Why Your Unit Tests Aren’t Catching Real Bugs
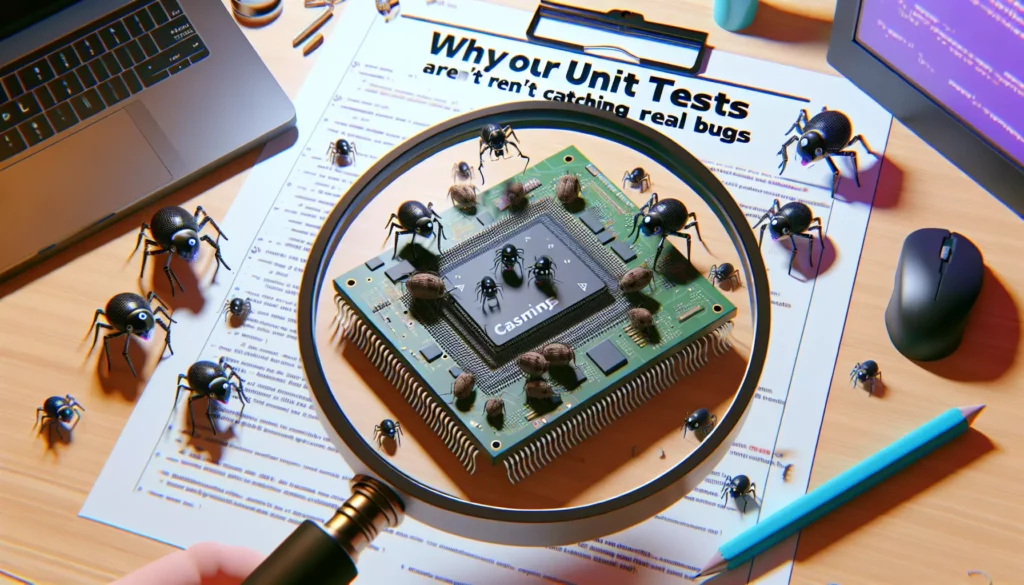
Testing is a critical component of software development, with unit testing often serving as the first line of defense against bugs. Yet many development teams find themselves puzzled when production issues emerge despite having robust test coverage. If you’ve ever wondered, “How did this bug make it to production when we have 90% test coverage?” you’re not alone.
This disconnect between test coverage metrics and actual bug prevention effectiveness points to fundamental issues in how we approach testing. In this article, we’ll explore why your unit tests might be falling short and provide actionable strategies to make your testing more effective at catching real bugs.
The False Security of Test Coverage
Test coverage is often treated as the gold standard for measuring testing effectiveness. A high percentage suggests comprehensive testing and, by extension, high-quality code. However, this metric can be deeply misleading.
Coverage Metrics Don’t Equal Quality
Consider this: you could achieve 100% line coverage with tests that don’t actually verify the correct behavior of your code. For example, a test might execute every line of a function without checking if the function produces the expected output under different conditions.
Here’s a simple example:
// Function to calculate discount
function calculateDiscount(price, discountPercentage) {
if (discountPercentage > 50) {
discountPercentage = 50; // Cap at 50%
}
return price * (discountPercentage / 100);
}
// Test
test('calculateDiscount applies discount correctly', () => {
const result = calculateDiscount(100, 20);
expect(result).toBe(20);
});
This test achieves line coverage for the function but fails to test the branch where the discount percentage is capped at 50%. A real bug in that logic would go undetected.
The Myth of Coverage Completeness
Even with 100% branch coverage, your tests might miss important scenarios. Coverage tools track whether code paths are executed, not whether they’re tested meaningfully against various inputs and conditions.
For instance, consider a function that processes user input:
function validateUsername(username) {
if (!username || typeof username !== 'string') {
return false;
}
if (username.length < 3 || username.length > 20) {
return false;
}
if (!/^[a-zA-Z0-9_]+$/.test(username)) {
return false;
}
return true;
}
A test that calls this function with a valid username and an empty string would achieve branch coverage but miss bugs related to handling usernames with special characters, Unicode characters, or those at the boundary conditions (exactly 3 or 20 characters).
Common Reasons Unit Tests Miss Real Bugs
Let’s dive deeper into why unit tests often fail to catch the bugs that matter most.
