Why Your Testing Environment Isn’t Catching Real World Scenarios
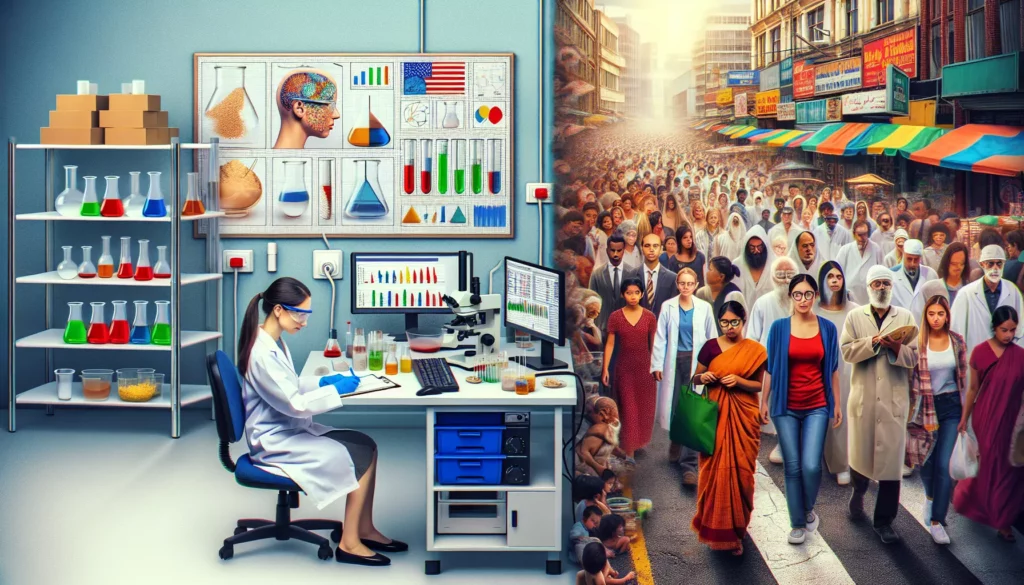
In the world of software development, testing is the safety net that prevents bugs from reaching production. Yet, many organizations find themselves puzzled when issues that never appeared in testing suddenly emerge in the real world. Despite extensive test suites, well-crafted unit tests, and dedicated QA teams, software continues to fail in unexpected ways once it reaches users.
This disconnect between testing environments and production realities is more common than you might think. As developers, we strive for perfection, but the gap between our controlled testing environments and the chaotic real world often leads to unforeseen problems.
In this comprehensive guide, we’ll explore why your testing environment might not be catching real world scenarios, and more importantly, what you can do to bridge this critical gap.
The Illusion of Complete Testing
Many development teams operate under what could be called the “illusion of complete testing” – the belief that their test suite adequately covers all possible scenarios. This misconception stems from several factors:
The Controlled Environment Fallacy
Testing environments are, by definition, controlled spaces. They’re designed to be predictable, stable, and consistent. This stands in stark contrast to production environments, which are subject to unpredictable user behavior, varying load patterns, and a multitude of external dependencies.
When we test in these sanitized environments, we’re essentially testing under ideal conditions that rarely exist in the real world. It’s like testing a car’s performance on a perfectly smooth track and then being surprised when it struggles on a bumpy country road.
The Confirmation Bias in Test Design
As humans, we’re prone to confirmation bias – the tendency to search for, interpret, and recall information in a way that confirms our preexisting beliefs. This bias manifests in how we design tests.
When writing tests, developers often unconsciously focus on scenarios that confirm their code works correctly rather than actively trying to break it. This results in tests that validate expected behavior but miss edge cases and unexpected inputs that real users might encounter.
The Complexity of Modern Software Systems
Modern software systems are incredibly complex, often comprising multiple services, third party dependencies, databases, and external APIs. This complexity makes it nearly impossible to test every possible interaction and failure mode.
