Working with Constraints: How to Handle Limited Time, Memory, or Other Resources During a Coding Interview
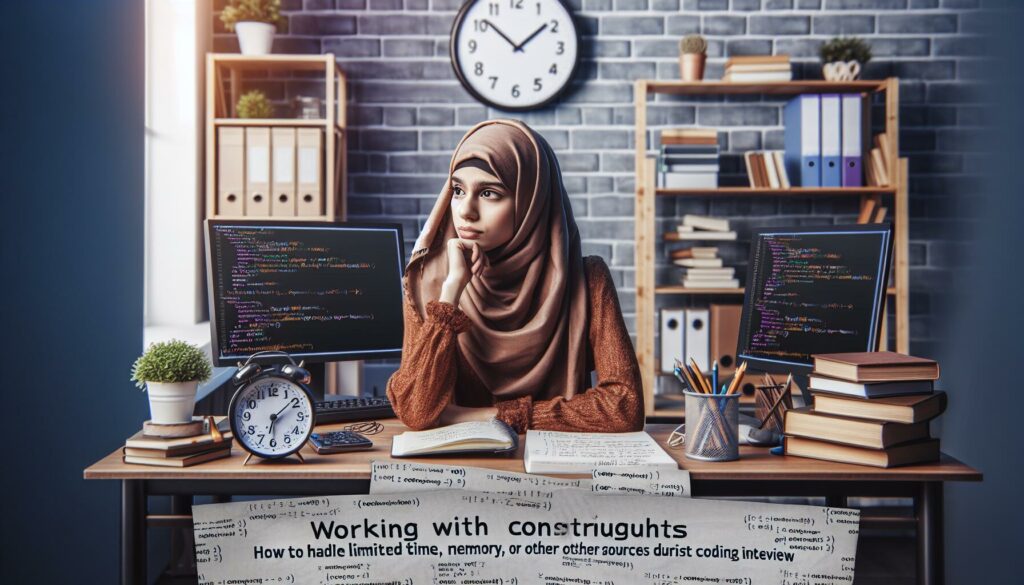
Coding interviews are a crucial part of the hiring process for software engineering positions, especially at major tech companies. These interviews often present candidates with complex problems that need to be solved under various constraints, such as limited time, memory, or other resources. Successfully navigating these constraints is not only essential for passing the interview but also demonstrates your ability to write efficient and scalable code in real-world scenarios.
In this comprehensive guide, we’ll explore strategies for working with constraints during coding interviews, focusing on how to optimize your solutions for limited resources while maintaining correctness. We’ll cover various aspects of constraint management, provide practical examples, and offer tips to help you excel in your next coding interview.
Understanding the Importance of Constraints in Coding Interviews
Before diving into specific strategies, it’s crucial to understand why interviewers often impose constraints during coding interviews:
- Simulating real-world scenarios: In actual development environments, resources are rarely unlimited. Constraints in interviews mimic real-world challenges.
- Testing problem-solving skills: Constraints force candidates to think creatively and demonstrate their ability to optimize solutions.
- Evaluating algorithmic knowledge: Constraints often require candidates to apply specific algorithms or data structures to meet efficiency requirements.
- Assessing code quality: Working within constraints can reveal a candidate’s ability to write clean, maintainable code under pressure.
Common Types of Constraints in Coding Interviews
Coding interviews typically involve one or more of the following constraints:
- Time constraints: Limited time to solve the problem or requirements for specific time complexity (e.g., O(n) or O(log n)).
- Memory constraints: Restrictions on the amount of additional memory that can be used or requirements for specific space complexity.
- Input/Output constraints: Limitations on how data can be accessed or manipulated (e.g., read-only input arrays or single-pass algorithms).
- Language or library constraints: Restrictions on using certain built-in functions or libraries.
