Why You’re Not Learning From Your Mistakes When Coding
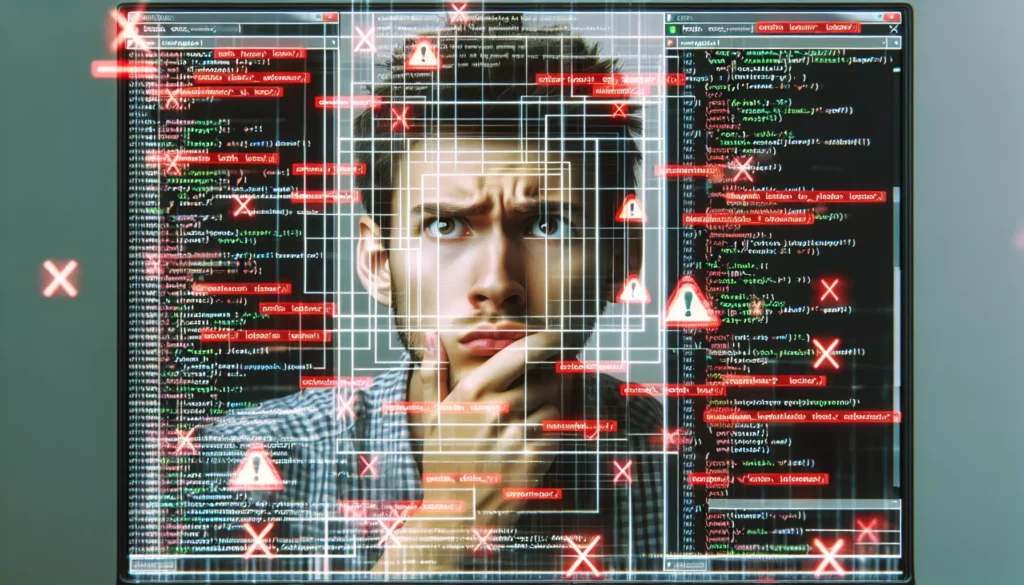
When you’re learning to code, mistakes are inevitable. In fact, they’re an essential part of the learning process. But there’s a crucial difference between making mistakes and actually learning from them. Many aspiring programmers find themselves trapped in a cycle of repeating the same errors, wondering why they aren’t making progress despite putting in hours of practice.
The truth is that simply making mistakes isn’t enough—you need to develop a systematic approach to understanding, analyzing, and learning from them. In this comprehensive guide, we’ll explore why you might be struggling to learn from your coding mistakes and provide actionable strategies to transform those errors into valuable learning opportunities.
Table of Contents
- Understanding the Value of Mistakes in Coding
- Common Patterns That Prevent Learning From Mistakes
- Effective Strategies for Learning From Coding Errors
- Developing a Systematic Debugging Process
- The Power of Documenting Your Mistakes
- Cultivating a Growth Mindset for Coding
- Tools and Resources to Support Your Learning Journey
- Advanced Techniques for Error Analysis
- Conclusion: Transforming Mistakes into Mastery
Understanding the Value of Mistakes in Coding
Mistakes in programming aren’t just inevitable—they’re valuable. Every error presents an opportunity to deepen your understanding of programming concepts, syntax, and problem-solving approaches. The most successful developers aren’t those who make the fewest mistakes; they’re the ones who extract the most learning from each error they encounter.
Consider how children learn to walk. They don’t read instructions or watch tutorials—they try, fall, adjust, and try again. Each fall provides feedback that helps them refine their approach. Programming works similarly: the feedback loop of writing code, encountering errors, fixing them, and trying again builds neural pathways that strengthen your coding abilities.
Research in cognitive science supports this view. A study published in the Journal of Experimental Psychology found that making errors during learning can actually lead to better long-term retention of information, provided those errors are corrected and understood. This phenomenon, known as “productive failure,” suggests that struggling with problems before finding solutions leads to deeper learning than being shown the correct method immediately.
Types of Coding Mistakes
Understanding the different categories of coding mistakes can help you approach them more systematically:
- Syntax errors: Mistakes in the “grammar” of the programming language, like missing semicolons or brackets.
- Logic errors: Flaws in the reasoning or algorithm that lead to incorrect results.
- Runtime errors: Issues that occur during program execution, often due to unexpected inputs or states.
- Conceptual errors: Misunderstandings about how programming concepts or data structures work.
