Why Your Technical Decisions Aren’t Standing the Test of Time
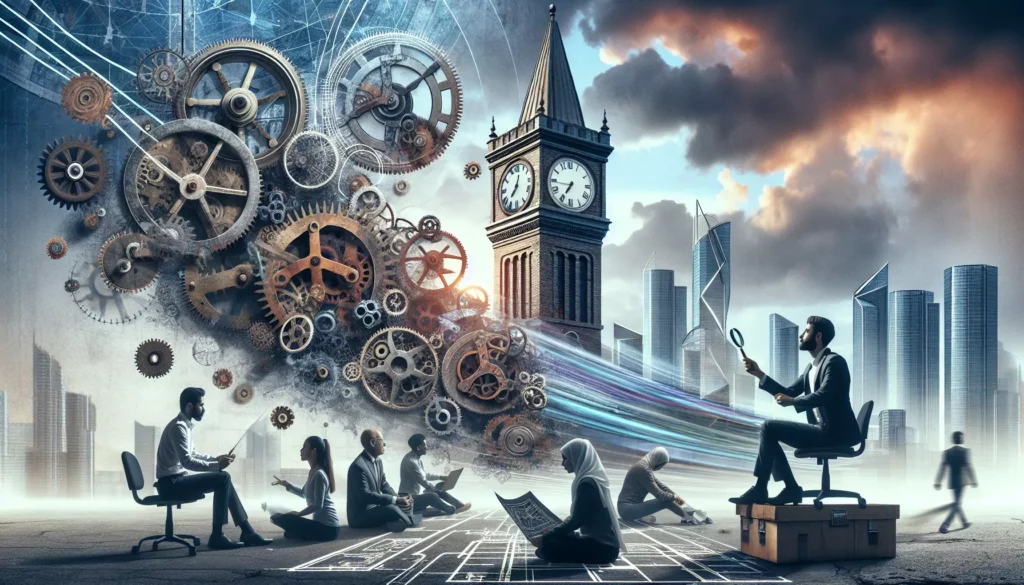
In the fast paced world of software development, making technical decisions that last is increasingly challenging. Today’s innovative solution can become tomorrow’s technical debt, leaving teams struggling with legacy systems that are difficult to maintain and scale. At AlgoCademy, we’ve observed this pattern across organizations of all sizes, from startups to FAANG companies.
The consequences of short lived technical decisions extend beyond just maintenance headaches. They impact team morale, product stability, and ultimately the bottom line. In this comprehensive guide, we’ll explore why technical decisions often fail to stand the test of time and provide actionable strategies to make more durable choices.
The Hidden Cost of Ephemeral Technical Decisions
Before diving into solutions, let’s understand the true cost of technical decisions that don’t last:
- Mounting Technical Debt: Short sighted decisions accumulate, creating a compounding burden on development teams.
- Decreased Velocity: Teams spend increasing amounts of time maintaining old systems rather than building new features.
- Knowledge Silos: As systems become more complex, knowledge becomes concentrated in fewer individuals, creating organizational risk.
- Onboarding Friction: New team members struggle to understand convoluted systems built on outdated decisions.
- Innovation Barriers: Legacy architectures make adopting new technologies and methodologies difficult.
According to research by McKinsey, organizations with significant technical debt spend up to 40% of their development resources on maintenance rather than innovation. This opportunity cost is often invisible on financial statements but has real impact on competitiveness.
Common Patterns of Technical Decision Failure
After analyzing hundreds of software projects at AlgoCademy, we’ve identified several recurring patterns that lead to technical decisions aging poorly:
1. Trend Chasing Without Strategic Evaluation
The technology landscape is filled with new frameworks, libraries, and tools promising revolutionary improvements. The temptation to adopt the latest technology is strong, but without proper evaluation, these choices often lead to regret.
Consider the JavaScript ecosystem over the past decade. Teams that jumped between Angular, React, Vue, and Svelte with each new release often found themselves maintaining multiple codebases with different paradigms. Meanwhile, organizations that carefully evaluated each technology against their specific needs before adoption generally fared better.
// Example: Trend chasing in action
// 2016: "Let's rewrite everything in Angular!"
angularApp.component('userProfile', {
templateUrl: 'user-profile.html',
controller: UserProfileController
});
// 2018: "React is better now, let's switch!"
class UserProfile extends React.Component {
render() {
return <div className="profile">{this.props.name}</div>;
}
}
// 2020: "Hooks are the future!"
function UserProfile({ name }) {
return <div className="profile">{name}</div>;
}
// 2022: "Let's try Svelte!"
