Why You’re Learning Features But Not Programming Principles
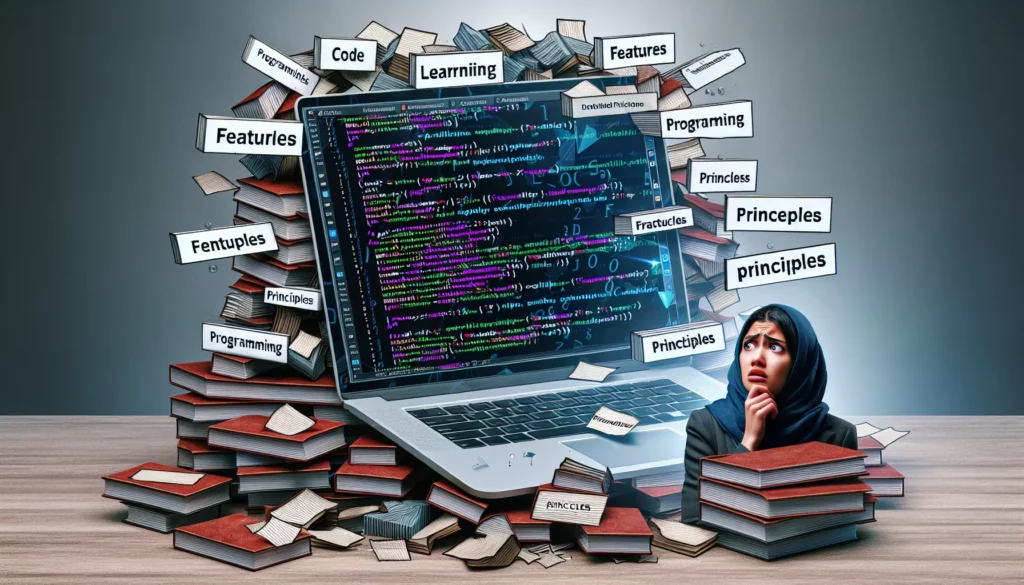
In the ever-evolving landscape of programming education, a concerning trend has emerged: many aspiring developers focus heavily on learning specific features of languages or frameworks while overlooking the fundamental principles that power effective programming. This imbalance can lead to significant gaps in understanding that ultimately hinder your growth as a developer.
At AlgoCademy, we’ve observed thousands of coding journeys, and we’ve noticed that those who excel in technical interviews and thrive in their careers are those who master programming principles rather than just memorizing language features.
The Feature Learning Trap
Let’s start by identifying the problem. When you’re new to programming, it’s easy to fall into the “feature learning trap” – focusing on syntax and specific language capabilities rather than understanding the underlying concepts that make those features useful.
Consider this scenario: You learn that Python has list comprehensions, a powerful feature that allows you to create lists using a compact syntax:
squares = [x**2 for x in range(10)]
You might memorize this syntax and use it frequently, but do you understand:
- The iteration principle behind it?
- How memory allocation works during this operation?
- When this approach is more efficient than alternatives?
- How similar functionality could be implemented in languages without this specific feature?
Without understanding these principles, you’re merely collecting syntax patterns rather than building transferable knowledge.
Why We Focus on Features
There are several reasons why programmers tend to gravitate toward learning features rather than principles:
1. Immediate Gratification
Learning a new feature often provides immediate results. You can quickly see something working on your screen, which feels rewarding. In contrast, understanding principles like memory management or algorithmic complexity might not yield visible results right away.
2. Tutorial Culture
Many programming tutorials and courses are structured around teaching specific features or frameworks. “How to use React hooks,” “10 Python features you should know,” or “New features in Java 17” are common tutorial titles. This approach creates the impression that mastering a collection of features equals mastering programming.
3. Job Requirements
Job listings often emphasize specific technologies and features rather than fundamental knowledge. When a job posting requires “experience with Angular,” it’s tempting to focus exclusively on learning Angular’s features rather than understanding the principles of component-based architecture that would apply to any framework.
4. Overwhelming Complexity
Programming principles can seem abstract and complex. It’s easier to learn how to use a specific API than to understand the design patterns that informed its creation.