Testing Implementation, Not Behavior
A common mistake is writing tests that are tightly coupled to the implementation details rather than focusing on the expected behavior of the code. When tests mirror the implementation logic, they’re likely to contain the same flawed assumptions as the code they’re testing.
For example:
// Implementation
function sortUsersByActivity(users) {
return users.sort((a, b) => b.lastActiveTimestamp - a.lastActiveTimestamp);
}
// Test tightly coupled to implementation
test('sortUsersByActivity sorts users correctly', () => {
const users = [
{ id: 1, lastActiveTimestamp: 100 },
{ id: 2, lastActiveTimestamp: 200 }
];
const sorted = sortUsersByActivity(users);
// Test assumes the same sorting logic as implementation
expect(sorted[0].id).toBe(2);
expect(sorted[1].id).toBe(1);
});
This test will pass even if the sorting logic is flawed because it makes the same assumptions as the code. A better approach would be to create test data with known expected outcomes and verify those outcomes without assuming how the sorting is implemented.
Happy Path Fixation
Many test suites focus extensively on the “happy path” – the expected flow when everything works correctly. While this is important, bugs often lurk in edge cases, error handling, and unexpected inputs.
Consider this password validation function:
function isPasswordStrong(password) {
return password.length >= 8 &&
/[A-Z]/.test(password) &&
/[a-z]/.test(password) &&
/[0-9]/.test(password);
}
// Happy path test
test('isPasswordStrong validates strong password', () => {
expect(isPasswordStrong('StrongPwd123')).toBe(true);
});
This test only verifies that a strong password passes validation. It doesn’t check what happens with:
- Empty passwords
- Null or undefined values
- Passwords with exactly 8 characters
- Passwords missing one of the required character types
A more comprehensive test suite would explore these scenarios.
Mocking Complexity Away
Mocks and stubs are essential tools in unit testing, but they can also hide integration issues. When you replace complex dependencies with simplified mocks, you might miss bugs that occur when these components interact in the real world.
// Function that relies on a database call
async function getUserSubscriptionStatus(userId) {
const user = await database.findUser(userId);
return user ? user.subscriptionStatus : 'none';
}
// Test with mock
test('getUserSubscriptionStatus returns user subscription', async () => {
// Mock that always returns a user with 'active' status
database.findUser = jest.fn().mockResolvedValue({
subscriptionStatus: 'active'
});
const status = await getUserSubscriptionStatus('user123');
expect(status).toBe('active');
});
This test will pass, but it doesn’t verify how the function behaves when the database returns unexpected data, throws an error, or times out. The mock simplifies the dependency to the point where it no longer represents real-world behavior.
Insufficient Test Data Variety
Many tests use a limited set of test data that doesn’t represent the diversity of inputs the code will encounter in production. This is particularly problematic for functions that process user input or external data.
For a function that calculates age from a birth date:
function calculateAge(birthDate) {
const today = new Date();
const birth = new Date(birthDate);
let age = today.getFullYear() - birth.getFullYear();
// Adjust for months and days
const monthDiff = today.getMonth() - birth.getMonth();
if (monthDiff < 0 || (monthDiff === 0 && today.getDate() < birth.getDate())) {
age--;
}
return age;
}
// Limited test data
test('calculateAge returns correct age', () => {
// Testing with a date that's not near month/day boundaries
const birthDate = '1990-05-15';
const expectedAge = new Date().getFullYear() - 1990;
if (new Date().getMonth() < 4 ||
(new Date().getMonth() === 4 && new Date().getDate() < 15)) {
expectedAge--;
}
expect(calculateAge(birthDate)).toBe(expectedAge);
});
This test only verifies one scenario. It doesn’t check edge cases like:
- Birth date is today (age should be 0)
- Birth date is tomorrow (should handle future dates appropriately)
- Birth date is February 29 in a leap year
- Invalid date formats
Integration Issues Slip Through Unit Tests
Unit tests, by definition, focus on isolated components. While this is valuable for verifying individual functions and classes, it means that integration issues often go undetected.
Component Interaction Bugs
Many bugs occur at the boundaries between components, where assumptions about inputs, outputs, and behavior may not align. These issues are invisible when components are tested in isolation.
