Word Break Problem: Mastering Dynamic Programming for String Manipulation
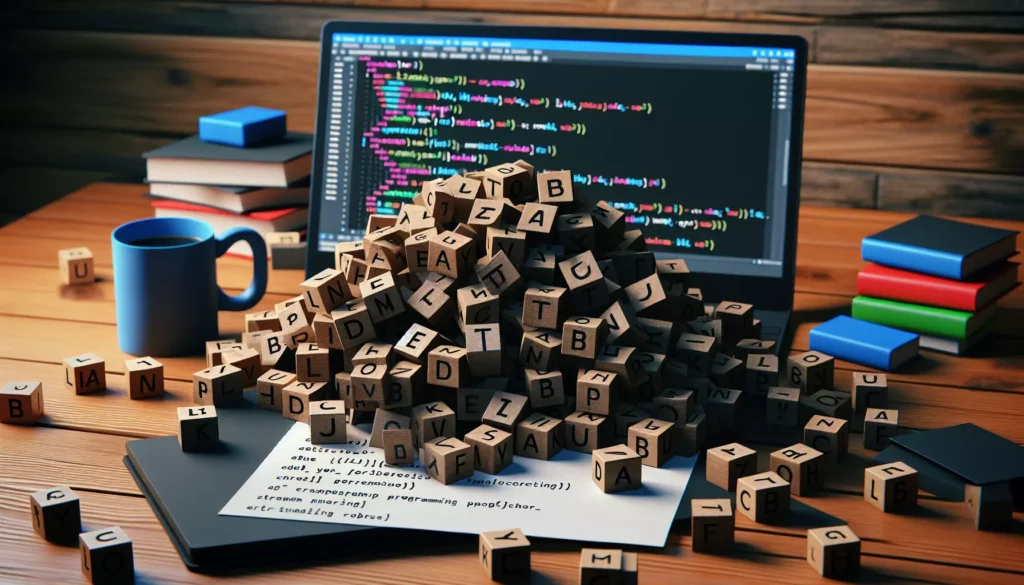
Welcome to another exciting deep dive into algorithmic problem-solving! Today, we’re tackling the Word Break problem, a classic challenge that showcases the power of dynamic programming in string manipulation. This problem is not only a favorite among interviewers at top tech companies like Google, Amazon, and Facebook, but it’s also a fantastic exercise to sharpen your coding skills and algorithmic thinking.
What is the Word Break Problem?
Before we dive into the solution, let’s clearly define the problem:
Given a string
s
and a dictionary of stringswordDict
, determine ifs
can be segmented into a space-separated sequence of one or more dictionary words.
For example:
Input: s = "leetcode", wordDict = ["leet", "code"]
Output: true
Explanation: Return true because "leetcode" can be segmented as "leet code".
This problem might seem straightforward at first glance, but it quickly becomes complex when you consider longer strings and larger dictionaries. That’s where the beauty of dynamic programming comes into play.
Why is the Word Break Problem Important?
The Word Break problem is significant for several reasons:
- Real-world applications: This problem has practical applications in areas like natural language processing, text analysis, and autocorrect systems.
- Dynamic Programming showcase: It’s an excellent example of how dynamic programming can optimize solutions for problems with overlapping subproblems.
- Interview favorite: Due to its balance of difficulty and the opportunity to showcase problem-solving skills, it’s a popular choice in technical interviews.
- Algorithm design skills: Solving this problem requires careful consideration of time and space complexity, pushing you to think critically about efficient algorithm design.
Approaching the Word Break Problem
Let’s break down our approach to solving this problem:
1. Brute Force Approach
The most straightforward approach would be to try all possible word combinations from the dictionary to see if we can construct the given string. However, this method quickly becomes inefficient for longer strings, as the number of combinations grows exponentially.
2. Dynamic Programming Solution
A more efficient approach uses dynamic programming. The key insight is that we can break down the problem into smaller subproblems and store their results to avoid redundant computations.
Here’s the step-by-step thought process:
- Create a boolean array
dp
of lengthn+1
, wheren
is the length of the input strings
. dp[i]
will be true if the substrings[0:i]
can be segmented into words from the dictionary.- Initialize
dp[0]
as true, representing an empty string. - Iterate through the string, for each index
i
:- Check all possible substrings ending at
i
. - If any such substring is in the dictionary and the remaining part (before this substring) is also valid (according to our
dp
array), markdp[i]
as true.
- Check all possible substrings ending at
- The final answer will be stored in
dp[n]
.
