Why Your Version Control Strategy Is Creating Merge Conflicts
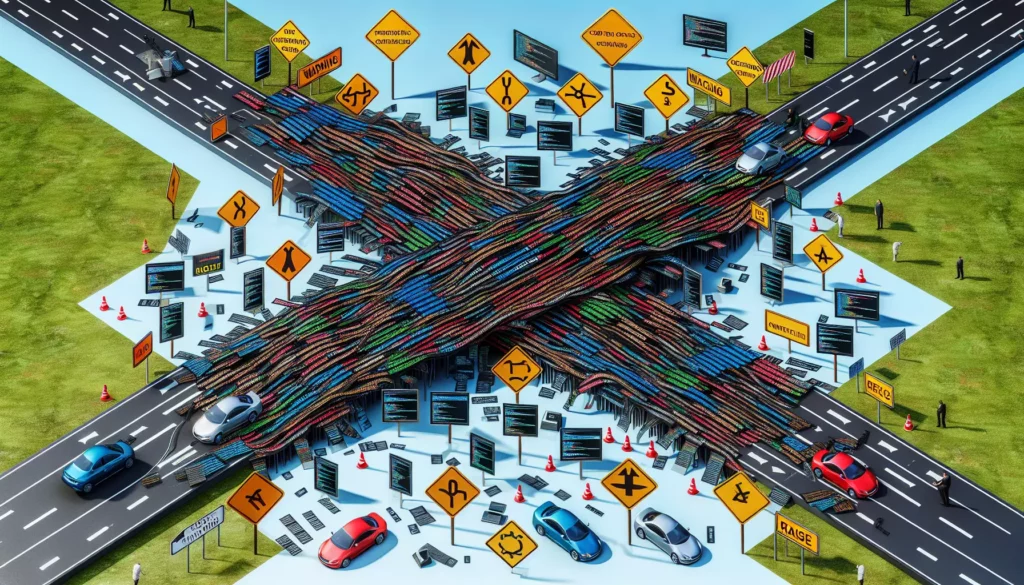
Version control is the backbone of modern software development, allowing teams to collaborate effectively on code. However, many developers find themselves spending more time resolving merge conflicts than actually writing code. If you’re constantly battling merge conflicts, it might not be just bad luck—your version control strategy could be the culprit.
In this comprehensive guide, we’ll explore why merge conflicts happen, how your team’s workflow might be contributing to the problem, and practical strategies to minimize these frustrating roadblocks.
Understanding Merge Conflicts: The Root Cause
Before we dive into solutions, let’s understand what merge conflicts actually are and why they occur. A merge conflict happens when Git can’t automatically resolve differences between two commits. This typically occurs when two developers modify the same line of code, or when one developer edits a file that another developer has deleted.
Git is remarkably good at merging changes automatically, but it has its limits. When faced with conflicting changes, Git throws up its hands and asks you to decide which changes should stay.
Anatomy of a Merge Conflict
When you encounter a merge conflict, Git modifies the affected files to show both versions of the conflicting code:
<<<<<<< HEAD
console.log("This is the current branch version");
=======
console.log("This is the incoming branch version");
>>>>>>> feature-branch
The content between <<<<<<< HEAD
and =======
shows the code in your current branch. The content between =======
and >>>>>>> feature-branch
shows the code in the branch you’re trying to merge.
Resolving this conflict manually requires deciding which version to keep or how to combine them, removing the conflict markers, and then completing the merge.
Common Version Control Strategies That Lead to Conflicts
If your team is experiencing frequent merge conflicts, your version control strategy might be creating unnecessary friction. Here are the most common problematic practices:
1. Long-Lived Feature Branches
One of the most common causes of merge conflicts is working on feature branches that live too long without being merged back into the main branch. The longer a branch exists separately from the main codebase, the more likely it is to conflict when finally merged.
Why it’s problematic: While your feature branch exists, the main branch continues to evolve. Other developers commit changes that might affect the same files you’re working on. By the time you’re ready to merge, the main branch might look very different from when you created your branch.
