Z Algorithm: Mastering Efficient Pattern Matching in Strings
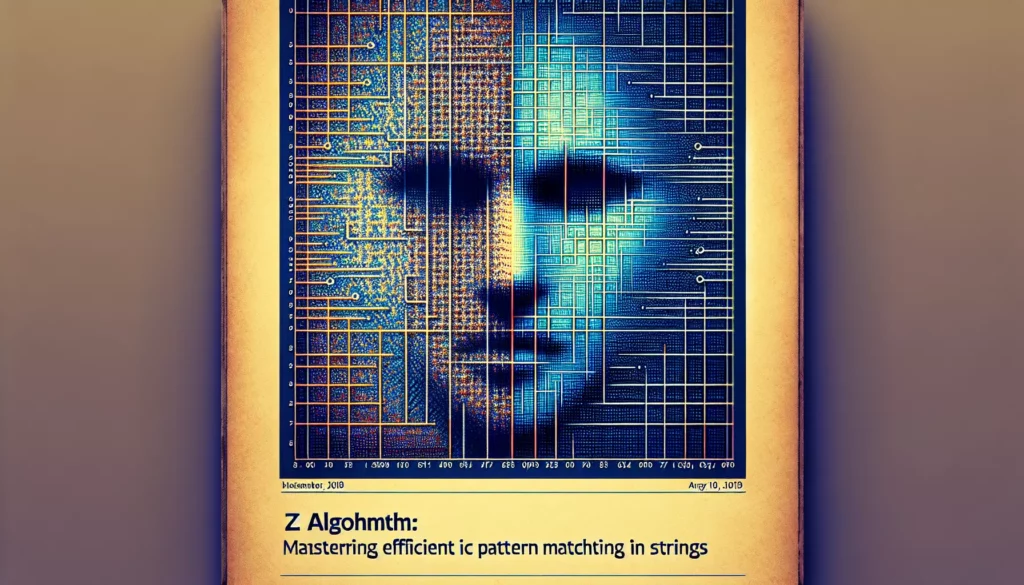
In the world of computer science and programming, efficient string matching algorithms play a crucial role in various applications, from text editors to bioinformatics. One such powerful algorithm that often flies under the radar is the Z Algorithm. This linear-time pattern matching algorithm offers an elegant solution to the problem of finding all occurrences of a pattern within a text. In this comprehensive guide, we’ll dive deep into the Z Algorithm, exploring its inner workings, implementation, and practical applications.
What is the Z Algorithm?
The Z Algorithm, named after its creator Zvi Galil, is a string matching algorithm that preprocesses the pattern to create an auxiliary array called the Z-array. This array allows for efficient pattern matching in linear time complexity, making it a valuable tool for programmers and computer scientists alike.
At its core, the Z Algorithm calculates the length of the longest substring starting from each position in the input string that matches a prefix of the string. This information is then used to perform fast pattern matching without the need for backtracking.
Understanding the Z-array
Before we delve into the algorithm itself, it’s crucial to understand the Z-array, which is the heart of the Z Algorithm. For a string S of length n, the Z-array Z[i] is defined as the length of the longest substring starting from S[i] that is also a prefix of S.
For example, consider the string “aabaacd”:
S = "aabaacd"
Z = [0, 1, 0, 2, 1, 0, 0]
Let’s break down the Z-array:
- Z[0] is always 0 by definition.
- Z[1] = 1 because the longest substring starting at index 1 that matches a prefix is “a”.
- Z[2] = 0 because “b” doesn’t match the prefix “a”.
- Z[3] = 2 because “aa” matches the prefix “aa”.
- Z[4] = 1 because “a” matches the prefix “a”.
- Z[5] = 0 because “c” doesn’t match the prefix “a”.
- Z[6] = 0 because “d” doesn’t match the prefix “a”.
The Z Algorithm: Step-by-Step
Now that we understand the Z-array, let’s walk through the Z Algorithm step-by-step:
- Concatenate the pattern and text with a special character (e.g., “$”) in between.
- Initialize the Z-array with zeros.
- Maintain two variables: L and R, which represent the leftmost and rightmost indices of a Z-box (a substring that matches a prefix).
