Why You’re Memorizing Syntax But Still Can’t Solve Real Problems
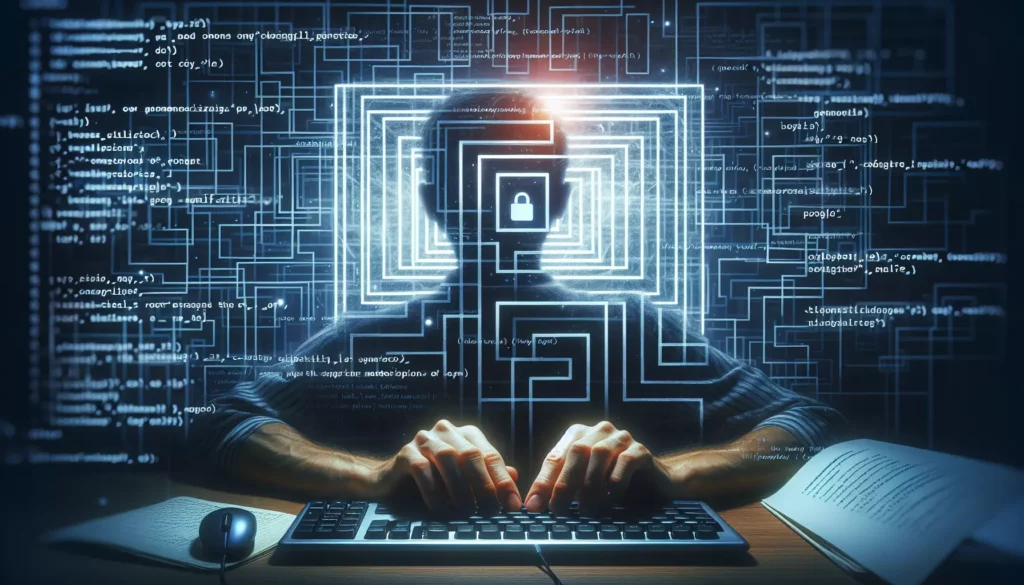
We’ve all been there: spending hours memorizing programming language syntax, feeling confident with our knowledge of loops, conditionals, and data structures, only to freeze when faced with an actual coding problem. This disconnect between knowing syntax and solving problems is a common frustration for many aspiring programmers.
In this comprehensive guide, we’ll explore why simply memorizing syntax isn’t enough to become a proficient programmer, and provide actionable strategies to bridge the gap between syntax knowledge and problem-solving abilities.
The Syntax Trap: Why Memorization Isn’t Enough
Learning to code is often compared to learning a new language, but this analogy can be misleading. In natural languages, knowing vocabulary and grammar rules can get you quite far in communication. In programming, however, syntax knowledge is merely the entry point.
The False Comfort of Syntax Mastery
Many beginners fall into what we call the “syntax trap” – the belief that memorizing all the commands, functions, and language constructs will make them competent programmers. They might spend weeks perfecting their understanding of array methods or string manipulations, only to discover they can’t use this knowledge to build even simple applications.
Consider this scenario: You’ve memorized how to declare variables, write loops, and create functions in JavaScript. But when asked to write a program that finds the most frequent character in a string, you’re stumped. Why? Because real programming involves combining these syntax elements in logical ways to solve problems – a skill that memorization alone doesn’t develop.
The Illusion of Understanding
When you follow along with tutorials or copy code from examples, you might feel like you understand the material. This is what cognitive scientists call the “illusion of understanding” – the mistaken belief that comprehension has occurred when it actually hasn’t.
Reading or copying code activates recognition memory, which is different from the recall and application skills needed for problem-solving. You might recognize a for-loop when you see it, but generating one from scratch to solve a specific problem requires deeper understanding.
The Missing Pieces: What You Need Beyond Syntax
If syntax knowledge isn’t enough, what else do you need to become an effective problem solver? Let’s explore the critical components that transform syntax knowledge into practical programming ability.