2. Large, Monolithic Commits
Another common issue is making large, infrequent commits that touch many files at once. This approach increases the likelihood of overlapping with someone else’s work.
Why it’s problematic: When you modify many files in a single commit, you create a larger surface area for potential conflicts. If another developer has modified any of those files, you’ll face conflicts during the merge.
3. Poor Branch Organization
Without a clear branching strategy, teams often create branches haphazardly, leading to a complex web of dependencies that becomes difficult to merge.
Why it’s problematic: If branches are created from outdated versions of the codebase or if multiple branches modify the same components without coordination, merge conflicts become inevitable.
4. Lack of Communication About Code Ownership
When team members aren’t aware of who’s working on what, they might unknowingly make changes to the same parts of the codebase.
Why it’s problematic: Without clear communication, two or more developers might independently decide to refactor the same component or fix the same bug, leading to conflicts when their changes are merged.
5. Insufficient Code Modularity
If your codebase lacks proper modularity, changes tend to ripple across multiple files, increasing the chance of conflicts.
Why it’s problematic: Highly coupled code means that even small feature additions might require changes to shared components, creating hotspots where conflicts frequently occur.
Strategies to Minimize Merge Conflicts
Now that we understand the common causes of merge conflicts, let’s explore strategies to minimize them. Implementing these approaches can dramatically reduce the frequency and severity of conflicts in your team’s workflow.
1. Adopt a Trunk-Based Development Approach
Trunk-based development is a version control strategy where developers make small, frequent commits directly to the main branch (the “trunk”) or to short-lived feature branches that are quickly merged back.
How it helps: By keeping branches short-lived and merging frequently, you reduce the divergence between branches, minimizing the potential for conflicts. When conflicts do occur, they’re smaller and easier to resolve.
Implementation steps:
- Commit to the main branch directly for small changes
- Use feature flags to hide incomplete features in production
- Create short-lived feature branches for larger changes
- Merge or rebase with the main branch at least daily
Many successful development teams, including those at Google and Facebook, have adopted variations of trunk-based development to reduce merge conflicts and speed up their development cycles.
2. Implement Continuous Integration
Continuous Integration (CI) is the practice of automatically building and testing code changes as soon as they’re committed. This approach encourages developers to integrate their changes frequently.
How it helps: CI enforces frequent integration, which naturally reduces the size of changes and the potential for conflicts. It also provides early feedback on integration issues.
Implementation steps:
- Set up automated builds triggered by commits
- Include automated tests in your CI pipeline
- Configure notifications for build failures
- Make fixing broken builds a top priority
Tools like Jenkins, GitHub Actions, CircleCI, and Travis CI can help you implement a robust CI pipeline.
3. Use Feature Flags for Large Features
Feature flags (also known as feature toggles) allow you to merge incomplete features into the main branch while keeping them disabled in production.
How it helps: With feature flags, you can merge code more frequently, even when features aren’t complete. This reduces branch lifetime and the potential for conflicts.
Implementation example:
// A simple feature flag implementation
if (featureFlags.isEnabled('new-user-dashboard')) {
// New dashboard code
} else {
// Old dashboard code
}
Tools like LaunchDarkly, Split.io, and Flagsmith provide more sophisticated feature flag management for larger teams.
4. Establish a Clear Branching Strategy
A well-defined branching strategy ensures that everyone on the team follows the same patterns when creating and merging branches.
How it helps: With clear guidelines, teams can avoid creating branches that are likely to conflict. A good strategy also defines when and how to merge, reducing the chaos that can lead to conflicts.
Popular branching strategies:
- GitFlow: A strict branching model with dedicated branches for features, releases, and hotfixes
- GitHub Flow: A simpler approach focused on short-lived feature branches off the main branch
- GitLab Flow: A middle ground that adds environment branches to the GitHub Flow model
Choose a strategy that matches your team’s size, release cadence, and the nature of your project.
5. Practice Regular Rebasing or Merging
Regularly updating your feature branches with changes from the main branch helps prevent large conflicts from building up.
How it helps: By frequently incorporating the latest changes from the main branch, you address small conflicts as they arise rather than facing a large conflict when you’re ready to merge.
