Why You’re Comfortable With Code But Scared of Math in Programming
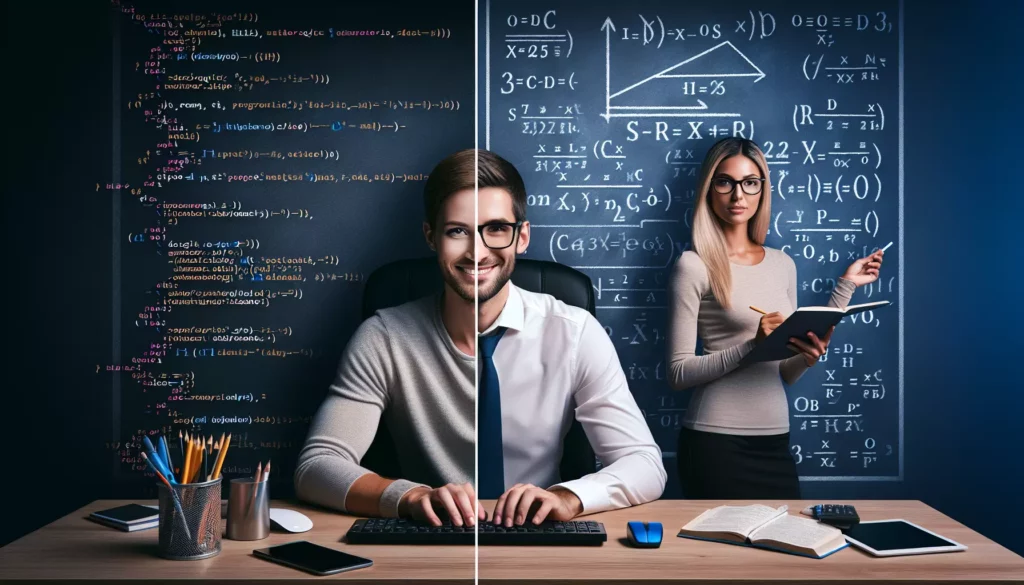
Have you ever found yourself confidently writing lines of code, only to freeze when a mathematical concept appears in a programming problem? You’re not alone. Many programmers experience a strange disconnect: they feel at home with syntax, functions, and data structures, but become anxious when faced with anything that resembles formal mathematics.
This phenomenon is surprisingly common, even among experienced developers. In this article, we’ll explore why this disconnect exists, why it matters, and most importantly, how to overcome it to become a more well-rounded programmer.
The Common Disconnect Between Coding and Math
Let’s start with a scenario many programmers know all too well:
You’re working through a coding challenge. Everything’s going great as you design classes, implement functions, and handle data. Then suddenly, the problem requires calculating the probability of an event or optimizing an algorithm using calculus concepts. Your confidence evaporates, and that familiar feeling of math anxiety creeps in.
This experience highlights a curious paradox in programming education. Programming itself is built on mathematical foundations, yet many programmers feel comfortable with code while experiencing anxiety about the mathematical concepts that underlie it.
The Symptoms of Math Anxiety in Programming
How do you know if you’re experiencing this disconnect? Here are some common signs:
- You confidently tackle syntax and programming concepts but avoid algorithm challenges
- You feel a sense of dread when mathematical notation appears in documentation
- You implement libraries for complex calculations without understanding their mathematical foundations
- You prefer to search for existing solutions rather than derive mathematical approaches yourself
- You feel intimidated by fields like machine learning or cryptography because of their mathematical requirements
If any of these resonate with you, you’re experiencing what many in the field do: comfort with code but apprehension about math.
Why This Disconnect Exists
To understand this phenomenon, we need to look at several factors that contribute to the code-math disconnect.
Different Learning Pathways
Modern programming education often takes a practical, project-based approach. Many developers learn through:
- Building projects
- Following tutorials
- Solving practical problems
- Learning frameworks and libraries
This hands-on approach makes coding accessible and immediately rewarding. You write code, see results, and get instant feedback. This creates a positive learning loop that builds confidence.
In contrast, mathematics is traditionally taught in a more abstract, theoretical manner:
- Learning theorems and proofs
- Working with abstract concepts
- Focusing on formal notation
- Building from fundamental principles
Without clear, immediate applications, mathematical concepts can feel disconnected from practical programming tasks. This educational divide creates a comfort gap between coding and mathematical thinking.
The Abstraction Level Difference
Modern programming languages offer high levels of abstraction. Consider this Python code that sorts a list:
numbers = [4, 2, 7, 1, 9]
sorted_numbers = sorted(numbers)
print(sorted_numbers) # Outputs: [1, 2, 4, 7, 9]
You don’t need to understand the mathematical principles of sorting algorithms to use this feature. The language abstracts away the complexity, making programming accessible.