Algorithmic Thinking
Algorithmic thinking is the ability to break down problems into sequential, logical steps. It’s about developing a mental model of how to approach problems systematically.
For example, if you need to find the largest number in an array, algorithmic thinking helps you formulate a step-by-step approach:
- Start by assuming the first element is the largest
- Compare each subsequent element to your current “largest”
- If you find a larger element, update your “largest” value
- Continue until you’ve examined all elements
- Return the “largest” value
This thought process is independent of any specific programming language. Once you have the algorithm, translating it into code becomes much easier, regardless of the syntax you’re using.
Pattern Recognition
Experienced programmers recognize common patterns in problems. They know that certain problem types call for specific approaches:
- Need to process each element in a collection? That’s an iteration pattern.
- Need to find something in a sorted array quickly? That’s a binary search pattern.
- Need to build something up incrementally? That might be a dynamic programming pattern.
Developing this pattern recognition takes time and exposure to many different problems. As you solve more problems, you’ll start recognizing similarities and can apply proven strategies rather than starting from scratch each time.
Mental Models of Program Execution
Strong programmers have a clear mental model of how their code executes. They can “run the program in their head,” tracing through the steps the computer will take and predicting the outcomes.
This ability helps tremendously with debugging and allows programmers to detect issues before they even run their code. For example, when working with recursion, being able to mentally trace through the call stack helps understand why a particular solution works or fails.
The Problem-Solving Mindset
Beyond technical skills, effective problem-solving requires developing the right mindset – a way of approaching challenges that fosters creativity and persistence.
Embracing the Struggle
Real learning happens when you struggle with problems. In fact, cognitive science shows that the more you have to work for the solution, the better you’ll remember it. This concept, known as “desirable difficulty,” explains why passive learning (reading or watching videos) is less effective than active problem-solving.
Next time you’re tempted to look up a solution immediately, remember that the struggle itself is valuable. Give yourself time to work through the problem, even if it feels uncomfortable.
Developing Resilience
Programming involves constant problem-solving, which means you’ll encounter frequent failures and dead ends. Developing resilience – the ability to persist despite setbacks – is crucial for long-term success.
When you hit a wall with a problem, instead of thinking “I can’t solve this,” try reframing it as “I haven’t solved this yet.” This growth mindset keeps you engaged with the challenge rather than defeated by it.
Curiosity Over Memorization
The most effective programmers are driven by curiosity – they want to understand how things work, not just memorize what to do. This curiosity leads them to experiment, explore edge cases, and build a deeper understanding of programming concepts.
Instead of memorizing a specific solution to a sorting problem, for instance, get curious about why one algorithm is more efficient than another. This deeper understanding will serve you better in the long run.
Bridging the Gap: Practical Strategies to Improve Problem-Solving
Now that we understand what’s missing, let’s explore practical strategies to transform syntax knowledge into problem-solving ability.
Strategy 1: Practice Deliberate Problem Decomposition
When faced with a complex problem, resist the urge to start coding immediately. Instead, practice breaking the problem down into smaller, manageable sub-problems.
For example, if tasked with building a to-do list application, you might break it down as follows:
- Create a data structure to store tasks
- Implement functionality to add a new task
- Implement functionality to mark a task as complete
- Implement functionality to delete a task
- Create a user interface to interact with these functions
By tackling each sub-problem individually, you make progress without getting overwhelmed by the complexity of the entire project.
Strategy 2: Solve Problems Before Coding
Before writing any code, solve the problem conceptually. Use pseudocode or plain English to outline your approach. This separates the logical thinking from the syntax concerns, allowing you to focus on the algorithm first.
For example, to find the most frequent character in a string, you might write:
1. Create a dictionary to count occurrences of each character
2. For each character in the string:
a. If character is in dictionary, increment its count
b. Otherwise, add it to dictionary with count 1
3. Find the character with the highest count in the dictionary
4. Return that character
Once you have this logical framework, translating it to code becomes much more straightforward.