<script>
export let name;
</script>
<div class="profile">{name}</div>
2. Optimizing Prematurely for Scale
Donald Knuth famously stated that “premature optimization is the root of all evil.” Many technical decisions fail because they’re made with hypothetical future scale in mind rather than current needs.
For example, adopting complex microservice architectures before they’re necessary can introduce distributed systems challenges without providing immediate benefits. Similarly, choosing NoSQL databases for simple CRUD applications often adds complexity without corresponding advantages.
// Premature optimization example:
// Complex caching mechanism added before needed
class DataService {
constructor() {
this.cache = new LRUCache(1000);
this.cacheTTL = 3600; // 1 hour
this.cacheHits = 0;
this.cacheMisses = 0;
}
async getData(id) {
// Complex caching logic for a service that rarely gets duplicate requests
const cacheKey = `data_${id}`;
if (this.cache.has(cacheKey)) {
this.cacheHits++;
return this.cache.get(cacheKey);
}
this.cacheMisses++;
const data = await this.fetchFromDatabase(id);
this.cache.set(cacheKey, data, this.cacheTTL);
return data;
}
}
3. Ignoring Operational Complexity
Many technical decisions look great on paper but fail to account for the operational burden they create. Technologies that require specialized knowledge, complex deployment processes, or extensive monitoring can become unsustainable as teams change and priorities shift.
For instance, Kubernetes offers powerful container orchestration capabilities, but for small teams with simple deployment needs, it can introduce more complexity than value. The same applies to intricate CI/CD pipelines, complex build systems, and custom infrastructure.
4. Underestimating the Importance of Developer Experience
Technical decisions that create friction for developers tend to be abandoned or worked around over time. This includes choices that make local development difficult, slow down build times, or require extensive configuration for common tasks.
Teams that prioritize developer experience generally produce more maintainable and consistent code. This includes investing in tools, documentation, and processes that make development more efficient and enjoyable.
5. Making Decisions in Isolation
Decisions made without considering the broader technical and business context rarely stand the test of time. This includes choosing technologies that don’t integrate well with existing systems, adopting patterns that don’t align with team skills, or implementing solutions that don’t support business objectives.
Cross functional collaboration is essential for making technical decisions that last. This means including perspectives from product, design, operations, and business stakeholders in the decision making process.
Principles for Making Enduring Technical Decisions
Now that we understand the common pitfalls, let’s explore principles that can help make technical decisions more durable:
1. Value Simplicity Over Complexity
Simple solutions are easier to understand, maintain, and adapt. Before introducing complexity, ask whether it’s truly necessary to solve the problem at hand.
The Unix philosophy of “do one thing and do it well” remains relevant decades after its formulation. Systems composed of simple, focused components tend to be more resilient to change than monolithic architectures.
// Complex approach
class UserManager {
constructor(db, cache, notifier, logger, analytics) {
this.db = db;
this.cache = cache;
this.notifier = notifier;
this.logger = logger;
this.analytics = analytics;
}
async createUser(userData) {
// Complex implementation with many dependencies
// ...
}
}
// Simpler approach
class UserRepository {
constructor(db) {
this.db = db;
}
async createUser(userData) {
return this.db.users.create(userData);
}
}
// Separate concerns into focused components
2. Adopt the “Boring Technology” Mindset
There’s wisdom in choosing established, proven technologies for critical systems. Dan McKinley’s concept of a “technology innovation credit” suggests limiting new technology adoption to areas where it provides substantial benefits.
For example, relational databases like PostgreSQL have stood the test of time for structured data storage. Similarly, battle tested languages like Python, Java, and JavaScript have extensive ecosystems and community support.
This doesn’t mean never adopting new technologies, but rather being strategic about where and when to introduce them.
3. Design for Evolutionary Architecture
Rather than attempting to predict future requirements perfectly, focus on creating systems that can evolve gracefully. This includes:
- Loose coupling: Minimizing dependencies between components
- High cohesion: Grouping related functionality together
- Clear interfaces: Defining stable contracts between components
- Incremental migration paths: Enabling gradual system changes
The strangler fig pattern, for instance, allows gradually replacing legacy systems by intercepting calls to the old system and routing them to new components over time.
// Example of evolutionary architecture with adapter pattern
// This allows changing the underlying implementation without affecting clients
// Stable interface
interface PaymentProcessor {
processPayment(amount: number, paymentInfo: PaymentInfo): Promise<PaymentResult>;
}
// Original implementation
class StripePaymentProcessor implements PaymentProcessor {
processPayment(amount: number, paymentInfo: PaymentInfo): Promise<PaymentResult> {
// Implementation using Stripe
}
}
// Later, we can add a new implementation without changing clients
class BraintreePaymentProcessor implements PaymentProcessor {
processPayment(amount: number, paymentInfo: PaymentInfo): Promise<PaymentResult> {
// Implementation using Braintree
}
}
4. Make Decisions Reversible When Possible
Amazon’s Jeff Bezos distinguishes between “Type 1” (irreversible) and “Type 2” (reversible) decisions. Whenever possible, structure technical choices to be reversible, allowing for course correction as more information becomes available.