Even with extensive integration testing, the sheer number of possible states and interactions in a complex system means that some scenarios will inevitably go untested.
Common Testing Environment Limitations
Let’s examine specific ways in which testing environments typically fall short of representing real world conditions:
Network Conditions and Latency
In most testing environments, network connections are reliable, fast, and low-latency. Developers often work on powerful machines with high-speed internet connections, and even dedicated testing environments typically have excellent network infrastructure.
In contrast, real users might access your application:
- On spotty mobile connections that frequently drop packets
- From geographical locations far from your servers, introducing significant latency
- Behind corporate firewalls or restrictive network policies
- On throttled connections with limited bandwidth
An application that performs flawlessly in your testing environment might time out, fail to load resources, or behave unpredictably under these varied network conditions.
Hardware and Device Diversity
The diversity of devices and hardware configurations used by real users is staggering. Your application might need to function on:
- High-end workstations with multiple cores and abundant RAM
- Budget smartphones with limited processing power
- Tablets with different screen sizes and aspect ratios
- Older machines running outdated operating systems
- Devices with unusual hardware configurations or accessibility peripherals
Testing environments rarely capture this diversity, often focusing on a few common configurations or emulators that approximate but don’t fully replicate real device behavior.
Data Volume and Variety
Testing environments typically use small, carefully curated datasets that don’t represent the volume or variety of data found in production. This leads to several blind spots:
- Performance issues that only appear with large datasets
- Edge cases involving unusual or unexpected data formats
- Memory leaks that aren’t apparent with limited data processing
- Race conditions that emerge only under high-throughput scenarios
A query that executes instantly against a test database with a few thousand records might bring a production system to its knees when run against millions of records.
User Behavior Unpredictability
Perhaps the most significant limitation of testing environments is their inability to predict the vast range of ways users will interact with your software. Users will:
- Click buttons multiple times in rapid succession
- Enter unexpected inputs (including malicious ones)
- Use browser features like back/forward navigation in unanticipated ways
- Leave applications idle for extended periods before resuming
- Access features in sequences that developers never imagined
No test suite, no matter how comprehensive, can anticipate all these behaviors.
The Hidden Costs of Inadequate Testing
When testing environments fail to catch real world issues, the consequences extend far beyond the immediate technical problems:
Customer Trust Erosion
Each production issue that affects users chips away at their trust in your product. While users might forgive occasional minor issues, repeated problems or significant failures can permanently damage your reputation.
This erosion of trust is particularly damaging for B2B software, where reliability is often a key selling point and where contracts might include service level agreements (SLAs) with financial penalties for downtime.
Increased Support Costs
Production issues that slip through testing inevitably increase support costs. Your support team must handle more tickets, engineers need to be pulled from development work to address urgent issues, and management must allocate resources to crisis response rather than planned initiatives.
These costs can be substantial, especially for organizations with large user bases where even a small issue can generate thousands of support requests.
Developer Productivity Impact
The psychological impact on development teams shouldn’t be underestimated. Constantly firefighting production issues leads to:
- Reduced morale as developers feel their work is always flawed
- Context switching costs as developers are pulled from planned work to fix urgent issues
- Increased stress and potential burnout from unpredictable crisis response duties
- Less time for thoughtful code improvement and technical debt reduction
Over time, these factors can lead to a negative cycle where rushed fixes introduce new issues, further straining the team.
Bridging the Gap: Strategies for More Realistic Testing
While it’s impossible to perfectly simulate every real world scenario, several strategies can help bridge the gap between testing environments and production realities:
Chaos Engineering: Embracing Controlled Failure
Chaos engineering, pioneered by Netflix with their Chaos Monkey tool, involves deliberately introducing failures into your system to test its resilience. This approach acknowledges that failures will happen and focuses on building systems that degrade gracefully rather than catastrophically.