Mathematics, however, often requires engaging with abstractions directly. When you encounter Big O notation or algorithm efficiency analysis, you’re suddenly asked to think about mathematical patterns rather than just using pre-built functions.
Emotional and Psychological Factors
Past negative experiences with math education can create lasting emotional barriers. Many people carry “math trauma” from school experiences where:
- They were made to feel inadequate for not understanding quickly
- Math was presented as a talent rather than a learnable skill
- Speed was valued over understanding
- Mistakes were treated as failures rather than learning opportunities
These experiences create a psychological barrier that doesn’t typically exist with coding, which many people discover later in life through more positive, self-directed learning experiences.
The Language Barrier
Programming languages are designed to be readable and approachable:
if user_age >= 18:
print("You can vote!")
Even without programming experience, you can likely guess what this code does.
In contrast, mathematical notation can seem like a foreign language to the uninitiated:
∑(i=1 to n) i = n(n+1)/2
This notation efficiently communicates to those fluent in mathematical language but can be intimidating to others. The unfamiliarity creates discomfort that doesn’t exist with the more English-like syntax of programming languages.
Why Bridging This Gap Matters
Before discussing how to overcome this disconnect, let’s understand why it’s worth addressing.
Mathematics Unlocks Deeper Understanding
While you can code effectively without deep mathematical knowledge in many areas, understanding the mathematical foundations provides several advantages:
- Problem-solving versatility: Mathematical thinking helps you approach novel problems without relying on existing solutions
- Efficiency improvements: Understanding algorithm complexity and optimization often requires mathematical analysis
- First-principles reasoning: Math helps you reason from first principles rather than pattern matching to known solutions
Career Advancement Opportunities
Many advanced and high-growth fields in programming have significant mathematical components:
- Machine learning and AI
- Computer graphics and game development
- Cryptography and security
- Data science and analytics
- Algorithmic trading
Comfort with mathematical concepts opens doors to these specialized and often lucrative career paths.
Breaking Through Plateaus
Many programmers hit a ceiling in their growth when they avoid the mathematical aspects of programming. Embracing math can help break through these plateaus and continue advancing in skill and understanding.
Reframing Math for Programmers
The first step in overcoming math anxiety is changing how you think about mathematics in relation to programming.
Math Is a Tool, Not a Test
In school, math is often presented as a series of tests to pass. In programming, math should be viewed as a powerful tool in your toolkit—something that helps you solve problems more effectively.
When you encounter a mathematical concept in programming, ask yourself: “How can this help me solve real problems?” rather than “Will I be judged on my understanding of this?”
Programming Already Uses Mathematical Thinking
You may be using mathematical thinking more than you realize. Consider these programming concepts that are inherently mathematical:
- Boolean logic: When you write conditional statements, you’re using logical operators from mathematical logic
- Functions: Programming functions are directly related to mathematical functions
- Iteration: Loops in programming relate to concepts like sequences and recurrence relations in math
- Data structures: Many data structures (graphs, trees, sets) come directly from discrete mathematics
Recognizing that you’re already using mathematical concepts can help reduce the perceived gap between coding and math.
Concrete Before Abstract
Mathematics education often starts with abstract concepts, but programmers typically learn better by starting with concrete examples. When approaching a mathematical concept, try to:
- Implement it in code first
- Experiment with examples
- Gradually build intuition
- Then explore the formal theory
This approach leverages your existing comfort with code to build a bridge to mathematical understanding.
Practical Strategies for Overcoming Math Anxiety in Programming
Now let’s explore practical approaches to becoming more comfortable with mathematics in programming.
Start with Discrete Mathematics
Discrete mathematics is directly applicable to programming and often more approachable than calculus or linear algebra for programmers. Key topics include:
- Logic and Boolean algebra
- Set theory
- Graph theory
- Combinatorics
- Recursion and induction
These topics have clear applications in programming and can serve as a gateway to mathematical thinking.
Implement Mathematical Concepts in Code
One of the best ways to understand mathematical concepts is to implement them in code. For example, instead of just reading about the Fibonacci sequence, code it:
def fibonacci(n):
if n <= 1:
return n
else:
return fibonacci(n-1) + fibonacci(n-2)
# More efficient iterative implementation
def fibonacci_iterative(n):
a, b = 0, 1
for i in range(n):
a, b = b, a + b
return a
By implementing and comparing both recursive and iterative approaches, you gain insight into both the mathematical definition and practical considerations like efficiency.
