Why Your Solutions Work But Aren’t Maintainable: Building Code That Lasts
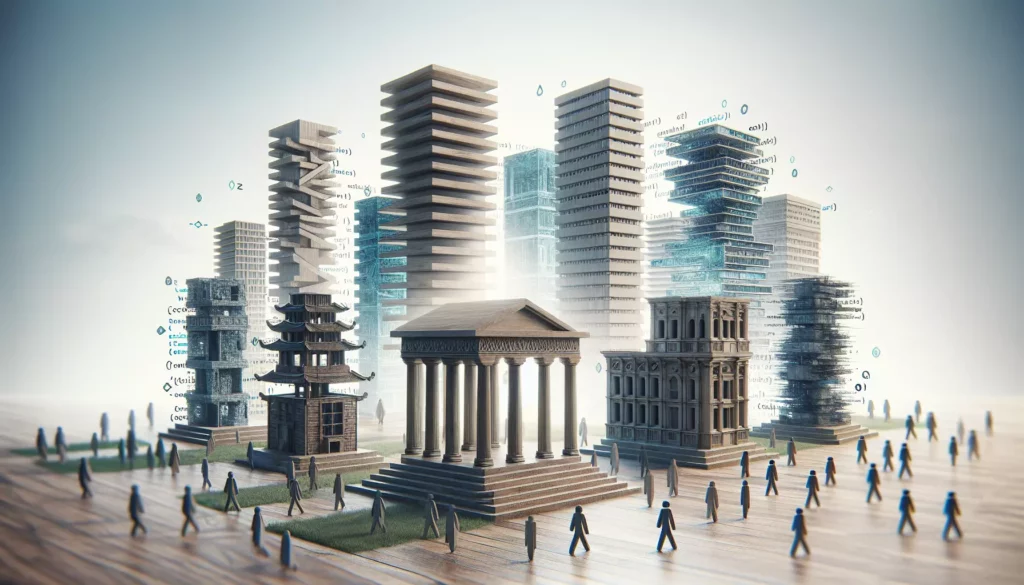
Writing code that works is only half the battle in software development. Many developers find themselves trapped in a cycle of creating solutions that function perfectly today but become maintenance nightmares tomorrow. This phenomenon is all too common, especially among those who are focused on getting the job done quickly without considering the long-term implications of their code structure.
At AlgoCademy, we see this pattern frequently with learners who can solve algorithmic problems but struggle to write code that remains viable in professional environments. The ability to craft maintainable code separates novice programmers from seasoned software engineers. In this comprehensive guide, we’ll explore why your solutions might work but lack maintainability, and how you can transform your coding approach for long-term success.
Understanding Code Maintainability
Before diving into specific issues, let’s establish what we mean by “maintainable code.” Maintainable code is software that can be easily understood, modified, and extended by developers who didn’t write the original implementation. It’s code that remains viable even as requirements change and systems evolve.
The True Cost of Unmaintainable Code
According to industry research, maintenance accounts for 60-80% of the total cost of software ownership. When code isn’t maintainable, organizations face:
- Increased time to implement new features
- Higher risk when making changes
- Greater difficulty onboarding new team members
- Technical debt that compounds over time
- Decreased team morale and productivity
Even if your code solves the immediate problem perfectly, these hidden costs can far outweigh the initial benefits of a quick solution.
Common Signs Your Code Lacks Maintainability
Let’s examine the telltale signs that your solutions, while functional, may be heading toward maintainability issues.
1. Excessive Function Length
One of the most common issues we see is functions that stretch for dozens or even hundreds of lines. Long functions typically try to accomplish too many things at once, making them difficult to understand, test, and modify.
Example of an unmaintainable function:
function processUserData(userData) {
// 100+ lines of code that:
// Validates user input
// Transforms data format
// Saves to database
// Sends confirmation email
// Updates analytics
// Logs activity
// Returns response
}
Functions this large violate the Single Responsibility Principle and become “black boxes” that other developers fear to touch.