This might include:
- Using feature flags to gradually roll out changes
- Implementing the adapter pattern to abstract away implementation details
- Building migration tools alongside new systems
- Maintaining backward compatibility during transitions
5. Document Decision Context, Not Just Outcomes
Technical decisions make sense in a particular context, but that context often gets lost over time. Architecture Decision Records (ADRs) capture not just what was decided, but why it was decided, including:
- The problem being solved
- Constraints and requirements
- Alternatives considered
- Expected consequences
- Future decision points
This documentation helps future team members understand the rationale behind decisions and evaluate whether they remain valid as circumstances change.
// Example Architecture Decision Record (ADR)
# ADR-001: Adoption of GraphQL for API Layer
## Status
Accepted
## Context
Our REST API has grown organically, resulting in:
- Multiple endpoints with similar but slightly different data
- Clients frequently needing to make multiple requests
- Difficulties maintaining consistent documentation
- Feature teams unable to independently evolve their APIs
## Decision
We will adopt GraphQL as our primary API technology for new features and
gradually migrate existing REST endpoints.
## Alternatives Considered
1. Continue with REST and improve documentation/consistency
2. Adopt gRPC for performance-critical paths
3. Create a new REST API version with better design
## Consequences
Positive:
- Clients can request exactly the data they need
- Strong typing and introspection improve developer experience
- Schema serves as a single source of truth for API capabilities
Negative:
- Learning curve for team members unfamiliar with GraphQL
- Need to manage complexity of GraphQL resolver layer
- Potential performance issues with complex queries
## Implementation Plan
1. Start with a single non-critical feature
2. Develop internal expertise and best practices
3. Create guidelines for schema design
4. Build tooling for testing and monitoring
Practical Strategies for Different Time Horizons
Different decisions need different time horizons. Here’s how to approach technical decisions based on their expected lifespan:
Short Term Decisions (0-1 Years)
For solutions addressing immediate needs that will likely be replaced:
- Optimize for speed and simplicity rather than perfect architecture
- Clearly label temporary solutions as technical debt to be addressed later
- Avoid dependencies that could make replacement difficult
- Document constraints that led to the temporary approach
Medium Term Decisions (1-3 Years)
For solutions expected to last through several development cycles:
- Balance innovation with stability by using proven technologies for core components
- Design clear component boundaries to allow for partial replacements
- Invest in automated testing to enable safer evolution
- Consider operational requirements like monitoring and scalability
Long Term Decisions (3+ Years)
For foundational architecture decisions expected to last:
- Prioritize technologies with strong communities and long term support
- Design for extensibility rather than trying to predict all future requirements
- Make larger upfront investments in tooling and documentation
- Consider industry standards over proprietary solutions
- Plan for graceful evolution rather than assuming permanence
Case Studies: Technical Decisions That Stood the Test of Time
Learning from successful examples can provide valuable insights. Here are some notable cases of technical decisions that have proven durable:
Case Study 1: Amazon’s Service-Oriented Architecture
In the early 2000s, Amazon made the decision to move from a monolithic architecture to a service-oriented approach. This decision, which predated the current microservices trend, has enabled Amazon to scale both its technology and organization for over two decades.
Key factors in this decision’s longevity:
- Services were defined around business capabilities rather than technical functions
- Teams were organized around services, creating alignment between organizational and technical boundaries
- Clear interfaces and APIs enabled independent evolution of services
- The approach allowed for gradual migration rather than a “big bang” rewrite
Case Study 2: UNIX Philosophy
The UNIX philosophy, developed in the 1970s, continues to influence modern software design:
- Write programs that do one thing and do it well
- Write programs to work together
- Write programs to handle text streams, because that is a universal interface
These principles have proven remarkably durable across generations of technology, from command-line tools to microservices architectures.
Case Study 3: Python’s Backward Compatibility
Python’s commitment to backward compatibility (with the notable exception of the 2-to-3 transition) has contributed to its longevity and widespread adoption. The language has evolved significantly while maintaining compatibility with existing codebases, allowing for incremental adoption of new features.