Practical implementations of chaos engineering include:
- Randomly terminating servers or containers to test recovery mechanisms
- Introducing network latency or packet loss to test timeout handling
- Simulating service dependencies going offline to test fallback strategies
- Consuming system resources (CPU, memory) to test performance degradation handling
By proactively causing failures in controlled circumstances, teams can identify weaknesses before they affect real users.
Production Monitoring and Observability
Robust monitoring and observability tools provide visibility into how your application behaves in production, helping you catch issues that testing missed. Modern observability goes beyond simple metrics to provide deep insights into system behavior:
- Distributed tracing to follow requests across service boundaries
- Detailed performance profiling to identify bottlenecks
- Error tracking with context to understand failure modes
- User session recording to see exactly how users experience issues
These tools allow teams to detect anomalies, understand their impact, and quickly diagnose root causes.
Canary Releases and Feature Flags
Rather than releasing new features to all users simultaneously, canary releases and feature flags allow for more controlled deployments:
- Canary releases deploy changes to a small percentage of users first, allowing teams to monitor for issues before expanding the rollout
- Feature flags enable features to be toggled on or off without redeployment, providing fine-grained control over what functionality is available to which users
These approaches limit the blast radius of potential issues and provide early warning of problems that testing didn’t catch.
Environment Parity: Production-Like Testing
While perfect replication of production environments is often impractical, teams can strive for greater parity between testing and production:
- Using containerization to ensure consistent environments across development, testing, and production
- Testing with anonymized production data (with appropriate privacy controls)
- Implementing infrastructure as code to maintain consistent configurations
- Running performance tests against environments scaled proportionally to production
The closer testing environments resemble production, the more likely they are to catch real world issues.
Advanced Testing Approaches for Real World Scenarios
Beyond the foundational strategies, several advanced approaches can further improve your ability to catch real world issues before they affect users:
Property Based Testing
Traditional unit tests verify specific inputs and outputs, but property based testing takes a different approach. Instead of testing individual cases, it focuses on verifying properties that should hold true for all inputs.
For example, rather than testing that sorting function works for a specific array, property based testing would verify properties like “the sorted array has the same length as the input” and “every element in the sorted array is greater than or equal to the previous element.”
Tools like QuickCheck (Haskell), Hypothesis (Python), and jsverify (JavaScript) can generate thousands of test cases automatically, often finding edge cases that developers would never think to test manually.
Load Testing Beyond Breaking Points
Many load testing approaches focus on verifying that a system can handle expected peak loads. While valuable, this doesn’t tell you how the system will behave when those limits are exceeded.
More comprehensive load testing should explore:
- Graceful degradation under extreme load
- Recovery behavior after load subsides
- Failure modes when system limits are reached
- Resource exhaustion scenarios (memory, connections, file handles)
Understanding how your system fails under extreme conditions helps you implement appropriate safeguards and fallbacks.
User Journey Testing with Synthetic Monitoring
Synthetic monitoring involves automating key user journeys and regularly executing them against your production environment. Unlike traditional end-to-end tests, synthetic monitoring:
- Runs continuously against actual production systems
- Tests from multiple geographic locations
- Measures real performance as experienced by users
- Alerts teams to degradation or failures immediately
This approach bridges the gap between pre-deployment testing and production monitoring, providing early warning of issues that affect critical user journeys.
Fault Injection Testing
Building on the principles of chaos engineering, fault injection testing deliberately introduces specific faults into systems to observe their behavior. This might include:
- Corrupting data in transit to test validation and error handling
- Introducing timing issues to expose race conditions
- Simulating partial system failures to test degraded operation modes
- Manipulating system clocks to test time-dependent functionality
By precisely targeting potential failure points, teams can verify that their error handling works as expected.
Implementation Challenges and Practical Solutions
While the strategies above can significantly improve testing effectiveness, implementing them presents several challenges:
Resource Constraints
Challenge: Creating and maintaining production-like testing environments can be expensive, especially for large-scale systems.