- Design errors: Poor architectural decisions that lead to code that’s difficult to maintain or scale.
Each type of error requires a different approach to learning, but all can be valuable if properly analyzed.
Common Patterns That Prevent Learning From Mistakes
If you’re not learning from your coding mistakes, you might be falling into one or more of these common patterns:
1. Copy-Pasting Solutions Without Understanding
When faced with an error, it’s tempting to search for a solution online, find some code that fixes the problem, and paste it into your project without truly understanding why it works. While this might solve your immediate problem, it robs you of the learning opportunity the error presented.
This pattern is particularly common when working with complex frameworks or libraries. You might find yourself implementing solutions from Stack Overflow that “just work” without understanding the underlying principles. Over time, this creates knowledge gaps that will eventually catch up with you.
2. Focusing Only on Making Code Work
Many beginners measure success solely by whether their code runs without errors. This “make it work” mentality prioritizes short-term success over long-term learning. When your code finally executes correctly, you might feel relieved and immediately move on to the next challenge without reflecting on what went wrong and why your solution fixed it.
This approach misses the crucial reflection phase where deep learning occurs. The most valuable insights often come not from making the code work, but from understanding why it wasn’t working in the first place.
3. Emotional Reactions to Errors
Programming can be emotionally challenging. When you’ve spent hours on a problem only to face a wall of error messages, it’s natural to feel frustrated, discouraged, or even angry. These emotional reactions can prevent objective analysis of what went wrong.
If you find yourself getting emotionally overwhelmed by coding errors, you might start avoiding challenging problems altogether or developing negative associations with certain topics or languages. This emotional avoidance limits your growth as a programmer.
4. Rushing Through Problems
In our fast-paced world, there’s constant pressure to learn quickly and produce results. This can lead to rushing through coding problems without taking the time to fully understand them. When you encounter an error, you might implement the first solution that comes to mind rather than exploring multiple approaches and understanding the trade-offs.
This rushed approach often leads to superficial understanding and missed learning opportunities. The most effective learning happens when you give yourself permission to slow down and explore problems deeply.
5. Inconsistent Problem-Solving Approaches
Without a systematic approach to debugging and problem-solving, you might tackle each error differently, leading to inconsistent results and missed patterns. If you don’t have a reliable process for analyzing and learning from mistakes, valuable insights can slip through the cracks.
Developing a consistent methodology for approaching errors ensures that you extract maximum learning from each mistake and build a solid foundation of problem-solving skills.
Effective Strategies for Learning From Coding Errors
Now that we’ve identified common obstacles, let’s explore strategies that can help you transform coding errors into valuable learning experiences:
1. Practice Deliberate Error Analysis
When you encounter an error, resist the urge to immediately search for solutions. Instead, take time to analyze the error message and the surrounding code. Ask yourself:
- What exactly is the error telling me?
- What was I trying to accomplish with this code?
- What assumptions did I make that might be incorrect?
- Have I seen similar errors before, and what did they mean?
This deliberate analysis helps you develop a deeper understanding of the programming language and runtime environment. Over time, you’ll become better at interpreting error messages and identifying the root causes of problems.
2. Implement the “Explain It Like I’m Five” Technique
One powerful way to ensure you understand an error and its solution is to explain it in simple terms, as if you were explaining it to a five-year-old. This technique, sometimes called the “rubber duck debugging method,” forces you to break down complex concepts into simple language.
You can do this by:
- Explaining the error out loud to yourself or an imaginary listener
- Writing a simple explanation in a journal or documentation
- Teaching the concept to a peer or mentor
If you can’t explain the error and solution in simple terms, it’s a sign that you don’t fully understand it yet and should dig deeper.
3. Create a Personal Error Library
Start documenting the errors you encounter and the solutions you discover. This personal error library becomes an invaluable resource as you progress in your coding journey. For each error, record:
- The exact error message
- The context in which it occurred
- Your initial hypotheses about the cause
- The solution you implemented
- Why that solution worked
- Any resources or references that helped you
Over time, this library will reveal patterns in your mistakes and help you develop more efficient problem-solving strategies. It also serves as a personalized reference that’s tailored to your specific learning journey.
4. Practice Active Recall
After solving a problem, don’t just move on. Take time to actively recall the error and solution without looking at your notes or code. Try to explain to yourself:
- What was the root cause of the error?
- How did you identify that cause?