- Iterate through the concatenated string from index 1 to n-1:
- If i > R, compute Z[i] naively by comparing characters.
- If i ≤ R, set Z[i] = min(Z[i-L], R-i+1).
- While the substring continues to match, increment Z[i].
- If i+Z[i] > R, update L and R.
- Use the Z-array to find all occurrences of the pattern in the text.
Implementing the Z Algorithm
Let’s implement the Z Algorithm in Python to better understand its mechanics:
def z_algorithm(s):
n = len(s)
z = [0] * n
l, r = 0, 0
for i in range(1, n):
if i <= r:
z[i] = min(r - i + 1, z[i - l])
while i + z[i] < n and s[z[i]] == s[i + z[i]]:
z[i] += 1
if i + z[i] - 1 > r:
l, r = i, i + z[i] - 1
return z
def pattern_matching(text, pattern):
combined = pattern + "$" + text
z = z_algorithm(combined)
pattern_length = len(pattern)
results = []
for i in range(pattern_length + 1, len(combined)):
if z[i] == pattern_length:
results.append(i - pattern_length - 1)
return results
# Example usage
text = "ABABDABACDABABCABAB"
pattern = "ABABCABAB"
matches = pattern_matching(text, pattern)
print(f"Pattern found at indices: {matches}")
This implementation first creates the Z-array for the concatenated string (pattern + “$” + text) and then uses it to find all occurrences of the pattern in the text.
Time and Space Complexity
One of the key advantages of the Z Algorithm is its efficiency:
- Time Complexity: O(n + m), where n is the length of the text and m is the length of the pattern. This linear time complexity makes it especially useful for long strings.
- Space Complexity: O(n + m) to store the concatenated string and the Z-array.
The linear time complexity is achieved because each character is compared at most twice: once when extending a Z-box and once when comparing with the pattern.
Advantages of the Z Algorithm
The Z Algorithm offers several advantages over other string matching algorithms:
- Linear Time Complexity: As mentioned, the O(n + m) time complexity makes it efficient for large strings.
- No Preprocessing of Pattern: Unlike KMP or Boyer-Moore algorithms, the Z Algorithm doesn’t require separate preprocessing of the pattern.
- Versatility: The Z-array can be used for various string-related problems beyond simple pattern matching.
- Easy to Implement: The algorithm is relatively straightforward to code and understand.
Applications of the Z Algorithm
The Z Algorithm finds applications in various areas of computer science and beyond:
- String Matching: Its primary use is in finding all occurrences of a pattern in a text.
- Palindrome Detection: By reversing a string and concatenating it with the original, the Z Algorithm can efficiently find palindromes.
- Compression: The Z-array can be used in certain compression algorithms to identify repeated substrings.
- Bioinformatics: For finding repeated DNA sequences or matching protein structures.
- Text Editors: Implementing “find” functionality in text editing software.
- Plagiarism Detection: Identifying copied passages in large bodies of text.
Comparison with Other String Matching Algorithms
To fully appreciate the Z Algorithm, it’s worth comparing it to other popular string matching algorithms:
1. Naive String Matching
The naive approach involves checking every possible position in the text for a match with the pattern.
- Time Complexity: O(nm) in the worst case
- Advantage: Simple to implement
- Disadvantage: Inefficient for large texts or patterns
2. Knuth-Morris-Pratt (KMP) Algorithm
KMP uses a failure function to skip characters when a mismatch occurs.
- Time Complexity: O(n + m)
- Advantage: Efficient for single pattern matching
- Disadvantage: Requires preprocessing of the pattern
3. Boyer-Moore Algorithm
Boyer-Moore uses bad character and good suffix heuristics to skip characters.
- Time Complexity: O(n + m) in the best case, O(nm) in the worst case
- Advantage: Very fast in practice, especially for large alphabets
- Disadvantage: Complex preprocessing and implementation
4. Rabin-Karp Algorithm
Rabin-Karp uses hashing to compare substrings.
- Time Complexity: O(n + m) average case, O(nm) worst case
- Advantage: Good for multiple pattern matching
- Disadvantage: Can have false positives due to hash collisions
Compared to these algorithms, the Z Algorithm offers a good balance of efficiency, simplicity, and versatility. Its linear time complexity and lack of pattern preprocessing make it particularly attractive for certain applications.
