Why Syntax Mastery Isn’t as Important as Creative Problem-Solving in Coding
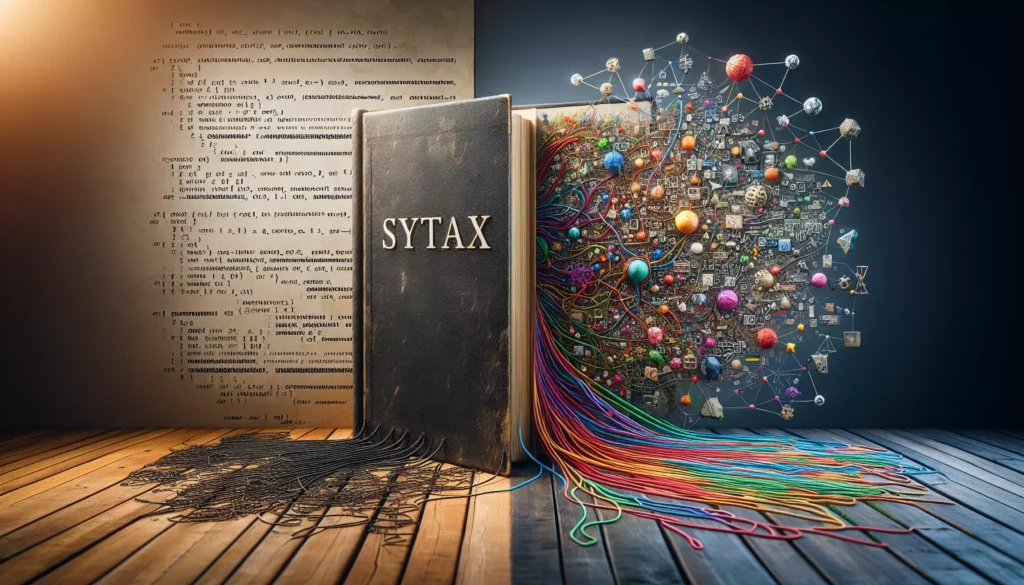
In the world of programming and software development, there’s often a misconception that mastering syntax is the key to becoming a successful coder. While knowing the ins and outs of a programming language is undoubtedly important, it’s not the be-all and end-all of coding prowess. In fact, creative problem-solving skills are far more valuable in the long run. This article will explore why focusing on problem-solving abilities can make you a better programmer and how platforms like AlgoCademy are shifting the focus from syntax memorization to algorithmic thinking.
The Limitations of Syntax Mastery
Let’s start by examining why an exclusive focus on syntax mastery can be limiting:
1. Languages Evolve Rapidly
Programming languages are constantly evolving. New versions are released, syntax changes, and best practices shift. If you spend all your time memorizing the exact syntax of a language, you might find your knowledge outdated sooner than you think. For example, consider the changes between Python 2 and Python 3, or the frequent updates to JavaScript frameworks. Syntax-focused learning can leave you playing catch-up.
2. Transferability is Limited
When you focus solely on syntax, your skills become language-specific. This can make it challenging to switch between languages or adapt to new technologies. In contrast, problem-solving skills are universal and can be applied across different programming paradigms and languages.
3. Syntax Doesn’t Equal Understanding
Knowing how to write syntactically correct code doesn’t necessarily mean you understand what the code does or why it works. This can lead to the creation of inefficient or poorly structured programs that are difficult to maintain or scale.
4. Tools Can Handle Syntax
Modern integrated development environments (IDEs) and code editors come with powerful autocompletion and syntax checking features. These tools can handle much of the syntax-related work, allowing developers to focus on the logic and structure of their code.
The Power of Creative Problem-Solving
Now, let’s explore why creative problem-solving is crucial for programmers:
1. Adaptability in a Changing Landscape
The tech industry is known for its rapid pace of change. New technologies, frameworks, and methodologies emerge constantly. Problem-solving skills allow developers to adapt quickly to these changes, as they can apply their logical thinking to new situations and technologies.
2. Efficient Solution Design
Creative problem-solvers can design more efficient and elegant solutions. They can look at a problem from multiple angles, consider various approaches, and choose the most appropriate one. This leads to code that is not only functional but also optimized and maintainable.