- What solution did you implement and why?
- What would you do differently next time?
This practice of active recall strengthens neural connections and improves long-term retention of what you’ve learned. It transforms passive exposure to errors into active learning experiences.
5. Implement Spaced Repetition
Spaced repetition is a learning technique that involves reviewing information at increasing intervals. Apply this to your coding errors by:
- Reviewing your error library regularly
- Recreating challenging problems from memory
- Testing yourself on concepts that previously caused errors
This systematic review ensures that you retain what you’ve learned from past mistakes and can apply those lessons to new challenges.
Developing a Systematic Debugging Process
A structured approach to debugging can significantly improve your ability to learn from mistakes. Here’s a step-by-step process you can adapt to your own needs:
1. Reproduce the Error
Before you can fix an error, you need to reliably reproduce it. This means understanding the exact conditions under which the error occurs. Create a minimal test case that demonstrates the issue without unnecessary complexity.
For example, if you’re experiencing an error in a complex web application, try to isolate the problematic code into a simple script that reproduces the error. This isolation helps you focus on the core issue without distractions.
2. Read the Error Message Carefully
Error messages contain valuable information, but many beginners skim over them or feel intimidated by technical language. Take time to read the entire message and understand each component:
- The type of error (e.g., SyntaxError, TypeError, ReferenceError)
- The specific message describing what went wrong
- The location (file and line number) where the error occurred
- The stack trace showing the sequence of function calls leading to the error
Learning to decode error messages is a skill that improves with practice and pays enormous dividends in your programming career.
3. Form a Hypothesis
Based on the error message and your understanding of the code, form a hypothesis about what might be causing the problem. Be specific and testable. For example, instead of thinking “Something’s wrong with my loop,” hypothesize that “The loop might be continuing past the end of the array because I’m using the wrong condition in my termination check.”
This hypothesis-driven approach develops your analytical thinking and helps you build mental models of how code executes.
4. Test Your Hypothesis
Modify your code based on your hypothesis and see if it resolves the error. If not, refine your hypothesis based on new information. This scientific approach to debugging helps you develop a deeper understanding of programming concepts and how they interact.
Tools like debuggers, print statements, and logging can provide additional information to test your hypotheses. For example:
// Using console.log to test a hypothesis about variable values
function calculateTotal(items) {
console.log("Items received:", items);
let total = 0;
for (let i = 0; i <= items.length; i++) {
console.log(`Processing item at index ${i}:`, items[i]);
total += items[i].price;
}
return total;
}
The console logs might reveal that you’re accessing an index that doesn’t exist, helping you identify an off-by-one error in your loop condition.
5. Reflect and Generalize
After fixing the error, take time to reflect on what you learned. Ask yourself:
- What was the root cause of this error?
- Is this a common type of mistake I make?
- What general principle can I extract from this experience?
- How can I prevent similar errors in the future?
This reflection helps you generalize your learning beyond the specific error to broader programming concepts and patterns.
The Power of Documenting Your Mistakes
Documentation is a powerful tool for learning from mistakes. By recording your errors and solutions, you create a personalized learning resource that grows with you. Here are effective documentation strategies:
1. Maintain a Coding Journal
A coding journal can be as simple as a text file or notebook where you record your daily experiences, challenges, and insights. For each coding session, note:
- What you were trying to accomplish
- Errors or challenges you encountered
- How you resolved those issues
- New concepts or techniques you learned
- Questions that arose during your work
Reviewing this journal periodically helps you identify patterns in your learning and areas that need more attention.
2. Create Annotated Code Examples
When you solve a particularly challenging problem, save the code with detailed annotations explaining:
// Problem: Function was returning NaN when summing prices
// Root cause: Some price values were strings instead of numbers
// Solution: Convert all values to numbers before adding
function calculateTotal(items) {
let total = 0;
for (let i = 0; i < items.length; i++) {
// Convert price to number to ensure proper addition
// This prevents string concatenation when price is a string
total += Number(items[i].price);
}
return total;
}
These annotated examples serve as valuable references when you encounter similar issues in the future.
3. Build a Personal Wiki or Knowledge Base
For more structured documentation, consider creating a personal wiki or knowledge base. Tools like Notion, Obsidian, or even GitHub repositories can help you organize your coding knowledge by topics, languages, or error types.
Within this knowledge base, create templates for documenting errors that include:
- Error category (syntax, logic, runtime, etc.)