Git commands for staying up to date:
# Using merge to update your feature branch
git checkout feature-branch
git merge main
# Using rebase to update your feature branch
git checkout feature-branch
git rebase main
The choice between merge and rebase depends on your team’s preferences and workflow. Rebase creates a cleaner history but requires more care when working with shared branches.
6. Improve Code Modularity
Well-modularized code with clear separation of concerns reduces the likelihood of developers needing to modify the same files.
How it helps: When code is properly modularized, features can often be developed in isolation without touching shared components, reducing the chance of conflicts.
Modularity principles:
- Single Responsibility Principle: Each module should have only one reason to change
- Encapsulation: Hide implementation details behind well-defined interfaces
- Dependency Injection: Reduce hard-coded dependencies between components
- Clear API boundaries: Define explicit interfaces between modules
Refactoring towards better modularity is a long-term investment that pays dividends in reduced merge conflicts.
Tools and Techniques for Better Conflict Resolution
Even with the best strategies, some conflicts are inevitable. When they occur, having the right tools and techniques can make resolution faster and less painful.
1. Use Visual Merge Tools
Visual diff and merge tools provide a graphical interface for resolving conflicts, making it easier to understand and combine changes.
Popular tools:
- VS Code: Built-in merge conflict resolution
- GitKraken: Visual Git client with merge tools
- Kdiff3: Three-way merge tool
- Meld: Visual diff and merge tool
Configure your preferred tool in Git:
git config --global merge.tool kdiff3
git config --global mergetool.kdiff3.path /path/to/kdiff3
2. Understand Git’s Merge Strategies
Git offers several merge strategies that can be more appropriate for certain situations.
Common merge strategies:
- recursive (default): Standard merge strategy for most situations
- ours: Keep our version in cases of conflict
- theirs: Keep their version in cases of conflict
- patience: More careful approach that can produce cleaner merges for certain types of changes
Specify a strategy during merge:
git merge --strategy=patience feature-branch
3. Learn Advanced Git Commands
Mastering Git’s more advanced features can help you handle complex merge situations.
Useful commands:
- git rerere: Reuse recorded conflict resolutions
- git cherry-pick: Apply specific commits from one branch to another
- git rebase –interactive: Rewrite history to create cleaner merges
Enable rerere to remember how you’ve resolved conflicts:
git config --global rerere.enabled true
4. Establish Conflict Resolution Protocols
Having a team protocol for handling conflicts ensures consistent resolution and reduces confusion.
Elements of a good protocol:
- Who is responsible for resolving conflicts (e.g., the developer merging their branch)
- When to involve the original authors of conflicting code
- How to document resolution decisions
- Post-resolution testing requirements
Real-World Examples: Before and After
Let’s look at how teams have transformed their version control strategies to reduce merge conflicts.
Case Study 1: The Long-Lived Branch Nightmare
Before: A web development team was working on a major redesign in a feature branch that lived for three months. When it came time to merge, they faced hundreds of conflicts because the main branch had evolved significantly.
After: The team adopted a component-based approach, breaking the redesign into smaller, independent features. They used feature flags to hide incomplete components while merging frequently to the main branch. Merge conflicts dropped by 80%.
Case Study 2: Microservice Migration
Before: A team migrating from a monolithic architecture to microservices was experiencing constant conflicts as multiple developers modified the same shared libraries and interfaces.
After: They established clear ownership boundaries for each microservice and created well-defined APIs between services. They also implemented contract testing to ensure compatibility without tight coupling. Conflicts became rare and were mostly limited to shared infrastructure code.
Case Study 3: CI Transformation
Before: A team with weekly integration was suffering from “merge day” dread, with developers often spending an entire day resolving conflicts.
After: They implemented continuous integration with automated testing and a rule that no branch could go more than one day without being updated from the main branch. Merge conflicts became smaller and more manageable, and “merge day” disappeared from their vocabulary.
Common Objections and How to Address Them
Changing version control strategies often meets resistance. Here’s how to address common objections:
“Trunk-based development isn’t feasible for our complex project”
Response: Even complex projects can adopt trunk-based principles by using feature flags and improving modularity. Start with smaller, less critical components to build confidence in the approach.