Consider two components:
// Component A: User service
class UserService {
async getUser(id) {
const user = await database.findUser(id);
return {
id: user.id,
name: user.name,
isActive: user.status === 'active'
};
}
}
// Component B: Notification service
class NotificationService {
async sendNotification(userId, message) {
const user = await userService.getUser(userId);
if (user.isActive) {
await notificationProvider.send(user.id, message);
return true;
}
return false;
}
}
Unit tests for both services might pass, but an integration bug could occur if the database returns a user with a status of “ACTIVE” (uppercase) instead of “active”. The UserService would mark the user as inactive, and notifications would fail, despite the user having an active status in the database.
Environment and Configuration Differences
Unit tests typically run in a controlled environment that may differ significantly from production. Configuration settings, environment variables, and system resources can all affect how code behaves in the real world.
For example, a function might work perfectly in tests but fail in production due to:
- Different timezone settings
- Lower memory or CPU resources
- Network latency or reliability issues
- Different file system permissions
- Database connection limits
These environmental factors are difficult to simulate in unit tests but can be the source of persistent bugs.
Race Conditions and Timing Issues
Asynchronous code, particularly in multi-threaded or event-driven systems, can exhibit race conditions and timing issues that are nearly impossible to detect with traditional unit tests.
Consider this example of a potential race condition:
class UserCounter {
constructor() {
this.count = 0;
}
async incrementAndLog() {
const currentCount = this.count;
// Simulate an async operation
await someAsyncOperation();
// Update and log
this.count = currentCount + 1;
console.log(`User count: ${this.count}`);
}
}
If multiple calls to incrementAndLog()
occur concurrently, they might all read the same initial value of count
, leading to incorrect increments. A unit test that calls this method sequentially would never reveal this issue.
How to Make Your Tests Catch Real Bugs
Now that we understand why unit tests often miss important bugs, let’s explore strategies to improve their effectiveness.
Focus on Behavior, Not Implementation
Write tests that verify what your code should do, not how it does it. This approach, often called “black-box testing,” helps ensure that your tests remain valid even if the implementation changes.
// Implementation-focused test (fragile)
test('sortUsers sorts by calling Array.sort with timestamp comparison', () => {
const users = [{id: 1, timestamp: 100}, {id: 2, timestamp: 200}];
const sortSpy = jest.spyOn(Array.prototype, 'sort');
sortUsers(users);
expect(sortSpy).toHaveBeenCalled();
});
// Behavior-focused test (robust)
test('sortUsers returns users in descending order by timestamp', () => {
const users = [
{id: 1, timestamp: 100},
{id: 2, timestamp: 300},
{id: 3, timestamp: 200}
];
const result = sortUsers(users);
expect(result[0].id).toBe(2);
expect(result[1].id).toBe(3);
expect(result[2].id).toBe(1);
});
The second test verifies the expected outcome without making assumptions about how sorting is implemented.
Test Edge Cases Systematically
Identify and test edge cases systematically. For each function, consider:
- Empty or null inputs
- Boundary values (minimum, maximum, just inside/outside limits)
- Invalid formats or types
- Unexpected combinations of valid inputs
Property-based testing tools like fast-check (JavaScript) or QuickCheck (Haskell) can help generate diverse test cases automatically.
// Manual edge case testing
test('validateAge handles edge cases', () => {
// Minimum valid age
expect(validateAge(18)).toBe(true);
// Just below minimum
expect(validateAge(17)).toBe(false);
// Maximum valid age
expect(validateAge(120)).toBe(true);
// Above maximum
expect(validateAge(121)).toBe(false);
// Invalid inputs
expect(validateAge(-5)).toBe(false);
expect(validateAge(null)).toBe(false);
expect(validateAge('eighteen')).toBe(false);
});
// Property-based testing with fast-check
test('validateAge properties', () => {
// Valid age range property
fc.assert(
fc.property(fc.integer(18, 120), (age) => {
expect(validateAge(age)).toBe(true);
})
);
// Invalid age range property
fc.assert(
fc.property(fc.integer(-1000, 17), (age) => {
expect(validateAge(age)).toBe(false);
})
);
});
Use Realistic Mocks
When mocking dependencies, strive to create mocks that behave like the real components. This includes simulating error conditions, delays, and edge cases.
// Simplistic mock
database.findUser = jest.fn().mockResolvedValue({
id: 'user123',
name: 'Test User',
status: 'active'
});
// More realistic mock
database.findUser = jest.fn().mockImplementation(async (id) => {
// Simulate network delay
await new Promise(resolve => setTimeout(resolve, 50));
// Simulate different responses based on input
if (!id) {
throw new Error('User ID is required');
}
if (id === 'nonexistent') {
return null;
}
if (id === 'error') {
throw new Error('Database connection failed');
}
return {
id,
name: `User ${id}`,
status: id.includes('inactive') ? 'inactive' : 'active'
};
});
The second mock provides a more realistic simulation of database behavior, including error handling and conditional responses.