- Specific error message
- Code context
- Root cause analysis
- Solution implemented
- Related concepts or patterns
- Resources for further learning
This structured approach makes it easier to review and connect related concepts over time.
4. Share Your Learnings
One of the most effective ways to solidify your understanding is to share what you’ve learned with others. Consider:
- Writing blog posts about interesting errors you’ve solved
- Contributing to Stack Overflow or other community forums
- Creating tutorials or walkthroughs for common issues
- Mentoring other learners and helping them understand similar concepts
Teaching forces you to clarify your thinking and often reveals gaps in your understanding that you can then address.
Cultivating a Growth Mindset for Coding
Your mindset significantly impacts how you respond to and learn from mistakes. Developing a growth mindset—the belief that abilities can be developed through dedication and hard work—is crucial for effective learning from coding errors.
1. Embrace Challenges as Opportunities
Instead of seeing difficult coding problems as threats to your confidence or competence, view them as opportunities to stretch your abilities. Research by psychologist Carol Dweck shows that people with a growth mindset seek out challenges and persist longer when faced with obstacles.
When you encounter a particularly difficult error, remind yourself: “This is challenging, but working through it will make me a better programmer.”
2. Value Process Over Outcome
In coding, as in many fields, the journey often teaches more than the destination. Focus on the quality of your problem-solving process rather than just whether you ultimately fixed the error.
Ask yourself:
- Was my approach systematic and thoughtful?
- Did I consider multiple possibilities?
- What did I learn about debugging techniques?
- How did my understanding deepen through this process?
This process-oriented approach helps you extract value from every coding experience, regardless of the immediate outcome.
3. Practice Self-Compassion
Programming can be frustrating, and it’s easy to be hard on yourself when facing persistent errors. Research shows that self-compassion—treating yourself with the same kindness you would offer a friend—actually improves learning outcomes and resilience.
When you make a mistake, avoid negative self-talk like “I’m terrible at this” or “I’ll never get it.” Instead, acknowledge the difficulty and your feelings about it: “This is challenging, and it’s normal to feel frustrated. What can I learn from this situation?”
4. Develop a Curiosity Orientation
Approach errors with curiosity rather than judgment. Instead of thinking “I made a stupid mistake,” ask “What’s happening here? Why did the system respond this way?”
This curiosity orientation transforms errors from failures into fascinating puzzles to solve. It also reduces emotional reactivity and helps you maintain a clearer analytical perspective.
5. Recognize the Role of Effort and Strategy
In a growth mindset, effort and strategy are seen as pathways to mastery. When you encounter a difficult error, focus on:
- Adjusting your effort (perhaps you need to spend more time understanding the underlying concepts)
- Refining your strategy (maybe your current debugging approach isn’t effective for this type of problem)
- Seeking appropriate resources (tutorials, documentation, or mentorship)
This approach keeps you focused on factors within your control rather than attributing difficulties to fixed traits like “talent” or “intelligence.”
Tools and Resources to Support Your Learning Journey
The right tools and resources can significantly enhance your ability to learn from coding mistakes. Here are some recommendations:
1. Debugging Tools
Modern integrated development environments (IDEs) and browsers come with powerful debugging tools that help you understand what’s happening in your code:
- Integrated debuggers: Tools like VS Code’s debugger, Chrome DevTools, or PyCharm’s debugger allow you to set breakpoints, inspect variables, and step through code execution.
- Linters and static analyzers: Tools like ESLint (JavaScript), Pylint (Python), or RuboCop (Ruby) can identify potential errors before you even run your code.
- Error tracking services: For larger projects, services like Sentry or Rollbar help you track and analyze runtime errors.
Learning to use these tools effectively gives you deeper insights into your code’s behavior and helps you identify the root causes of errors more quickly.
2. Educational Resources for Error Understanding
Certain resources are particularly valuable for understanding common errors and their solutions:
- Language-specific error guides: Many programming languages have guides dedicated to common errors and their resolutions.
- Interactive debugging tutorials: Platforms like Codecademy or freeCodeCamp offer courses specifically on debugging and error handling.
- Error-centered books: Books like “Debugging: The 9 Indispensable Rules for Finding Even the Most Elusive Software and Hardware Problems” by David J. Agans provide systematic approaches to error analysis.
These resources help you develop a more structured understanding of errors and how to approach them.