Strategy 3: Implement the Feynman Technique
Named after physicist Richard Feynman, this technique involves explaining a concept in simple terms as if teaching it to someone else. If you can’t explain your solution clearly, you probably don’t understand it well enough.
After solving a problem, practice explaining your solution out loud, focusing on:
- Why you chose this approach
- How the algorithm works step by step
- What other approaches you considered and why you didn’t use them
This process reinforces your understanding and helps identify any gaps in your reasoning.
Strategy 4: Start with Paper, Not IDE
Modern IDEs with autocomplete and syntax highlighting make it easy to write code without fully understanding it. Try solving problems on paper first, where you have no syntax checking or documentation lookup.
This approach forces you to rely on your understanding rather than tools, revealing areas where your knowledge might be superficial. It also mimics the environment of many technical interviews, providing valuable practice.
Strategy 5: Practice Consistent Trace-Through
Develop the habit of manually tracing through your code with specific examples before running it. This builds your mental model of program execution and helps catch logical errors early.
For example, if you write a function to reverse a string, trace through its execution with the input “hello” by tracking the state of each variable at each step. This exercise strengthens your ability to predict how code will behave.
Building a Problem-Solving Toolkit
As you develop your problem-solving skills, it’s helpful to build a toolkit of strategies for tackling different types of problems.
The Brute Force Approach
Always start by considering the simplest, most direct solution, even if it’s not efficient. This “brute force” approach gives you a baseline solution that you can optimize later.
For example, to find if a list contains duplicate elements, a brute force approach would compare each element with every other element:
function hasDuplicates(array) {
for (let i = 0; i < array.length; i++) {
for (let j = i + 1; j < array.length; j++) {
if (array[i] === array[j]) {
return true;
}
}
}
return false;
}
While this isn’t the most efficient solution (it has O(n²) time complexity), it’s correct and provides a starting point for optimization.
Working Backwards
Sometimes it’s easier to start with the desired outcome and work backwards to the initial state. This approach is particularly useful for problems involving transformations or state changes.
For instance, if you need to find the input that produces a specific output when passed through a function, start with the output and reverse the operations to find the input.
Simplify and Generalize
When facing a complex problem, try solving a simpler version first, then generalize your solution. This incremental approach builds confidence and often reveals insights about the full problem.
For example, before tackling sorting an array of objects based on multiple criteria, start by sorting an array of numbers. Once that works, extend your solution to handle the more complex case.
Divide and Conquer
Many efficient algorithms use a divide-and-conquer approach, breaking a problem into smaller instances of the same problem, solving those instances, and combining the results.
Classic examples include merge sort and binary search. Understanding this pattern helps you recognize when it can be applied to new problems.
Using Data Structures Effectively
Often, selecting the right data structure is half the battle in solving a problem efficiently. Different data structures excel at different operations:
- Arrays provide fast access by index but slow insertions/deletions
- Hash tables offer fast lookups, insertions, and deletions
- Trees are excellent for hierarchical data and maintaining order
- Graphs represent complex relationships between entities
Understanding the strengths and weaknesses of each data structure helps you make informed choices when designing solutions.
Common Obstacles to Problem-Solving Growth
As you work to improve your problem-solving skills, be aware of these common obstacles that can hinder your progress.
Tutorial Hell
“Tutorial hell” refers to the state of endlessly consuming tutorials without applying what you learn to solve real problems. While tutorials provide valuable information, they typically guide you through pre-defined problems with clear solutions.
To escape tutorial hell, set a ratio of learning to application – for every hour of tutorial content, spend at least two hours solving problems independently. This forces you to apply your knowledge in new contexts.
Premature Optimization
Worrying too much about writing the most efficient code from the start can paralyze your problem-solving process. Remember the programming adage: “Make it work, make it right, make it fast” – in that order.