2. Mysterious Variable Names
Variables named x
, temp
, or data
might make sense while you’re writing the code, but they become cryptic puzzles for future readers, including your future self.
function calc(a, b, c) {
let x = a * 2;
let y = b / 4;
let z = c % 3;
return x + y * z;
}
Compare this to descriptive naming:
function calculateFee(basePrice, quantity, discountCode) {
let baseFee = basePrice * 2;
let quantityDiscount = quantity / 4;
let promoValue = discountCode % 3;
return baseFee + quantityDiscount * promoValue;
}
3. Lack of Documentation
Code without comments or documentation forces every new developer to reverse-engineer your thinking. While self-documenting code is valuable, complex logic and business rules often need explicit explanation.
4. Duplicate Code
When the same logic appears in multiple places, changes require updates in multiple locations. This inevitably leads to inconsistencies when one instance gets updated while others are forgotten.
5. Deep Nesting
Deeply nested conditionals and loops create code that’s difficult to follow and prone to subtle bugs:
function processOrder(order) {
if (order) {
if (order.items) {
if (order.items.length > 0) {
for (let i = 0; i < order.items.length; i++) {
if (order.items[i].inStock) {
if (order.items[i].quantity > 0) {
// Actual business logic buried 6 levels deep
}
}
}
}
}
}
}
6. No Tests
Code without tests can’t be confidently modified. Without a safety net of automated tests, developers must manually verify that changes don’t break existing functionality, which is time-consuming and error-prone.
The Technical Interview Trap
Many programmers, especially those preparing for technical interviews at major tech companies, fall into a pattern of optimizing for immediate solutions rather than maintainability. This makes sense in the context of interviews, where you’re typically judged on:
- Solving problems quickly
- Optimal time and space complexity
- Correctness of the solution
Unfortunately, this mindset can carry over into professional work, where different priorities should apply. In real-world development, code that’s slightly less optimal but much more maintainable is often the better choice.
At AlgoCademy, we teach not just how to solve problems, but how to solve them in ways that create sustainable code bases. Let’s look at how to transform working but unmaintainable solutions into code that stands the test of time.
Principles of Maintainable Code
1. Single Responsibility Principle
Each function or class should have one reason to change. When components have a single responsibility, they’re easier to understand, test, and modify.
Before:
function handleUserRegistration(userData) {
// Validate email format
if (!userData.email.includes('@')) {
return { error: 'Invalid email format' };
}
// Check if user already exists
const existingUser = database.findUserByEmail(userData.email);
if (existingUser) {
return { error: 'Email already registered' };
}
// Hash password
const hashedPassword = hashFunction(userData.password);
// Create user record
const user = {
email: userData.email,
password: hashedPassword,
createdAt: new Date()
};
// Save to database
database.saveUser(user);
// Send welcome email
emailService.sendWelcomeEmail(userData.email);
// Log activity
logger.log('New user registered', userData.email);
return { success: true, userId: user.id };
}
After:
function validateUserData(userData) {
if (!userData.email.includes('@')) {
return { isValid: false, error: 'Invalid email format' };
}
// Other validations...
return { isValid: true };
}
function isEmailAlreadyRegistered(email) {
const existingUser = database.findUserByEmail(email);
return !!existingUser;
}
function createUserRecord(userData) {
const hashedPassword = hashFunction(userData.password);
return {
email: userData.email,
password: hashedPassword,
createdAt: new Date()
};
}
function handleUserRegistration(userData) {
const validation = validateUserData(userData);
if (!validation.isValid) {
return { error: validation.error };
}
if (isEmailAlreadyRegistered(userData.email)) {
return { error: 'Email already registered' };
}
const user = createUserRecord(userData);
database.saveUser(user);
emailService.sendWelcomeEmail(userData.email);
logger.log('New user registered', userData.email);
return { success: true, userId: user.id };
}
The refactored version separates concerns into distinct functions, making each part easier to understand, test, and potentially reuse.
2. DRY (Don’t Repeat Yourself)
Duplication is a maintainability killer. When logic is duplicated, changes require updates in multiple places, and inconsistencies inevitably arise.