The Cost of Feature-Focused Learning
While knowing language features is certainly important, relying solely on this knowledge comes with significant drawbacks:
Limited Adaptability
Technologies change rapidly. The JavaScript framework you master today might be obsolete in five years. However, principles like state management, component architecture, and efficient DOM manipulation will remain relevant regardless of the framework du jour.
Consider developers who learned jQuery extensively in the 2000s but struggled when the industry shifted toward frameworks like React and Vue. Those who understood the principles behind DOM manipulation and event handling adapted more quickly than those who had merely memorized jQuery methods.
Difficulty Solving Novel Problems
Technical interviews at top companies rarely test your knowledge of language features. Instead, they assess your ability to apply programming principles to solve novel problems.
A candidate who understands recursion as a principle can apply it in any language, while someone who has only memorized Python’s syntax for recursive functions might struggle when asked to implement recursion in a different language.
Inefficient Solutions
Without understanding principles like time and space complexity, you might choose inefficient approaches to problems. For example, you might use a nested loop with O(n²) complexity when an O(n) solution exists, simply because you’re familiar with the syntax of loops but not with algorithmic efficiency principles.
Dependency on Documentation
Developers who focus on features often find themselves constantly referring to documentation because they haven’t internalized the principles that would allow them to reason through problems independently.
Core Programming Principles Every Developer Should Master
Instead of just learning features, focus on understanding these fundamental principles that transcend specific languages or frameworks:
1. Abstraction
Abstraction is the process of simplifying complex systems by modeling classes appropriate to the problem, and working at the most appropriate level of inheritance for a given aspect of the problem.
Rather than just learning how to create a class in Java or Python, understand why abstraction matters and how it helps manage complexity. Consider how you might design a system to model a library, focusing on what details are essential and what can be abstracted away.
2. Algorithmic Thinking
Algorithmic thinking involves formulating problems in a way that allows us to use computational methods to solve them efficiently.
Instead of just memorizing sorting algorithms, understand the principles behind them: divide and conquer, greedy approaches, and dynamic programming. This knowledge allows you to derive solutions rather than recall them.
3. Data Structures
Understanding data structures goes beyond knowing how to use lists, dictionaries, or arrays in your language of choice. It involves understanding:
- The memory implications of different structures
- Access patterns and their performance characteristics
- When to use one structure over another based on the problem requirements
For example, don’t just learn how to create a hash map in Java; understand how hash tables work internally, why collisions occur, and how they’re resolved.
4. Complexity Analysis
Time and space complexity analysis allows you to evaluate the efficiency of your code. Understanding Big O notation and being able to analyze your own code’s performance is crucial for writing scalable applications.
Rather than just knowing that nested loops are “slow,” understand precisely why an O(n²) algorithm becomes problematic at scale and how to identify opportunities for optimization.
5. State Management
Managing state is a fundamental challenge in programming. Whether you’re working on a web application, a mobile app, or an embedded system, understanding how to track, update, and maintain state is essential.
Don’t just learn Redux or another state management library; understand the principles of immutability, state transitions, and side effects that underlie all state management approaches.
6. Concurrency and Parallelism
As applications become more complex and hardware includes more cores, understanding how to write code that can execute concurrently or in parallel becomes increasingly important.
Rather than just learning the syntax for creating threads in Java or using async/await in JavaScript, understand the principles of race conditions, deadlocks, and thread safety that apply across all concurrent programming.
7. Error Handling
Robust software must handle errors gracefully. Understanding different approaches to error handling (exceptions, error codes, Option/Maybe types) and when to use each is more valuable than knowing the specific syntax for try/catch blocks in a particular language.
How to Shift from Features to Principles
If you recognize that you’ve been focusing too much on features rather than principles, here are strategies to rebalance your learning:
1. Study Computer Science Fundamentals
Even if you didn’t study computer science formally, resources like:
- MIT’s OpenCourseWare
- Stanford’s online courses
- Books like “Structure and Interpretation of Computer Programs”
- AlgoCademy’s algorithmic thinking courses
can help you build a solid foundation in programming principles.