Visualize Concepts
Many mathematical concepts become clearer when visualized. Use your programming skills to create visualizations of mathematical concepts. For instance, you could visualize sorting algorithms:
import matplotlib.pyplot as plt
import numpy as np
import random
def visualize_bubble_sort(arr):
n = len(arr)
iterations = []
# Make a copy to avoid modifying the original
array = arr.copy()
iterations.append(array.copy())
# Bubble sort with visualization
for i in range(n):
for j in range(0, n-i-1):
if array[j] > array[j+1]:
array[j], array[j+1] = array[j+1], array[j]
iterations.append(array.copy())
# Create visualization
fig, axes = plt.subplots(len(iterations), 1, figsize=(8, len(iterations)))
for i, data in enumerate(iterations):
axes[i].bar(range(len(data)), data)
axes[i].set_title(f"Iteration {i}")
plt.tight_layout()
plt.show()
# Example usage
data = list(range(10))
random.shuffle(data)
visualize_bubble_sort(data)
By visualizing algorithms and mathematical concepts, you can build intuition that makes the formal mathematics less intimidating.
Learn Math Through Programming Challenges
Platforms like Project Euler, LeetCode, and HackerRank offer programming challenges that often incorporate mathematical concepts. These platforms allow you to:
- Approach math through coding
- Build mathematical intuition through practice
- See immediate applications of mathematical concepts
Start with easier problems and gradually work your way up to more mathematically complex challenges.
Study Algorithms and Their Analysis
Algorithm analysis offers a practical entry point to mathematical thinking in programming. When you analyze an algorithm’s time complexity, you’re using mathematical reasoning to understand its performance characteristics.
For example, understanding why quicksort has an average time complexity of O(n log n) requires grasping concepts from both mathematics and computer science.
def quicksort(arr):
if len(arr) <= 1:
return arr
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quicksort(left) + middle + quicksort(right)
By implementing algorithms and analyzing their performance, you can build a bridge between coding and mathematical thinking.
Explore Applied Mathematics in Your Projects
Incorporate mathematical components into your personal projects to build familiarity in a low-pressure environment. Some ideas include:
- Adding data visualization with statistical analysis to a web application
- Building a simple game that uses physics calculations
- Creating a financial calculator that computes compound interest
- Implementing a recommendation system using basic similarity metrics
These projects allow you to apply mathematical concepts in contexts where you’re already comfortable.
Case Studies: Mathematical Concepts in Everyday Programming
To further illustrate how mathematics appears in practical programming, let’s look at some common scenarios.
Case Study 1: Web Development and Geometry
Even in web development, which many consider less mathematically intensive, geometric concepts appear regularly. Consider responsive layouts and animations:
// Calculating the position of an element in a circular layout
function positionInCircle(index, total, radius) {
const angle = (index / total) * Math.PI * 2; // Convert to radians
const x = radius * Math.cos(angle);
const y = radius * Math.sin(angle);
return { x, y };
}
// Using the function to position elements
const elements = document.querySelectorAll('.circle-item');
const totalElements = elements.length;
const radius = 200; // pixels
elements.forEach((el, index) => {
const position = positionInCircle(index, totalElements, radius);
el.style.transform = `translate(${position.x}px, ${position.y}px)`;
});
This example uses trigonometry to position elements in a circle—a practical application of mathematics in frontend development.
Case Study 2: Database Queries and Set Theory
When working with databases, you’re using concepts from set theory, even if you don’t realize it. SQL operations like UNION, INTERSECT, and JOIN are direct implementations of set operations:
-- Set union: Customers from either the US or Canada
SELECT * FROM customers WHERE country = 'USA'
UNION
SELECT * FROM customers WHERE country = 'Canada';
-- Set intersection: Products that are both discounted and in stock
SELECT product_id FROM discounted_products
INTERSECT
SELECT product_id FROM in_stock_products;
Understanding the mathematical foundations of these operations helps write more efficient queries and better understand query execution plans.
Case Study 3: Machine Learning and Linear Algebra
Machine learning applications heavily rely on linear algebra. Consider a simple linear regression implementation:
import numpy as np
# Sample data
X = np.array([[1, 1], [1, 2], [2, 2], [2, 3]]) # Features
y = np.array([6, 8, 9, 11]) # Target values
# Add bias term
X_b = np.c_[np.ones((X.shape[0], 1)), X]
# Normal equation: θ = (X^T X)^(-1) X^T y
theta = np.linalg.inv(X_b.T.dot(X_b)).dot(X_b.T).dot(y)
print("Model parameters:", theta)
# Predict for new data
new_X = np.array([[3, 5]])
new_X_b = np.c_[np.ones((new_X.shape[0], 1)), new_X]
prediction = new_X_b.dot(theta)
print("Prediction:", prediction)
This example uses linear algebra concepts like matrix multiplication and inversion to find the optimal parameters for a linear regression model.