“We need long-lived branches for our release cycle”
Response: Consider using release branches that are created just before release, rather than developing on long-lived feature branches. This allows most development to happen on short-lived branches while still supporting your release process.
“Frequent commits will introduce bugs into the main branch”
Response: Combine frequent commits with robust automated testing and feature flags. This gives you the benefits of early integration while protecting the stability of your production environment.
“Our team is distributed across time zones, making frequent integration difficult”
Response: Asynchronous communication tools and clear documentation become even more important in distributed teams. Automated CI/CD pipelines can help bridge the time zone gap by providing immediate feedback on integration issues.
Measuring Success: KPIs for Merge Conflict Reduction
To track the effectiveness of your improved version control strategy, monitor these key performance indicators:
1. Frequency of Merge Conflicts
Track how often merge conflicts occur. A successful strategy should show a downward trend in conflict frequency.
2. Time Spent Resolving Conflicts
Measure the time developers spend resolving conflicts. This can be tracked through time-tracking tools or estimated based on team feedback.
3. Size of Conflicts
Count the number of files and lines affected by typical conflicts. Smaller conflicts are easier to resolve and indicate better integration practices.
4. Branch Lifetime
Monitor how long branches exist before being merged. Shorter lifetimes generally correlate with fewer conflicts.
5. Lead Time for Changes
Measure how long it takes for a code change to go from commit to deployment. Reducing merge conflicts should help decrease this time.
Implementation Plan: Transitioning to a Better Strategy
Changing your version control strategy requires careful planning. Here’s a phased approach to transition smoothly:
Phase 1: Assessment and Planning (1-2 Weeks)
- Analyze current merge conflict patterns
- Document your existing workflow
- Define KPIs for measuring improvement
- Choose an appropriate branching strategy
- Create a rollout plan
Phase 2: Infrastructure and Training (2-4 Weeks)
- Set up CI/CD pipelines
- Implement feature flag infrastructure
- Train the team on new workflows and tools
- Document new processes and best practices
Phase 3: Pilot Implementation (2-4 Weeks)
- Start with a single team or component
- Apply new workflow to new features first
- Gather feedback and refine the process
- Measure initial results against KPIs
Phase 4: Full Rollout (1-3 Months)
- Extend new practices to all teams
- Refactor problematic areas of the codebase
- Continue monitoring and improving
- Celebrate early wins to build momentum
Conclusion: A Conflict-Free Future
Merge conflicts don’t have to be a daily frustration for your development team. By understanding the root causes and implementing a strategic approach to version control, you can dramatically reduce both the frequency and severity of conflicts.
Remember these key principles:
- Integrate frequently to minimize divergence between branches
- Keep branches short-lived and focused on specific changes
- Use feature flags to separate code integration from feature release
- Improve code modularity to reduce overlap between developers’ work
- Establish clear processes for branch creation and merging
- Invest in tools and training for efficient conflict resolution
The time you invest in optimizing your version control strategy will pay dividends in developer productivity, code quality, and team morale. Instead of dreading merge conflicts, your team can focus on what matters most: building great software.
What version control strategies have worked best for your team? Have you successfully reduced merge conflicts with a particular approach? Share your experiences in the comments below!
Additional Resources
Books
- “Git for Teams” by Emma Jane Hogbin Westby
- “Continuous Delivery” by Jez Humble and David Farley
- “Feature Toggles” by Pete Hodgson
Online Resources
- Git documentation on merge strategies
- Atlassian’s Git tutorials and guides
- Martin Fowler’s article on Feature Toggles
- Trunk Based Development website (trunkbaseddevelopment.com)
Tools
- Git GUI clients with merge tools (GitKraken, SourceTree)
- Feature flag services (LaunchDarkly, Split.io)
- CI/CD platforms (Jenkins, GitHub Actions, CircleCI)
- Merge analysis tools (Git Tower, GitPrime)
By implementing the strategies outlined in this guide, you’ll be well on your way to a smoother, more productive development workflow with fewer merge conflicts and happier developers.