Word Ladder Problem: A Deep Dive into Algorithmic Problem-Solving
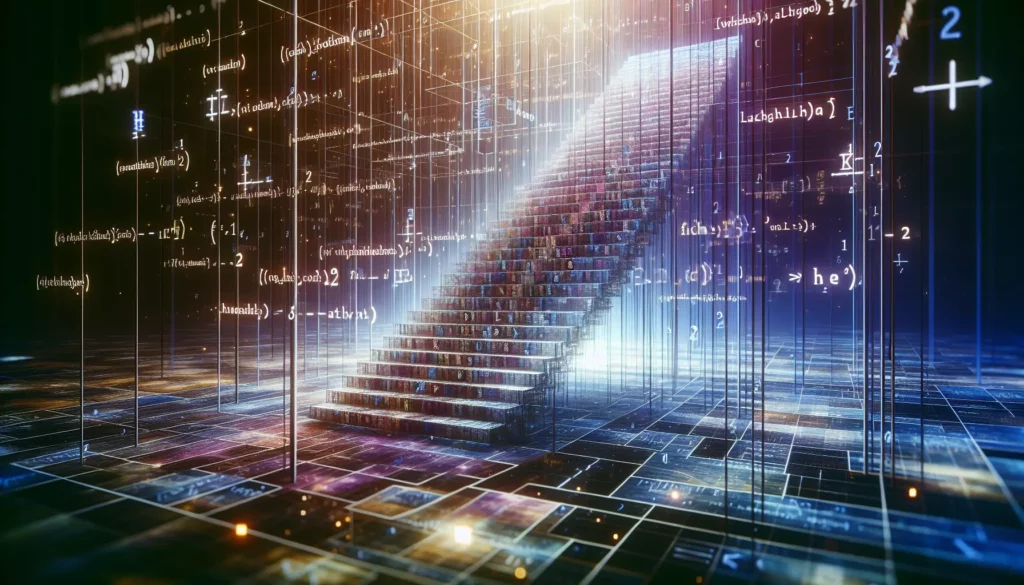
Welcome to another exciting exploration of algorithmic problem-solving! Today, we’re going to tackle the fascinating Word Ladder problem, a classic challenge that tests your graph traversal and string manipulation skills. This problem is not only a favorite in coding interviews but also a great exercise to sharpen your algorithmic thinking.
What is the Word Ladder Problem?
The Word Ladder problem, first proposed by Lewis Carroll in 1877, is a word game where the goal is to transform one word into another by changing a single letter at a time. Each intermediate word in the sequence must be a valid word. For example, transforming “COLD” to “WARM” might look like this:
COLD → CORD → CARD → WARD → WARM
In the context of computer science and algorithms, the Word Ladder problem asks us to find the shortest such transformation between two given words, if one exists.
Problem Statement
Given two words, beginWord
and endWord
, and a dictionary wordList
, return the length of the shortest transformation sequence from beginWord
to endWord
, such that:
- Only one letter can be changed at a time.
- Each transformed word must exist in the word list.
Return 0 if there is no such transformation sequence.
Understanding the Problem
Before we dive into solving the Word Ladder problem, let’s break it down and understand its key components:
- Graph Representation: We can think of this problem as a graph where each word is a node, and an edge exists between two words if they differ by exactly one letter.
- Shortest Path: Finding the shortest transformation sequence is equivalent to finding the shortest path in this graph from the begin word to the end word.
- Dictionary Constraint: All intermediate words must exist in the given dictionary, which limits our search space.
Approach to Solving the Word Ladder Problem
To solve the Word Ladder problem efficiently, we’ll use a Breadth-First Search (BFS) approach. BFS is ideal for finding the shortest path in an unweighted graph, which is exactly what we need here. Here’s a step-by-step breakdown of our approach:
- Convert the word list to a set for O(1) lookup time.
- Initialize a queue with the begin word and its level (which is 1).
- While the queue is not empty:
- Dequeue a word and its level.
- If this word is the end word, return its level.
- Generate all possible one-letter transformations of the current word.
- For each valid transformation (exists in the word list):
- Add it to the queue with an incremented level.
- Remove it from the word list to avoid cycles.
- If we exhaust the queue without finding the end word, return 0.