Solutions:
- Use ephemeral environments that spin up only when needed for testing
- Implement representative scaling where test environments mirror production architecture but at a smaller scale
- Leverage cloud resources with pay-as-you-go pricing for intensive testing phases
- Prioritize production parity for critical components while using simpler mocks for less critical dependencies
Test Data Management
Challenge: Using realistic data volumes while maintaining privacy and compliance requirements.
Solutions:
- Develop data anonymization pipelines that preserve statistical properties while removing personal information
- Create data generation tools that produce synthetic datasets with realistic characteristics
- Implement data subsetting techniques that maintain relational integrity while reducing volume
- Use production data sampling with appropriate access controls and legal safeguards
Testing Culture and Priorities
Challenge: Building a culture that values thorough testing when development speed is often prioritized.
Solutions:
- Track and publicize “escaped defects” metrics to highlight the cost of inadequate testing
- Implement “testing champions” within development teams to advocate for testing best practices
- Include testing considerations in definition of done criteria for all work
- Share post-mortems of production issues that could have been caught by better testing
Skill and Knowledge Gaps
Challenge: Advanced testing approaches require specialized knowledge that teams may lack.
Solutions:
- Provide targeted training on specific testing methodologies
- Partner with experts or consultants for initial implementation
- Start with simplified versions of advanced techniques and gradually increase sophistication
- Build reusable testing frameworks that encapsulate complexity
Code Examples: Implementing Realistic Testing
Let’s examine some practical code examples that implement the strategies discussed above:
Network Condition Simulation with Toxiproxy
Toxiproxy is a TCP proxy designed for simulating network conditions in testing environments. Here’s how you might use it to test how your application handles network latency:
// First, set up a Toxiproxy instance for your database connection
const { Toxiproxy } = require('toxiproxy-node-client');
const toxiproxy = new Toxiproxy('http://localhost:8474');
async function testWithNetworkLatency() {
// Create or get a proxy for your database connection
let dbProxy = await toxiproxy.createProxy({
name: 'mysql',
listen: 'localhost:3306',
upstream: 'my-actual-db:3306'
});
// Add 1000ms latency to all database requests
await dbProxy.addToxic({
type: 'latency',
attributes: {
latency: 1000,
jitter: 100
}
});
// Run your tests against the proxied connection
await runDatabaseTests();
// Remove the toxic condition
await dbProxy.removeToxic('latency');
}
This approach allows you to verify that your application properly handles database queries that take longer than expected, potentially identifying timeout issues or UI problems that only occur under high latency.
Property Based Testing with Jest and fast-check
For JavaScript applications, combining Jest with fast-check enables powerful property based testing:
import fc from 'fast-check';
import { sortArray } from './arrayUtils';
describe('Array sorting', () => {
test('sort should maintain the same array length', () => {
fc.assert(
fc.property(fc.array(fc.integer()), (arr) => {
const sorted = sortArray(arr);
return sorted.length === arr.length;
})
);
});
test('sort should produce elements in non-decreasing order', () => {
fc.assert(
fc.property(fc.array(fc.integer()), (arr) => {
const sorted = sortArray(arr);
for (let i = 1; i < sorted.length; i++) {
if (sorted[i] < sorted[i-1]) return false;
}
return true;
})
);
});
test('sort should contain all the original elements', () => {
fc.assert(
fc.property(fc.array(fc.integer()), (arr) => {
const sorted = sortArray(arr);
// Create frequency maps
const freqBefore = new Map();
const freqAfter = new Map();
for (const item of arr) {
freqBefore.set(item, (freqBefore.get(item) || 0) + 1);
}
for (const item of sorted) {
freqAfter.set(item, (freqAfter.get(item) || 0) + 1);
}
// Check that frequencies match
for (const [key, value] of freqBefore) {
if (freqAfter.get(key) !== value) return false;
}
return true;
})
);
});
});
Instead of testing a few specific cases, this approach automatically generates hundreds of test cases, systematically exploring the behavior of your sorting function across a wide range of inputs.