Resources for Building Mathematical Confidence
If you’re ready to strengthen the mathematical side of your programming knowledge, here are some resources specifically designed for programmers:
Books
- “Concrete Mathematics” by Graham, Knuth, and Patashnik – Written by programming legend Donald Knuth, this book bridges the gap between programming and mathematics
- “Mathematics for Computer Science” by Lehman, Leighton, and Meyer – Focuses on the math most relevant to computer science
- “Grokking Algorithms” by Aditya Bhargava – Presents algorithms and their mathematical foundations with visual explanations
- “Practical Statistics for Data Scientists” by Bruce, Bruce, and Gedeck – Connects statistical concepts to programming applications
Online Courses
- Discrete Mathematics for Computer Science (Coursera) – Covers the essential math foundations for CS
- Mathematics for Machine Learning (Coursera) – Explains linear algebra and statistics in the context of ML applications
- Algorithms, Part I & II (Princeton University on Coursera) – Teaches algorithms with their mathematical analysis
- Introduction to Computational Thinking and Data Science (MIT on edX) – Connects mathematical concepts to practical programming
Interactive Platforms
- Project Euler – Programming challenges that often require mathematical insight
- Brilliant.org – Interactive courses on math and computer science with visual explanations
- Desmos – Online graphing calculator for visualizing mathematical concepts
- AlgoCademy – Offers tutorials that connect algorithmic thinking with mathematical concepts
Communities
- Math for Programmers subreddit – Discussion forum focused on mathematical topics relevant to programming
- Computer Science Stack Exchange – Q&A site where theoretical CS questions (often mathematical) are discussed
- Math & Programming Discord servers – Real-time discussion groups where you can ask questions
Changing Your Mindset: Growth vs. Fixed Thinking
Perhaps the most important factor in overcoming math anxiety is adopting a growth mindset about mathematical ability.
Recognize That Mathematical Ability Is Not Fixed
Research in cognitive psychology has consistently shown that mathematical ability is not innate but developed through practice and learning. Just as you weren’t born knowing how to program, you weren’t born with or without a “math gene.”
Embrace the Learning Process
When learning mathematical concepts:
- Value the process of understanding over getting the right answer
- Recognize that confusion is a natural part of learning, not a sign of inability
- Celebrate small victories and improvements
- View challenges as opportunities for growth rather than tests of ability
Learn From Multiple Perspectives
If one explanation doesn’t click, seek different explanations. Mathematical concepts can be approached from multiple angles:
- Visual/geometric
- Algebraic/symbolic
- Computational/algorithmic
- Applied/contextual
Finding the perspective that resonates with your thinking style can make a concept suddenly clear.
Real Programmer Stories: Overcoming Math Anxiety
To inspire your journey, here are stories from real programmers who bridged the gap between coding comfort and mathematical confidence:
From Web Developer to Machine Learning Engineer
“I spent five years as a frontend developer, avoiding anything that seemed too mathematical. When I became interested in machine learning, I forced myself to tackle linear algebra and statistics. I started by implementing simple algorithms from scratch, visualizing the results, and gradually building intuition. The breakthrough came when I realized I could use my programming skills as a bridge to understanding the math. Now I work as a machine learning engineer and actually enjoy the mathematical aspects of my job.”
— Maria, Machine Learning Engineer
Finding Math Through Game Development
“Math was my worst subject in school. But when I started programming games, I needed to understand vectors, physics, and trigonometry to create the experiences I wanted. I found that implementing these concepts in code made them concrete and understandable in a way that textbooks never achieved. Now I see mathematics as a creative tool rather than an academic hurdle.”
— Jackson, Game Developer
Algorithmic Trading and Financial Mathematics
“I always considered myself ‘not a math person’ despite being a competent programmer. When I joined a fintech startup, I had to face concepts like stochastic calculus and time-series analysis. Instead of trying to learn the math in abstract, I paired up with a mathematically-minded colleague. They explained the concepts, and I implemented them in code. This collaborative approach helped me build a bridge between programming and mathematics that I couldn’t have constructed alone.”
— Raj, Quantitative Developer
Conclusion: The Programmer’s Path to Mathematical Confidence
The disconnect between comfort with code and anxiety about math is a common experience among programmers, but it’s not an insurmountable divide. By understanding the origins of this gap, reframing your relationship with mathematics, and taking practical steps to build mathematical confidence, you can develop a more balanced skill set that enhances your capabilities as a programmer.
Remember these key takeaways:
- The coding-math disconnect stems from differences in learning approaches, abstraction levels, and psychological factors
- Bridging this gap opens doors to advanced fields and deeper understanding of programming concepts
- Start with concrete implementations before tackling abstract theory
- Use your programming skills as a bridge to mathematical understanding
- Adopt a growth mindset about mathematical ability
- Leverage visual and interactive tools to build intuition
Your journey from math anxiety to mathematical confidence in programming doesn’t have to be completed overnight. Each small step builds confidence and capability that will serve you throughout your programming career.
Whether you aspire to work in mathematically intensive fields like machine learning or simply want to deepen your understanding of the code you write, embracing the mathematical aspects of programming will make you a more versatile, confident, and effective developer.
What mathematical concept will you implement in code this week?