2. Implement Core Data Structures and Algorithms from Scratch
Don’t just use the built-in list or dictionary in your language. Try implementing a linked list, binary search tree, or hash table from scratch. This exercise forces you to understand the principles behind these structures.
For example, implementing a basic hash table might look like:
class SimpleHashTable:
def __init__(self, size=100):
self.size = size
self.table = [None] * size
def _hash(self, key):
# Simple hash function
return sum(ord(c) for c in str(key)) % self.size
def put(self, key, value):
index = self._hash(key)
if self.table[index] is None:
self.table[index] = []
# Handle collisions with chaining
for i, (k, v) in enumerate(self.table[index]):
if k == key:
self.table[index][i] = (key, value)
return
self.table[index].append((key, value))
def get(self, key):
index = self._hash(key)
if self.table[index] is None:
return None
for k, v in self.table[index]:
if k == key:
return v
return None
This implementation teaches you about hashing functions, collision resolution, and the trade-offs involved in hash table design – principles that apply regardless of which language or library you use in the future.
3. Read Source Code of Libraries You Use
Instead of just using libraries, read their source code to understand how they implement features. This practice reveals the principles and patterns employed by experienced developers.
For example, reading through parts of the React source code can teach you about reconciliation algorithms and efficient UI updates, principles that apply to any UI framework.
4. Solve Algorithm Problems
Platforms like AlgoCademy, LeetCode, and HackerRank offer problems that test your understanding of programming principles rather than language features. Regularly solving these problems helps develop algorithmic thinking.
5. Build Projects in Different Languages
Try implementing the same project in multiple programming languages. This exercise forces you to focus on the principles that remain constant across languages while adapting to different syntax and features.
For example, build a simple REST API in Node.js, then rebuild it in Python with Flask, and again in Go. You’ll quickly identify the common principles (routing, HTTP methods, request/response handling) that transcend the specific features of each language or framework.
6. Teach Others
Teaching is one of the best ways to solidify your understanding of principles. When you explain concepts to others, you’re forced to articulate the “why” behind the “how,” which deepens your own understanding.
7. Read Books That Focus on Principles
Some recommended titles include:
- “Clean Code” by Robert C. Martin
- “Design Patterns: Elements of Reusable Object-Oriented Software” by the Gang of Four
- “Code Complete” by Steve McConnell
- “The Pragmatic Programmer” by Andrew Hunt and David Thomas
These books focus on timeless principles rather than specific language features.
Balancing Features and Principles
While this article emphasizes the importance of principles, we’re not suggesting that learning features is worthless. In fact, a balanced approach that combines both is ideal:
Learn Principles First, Features Second
When approaching a new concept, start by understanding the underlying principle before diving into the specific implementation in your language of choice.
For example, when learning about asynchronous programming:
- First, understand the concepts of concurrency, the event loop, and callbacks.
- Then, learn how these principles are implemented in JavaScript’s Promises or Python’s asyncio.
Use Features as Concrete Examples of Principles
Language features can serve as tangible examples that help you understand abstract principles. For instance, JavaScript’s closures provide a concrete way to understand lexical scoping and function environments.
Recognize When Features Embody New Principles
Sometimes, new language features introduce or emphasize important principles. For example, Rust’s ownership system embodies principles of memory safety and concurrency without data races. In these cases, learning the feature is a gateway to understanding valuable principles.
Case Study: Preparing for Technical Interviews
Let’s examine how a principle-focused approach differs from a feature-focused approach when preparing for technical interviews at major tech companies.
Feature-Focused Preparation:
A developer focusing on features might:
- Memorize the syntax for common operations in their preferred language
- Learn specific library functions that can solve common problems
- Practice using built-in methods to manipulate data structures
During the interview, this developer might struggle when:
- Asked to solve a problem that doesn’t match a pattern they’ve memorized
- The interviewer asks them to use a different language or restricts the use of certain built-in functions
- They need to optimize their solution beyond the obvious approach
Principle-Focused Preparation:
A developer focusing on principles might:
- Study algorithmic paradigms like dynamic programming, greedy algorithms, and divide and conquer
- Understand the time and space complexity implications of different approaches
- Practice implementing data structures from scratch to understand their internals
During the interview, this developer can:
- Adapt to novel problems by applying familiar principles in new contexts
- Switch between languages by focusing on the algorithm rather than syntax
- Identify optimization opportunities based on their understanding of computational complexity
Real Interview Example:
Consider this common interview problem: “Find the first non-repeating character in a string.”