Chaos Testing with Chaos Toolkit
Chaos Toolkit provides a declarative way to define and execute chaos experiments. Here’s an example experiment that tests how your application handles a database failure:
{
"version": "1.0.0",
"title": "Database failure resilience test",
"description": "Verify that the application can handle database outages gracefully",
"tags": ["database", "resilience"],
"steady-state-hypothesis": {
"title": "Application is healthy",
"probes": [
{
"type": "http",
"name": "api-responds",
"tolerance": 200,
"url": "https://my-application/health"
}
]
},
"method": [
{
"type": "action",
"name": "stop-database",
"provider": {
"type": "process",
"path": "kubectl",
"arguments": ["scale", "deployment", "database", "--replicas=0"]
}
},
{
"type": "probe",
"name": "api-degrades-gracefully",
"tolerance": {
"type": "regex",
"pattern": ".*Service Temporarily Unavailable.*"
},
"url": "https://my-application/data-endpoint"
},
{
"type": "action",
"name": "restart-database",
"provider": {
"type": "process",
"path": "kubectl",
"arguments": ["scale", "deployment", "database", "--replicas=1"]
}
}
],
"rollbacks": [
{
"type": "action",
"name": "restore-database",
"provider": {
"type": "process",
"path": "kubectl",
"arguments": ["scale", "deployment", "database", "--replicas=1"]
}
}
]
}
This experiment verifies that your application responds appropriately when the database becomes unavailable and recovers properly when service is restored – conditions that are difficult to test in traditional testing environments.
Measuring Testing Effectiveness
How do you know if your testing strategy is effectively catching real world issues? Several metrics and approaches can help:
Escaped Defects Analysis
Track and categorize production issues that weren’t caught in testing. For each issue, analyze:
- Why testing didn’t catch it (missing test case, environment difference, etc.)
- What testing approach would have been most likely to catch it
- The severity and impact of the issue
This analysis helps identify patterns and prioritize improvements to your testing strategy.
Test Coverage Beyond Code Coverage
While code coverage (the percentage of code executed during tests) is a common metric, more sophisticated coverage measures provide better insights:
- Path coverage: What percentage of possible execution paths through the code are tested?
- Data flow coverage: Are all data transformations and state changes tested?
- Boundary coverage: Are edge cases and limit conditions thoroughly tested?
- Requirement coverage: What percentage of functional requirements have associated tests?
These measures provide a more nuanced view of testing thoroughness.
Mean Time To Detection (MTTD)
For issues that do reach production, measure how quickly they’re detected. A decreasing MTTD indicates that your monitoring and observability tools are becoming more effective at catching issues early, before they affect many users.
User-Reported vs. System-Detected Issues
Track what percentage of production issues are first reported by users versus being detected by your monitoring systems. As your testing and monitoring improves, the ratio should shift toward system-detected issues, indicating that you’re catching problems before users experience them.
Conclusion: Embracing the Complexity of Real World Testing
The gap between testing environments and real world scenarios is not a problem to be solved once and forgotten, but rather an ongoing challenge that requires continuous attention and improvement. As systems grow more complex and user expectations rise, the sophistication of testing approaches must evolve accordingly.
The most successful testing strategies acknowledge this reality and embrace a multi-faceted approach:
- Combining traditional testing methodologies with newer techniques like chaos engineering and property based testing
- Blurring the line between pre-deployment testing and production monitoring
- Building systems that are resilient to failure rather than assuming failures can be entirely prevented
- Creating a culture that values thorough testing as an essential component of quality software
By embracing the complexity of real world scenarios in your testing approach, you can build more reliable systems, reduce production incidents, and ultimately deliver better experiences to your users.
Remember that perfect testing is impossible, but significant improvement is always within reach. Each step toward more realistic testing brings you closer to the confidence that your software will perform as expected, not just in the controlled environment of your test suite, but in the messy, unpredictable real world where your users live.