First focus on creating a solution that correctly solves the problem, then refine it for readability and maintainability, and only then optimize for performance if necessary.
Isolation
Programming in isolation limits your exposure to different approaches and problem-solving strategies. Engaging with a community – whether online forums, local meetups, or study groups – exposes you to diverse perspectives and solutions.
Code reviews, pair programming, and discussing problems with others can highlight blind spots in your thinking and introduce you to new techniques.
Fear of Starting from Scratch
Many programmers hesitate to discard their initial approach, even when it’s not working well. This sunk cost fallacy can prevent you from finding more elegant solutions.
Practice the art of starting over – if you’ve spent significant time on an approach without progress, try setting it aside and approaching the problem from a completely different angle.
Measuring Your Progress: Beyond Syntax Tests
How do you know if you’re improving as a problem solver? Traditional programming tests often focus on syntax knowledge, but assessing problem-solving ability requires different metrics.
From Tutorial Code to Original Solutions
One clear sign of progress is moving from modifying existing code to creating original solutions. If you can start with a blank editor and build a working solution without referencing similar code, you’re developing genuine problem-solving skills.
Handling Ambiguity
Real-world programming problems rarely come with perfect specifications. As your problem-solving abilities improve, you’ll become more comfortable with ambiguity – asking clarifying questions, making reasonable assumptions, and designing flexible solutions that can adapt as requirements evolve.
Efficiency of Solutions
Over time, your initial solutions should become more efficient without extra effort. Where you once naturally wrote O(n²) algorithms, you might start defaulting to O(n log n) or O(n) approaches as your pattern recognition improves.
Debugging Speed
Strong problem solvers debug more efficiently because they can predict where issues might occur and have better mental models of program execution. Tracking how quickly you can identify and fix bugs provides insight into your growing problem-solving abilities.
Advanced Problem-Solving Techniques
As your skills develop, consider these advanced techniques to further enhance your problem-solving abilities.
Test-Driven Development (TDD)
TDD reverses the typical development process by writing tests before implementing solutions. This approach clarifies requirements, prevents scope creep, and ensures your solution works as expected.
The basic TDD workflow follows these steps:
- Write a test that defines a function or feature improvement
- Run the test, which should fail since the feature doesn’t exist yet
- Write the minimal amount of code necessary to make the test pass
- Refactor the code while ensuring the test still passes
- Repeat for the next feature or improvement
This iterative process builds problem-solving muscles by forcing you to think about expected outcomes before diving into implementation.
Constraint-Based Thinking
Sometimes adding constraints can spark creativity rather than limiting it. Try solving problems with artificial constraints like:
- Using no built-in functions or libraries
- Minimizing memory usage or computational complexity
- Limiting solution size (e.g., “solve this in under 20 lines of code”)
- Using only certain programming constructs (e.g., no loops, only recursion)
These self-imposed limitations push you to explore alternative approaches and deepen your understanding of programming fundamentals.
Rubber Duck Debugging
Named for the practice of explaining your code to a rubber duck (or any inanimate object), this technique leverages the power of verbalization to clarify thinking.
When stuck on a problem, explain your code line by line to an imaginary listener. The act of articulating your approach often reveals logical flaws or overlooked details. This works because explanation requires organizing your thoughts coherently, which can highlight gaps in your reasoning.
From Theory to Practice: Building a Problem-Solving Routine
Knowing what to do is one thing; incorporating these practices into your daily routine is another. Here’s a structured approach to systematically improve your problem-solving skills.
Daily Problem-Solving Practice
Commit to solving at least one new programming problem daily. Sites like LeetCode, HackerRank, or Codewars offer problems of varying difficulty levels. Start with easier problems and gradually increase difficulty as you gain confidence.
The key is consistency – regular practice, even with simple problems, builds stronger neural pathways than occasional intensive sessions.
