Why You’re Ready for Advanced Coding Challenges
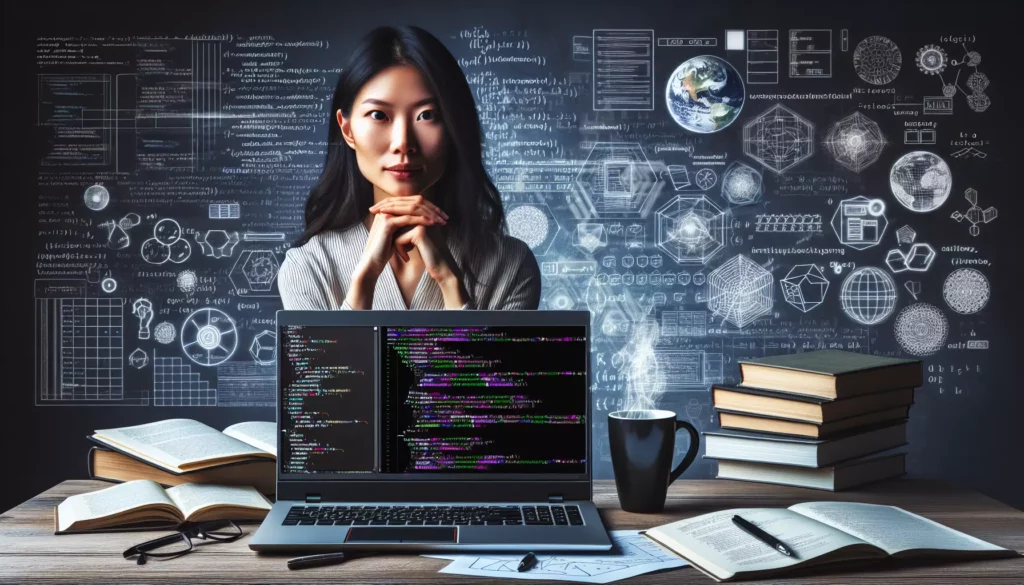
If you’ve been on your coding journey for a while now, you might be wondering if you’re ready to take on more advanced challenges. The truth is, many programmers underestimate their abilities and hesitate to push themselves to the next level. But here’s the thing: if you’re asking this question, chances are you’re more prepared than you think. Let’s explore why you’re likely ready for advanced coding challenges and how embracing them can skyrocket your programming career.
Signs You’re Ready for Advanced Coding
Before we dive into the benefits of tackling more complex coding problems, let’s look at some telltale signs that indicate you’re prepared for the challenge:
- You’ve mastered the basics of at least one programming language
- You can solve simple algorithmic problems with ease
- You’re comfortable with fundamental data structures like arrays, linked lists, and hash tables
- You’ve completed several personal coding projects
- You find yourself curious about optimizing your code for better performance
- You’re interested in learning about more complex algorithms and data structures
If you nodded along to most of these points, congratulations! You’re in a great position to level up your coding skills.
The Benefits of Tackling Advanced Coding Challenges
Now that we’ve established you’re ready let’s explore why embracing advanced coding challenges is crucial for your growth as a programmer:
1. Enhances Problem-Solving Skills
Advanced coding challenges push you to think outside the box. They require you to break down complex problems into smaller, manageable parts and develop innovative solutions. This process significantly improves your problem-solving abilities, a skill that’s invaluable in any programming role.
2. Prepares You for Technical Interviews
If you’re aiming for a position at a top tech company, you’ll need to ace their notoriously challenging technical interviews. These interviews often involve solving complex algorithmic problems on the spot. By regularly tackling advanced coding challenges, you’ll be better prepared to handle the pressure and complexity of these interviews.
3. Broadens Your Understanding of Computer Science
Advanced challenges often introduce you to sophisticated algorithms and data structures you might not encounter in day-to-day coding. This exposure deepens your understanding of computer science principles, making you a more well-rounded programmer.
4. Improves Code Efficiency
As you solve more complex problems, you’ll naturally start thinking about code efficiency and optimization. You’ll learn to write cleaner, faster, and more scalable code – skills that are highly valued in the industry.
5. Boosts Confidence
Successfully solving difficult problems is a great confidence booster. It reassures you of your abilities and motivates you to take on even greater challenges in your coding journey.
How to Approach Advanced Coding Challenges
Now that you’re convinced of the benefits, here’s how you can effectively approach advanced coding challenges:
1. Start with a Strong Foundation
Before diving into advanced topics, ensure you have a solid grasp of the fundamentals. Review basic data structures, algorithms, and time complexity analysis. This foundation will make it easier to understand and implement more complex concepts.
2. Practice Regularly
Consistency is key when it comes to improving your coding skills. Set aside time each day or week to work on coding challenges. Even 30 minutes a day can make a significant difference over time.
3. Use the Right Resources
There are numerous platforms and resources available for practicing advanced coding challenges. However, not all of them provide the structured learning experience you need to truly excel. This is where AlgoCademy comes in.
AlgoCademy offers a unique, AI-powered approach to mastering advanced coding challenges. With its interactive tutorials, step-by-step guidance, and focus on algorithmic thinking, AlgoCademy is designed to take you from a competent coder to a problem-solving maestro ready to ace any technical interview.
4. Analyze and Learn from Solutions
After attempting a problem, don’t just move on to the next one. Take time to analyze your solution and compare it with other efficient solutions. This practice will help you learn new techniques and approaches to problem-solving.