Combine Unit Tests with Integration Tests
Unit tests alone aren’t sufficient to catch all bugs. Implement a testing pyramid that includes:
- Unit tests for individual functions and classes
- Integration tests for component interactions
- End-to-end tests for critical user journeys
This multi-layered approach provides more comprehensive coverage and helps catch bugs that might slip through any single layer.
// Unit test for individual component
test('UserService.getUser transforms user data correctly', async () => {
database.findUser = jest.fn().mockResolvedValue({
id: 'user123',
name: 'Test User',
status: 'active'
});
const userService = new UserService(database);
const user = await userService.getUser('user123');
expect(user).toEqual({
id: 'user123',
name: 'Test User',
isActive: true
});
});
// Integration test for component interaction
test('NotificationService uses UserService to check user status', async () => {
// Set up real components instead of mocks
const database = new TestDatabase();
await database.addUser({
id: 'user123',
name: 'Test User',
status: 'active'
});
const userService = new UserService(database);
const notificationProvider = new TestNotificationProvider();
const notificationService = new NotificationService(userService, notificationProvider);
await notificationService.sendNotification('user123', 'Test message');
expect(notificationProvider.sentMessages).toContainEqual({
userId: 'user123',
message: 'Test message'
});
});
Implement Mutation Testing
Mutation testing is a powerful technique that evaluates the quality of your tests by introducing small changes (mutations) to your code and checking if your tests detect these changes.
Tools like Stryker (JavaScript), PITest (Java), or Mutmut (Python) automatically create mutations and run your tests against them. If your tests continue to pass despite the mutations, it indicates potential blind spots in your testing.
For example, a mutation might change:
// Original code
if (user.age >= 18) {
return 'adult';
} else {
return 'minor';
}
// Mutation 1: Change comparison operator
if (user.age > 18) {
return 'adult';
} else {
return 'minor';
}
// Mutation 2: Change return value
if (user.age >= 18) {
return 'ADULT';
} else {
return 'minor';
}
If your tests pass with these mutations, they’re not effectively verifying the behavior of your code.
Test in Production-Like Environments
When possible, run tests in environments that closely resemble production. This can help catch environment-specific issues before they affect users.
Techniques include:
- Containerized testing environments with Docker
- Staging environments that mirror production configuration
- Chaos engineering practices to simulate system failures
- Load testing to identify performance issues
While these approaches go beyond traditional unit testing, they’re essential for building truly reliable software.
Advanced Testing Strategies for Complex Systems
For complex systems with many moving parts, additional strategies can help catch elusive bugs.
Property-Based Testing
Property-based testing moves beyond specific examples to test properties that should hold true for all valid inputs. This approach can uncover edge cases that you might not have considered.
// Traditional example-based test
test('reverse function works correctly', () => {
expect(reverse([1, 2, 3])).toEqual([3, 2, 1]);
expect(reverse([4, 5])).toEqual([5, 4]);
});
// Property-based test
test('reverse function properties', () => {
fc.assert(
fc.property(fc.array(fc.anything()), (arr) => {
// Property 1: Reversing twice gives the original array
expect(reverse(reverse(arr))).toEqual(arr);
// Property 2: Length is preserved
expect(reverse(arr).length).toBe(arr.length);
// Property 3: First element becomes last
if (arr.length > 0) {
expect(reverse(arr)[arr.length - 1]).toEqual(arr[0]);
}
})
);
});
Property-based testing can discover bugs by generating hundreds of test cases automatically, including edge cases you might not have thought to test manually.
Fuzz Testing
Fuzz testing involves providing random, unexpected, or malformed inputs to your application to identify crashes, memory leaks, or other vulnerabilities.
While traditionally used for security testing, fuzzing can also uncover functional bugs, especially in code that processes user input or external data.