Applying These Principles at Different Career Stages
The approach to technical decision making evolves as you progress in your career. Here’s how these principles apply at different stages:
For Junior Developers
- Focus on understanding why decisions were made, not just how to implement them
- Learn from historical context by studying system evolution
- Seek mentorship from experienced developers when making design choices
- Practice explaining the rationale behind your technical decisions
For Mid-Level Developers
- Balance technical excellence with practical constraints
- Consider the full lifecycle of features, including maintenance
- Develop awareness of organizational context that influences technical decisions
- Lead smaller architectural decisions with guidance from senior team members
For Senior Developers and Architects
- Mentor others in making durable technical decisions
- Create decision-making frameworks for your organization
- Balance short-term needs with long-term sustainability
- Recognize when to evolve existing architecture versus starting fresh
- Consider broader impacts across teams and systems
Common Objections and Counterarguments
Even with these principles, objections often arise when advocating for more durable technical decisions:
“We need to move fast and can’t afford to over-engineer”
Response: Durable decisions aren’t necessarily more complex or time-consuming. Often, simpler solutions last longer. The key is distinguishing between necessary complexity and accidental complexity. Investing in clear interfaces and boundaries can actually enable moving faster in the long run.
“We can’t predict the future, so why try to build for it?”
Response: The goal isn’t to predict specific future requirements but to create systems that can evolve gracefully as requirements change. This is about maintaining options rather than attempting perfect foresight.
“New technologies provide competitive advantages we can’t ignore”
Response: Innovation is important, but it should be applied strategically. Use the “innovation token” concept—spend your limited tokens on technologies that provide unique value to your core business, and stick with proven solutions elsewhere.
“Our business needs are changing too rapidly for long-term planning”
Response: Rapidly changing business needs actually increase the importance of stable technical foundations. The more your requirements change, the more you need architecture that can accommodate change without requiring complete rewrites.
Measuring the Durability of Technical Decisions
How do you know if your technical decisions are standing the test of time? Here are some metrics and indicators to consider:
Quantitative Metrics
- Change Frequency: How often does the component need significant rework?
- Defect Density: Are certain components consistently generating more bugs?
- Maintenance Time: How much developer time is spent maintaining versus enhancing?
- Dependency Updates: How difficult is it to update dependencies?
- Time to Onboard: How long does it take new team members to become productive?
Qualitative Indicators
- Developer Sentiment: Do developers avoid working on certain parts of the system?
- Architectural Drift: Is the actual implementation diverging from the intended architecture?
- Knowledge Distribution: Is knowledge of the system concentrated in a few individuals?
- Technical Debt Discussions: How frequently do technical debt issues block progress?
- Feature Delivery Time: Is the time to deliver new features increasing over time?
Creating a Decision-Making Framework for Your Team
To apply these principles consistently, consider establishing a decision-making framework that includes:
1. Decision Classification
- Strategic decisions that affect the entire system architecture
- Tactical decisions that affect specific components or features
- Operational decisions that affect day-to-day development
2. Decision Criteria
For each decision, consider factors such as:
- Alignment with business objectives
- Technical constraints and requirements
- Team capabilities and experience
- Integration with existing systems
- Operational impact
- Long-term maintenance considerations
3. Decision Process
Define a clear process for making and documenting decisions:
- Who needs to be involved in different types of decisions
- How alternatives should be evaluated
- What documentation is required (e.g., ADRs)
- How decisions will be communicated to the broader team
- When and how decisions should be revisited
4. Learning Loop
Establish mechanisms for learning from past decisions:
- Regular architecture reviews
- Post-implementation retrospectives
- Technical debt tracking and prioritization
- Knowledge sharing sessions about system evolution
Conclusion: Building for the Long Term
Making technical decisions that stand the test of time isn’t about predicting the future perfectly. It’s about creating systems that can evolve gracefully as requirements change, technologies advance, and teams transform.
The principles outlined in this article—valuing simplicity, adopting proven technologies, designing for evolution, making reversible decisions, and documenting context—provide a foundation for more durable technical choices.
At AlgoCademy, we’ve observed that teams who consistently apply these principles not only build more maintainable systems but also deliver more value to their users over time. They spend less time fighting technical debt and more time creating innovative solutions to real problems.
Remember that even the best technical decisions have a limited lifespan. The goal isn’t to build systems that never change, but rather systems that can change in controlled, intentional ways as needs evolve.
By approaching technical decisions with both immediate needs and long-term sustainability in mind, you can create architecture that serves as a foundation for innovation rather than a barrier to it.
Key Takeaways
- Value simplicity over unnecessary complexity
- Be strategic about adopting new technologies
- Design systems that can evolve incrementally
- Make decisions reversible when possible
- Document not just what was decided, but why
- Consider different time horizons for different decisions
- Learn from examples of durable technical choices
- Establish a consistent decision-making framework
- Measure the durability of your decisions over time
By applying these principles, you can make technical decisions that not only solve today’s problems but continue providing value for years to come.