Implementing the Solution
Now that we understand the approach, let’s implement it in Python:
def wordBreak(s: str, wordDict: List[str]) -> bool:
n = len(s)
dp = [False] * (n + 1)
dp[0] = True
word_set = set(wordDict) # Convert list to set for O(1) lookup
for i in range(1, n + 1):
for j in range(i):
if dp[j] and s[j:i] in word_set:
dp[i] = True
break
return dp[n]
Let’s break down this implementation:
- We initialize our
dp
array withFalse
values, except fordp[0]
which is set toTrue
(empty string case). - We convert
wordDict
to a set for constant-time lookups. - We iterate through each character of the string (outer loop).
- For each character, we check all possible substrings ending at that character (inner loop).
- If we find a valid word and the remaining part is also valid, we mark the current position as
True
in ourdp
array. - Finally, we return the value of
dp[n]
, which tells us if the entire string can be segmented.
Time and Space Complexity Analysis
Understanding the time and space complexity of our solution is crucial for optimizing performance and scaling to larger inputs.
Time Complexity
The time complexity of our dynamic programming solution is O(n^2 * m), where:
n
is the length of the input strings
m
is the average length of words inwordDict
This is because:
- We have two nested loops iterating over the string, giving us O(n^2) iterations.
- For each iteration, we perform a substring operation and a set lookup, which takes O(m) time on average.
While this is significantly better than the exponential time complexity of the brute force approach, it’s worth noting that for very large inputs, this could still be optimized further.
Space Complexity
The space complexity of our solution is O(n + k), where:
n
is the length of the input strings
(for ourdp
array)k
is the total length of all words inwordDict
(for our word set)
This space complexity is generally considered efficient, as it grows linearly with the input size.
Common Variations and Follow-up Questions
In technical interviews, it’s common for interviewers to ask follow-up questions or present variations of the original problem. Here are some you might encounter:
1. Return All Possible Word Breaks
Instead of just returning a boolean, you might be asked to return all possible ways to break the string into dictionary words. This variation requires a slight modification to our dynamic programming approach, often combined with backtracking.
2. Optimize for Space
You might be challenged to optimize the space complexity. One approach could be to use a sliding window instead of storing the entire dp
array.
3. Handle Very Large Inputs
For extremely large inputs, even O(n^2) time complexity might be too slow. In such cases, you might need to explore more advanced techniques like Trie data structures or suffix arrays.
4. Minimum Number of Breaks
A variation might ask for the minimum number of breaks needed to segment the string into dictionary words. This would require modifying our DP approach to store counts instead of boolean values.
Real-world Applications
Understanding the Word Break problem and its solutions can be incredibly valuable in various real-world scenarios:
1. Natural Language Processing (NLP)
In languages without clear word boundaries (like Chinese), word segmentation is a crucial task. The Word Break problem is at the heart of many word segmentation algorithms used in NLP.
2. Autocorrect and Predictive Text
When you’re typing on your smartphone, the autocorrect feature often needs to determine the most likely word or phrase you’re trying to type. This involves breaking down the input into possible words, similar to our Word Break problem.
3. Search Engines
Search engines often need to break down queries into meaningful components. For instance, “newyorkcity” might need to be interpreted as “new york city”. This is essentially a Word Break problem.
4. Cryptography
In certain cryptographic attacks, like ciphertext-only attacks on simple substitution ciphers, being able to efficiently break down text into valid words is crucial.
Tips for Mastering the Word Break Problem
To truly master this problem and similar dynamic programming challenges, consider the following tips:
- Practice variations: Don’t just stick to the basic problem. Try solving the variations we discussed earlier to deepen your understanding.
- Optimize your solution: Once you have a working solution, always think about how you can make it more efficient in terms of time and space complexity.
- Understand the underlying principles: The Word Break problem is an excellent example of how to apply dynamic programming to string problems. Make sure you understand why DP works well here.
- Implement from scratch: Try implementing the solution without referring to notes. This will help solidify your understanding and improve your coding skills.
- Explain your approach: Practice explaining your solution as if you were in an interview. Being able to clearly communicate your thought process is just as important as coding the solution.
Conclusion
The Word Break problem is a fantastic example of how dynamic programming can be applied to solve complex string manipulation tasks efficiently. By breaking down the problem into smaller subproblems and storing intermediate results, we can avoid redundant computations and achieve a much more efficient solution than brute force approaches.
As you continue your journey in algorithmic problem-solving, remember that problems like Word Break are not just academic exercises. They represent fundamental techniques that are widely applicable in various areas of computer science and software development. Whether you’re preparing for technical interviews at top tech companies or working on real-world applications in NLP or search algorithms, the principles you’ve learned here will serve you well.
Keep practicing, stay curious, and always look for opportunities to optimize and improve your solutions. Happy coding!