// Simple fuzzer for a URL parser
function fuzzUrlParser(iterations = 1000) {
const fuzzGenerator = () => {
// Generate random strings that might break URL parsing
const chars = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789:/?.&%#[]@!$^*()_+-={}|\\';
let result = '';
const length = Math.floor(Math.random() * 100);
for (let i = 0; i < length; i++) {
result += chars.charAt(Math.floor(Math.random() * chars.length));
}
// Sometimes prepend http:// or https://
if (Math.random() > 0.7) {
result = (Math.random() > 0.5 ? 'http://' : 'https://') + result;
}
return result;
};
for (let i = 0; i < iterations; i++) {
const fuzzInput = fuzzGenerator();
try {
// Test that the parser doesn't crash
const result = parseUrl(fuzzInput);
// Verify that the result meets basic expectations
expect(typeof result).toBe('object');
} catch (error) {
// Log the input that caused the failure
console.error(`Fuzz test failed with input: ${fuzzInput}`);
throw error;
}
}
}
test('URL parser handles random inputs without crashing', () => {
fuzzUrlParser();
});
Snapshot Testing
Snapshot testing captures the output of a component or function and compares it to a previously saved “snapshot.” This approach is particularly useful for detecting unintended changes in complex outputs like UI components or API responses.
// Snapshot test for a React component
test('UserProfile renders correctly', () => {
const user = {
id: 'user123',
name: 'Jane Doe',
email: 'jane@example.com',
role: 'admin',
lastActive: '2023-05-15T14:30:00Z'
};
const component = renderer.create(
<UserProfile user={user} />
);
let tree = component.toJSON();
expect(tree).toMatchSnapshot();
});
// Snapshot test for an API response
test('getUserDetails API returns expected structure', async () => {
const response = await api.getUserDetails('user123');
expect(response).toMatchSnapshot();
});
Snapshot tests can detect unintended changes in output structure, making them valuable for catching regressions. However, they should be used carefully, as they can be brittle if the expected output changes frequently.
Concurrency Testing
For systems with concurrent operations, specialized testing approaches can help identify race conditions and timing issues.
// Testing a counter for race conditions
test('Counter handles concurrent increments correctly', async () => {
const counter = new Counter();
// Create 100 concurrent increment operations
const incrementPromises = Array(100).fill().map(() => counter.increment());
// Wait for all operations to complete
await Promise.all(incrementPromises);
// Verify that the counter has the expected value
expect(counter.value).toBe(100);
});
For more complex concurrency issues, tools like Java’s jcstress or specialized frameworks can help simulate various thread interleavings and timing scenarios.
Cultivating a Testing Mindset
Beyond specific techniques, effective testing requires a shift in mindset across the development team.
Think Like a User, Not a Developer
When writing tests, try to adopt the perspective of users who will interact with your system. They don’t know or care about your internal implementation details; they only care that the system behaves as expected.
This mindset shift can help you focus on testing behavior rather than implementation and identify scenarios that real users might encounter.
Embrace Test-Driven Development (TDD)
Test-Driven Development, where tests are written before the code they test, can help ensure that your code is designed with testability in mind. The TDD cycle of “Red-Green-Refactor” encourages a focus on behavior specification and incremental development.
By writing tests first, you’re forced to think about how your code will be used and what behaviors it should exhibit, leading to more focused and testable designs.
Treat Test Code as First-Class Code
Test code deserves the same care and attention as production code. This means:
- Applying clean code principles to test code
- Refactoring tests when they become unwieldy
- Creating reusable test utilities and fixtures
- Reviewing test code with the same rigor as production code
High-quality test code is more likely to catch bugs and less likely to become a maintenance burden.
Learn from Production Bugs
When bugs do make it to production, use them as learning opportunities:
- Add regression tests that would have caught the bug
- Analyze why existing tests didn’t catch it
- Identify patterns in escaped bugs and adjust testing strategies accordingly
This continuous improvement cycle helps strengthen your testing approach over time.
Conclusion
Unit tests are a valuable tool for catching bugs early in the development process, but they’re not a silver bullet. By understanding their limitations and complementing them with other testing approaches, you can build a more effective testing strategy that catches real bugs before they affect users.
Remember that effective testing is about more than just achieving high coverage metrics. It requires a thoughtful approach to test design, a commitment to testing diverse scenarios, and a willingness to evolve your testing strategies based on real-world experience.
By adopting the techniques and mindsets discussed in this article, you can move beyond superficial test coverage to create tests that genuinely protect your code from bugs. The result will be more reliable software, happier users, and fewer late-night debugging sessions.
Now, go forth and write tests that catch real bugs!