3. Debugging and Troubleshooting
When things go wrong (and they often do in programming), it’s problem-solving skills that save the day. Being able to logically trace through code, identify issues, and creatively come up with solutions is far more valuable than knowing every syntactical rule by heart.
4. Innovation and Advancement
The biggest breakthroughs in technology come from creative problem-solving, not from perfect syntax. Innovative solutions to complex problems drive the industry forward and create new possibilities in software development.
AlgoCademy’s Approach: Fostering Problem-Solving Skills
Platforms like AlgoCademy recognize the importance of problem-solving skills in coding education. Here’s how they’re shifting the focus:
1. Emphasis on Algorithmic Thinking
AlgoCademy places a strong emphasis on algorithmic thinking. This approach teaches students how to break down complex problems into smaller, manageable parts and develop step-by-step solutions. By focusing on algorithms, learners develop a problem-solving mindset that can be applied across different programming languages.
2. Interactive Coding Challenges
Instead of rote memorization of syntax, AlgoCademy provides interactive coding challenges. These challenges present real-world problems that require creative thinking to solve. By working through these challenges, learners develop their problem-solving muscles in a practical, hands-on way.
3. AI-Powered Assistance
AlgoCademy leverages AI to provide personalized guidance to learners. This AI assistance can help with syntax-related issues, allowing students to focus more on the logic and problem-solving aspects of coding. It’s like having a knowledgeable coding buddy who can handle the nitty-gritty details while you focus on the bigger picture.
4. Focus on Technical Interview Preparation
Many coding education platforms, including AlgoCademy, place a significant emphasis on preparing learners for technical interviews, particularly for major tech companies (often referred to as FAANG – Facebook, Amazon, Apple, Netflix, Google). These interviews typically focus more on problem-solving abilities and algorithmic thinking than on perfect syntax recall.
Balancing Syntax and Problem-Solving
While this article argues for the primacy of problem-solving skills, it’s important to note that syntax knowledge is still valuable. The key is finding the right balance. Here are some tips for developing both skills:
1. Learn by Doing
Instead of memorizing syntax, learn it through practical application. Work on projects, solve coding challenges, and build things. This way, you’ll naturally pick up syntax while honing your problem-solving skills.
2. Focus on Concepts, Not Commands
When learning a new language or framework, focus on understanding the underlying concepts rather than memorizing specific commands. This conceptual understanding will make it easier to adapt to syntax changes or learn new languages.
3. Practice Pseudocode
Before diving into coding, practice writing pseudocode. This language-agnostic way of planning your solution helps develop problem-solving skills without getting bogged down in syntax details.
4. Embrace Code Review
Participate in code reviews, both as a reviewer and a reviewee. This process exposes you to different problem-solving approaches and coding styles, helping you improve both your problem-solving skills and your understanding of good coding practices.
Real-World Examples: Problem-Solving Trumps Syntax
Let’s look at some real-world scenarios where problem-solving skills prove more valuable than syntax mastery:
1. Optimizing a Slow Algorithm
Imagine you have a perfectly syntactically correct function that sorts a list of numbers, but it’s too slow for large datasets. The solution isn’t in the syntax, but in understanding sorting algorithms and choosing or implementing a more efficient one.
Here’s a simple bubble sort implementation in Python:
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n - i - 1):
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
return arr
While this code is syntactically correct, it’s not efficient for large datasets. A problem-solver would recognize this and might implement a more efficient algorithm like quicksort:
def quicksort(arr):
if len(arr) <= 1:
return arr
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quicksort(left) + middle + quicksort(right)
The ability to recognize the need for a more efficient algorithm and implement it is a problem-solving skill, not a syntax one.
2. Debugging a Complex Issue
When faced with a bug in a large codebase, syntax knowledge alone won’t be enough. You need to be able to trace through the logic, form hypotheses about what might be causing the issue, and test those hypotheses. This requires creative problem-solving and logical thinking.
3. Designing a System Architecture
When designing the architecture for a new software system, the challenges are rarely about syntax. Instead, you need to consider scalability, maintainability, performance, and how different components will interact. This requires high-level problem-solving skills and the ability to foresee potential issues and design solutions for them.