A feature-focused developer might immediately reach for language-specific methods:
def first_non_repeating_char(s):
for char in s:
if s.count(char) == 1:
return char
return None
While this works, it has O(n²) time complexity because `count()` iterates through the string for each character.
A principle-focused developer would recognize this as a frequency counting problem and apply the principle of using a hash map for O(1) lookups:
def first_non_repeating_char(s):
# Count frequencies
char_counts = {}
for char in s:
char_counts[char] = char_counts.get(char, 0) + 1
# Find first non-repeating character
for char in s:
if char_counts[char] == 1:
return char
return None
This solution has O(n) time complexity and demonstrates an understanding of both the problem’s nature and efficient data structure usage – principles that transcend any specific language.
Long-Term Career Impact
The distinction between feature-focused and principle-focused learning becomes even more apparent as you progress in your career:
Junior Developers
At the junior level, both approaches might seem equally effective. Companies often hire junior developers based on their ability to contribute quickly using specific technologies.
Mid-Level Developers
As you move into mid-level roles, the limitations of feature-focused learning become apparent. Developers who understand principles can:
- Debug complex issues by reasoning about the underlying systems
- Learn new technologies more quickly by recognizing familiar principles
- Make better architectural decisions that consider performance, maintainability, and scalability
Senior Developers and Beyond
At senior levels, principle-focused developers truly shine. They can:
- Design systems that remain robust as requirements evolve
- Evaluate new technologies based on their underlying principles rather than hype
- Mentor junior developers by teaching them not just how to code, but how to think about code
- Contribute to technical decisions that affect the entire organization
The most successful tech leads, architects, and CTOs are invariably those who mastered principles rather than just accumulating knowledge of features.
How AlgoCademy Teaches Programming Principles
At AlgoCademy, we’ve designed our curriculum to emphasize principles while still teaching practical skills:
Problem-Based Learning
Rather than starting with language features, we present problems that require you to apply programming principles. By solving these problems, you naturally learn the relevant features in context.
Multiple Implementation Approaches
For each problem, we demonstrate multiple solutions with different algorithmic approaches. This helps you understand the trade-offs involved and the principles that guide choosing one approach over another.
Complexity Analysis
Every solution includes a thorough analysis of its time and space complexity, helping you develop intuition about algorithmic efficiency.
Interactive Visualizations
Our platform includes visualizations that illustrate how algorithms work, making abstract principles concrete and easier to understand.
AI-Powered Guidance
Our AI assistant doesn’t just tell you what’s wrong with your code; it helps you understand the principles you’re missing and guides you toward developing a deeper understanding.
Conclusion: The Path to Principled Programming
The journey from novice to expert programmer isn’t about accumulating knowledge of more and more language features. It’s about developing a deep understanding of programming principles that transcend specific languages, frameworks, or technologies.
By shifting your focus from features to principles, you’ll:
- Build transferable knowledge that remains valuable as technologies evolve
- Develop stronger problem-solving abilities that serve you in any context
- Prepare more effectively for technical interviews at top companies
- Position yourself for long-term career growth beyond just coding
Remember that features come and go, but principles endure. The next time you’re learning a new programming concept, ask yourself: “Am I understanding the underlying principle, or just memorizing the syntax?” Your future self will thank you for choosing the deeper path.
At AlgoCademy, we’re committed to helping you build not just your coding skills, but your computational thinking abilities. Our platform is designed to teach you both the “how” and the “why” of programming, preparing you not just for your next interview, but for a lifetime of growth as a developer.
Start your journey toward principled programming today, and discover the difference that deep understanding can make in your development career.