Your Roadmap to Landing Your Dream Software Engineering Job: A Comprehensive Guide
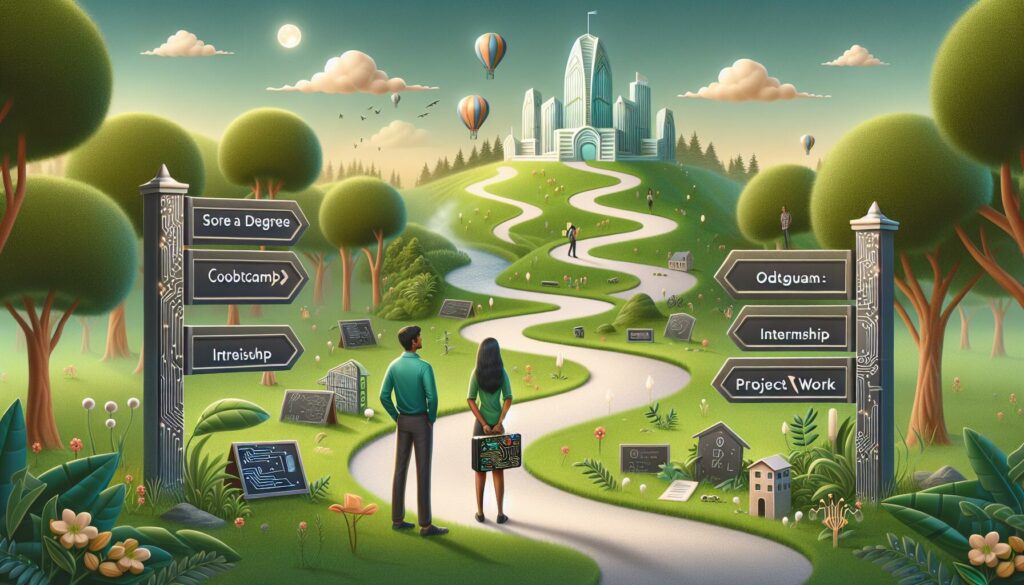
In today’s rapidly evolving tech landscape, software engineering stands out as one of the most dynamic and rewarding career paths. Whether you’re a recent graduate, a career changer, or simply looking to level up in the tech industry, this comprehensive guide will serve as your roadmap to landing your dream software engineering job. Let’s embark on this exciting journey together!
1. Understanding the Software Engineering Landscape
Before diving into the specifics of landing your dream job, it’s crucial to understand the broader landscape of software engineering. This field is vast and diverse, offering numerous specializations and career paths.
1.1 Types of Software Engineering Roles
- Front-End Engineer: Focuses on creating user interfaces and experiences.
- Back-End Engineer: Works on server-side logic and databases.
- Full-Stack Engineer: Combines both front-end and back-end skills.
- Mobile Developer: Specializes in creating apps for iOS, Android, or cross-platform.
- DevOps Engineer: Bridges the gap between development and operations.
- Machine Learning Engineer: Develops AI and machine learning systems.
1.2 Industry Trends and Demands
Stay informed about the latest trends in software engineering. Currently, areas like artificial intelligence, cloud computing, cybersecurity, and blockchain are seeing significant growth. Understanding these trends can help you align your skills with market demands.
2. Building a Strong Foundation
A solid foundation in computer science and programming is essential for any aspiring software engineer. Here’s what you need to focus on:
2.1 Core Computer Science Concepts
- Data Structures and Algorithms
- Object-Oriented Programming
- Database Management
- Operating Systems
- Computer Networks
2.2 Programming Languages
While the choice of language often depends on your specialization, some widely used languages include:
- Python
- JavaScript
- Java
- C++
- Go
- Ruby
Here’s a simple Python example to illustrate a basic concept:
# A simple function to calculate factorial
def factorial(n):
if n == 0 or n == 1:
return 1
else:
return n * factorial(n-1)
# Test the function
print(factorial(5)) # Output: 120
2.3 Version Control
Learn to use version control systems, particularly Git. This is crucial for collaborative coding and is used in almost all software development environments.
3. Gaining Practical Experience
Theory alone isn’t enough. To stand out in the job market, you need practical experience. Here’s how to get it:
3.1 Personal Projects
Build your own projects. This could be a web application, a mobile app, or even a simple tool that solves a problem you’ve encountered. These projects demonstrate your ability to apply your knowledge and can be showcased in your portfolio.