3. Community Support
Learning from others’ experiences can accelerate your growth:
- Stack Overflow: Beyond just finding solutions, study how experienced developers analyze and solve problems.
- GitHub Issues: Examining how open-source projects handle bugs can teach you about systematic debugging approaches.
- Coding meetups and study groups: Discussing errors and solutions with peers provides new perspectives on problem-solving.
- Mentorship: Working with a more experienced developer can help you identify blind spots in your error analysis approach.
These community resources expose you to different problem-solving styles and help you build a network of support for your coding journey.
4. Knowledge Management Tools
Organizing what you learn from mistakes is crucial for long-term retention:
- Note-taking apps: Tools like Notion, Evernote, or Obsidian help you create structured notes about errors and solutions.
- Spaced repetition software: Applications like Anki allow you to create flashcards for concepts that frequently cause errors, ensuring regular review.
- Personal knowledge bases: Tools like Foam or Roam Research help you connect related concepts and build a network of programming knowledge.
These tools support the documentation strategies discussed earlier and help you build a personalized learning system.
Advanced Techniques for Error Analysis
As you progress in your coding journey, consider these more advanced approaches to learning from mistakes:
1. Root Cause Analysis
Root cause analysis (RCA) is a systematic approach to identifying the fundamental cause of a problem. While often used in industrial settings, it’s equally valuable for coding errors:
- Define the problem: Clearly articulate what went wrong and the impact it had.
- Collect data: Gather all relevant information about the error, including context, inputs, and expected vs. actual outputs.
- Identify possible causes: Brainstorm all potential factors that might have contributed to the error.
- Determine root causes: Use techniques like the “5 Whys” (asking “why” repeatedly to dig deeper) to identify fundamental causes rather than symptoms.
- Develop solutions: Create and implement countermeasures to address the root causes.
- Verify effectiveness: Ensure that your solutions actually prevent the error from recurring.
This structured approach helps you move beyond surface-level fixes to address deeper issues in your code or understanding.
2. Error Pattern Recognition
Over time, you’ll start to recognize patterns in your errors. Developing this pattern recognition ability accelerates your learning:
- Periodically review your error documentation to identify recurring themes
- Create categories or taxonomies of errors you frequently encounter
- Develop personalized “error profiles” that highlight your common mistake patterns
- Create checklists for error-prone areas based on your historical patterns
This meta-analysis of your errors helps you develop targeted strategies for improvement and anticipate potential issues before they occur.
3. Deliberate Practice with Error Scenarios
Rather than waiting to encounter errors organically, you can create deliberate practice sessions focused on error handling:
- Create “broken code” exercises where you intentionally introduce errors and then fix them
- Practice reading and interpreting error messages without immediately looking at the code
- Set up pair programming sessions where one person introduces errors and the other debugs
- Challenge yourself to predict what errors might occur in a given piece of code
This proactive approach to error learning helps you build error-handling skills more systematically than simply reacting to errors as they arise.
4. Mental Models and Visualization
Developing strong mental models of how code executes helps you predict and understand errors:
- Practice tracing code execution mentally, step by step
- Create visual representations of data structures and algorithms
- Use memory diagrams to track variable states during execution
- Compare your mental execution model with actual program behavior
These visualization techniques help you develop intuition about how code works, making it easier to spot potential errors and understand why they occur.
Conclusion: Transforming Mistakes into Mastery
Learning to code is not a linear journey but a spiral of exploration, error, and insight. The most successful programmers aren’t those who avoid mistakes but those who transform each error into a stepping stone toward greater understanding and skill.
By implementing the strategies outlined in this guide—from systematic debugging processes to documentation practices, from mindset shifts to advanced error analysis techniques—you can break free from the cycle of repeating the same mistakes and start extracting maximum value from every error you encounter.
Remember that this transformation doesn’t happen overnight. It requires consistent practice, reflection, and a willingness to engage deeply with the challenges of programming. But with each error you analyze and learn from, you build not just technical knowledge but also the meta-skills of problem-solving, perseverance, and self-directed learning that characterize truly exceptional developers.
The next time you encounter a perplexing error message or a bug that seems impossible to fix, pause and remind yourself: this isn’t just an obstacle to overcome—it’s an opportunity to grow. With the right approach, every mistake becomes a master class in coding, custom-designed for your unique learning journey.
Happy coding, and even happier debugging!