Implementing the Solution
Let’s implement this solution in Python:
from collections import deque
def ladderLength(beginWord: str, endWord: str, wordList: List[str]) -> int:
word_set = set(wordList)
if endWord not in word_set:
return 0
queue = deque([(beginWord, 1)])
while queue:
word, level = queue.popleft()
if word == endWord:
return level
for i in range(len(word)):
for c in 'abcdefghijklmnopqrstuvwxyz':
next_word = word[:i] + c + word[i+1:]
if next_word in word_set:
word_set.remove(next_word)
queue.append((next_word, level + 1))
return 0
Breaking Down the Solution
Let’s analyze our implementation step by step:
- Initialization:
- We convert the
wordList
to a set for O(1) lookup time. - We check if the
endWord
is in the set. If not, we return 0 as no transformation is possible. - We initialize a queue with a tuple containing the
beginWord
and its level (1).
- We convert the
- BFS Loop:
- We continue while the queue is not empty.
- We dequeue a word and its level.
- If the dequeued word is the
endWord
, we’ve found the shortest path and return its level.
- Word Transformation:
- For each character position in the word, we try replacing it with each letter of the alphabet.
- If the transformed word exists in our word set, we:
- Remove it from the set to avoid revisiting.
- Add it to the queue with an incremented level.
- Termination:
- If we exhaust the queue without finding the
endWord
, we return 0.
- If we exhaust the queue without finding the
Time and Space Complexity Analysis
Let’s analyze the time and space complexity of our solution:
Time Complexity
The time complexity of this solution is O(26 * L * N), where:
- L is the length of each word
- N is the number of words in the word list
- 26 is the number of lowercase English letters
This is because in the worst case, we might need to try all possible transformations for each word, and for each position in the word, we try all 26 letters.
Space Complexity
The space complexity is O(N), where N is the number of words in the word list. This space is used to store the word set and the queue. In the worst case, we might need to store all words in the queue.
Optimizations and Variations
While our current solution is efficient, there are some optimizations and variations we can consider:
1. Bidirectional BFS
We can perform BFS from both the begin word and the end word simultaneously. This can significantly reduce the search space in many cases.
2. Using a Word Pattern
Instead of trying all 26 letters, we can generate a pattern for each word (e.g., “h*t” for “hot”) and use a dictionary to store words matching each pattern. This can be faster for large word lists.
3. Preprocessing the Word List
If we need to solve this problem multiple times with the same word list, we can preprocess the list to create an adjacency list representation of the word graph.
Common Pitfalls and Edge Cases
When implementing the Word Ladder solution, be aware of these common pitfalls and edge cases:
- Empty Word List: Always check if the word list is empty.
- Begin Word Not in List: Some variations of this problem may not include the begin word in the list. Decide how to handle this case.
- End Word Not in List: Always check if the end word is in the list before starting the search.
- Begin Word Equals End Word: Handle the case where the begin and end words are the same.
- Case Sensitivity: Be clear about whether the problem is case-sensitive or not.
Related Problems and Concepts
The Word Ladder problem is related to several other important algorithmic concepts and problems:
- Graph Traversal: This problem is essentially a graph traversal problem, specifically using BFS.
- Shortest Path in Unweighted Graph: The Word Ladder problem is a specific application of finding the shortest path in an unweighted graph.
- String Manipulation: The problem involves manipulating strings to generate word transformations.
- Set Operations: We use set operations for efficient word lookups and removals.
Real-World Applications
While the Word Ladder problem might seem like a fun puzzle, it has several real-world applications:
- Genetic Sequence Analysis: Similar algorithms are used to analyze mutations in genetic sequences.
- Natural Language Processing: Word transformation algorithms are useful in spell checkers and autocorrect features.
- Network Routing: The concept of finding the shortest transformation can be applied to finding the shortest path in network routing.
- Social Network Analysis: Similar algorithms can be used to find the shortest connection between two people in a social network.
Conclusion
The Word Ladder problem is a fascinating challenge that combines graph theory, breadth-first search, and string manipulation. By solving this problem, you’ve not only prepared yourself for potential interview questions but also gained insights into algorithms that have real-world applications in fields ranging from computational biology to social network analysis.
Remember, the key to mastering algorithmic problems like Word Ladder is practice and understanding the underlying concepts. Don’t just memorize the solution; try to understand why BFS works well for this problem, how the graph is implicitly formed, and how we can optimize our approach.
As you continue your journey in algorithmic problem-solving, you’ll find that many problems can be reduced to graph problems or shortest path problems, much like the Word Ladder. Keep practicing, and soon you’ll be tackling even more complex algorithmic challenges with confidence!
Further Reading and Resources
To deepen your understanding of the concepts used in the Word Ladder problem, consider exploring these related topics:
- Graph Theory and Its Applications
- Advanced BFS and DFS Techniques
- String Algorithms and Manipulations
- Time and Space Complexity Analysis
- Optimization Techniques in Algorithm Design
Happy coding, and may your algorithmic journey be filled with exciting challenges and rewarding solutions!