Optimizing the Z Algorithm
While the basic Z Algorithm is already efficient, there are ways to optimize it further for specific use cases:
1. Avoiding Concatenation
Instead of concatenating the pattern and text, you can modify the algorithm to work directly with separate pattern and text strings. This saves memory and avoids creating a new string.
2. Early Termination
If you only need to find the first occurrence of the pattern, you can modify the algorithm to return as soon as a match is found, potentially saving computation time.
3. Bit-level Parallelism
For small alphabets (e.g., DNA sequences), you can use bit-level parallelism to compare multiple characters at once, potentially speeding up the matching process.
4. Cache Optimization
Reorganizing data access patterns to be more cache-friendly can lead to significant speed improvements, especially for very large strings.
Common Pitfalls and How to Avoid Them
When implementing or using the Z Algorithm, be aware of these common pitfalls:
- Off-by-One Errors: Be careful with array indices, especially when dealing with the concatenated string.
- Integer Overflow: For very large strings, ensure that your variables can handle the size without overflow.
- Unnecessary Comparisons: Make sure to utilize the information in the Z-array effectively to avoid redundant character comparisons.
- Ignoring Edge Cases: Consider empty strings, single-character strings, and patterns longer than the text.
Practical Exercise: Implementing Z Algorithm for DNA Sequence Matching
Let’s put our knowledge of the Z Algorithm into practice with a real-world scenario. Suppose we’re working on a bioinformatics project and need to find all occurrences of a specific DNA sequence within a larger genome.
def dna_sequence_matcher(genome, sequence):
def z_function(s):
n = len(s)
z = [0] * n
l, r = 0, 0
for i in range(1, n):
if i <= r:
z[i] = min(r - i + 1, z[i - l])
while i + z[i] < n and s[z[i]] == s[i + z[i]]:
z[i] += 1
if i + z[i] - 1 > r:
l, r = i, i + z[i] - 1
return z
combined = sequence + "$" + genome
z = z_function(combined)
seq_len = len(sequence)
matches = []
for i in range(seq_len + 1, len(combined)):
if z[i] == seq_len:
matches.append(i - seq_len - 1)
return matches
# Example usage
genome = "ACGTACGTACGTACGTACGTACGT"
sequence = "ACGT"
results = dna_sequence_matcher(genome, sequence)
print(f"Sequence found at positions: {results}")
This implementation is optimized for DNA sequences, which have a small alphabet (A, C, G, T). It efficiently finds all occurrences of the target sequence within the genome.
Advanced Topics Related to the Z Algorithm
For those looking to deepen their understanding of string algorithms, here are some advanced topics related to the Z Algorithm:
1. Suffix Arrays and the Z Algorithm
The Z Algorithm can be used in conjunction with suffix arrays to solve various string problems efficiently. Understanding this relationship can lead to powerful string processing techniques.
2. Longest Common Prefix (LCP) Array
The Z-array is closely related to the LCP array. Exploring this connection can provide insights into both algorithms and their applications.
3. Streaming Algorithms
Adapting the Z Algorithm for streaming data, where the entire input is not available at once, presents interesting challenges and opportunities.
4. Approximate String Matching
Extending the Z Algorithm to handle approximate matches (with a certain number of allowed differences) opens up new applications in areas like spell checking and DNA sequence alignment.
Conclusion
The Z Algorithm is a powerful tool in the string matching arsenal, offering linear time complexity and versatility. Its ability to efficiently preprocess the input string and find all occurrences of a pattern makes it valuable in various applications, from text processing to bioinformatics.
By understanding the Z Algorithm, you’ve added a significant technique to your programming toolkit. As you continue to explore string algorithms, you’ll find that the concepts behind the Z Algorithm, such as the Z-array and efficient prefix matching, appear in various other advanced string processing techniques.
Remember, the key to mastering algorithms like the Z Algorithm is practice. Try implementing it for different problems, optimize it for specific use cases, and explore its connections to other string algorithms. With time and experience, you’ll develop a deep intuition for efficient string processing, a skill that’s invaluable in many areas of computer science and software development.
Happy coding, and may your strings always match efficiently!