Strategies for Handling Time Constraints
Time constraints are among the most common challenges in coding interviews. Here are some strategies to help you optimize your solutions for better time efficiency:
1. Understand Time Complexity
Before attempting to optimize your solution, ensure you have a solid understanding of time complexity and how it applies to different algorithms and data structures. Familiarize yourself with common time complexities:
- O(1): Constant time
- O(log n): Logarithmic time
- O(n): Linear time
- O(n log n): Linearithmic time
- O(n^2): Quadratic time
- O(2^n): Exponential time
2. Choose Appropriate Data Structures
Selecting the right data structure can significantly impact the time complexity of your solution. Consider the following examples:
- Use hash tables (e.g., HashSet or HashMap in Java) for O(1) average-case lookup, insertion, and deletion.
- Implement binary search trees or heaps for O(log n) operations on sorted data.
- Utilize arrays or linked lists for sequential access with O(n) time complexity.
3. Apply Efficient Algorithms
Familiarize yourself with common algorithmic techniques and their time complexities:
- Binary search: O(log n) for searching in sorted arrays
- Two-pointer technique: O(n) for many array-based problems
- Sliding window: O(n) for substring or subarray problems
- Dynamic programming: Often reduces exponential time to polynomial time
4. Optimize Nested Loops
Nested loops can quickly lead to high time complexity. Look for opportunities to reduce the number of nested loops or optimize their contents:
// Inefficient O(n^2) approach
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
// Do something
}
}
// Optimized O(n) approach (if possible)
for (int i = 0; i < n; i++) {
// Do something more efficiently
}
5. Use Memoization and Caching
For problems with overlapping subproblems, consider using memoization or caching to avoid redundant calculations:
// Memoization example for Fibonacci sequence
public int fibonacci(int n, Map<Integer, Integer> memo) {
if (n <= 1) return n;
if (memo.containsKey(n)) return memo.get(n);
int result = fibonacci(n - 1, memo) + fibonacci(n - 2, memo);
memo.put(n, result);
return result;
}
Strategies for Handling Memory Constraints
Memory constraints can be particularly challenging, especially when dealing with large datasets. Here are some strategies to optimize your solutions for better space efficiency:
1. Understand Space Complexity
Similar to time complexity, it’s crucial to understand space complexity and how it applies to different algorithms and data structures. Common space complexities include:
- O(1): Constant space
- O(n): Linear space
- O(n^2): Quadratic space
2. Use In-Place Algorithms
In-place algorithms modify the input directly without using additional data structures, resulting in O(1) extra space complexity. Examples include:
- In-place array reversal
- Quicksort or heapsort for sorting
- Cycle detection in linked lists
3. Optimize Data Structures
Choose space-efficient data structures and consider trade-offs between time and space complexity:
- Use bit manipulation techniques to store boolean flags in integers
- Implement memory-efficient data structures like sparse matrices for large, sparse datasets
- Consider using streams or iterators for processing large datasets without loading everything into memory
4. Avoid Unnecessary Object Creation
Minimize object creation, especially in loops or recursive calls:
// Inefficient approach (creates many String objects)
String result = "";
for (int i = 0; i < n; i++) {
result += someString;
}
// Optimized approach (uses StringBuilder)
StringBuilder result = new StringBuilder();
for (int i = 0; i < n; i++) {
result.append(someString);
}
return result.toString();
5. Use Tail Recursion
When dealing with recursive algorithms, consider using tail recursion to optimize stack space usage:
// Non-tail recursive factorial
public int factorial(int n) {
if (n == 0) return 1;
return n * factorial(n - 1);
}
// Tail recursive factorial
public int factorialTail(int n, int accumulator) {
if (n == 0) return accumulator;
return factorialTail(n - 1, n * accumulator);
}
Strategies for Handling Input/Output Constraints
Input/Output constraints can limit how you access or manipulate data. Here are some strategies to handle these constraints:
1. Single-Pass Algorithms
Develop algorithms that process the input in a single pass, especially useful for stream processing or when dealing with large datasets:
// Single-pass algorithm to find the maximum element
public int findMax(int[] nums) {
int max = Integer.MIN_VALUE;
