work by transforming keys into storage locations using a hash function. Here’s a simple breakdown:
- Hash Function: Converts the key into a hash code.
- Index Calculation: The hash code is used to find an index in an array where the value is stored.
- Collision Handling: If two keys hash to the same index, techniques like chaining or open addressing are used to resolve the conflict.
Common Use Cases
Hash tables are widely used in various applications due to their efficiency. Some common use cases include:
- Database Indexing: Quickly locate data without scanning the entire database.
- Caches: Store frequently accessed data for rapid retrieval.
- Symbol Tables: Used in compilers to keep track of variables and functions.
Hash tables are essential for scenarios where fast data retrieval is crucial, making them a cornerstone in computer science.
Algorithmic Complexity and Big O Notation
Understanding Big O Notation
Algorithmic complexity, often expressed using Big O notation, measures how efficient an algorithm is in terms of time and space. Here are some key complexities:
- O(1): Constant time, where the operation’s time remains the same regardless of input size.
- O(n): Linear time, where the operation’s time grows linearly with input size.
- O(log n): Logarithmic time, efficient for operations that halve the problem size each step, like binary search.
- O(n log n): Log-linear time, typical of efficient sorting algorithms like mergesort and heapsort.
- O(n²): Quadratic time, common in simpler sorting algorithms like bubble sort and selection sort, less efficient for large datasets.
Time Complexity
Time complexity tells us how the runtime of an algorithm changes as the input size grows. It’s crucial for understanding the upper bound or worst-case scenario of an algorithm’s runtime.
Space Complexity
Space complexity measures the amount of memory an algorithm needs to run as the input size increases. This helps in determining how much extra space is required for the algorithm to execute efficiently.
Understanding both time and space complexity is essential for writing efficient code and optimizing performance.
Choosing the Right Data Structure
Factors to Consider
When selecting a data structure, it’s like picking the right tool for a job. You need to think about what you need to do. Here are some key factors to consider:
- Data Size: How much data will you handle?
- Operations: What operations will you perform most often (e.g., searching, inserting, deleting)?
- Memory Usage: How much memory can you use?
- Speed Requirements: How fast do you need your operations to be?
Balancing Time and Space
Balancing time complexity and memory usage is crucial. Sometimes, you might need to use more memory to make your program run faster. Other times, you might need to save memory, even if it makes your program slower. Finding the right balance is key.
Examples of Optimal Choices
Here are some examples of choosing the right data structure for different tasks:
- Arrays: Great for quick access to elements by index.
- Linked Lists: Ideal for frequent insertions and deletions.
- Hash Tables: Perfect for fast data retrieval using keys.
- Trees: Useful for hierarchical data and quick searches.
- Graphs: Best for representing networks and relationships.
Remember, selecting the right data structure can make your code run smoothly and efficiently. It’s a quest for the perfect fit, ensuring your code runs seamlessly and efficiently.
Best Practices for Implementing Data Structures
Writing Efficient Code
When writing code, always aim for efficiency. Efficient code runs faster and uses fewer resources. Here are some tips to help you write efficient code:
- Choose the right data structure for the task.
- Avoid unnecessary computations and loops.
- Use built-in functions and libraries when possible.
Testing and Debugging
Testing and debugging are crucial steps in the coding process. They help you find and fix errors, ensuring your code works as expected. Follow these steps:
- Write test cases for different scenarios.
- Use debugging tools to step through your code.
- Check for edge cases and handle them appropriately.
Regular testing and debugging can save you a lot of time and headaches in the long run.
Maintaining Readability
Readable code is easier to understand and maintain. Here are some tips to keep your code clean and readable:
- Use meaningful variable and function names.
- Add comments to explain complex logic.
- Follow consistent coding conventions and style guides.
By following these best practices, you can create code that is not only functional but also efficient, reliable, and easy to maintain.
Resources for Learning Data Structures and Algorithms
Recommended Books
Books are a great way to get a deep understanding of data structures and algorithms. Here are some highly recommended ones:
- Introduction to Algorithms by Cormen et al.
- Algorithms by Robert Sedgewick and Kevin Wayne
Online Courses
Online courses can provide structured learning paths and interactive content. Some of the best platforms include:
- Coursera
- Udacity
- Hiike
Hiike offers a standout program called the Top 30 Program, which provides comprehensive DSA education along with personalized mentoring.
Community and Forums
Joining communities and forums can be very helpful for learning and staying motivated. Here are some popular ones:
- Stack Overflow
- Reddit’s r/learnprogramming
- GitHub Discussions
Engaging with a community can provide support, answer questions, and offer different perspectives on problems.
Coding Challenge Platforms
Practicing coding challenges is essential for mastering DSA. Some popular platforms are:
- LeetCode
- HackerRank
- CodeSignal
Interactive Websites
Interactive websites can help you visualize algorithms and data structures. Some useful ones include:
- VisuAlgo
- GeeksforGeeks
Study Groups
Forming or joining study groups can be beneficial. Collaborate with peers to share knowledge, solve problems, and stay motivated.
Conclusion
With the right resources, learning data structures and algorithms can be an enjoyable and rewarding journey. Start with the basics, practice regularly, and don’t hesitate to seek help from the community.
Looking to master data structures and algorithms? Our website offers a range of resources to help you get started. From interactive coding tutorials to a well-structured study plan, we have everything you need to succeed. Don’t miss out on the chance to boost your problem-solving skills and ace your coding interviews. Visit us today and start your journey towards becoming a coding pro!
Conclusion
In wrapping up our journey through data structures and algorithms, it’s clear that these concepts are the backbone of efficient coding. By understanding and mastering them, you unlock the ability to solve complex problems, optimize your code, and excel in technical interviews. Remember, every expert was once a beginner. So, keep practicing, stay curious, and don’t be afraid to dive deep into these topics. With dedication and the right resources, you’ll find that what once seemed complicated becomes second nature. Happy coding!
Frequently Asked Questions
What are data structures?
Data structures are ways to organize and store data in a computer so it can be accessed and modified efficiently. Examples include arrays, linked lists, stacks, and queues.
Why are data structures important in programming?
Data structures help in managing and organizing data efficiently, which is crucial for writing optimized and effective code. They also play a key role in solving complex problems.
What is an algorithm?
An algorithm is a set of step-by-step instructions or rules designed to perform a specific task or solve a problem. Algorithms can range from simple to complex.
How do algorithms and data structures work together?
Algorithms use data structures to manage and organize data. The choice of a particular data structure can greatly affect the efficiency of an algorithm.
What is Big O notation?
Big O notation is a way to describe the efficiency of an algorithm, especially in terms of time and space. It helps in understanding how an algorithm’s performance changes as the input size grows.
What are some common types of data structures?
Common types of data structures include arrays, linked lists, stacks, queues, trees, graphs, and hash tables. Each has its own specific use cases and advantages.
How can I choose the right data structure for my problem?
Choosing the right data structure depends on the specific requirements of your problem, such as the type of data, the operations you need to perform, and the efficiency you require.
Where can I learn more about data structures and algorithms?
You can learn more through books, online courses, tutorials, and community forums. Engaging with programming communities can also provide valuable insights and support.