The Role of Syntax in Problem-Solving
While this article emphasizes the importance of problem-solving over syntax mastery, it’s crucial to understand that syntax does play a role in effective problem-solving. Here’s how:
1. Syntax as a Tool
Think of syntax as a tool in your problem-solving toolbox. Just as a carpenter needs to know how to use their tools effectively, a programmer needs to know how to use syntax to implement their solutions. The key is to view syntax as a means to an end, not the end itself.
2. Syntax Fluency Speeds Up Implementation
While problem-solving is the key skill, being fluent in syntax allows you to implement your solutions more quickly and efficiently. It reduces the cognitive load of translating your logical solution into code, allowing you to focus more on the problem at hand.
3. Understanding Language Capabilities
Knowing the syntax and features of a language helps you understand what’s possible and can inform your problem-solving approach. For example, understanding Python’s list comprehensions might lead you to a more elegant solution to a data processing problem.
Developing Problem-Solving Skills
Now that we’ve established the importance of problem-solving skills, let’s look at some ways to develop them:
1. Practice, Practice, Practice
The best way to improve your problem-solving skills is through consistent practice. Platforms like AlgoCademy offer a variety of coding challenges that can help you hone these skills. Start with simpler problems and gradually increase the difficulty as you improve.
2. Break Down Problems
When faced with a complex problem, practice breaking it down into smaller, more manageable sub-problems. This divide-and-conquer approach is a key problem-solving strategy in programming.
3. Learn Multiple Approaches
For any given problem, try to come up with multiple solutions. This exercises your creative thinking and helps you understand the trade-offs between different approaches.
4. Study Algorithms and Data Structures
Understanding common algorithms and data structures provides you with a toolkit of problem-solving strategies. Knowing when and how to apply these can significantly improve your problem-solving abilities.
5. Analyze Others’ Solutions
After solving a problem, look at how others have solved it. This exposes you to different thinking patterns and problem-solving approaches, broadening your perspective.
The Future of Coding Education
As we move forward, the trend in coding education is likely to continue shifting towards emphasizing problem-solving skills. Here’s what we might expect:
1. More Focus on Computational Thinking
Educational programs are likely to place even more emphasis on computational thinking – the ability to break down complex problems and design algorithmic solutions. This skill transcends specific programming languages and prepares learners for a rapidly evolving tech landscape.
2. Increased Use of AI in Learning
AI-powered coding assistants, like the one used in AlgoCademy, are likely to become more sophisticated. These tools might handle more of the syntax-related aspects of coding, allowing learners to focus more on problem-solving and logic.
3. Project-Based Learning
There may be a greater emphasis on project-based learning, where students work on real-world problems and develop end-to-end solutions. This approach naturally encourages problem-solving and gives learners practical experience.
4. Cross-Disciplinary Coding Education
As coding becomes increasingly important in various fields, we might see more integration of coding education with other disciplines. This could lead to problem-solving challenges that require both coding skills and domain-specific knowledge, further emphasizing the importance of creative problem-solving.
Conclusion
While syntax knowledge is undoubtedly important in programming, it’s the ability to solve problems creatively and efficiently that truly sets great programmers apart. As we’ve explored, problem-solving skills are more transferable, adaptable, and valuable in the long run than mere syntax mastery.
Platforms like AlgoCademy are at the forefront of this shift in coding education, emphasizing algorithmic thinking and problem-solving over rote memorization of syntax. By focusing on developing these crucial skills, aspiring programmers can better prepare themselves for the challenges of real-world software development and the ever-changing landscape of technology.
Remember, programming languages may come and go, syntax may change, but the ability to approach problems logically, break them down, and devise creative solutions will always be in demand. So while you shouldn’t neglect learning syntax, make sure to invest significant time and effort into honing your problem-solving skills. It’s these skills that will truly make you stand out as a programmer and drive innovation in the world of technology.
As you continue your coding journey, whether through platforms like AlgoCademy or other resources, keep this balance in mind. Embrace the challenges, enjoy the process of solving problems, and remember that every bug you fix and every algorithm you optimize is another step in your growth as a creative problem-solver in the exciting world of programming.