for (int num : nums) {
max = Math.max(max, num);
}
return max;
}
2. Two-Pointer Technique
Use two pointers to solve problems efficiently, especially for array or linked list problems:
// Two-pointer technique to reverse a string
public void reverseString(char[] s) {
int left = 0, right = s.length - 1;
while (left < right) {
char temp = s[left];
s[left++] = s[right];
s[right--] = temp;
}
}
3. Sliding Window
Implement the sliding window technique for substring or subarray problems:
// Sliding window to find the longest substring without repeating characters
public int lengthOfLongestSubstring(String s) {
int n = s.length();
Set<Character> set = new HashSet<>();
int ans = 0, i = 0, j = 0;
while (i < n && j < n) {
if (!set.contains(s.charAt(j))) {
set.add(s.charAt(j++));
ans = Math.max(ans, j - i);
} else {
set.remove(s.charAt(i++));
}
}
return ans;
}
Strategies for Handling Language or Library Constraints
When faced with language or library constraints, consider the following strategies:
1. Implement Standard Algorithms
Be prepared to implement common algorithms and data structures from scratch:
- Sorting algorithms (e.g., quicksort, mergesort)
- Search algorithms (e.g., binary search)
- Data structures (e.g., linked list, binary search tree)
2. Use Basic Language Constructs
Rely on fundamental language constructs rather than advanced libraries:
// Using basic constructs to implement a queue
public class SimpleQueue<T> {
private List<T> data = new ArrayList<>();
public void enqueue(T item) {
data.add(item);
}
public T dequeue() {
if (isEmpty()) throw new NoSuchElementException();
return data.remove(0);
}
public boolean isEmpty() {
return data.isEmpty();
}
}
3. Understand Core Language Features
Familiarize yourself with core language features that can be used to implement more complex functionality:
- Bitwise operations for efficient calculations
- String manipulation techniques
- Basic file I/O operations
Balancing Efficiency with Correctness
While optimizing for constraints is important, it’s crucial to maintain the correctness of your solution. Here are some tips for striking the right balance:
1. Start with a Correct Solution
Begin by implementing a correct solution, even if it’s not the most efficient. This ensures you have a working baseline to optimize from.
2. Optimize Incrementally
Make small, incremental optimizations and test after each change to ensure correctness is maintained.
3. Communicate Your Thought Process
Explain your optimization strategies to the interviewer, discussing trade-offs and potential improvements.
4. Use Test Cases
Develop and use test cases to verify your solution’s correctness, including edge cases and large inputs.
5. Consider Time vs. Space Trade-offs
Be prepared to discuss trade-offs between time and space complexity, and choose the appropriate balance based on the problem requirements.
Practical Tips for Coding Interviews
To excel in coding interviews with constraints, consider these additional tips:
1. Practice, Practice, Practice
Regularly solve coding problems under simulated interview conditions, including time pressure and constraints.
2. Analyze Multiple Solutions
For each problem you practice, explore multiple solutions with different time and space complexities.
3. Learn to Estimate Complexity
Develop the ability to quickly estimate the time and space complexity of your solutions.
4. Stay Calm Under Pressure
Remember that interviewers are often more interested in your problem-solving process than a perfect solution.
5. Ask Clarifying Questions
Don’t hesitate to ask for clarification about constraints or requirements before diving into the solution.
Conclusion
Working with constraints in coding interviews is a crucial skill for any aspiring software engineer. By understanding common constraints, developing strategies to optimize your solutions, and balancing efficiency with correctness, you can significantly improve your performance in technical interviews.
Remember that handling constraints effectively is not just about passing interviews; it’s a valuable skill that translates directly to real-world software development. As you continue to practice and refine your skills, you’ll become more adept at writing efficient, scalable code that can handle the challenges of modern software engineering.
Keep pushing yourself to solve problems under various constraints, and don’t be afraid to explore different approaches. With dedication and practice, you’ll be well-prepared to tackle any coding interview and demonstrate your ability to create optimized solutions in resource-constrained environments.