5. Focus on Understanding, Not Just Solving
While solving problems is important, understanding the underlying concepts is crucial. AlgoCademy’s approach emphasizes this by providing detailed explanations and visualizations of complex algorithms and data structures, ensuring you grasp the core concepts behind each solution.
Common Advanced Coding Challenges You Might Encounter
To give you a taste of what’s ahead, here are some common types of advanced coding challenges you might encounter:
1. Dynamic Programming Problems
Dynamic programming is a method for solving complex problems by breaking them down into simpler subproblems. It’s often used in optimization problems. Here’s a simple example of a dynamic programming problem:
def fibonacci(n):
if n <= 1:
return n
fib = [0] * (n + 1)
fib[1] = 1
for i in range(2, n + 1):
fib[i] = fib[i-1] + fib[i-2]
return fib[n]
print(fibonacci(10)) # Output: 55
This code calculates the nth Fibonacci number using dynamic programming, which is much more efficient than the naive recursive approach for large values of n.
2. Graph Algorithms
Graph problems are common in advanced coding challenges. They often involve traversing or analyzing complex networks. Here’s a simple implementation of depth-first search (DFS) on a graph:
def dfs(graph, start, visited=None):
if visited is None:
visited = set()
visited.add(start)
print(start)
for next in graph[start] - visited:
dfs(graph, next, visited)
return visited
graph = {'0': set(['1', '2']),
'1': set(['0', '3', '4']),
'2': set(['0']),
'3': set(['1']),
'4': set(['2', '3'])}
dfs(graph, '0')
This code performs a depth-first search on a graph represented as an adjacency list.
3. Advanced Data Structures
You might encounter problems that require the use of more complex data structures like balanced binary search trees, heaps, or tries. Here’s a simple implementation of a min-heap:
import heapq
class MinHeap:
def __init__(self):
self.heap = []
def parent(self, i):
return (i - 1) // 2
def insert_key(self, k):
heapq.heappush(self.heap, k)
def decrease_key(self, i, new_val):
self.heap[i] = new_val
while i != 0 and self.heap[self.parent(i)] > self.heap[i]:
self.heap[i], self.heap[self.parent(i)] = self.heap[self.parent(i)], self.heap[i]
i = self.parent(i)
def extract_min(self):
return heapq.heappop(self.heap)
def delete_key(self, i):
self.decrease_key(i, float("-inf"))
self.extract_min()
def get_min(self):
return self.heap[0]
heap = MinHeap()
heap.insert_key(3)
heap.insert_key(2)
heap.delete_key(1)
heap.insert_key(15)
heap.insert_key(5)
heap.insert_key(4)
heap.insert_key(45)
print(heap.extract_min())
print(heap.get_min())
heap.decrease_key(2, 1)
print(heap.get_min())
This code implements a min-heap data structure with various operations like insert, delete, and extract minimum.
How AlgoCademy Can Help You Master Advanced Coding Challenges
While these examples give you a glimpse into the world of advanced coding challenges, mastering them requires a structured approach and consistent practice. This is where AlgoCademy shines.
1. Personalized Learning Path
AlgoCademy uses AI to create a personalized learning path based on your current skill level and learning goals. Whether you’re just starting with advanced challenges or preparing for FAANG interviews, AlgoCademy tailors its content to your needs.
2. Interactive Coding Environment
With AlgoCademy’s interactive coding environment, you can practice coding challenges directly in your browser. This hands-on approach allows you to immediately apply what you’ve learned, reinforcing your understanding of complex concepts.
3. Step-by-Step Guidance
For each challenge, AlgoCademy provides step-by-step guidance. If you’re stuck, you can get hints or see a detailed explanation of the solution. This feature ensures you’re never left frustrated or unable to progress.
4. Focus on Algorithmic Thinking
AlgoCademy doesn’t just teach you to solve specific problems; it trains you to think algorithmically. This skill is crucial for tackling any advanced coding challenge you might encounter in your career or during interviews.
5. Comprehensive Coverage
From dynamic programming to advanced graph algorithms, AlgoCademy covers all the topics you need to master to excel in technical interviews and real-world programming scenarios.
6. Progress Tracking
AlgoCademy tracks your progress, allowing you to see how you’re improving over time. This feature can be incredibly motivating and helps you identify areas where you might need more practice.
Taking the Next Step in Your Coding Journey
You’ve come a long way in your coding journey. You’ve mastered the basics, built projects, and now you’re ready for the next challenge. Advanced coding problems might seem daunting at first, but with the right approach and resources, you can conquer them.
Remember, every expert was once a beginner. The key to mastering advanced coding challenges is consistent practice, a structured learning approach, and the right guidance. With AlgoCademy, you have all these elements at your fingertips.
So, are you ready to take your coding skills to the next level? Are you prepared to tackle the challenges that will set you apart in technical interviews and your programming career? If your answer is yes (and we believe it is), then it’s time to embrace advanced coding challenges.
Don’t let self-doubt hold you back. You’re more ready than you think. With AlgoCademy as your coding companion, you’ll be solving complex algorithms, optimizing challenging problems, and acing technical interviews before you know it.
Your journey to becoming an elite programmer starts now. Embrace the challenge, push your limits, and watch your coding skills soar to new heights with AlgoCademy. Your future in tech is bright, and it’s time to shine!