Implement a Learning Journal
Maintain a learning journal to document your problem-solving journey. For each problem you tackle, record:
- The problem statement
- Your initial thoughts and approach
- Any obstacles encountered and how you overcame them
- The final solution and its time/space complexity
- Alternative approaches you discovered after solving
- Key insights or patterns recognized
Reviewing this journal periodically reinforces learning and helps identify recurring patterns in your problem-solving approach.
Build Projects, Not Just Exercises
While discrete exercises develop specific skills, building complete projects integrates those skills in realistic contexts. Projects force you to:
- Make design decisions with real-world trade-offs
- Connect different components into a cohesive system
- Handle unexpected challenges that arise during development
Start with small projects that interest you – a personal website, a command-line tool, or a simple game – and gradually tackle more complex applications as your confidence grows.
Seek and Provide Code Reviews
Having your code reviewed by others provides invaluable feedback on your problem-solving approach. Similarly, reviewing others’ code exposes you to different solutions and thinking styles.
Participate in open-source projects, coding communities, or peer learning groups where code review is practiced. These interactions accelerate learning by highlighting blind spots in your thinking and introducing you to new techniques.
The Continuous Learning Mindset
Problem-solving skills aren’t developed once and then complete – they require continuous refinement throughout your programming career.
Learning from Every Problem
After solving a problem, don’t immediately move on. Take time to reflect on what you learned and how it might apply to future challenges. Ask yourself:
- What pattern did this problem represent?
- What was the key insight that led to the solution?
- How could I apply this approach to other problems?
- What would make this solution more elegant or efficient?
This reflective practice transforms each problem from a one-time exercise into a building block for future problem-solving.
Studying Others’ Solutions
After solving a problem, examine how others approached it. This doesn’t mean looking up solutions before trying yourself, but rather learning from alternative approaches after you’ve created your own solution.
Pay attention to solutions that are more elegant, efficient, or readable than yours, and analyze what makes them superior. This comparative analysis develops your ability to evaluate and improve code.
Teaching as a Learning Tool
One of the most effective ways to solidify your understanding is to teach others. Whether through blogging, creating tutorials, mentoring beginners, or explaining concepts to peers, teaching forces you to organize your knowledge coherently and identify gaps in your understanding.
As the saying goes, “To teach is to learn twice.” By articulating your problem-solving process to others, you reinforce neural pathways and deepen your own comprehension.
Conclusion: Beyond Syntax to True Problem-Solving
The journey from syntax memorization to effective problem-solving isn’t quick or easy, but it’s the path to becoming a truly capable programmer. By focusing on algorithmic thinking, developing a problem-solving mindset, and practicing deliberate techniques, you can bridge the gap between knowing programming language constructs and applying them to solve real problems.
Remember that struggle is an essential part of the learning process. Each problem you tackle, especially those that initially seem beyond your abilities, strengthens your problem-solving muscles and builds confidence for future challenges.
The most valuable skill in programming isn’t memorizing syntax or algorithms – it’s learning how to approach new problems systematically and creatively. By shifting your focus from “what to type” to “how to think,” you’ll develop abilities that remain relevant regardless of which programming language or framework you’re using.
Start today by choosing one strategy from this guide and applying it to your next programming challenge. With consistent practice and the right mindset, you’ll transform from someone who knows programming syntax to someone who solves real problems through code.
Further Resources
To continue developing your problem-solving skills, explore these resources:
- Books: “Think Like a Programmer” by V. Anton Spraul, “Cracking the Coding Interview” by Gayle Laakmann McDowell
- Online Platforms: LeetCode, HackerRank, CodeSignal, Project Euler
- Communities: Stack Overflow, Reddit’s r/learnprogramming, GitHub discussions
- Courses: Algorithm specializations on platforms like Coursera, edX, or Udemy
Remember that becoming a strong problem solver is a marathon, not a sprint. Celebrate small victories, learn from setbacks, and trust that consistent effort will yield impressive results over time.