3.2 Open Source Contributions
Contributing to open source projects is an excellent way to gain experience, learn from others, and make connections in the tech community. Platforms like GitHub are great for finding projects to contribute to.
3.3 Internships
Internships provide real-world experience and often lead to full-time positions. They’re an excellent way to understand how software engineering works in a professional setting.
4. Building Your Online Presence
In the digital age, your online presence can significantly impact your job prospects. Here’s how to build a strong online profile:
4.1 GitHub Profile
Maintain an active GitHub profile showcasing your projects and contributions. Ensure your repositories are well-documented and your code is clean and readable.
4.2 LinkedIn
Create a professional LinkedIn profile. Highlight your skills, experiences, and projects. Connect with professionals in the field and engage with content relevant to software engineering.
4.3 Personal Website or Blog
Consider creating a personal website or blog where you can showcase your projects, write about your learning journey, and share your insights on tech topics.
5. Networking and Community Engagement
Networking plays a crucial role in finding opportunities and staying updated with industry trends.
5.1 Tech Meetups and Conferences
Attend local tech meetups and conferences. These events are great for learning, networking, and potentially finding job opportunities.
5.2 Online Communities
Join online communities like Stack Overflow, Reddit’s programming subreddits, or Discord servers focused on software development. Engage in discussions, ask questions, and share your knowledge.
5.3 Hackathons
Participate in hackathons. They’re excellent opportunities to build projects quickly, work in teams, and sometimes even win prizes or catch the eye of potential employers.
6. Preparing for the Job Search
When you feel ready to start applying for jobs, here’s how to prepare:
6.1 Tailoring Your Resume
Create a resume that highlights your skills, projects, and experiences relevant to software engineering. Tailor it for each job application, emphasizing the skills and experiences most relevant to that specific role.
6.2 Writing a Compelling Cover Letter
Your cover letter should complement your resume, not repeat it. Use it to tell your story, explain your passion for software engineering, and why you’re a great fit for the specific role and company.
6.3 Building a Portfolio
Compile your best projects into a portfolio. This could be part of your personal website or a separate document. Include descriptions of each project, the technologies used, and your role in its development.
7. Mastering the Interview Process
The interview process for software engineering roles can be rigorous. Here’s how to prepare:
7.1 Technical Interviews
Practice coding problems on platforms like LeetCode, HackerRank, or CodeSignal. Focus on data structures, algorithms, and problem-solving skills. Here’s an example of a common interview question:
# Two Sum Problem
def two_sum(nums, target):
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
return []
# Test the function
print(two_sum([2, 7, 11, 15], 9)) # Output: [0, 1]
7.2 Behavioral Interviews
Prepare for questions about your experiences, how you handle challenges, and your ability to work in a team. Use the STAR method (Situation, Task, Action, Result) to structure your responses.
7.3 System Design Interviews
For more senior roles, you might face system design questions. Study scalable systems, distributed systems, and practice designing high-level architectures for various applications.
8. Continuous Learning and Growth
The journey doesn’t end with landing your first job. Software engineering is a field of continuous learning and growth.
8.1 Stay Updated with Technology Trends
Follow tech blogs, podcasts, and news sites to stay informed about the latest developments in software engineering.
8.2 Pursue Further Education
Consider pursuing advanced degrees, certifications, or specialized courses to deepen your knowledge and advance your career.
8.3 Mentorship
Seek out mentors in your field. Their guidance can be invaluable for your professional growth. As you progress, consider mentoring others as well.
9. Understanding the Software Development Lifecycle
A crucial aspect of software engineering is understanding the entire lifecycle of software development. This knowledge will help you work more effectively in teams and contribute to projects at various stages.
9.1 Requirements Gathering
Learn how to effectively gather and analyze requirements from stakeholders. This involves understanding user needs, business objectives, and technical constraints.
9.2 Design
Familiarize yourself with design principles and patterns. This includes both high-level system architecture and low-level component design.
9.3 Implementation
This is where your coding skills come into play. Practice writing clean, efficient, and well-documented code.
9.4 Testing
Understand different types of testing (unit, integration, system, acceptance) and learn to write effective test cases. Here’s a simple example of a unit test in Python:
import unittest
def add(a, b):
return a + b
class TestAddFunction(unittest.TestCase):
def test_add_positive_numbers(self):
self.assertEqual(add(2, 3), 5)
def test_add_negative_numbers(self):
self.assertEqual(add(-1, -1), -2)
if __name__ == '__main__':
unittest.main()
9.5 Deployment
Learn about deployment processes, including continuous integration and continuous deployment (CI/CD) pipelines.
9.6 Maintenance
Understand the importance of maintaining and updating existing software systems.
10. Specializing in a Niche
While it’s important to have a broad understanding of software engineering, specializing in a particular area can make you stand out in the job market.
10.1 Web Development
If you’re interested in building web applications, focus on:
- Front-end frameworks like React, Angular, or Vue.js
- Back-end technologies like Node.js, Django, or Ruby on Rails
- Database management systems (SQL and NoSQL)
- RESTful API design
10.2 Mobile Development
For mobile app development, consider learning:
- iOS development with Swift
- Android development with Kotlin
- Cross-platform development with React Native or Flutter
10.3 Machine Learning and AI
If AI interests you, focus on:
- Python and its scientific libraries (NumPy, Pandas, Scikit-learn)
- Deep learning frameworks like TensorFlow or PyTorch
- Statistical analysis and data visualization
10.4 DevOps
For a career in DevOps, learn about:
- Cloud platforms (AWS, Azure, Google Cloud)
- Containerization (Docker, Kubernetes)
- Infrastructure as Code (Terraform, Ansible)
- Monitoring and logging tools
11. Soft Skills for Software Engineers
While technical skills are crucial, soft skills can often make the difference in your career progression. Focus on developing:
11.1 Communication
Learn to explain complex technical concepts to both technical and non-technical audiences. Practice writing clear documentation and giving presentations.
11.2 Teamwork
Software development is often a team effort. Learn to collaborate effectively, share knowledge, and work towards common goals.
11.3 Problem-Solving
Develop a methodical approach to problem-solving. Break down complex problems into manageable parts and learn to think critically about solutions.
11.4 Time Management
Learn to prioritize tasks, manage deadlines, and balance multiple responsibilities effectively.
11.5 Adaptability
The tech industry evolves rapidly. Cultivate a mindset that embraces change and continuous learning.
12. Navigating Your Career Path
As you progress in your software engineering career, consider the various paths available to you:
12.1 Technical Specialist
Deepen your expertise in a specific technology or domain, becoming a go-to expert in your organization.
12.2 Technical Leadership
Move towards roles like Tech Lead or Principal Engineer, where you’ll guide technical decisions and mentor other engineers.
12.3 Management
Transition into engineering management roles, focusing on team leadership and project management.
12.4 Entrepreneurship
Use your technical skills to start your own tech company or work as a freelance consultant.
13. Staying Motivated and Overcoming Challenges
The journey to becoming a successful software engineer can be challenging. Here are some tips to stay motivated:
13.1 Set Clear Goals
Define both short-term and long-term career goals. Regularly review and adjust these goals as you progress.
13.2 Celebrate Small Wins
Acknowledge your progress, no matter how small. Each bug fixed or concept learned is a step forward.
13.3 Join a Community
Surround yourself with like-minded individuals. Join coding communities, study groups, or find an accountability partner.
13.4 Practice Self-Care
Remember to take breaks, exercise, and maintain a healthy work-life balance to prevent burnout.
Conclusion: Your Journey Begins Now
Landing your dream software engineering job is an exciting and rewarding journey. It requires dedication, continuous learning, and perseverance. Remember, every expert was once a beginner. With the right mindset, skills, and preparation, you can navigate the path to a successful career in software engineering.
As you embark on this journey, keep this guide handy. Refer back to it as you progress, using it as a roadmap to guide your learning and career development. The world of software engineering is vast and full of opportunities. Your dream job is out there – now it’s time to go and claim it!
Ready to take the next step? Download our comprehensive eBook for even more detailed insights and strategies:
Download your free copy of “A Roadmap to Landing Your Dream Software Engineering Job” now!
Remember, the journey of a thousand miles begins with a single step. Take that step today, and start building the foundation for your dream career in software engineering. The future is bright, and it’s waiting for you to shape it with your code. Happy coding, and best of luck on your software engineering journey!