Before (with duplication):
function calculateRegularUserDiscount(orderTotal) {
if (orderTotal >= 100) {
return orderTotal * 0.1; // 10% discount for orders over $100
}
return 0;
}
function calculatePremiumUserDiscount(orderTotal) {
if (orderTotal >= 100) {
return orderTotal * 0.1; // 10% discount for orders over $100
}
return orderTotal * 0.05; // 5% discount for all premium orders
}
After (DRY):
function calculateVolumeDiscount(orderTotal) {
if (orderTotal >= 100) {
return orderTotal * 0.1; // 10% discount for orders over $100
}
return 0;
}
function calculateRegularUserDiscount(orderTotal) {
return calculateVolumeDiscount(orderTotal);
}
function calculatePremiumUserDiscount(orderTotal) {
const volumeDiscount = calculateVolumeDiscount(orderTotal);
if (volumeDiscount > 0) {
return volumeDiscount;
}
return orderTotal * 0.05; // 5% discount for all premium orders
}
By extracting the common logic into a separate function, we ensure that any changes to the volume discount calculation only need to be made in one place.
3. KISS (Keep It Simple, Stupid)
Simple solutions are easier to understand and maintain. Resist the urge to over-engineer or prematurely optimize your code.
Overly complex solution:
function isPrime(num) {
// Edge cases for efficiency
if (num <= 1) return false;
if (num <= 3) return true;
if (num % 2 === 0 || num % 3 === 0) return false;
// Using an optimization where we only check divisors of form 6k±1
let i = 5;
while (i * i <= num) {
if (num % i === 0 || num % (i + 2) === 0) return false;
i += 6;
}
return true;
}
Simpler, more maintainable solution:
function isPrime(num) {
// Handle basic cases
if (num <= 1) return false;
if (num === 2) return true;
// Check for divisibility by numbers from 2 to sqrt(num)
const limit = Math.sqrt(num);
for (let i = 2; i <= limit; i++) {
if (num % i === 0) return false;
}
return true;
}
While the first solution is more optimized, the second is much easier to understand and maintain. Unless performance is critical for this specific function, the simpler solution is likely better.
4. Meaningful Names
Clear, descriptive names for variables, functions, and classes make code self-documenting and easier to understand.
// Poor naming
function process(arr, val) {
let res = [];
for (let i = 0; i < arr.length; i++) {
if (arr[i] > val) {
res.push(arr[i]);
}
}
return res;
}
// Better naming
function filterValuesGreaterThan(array, threshold) {
let filteredValues = [];
for (let i = 0; i < array.length; i++) {
if (array[i] > threshold) {
filteredValues.push(array[i]);
}
}
return filteredValues;
}
5. Consistent Formatting and Style
Consistent code formatting makes it easier for developers to read and understand each other's code. Use linters and formatters to enforce consistency across your codebase.
Practical Strategies for More Maintainable Code
Now that we understand the principles, let's look at specific strategies you can apply to improve code maintainability.
1. Break Down Complex Functions
When a function grows beyond 20-30 lines, it's often a sign that it's doing too much. Look for logical segments that can be extracted into helper functions.
Signs that a function should be broken down:
- It has multiple levels of indentation
- It handles multiple steps in a process
- You find yourself adding comments to separate sections
- It has multiple return points with different meanings
2. Use Design Patterns Appropriately
Design patterns provide tested solutions to common problems, but they should be used judiciously. Applying patterns unnecessarily can add complexity without benefits.
Some useful patterns to know:
- Factory Pattern: For creating objects without specifying the exact class
- Strategy Pattern: For selecting an algorithm at runtime
- Observer Pattern: For maintaining a list of dependents that get notified of changes
- Decorator Pattern: For adding responsibilities to objects dynamically
3. Write Self-Documenting Code
While comments are important, code that explains itself through clear structure and naming reduces the need for extensive documentation.
// Requires a comment to explain
// Check if user has admin access or is in the finance department
if (user.role === 'admin' || user.department === 'finance') {
// Allow access
}
// Self-documenting alternative
function userHasAccessToFinancialReports(user) {
return user.role === 'admin' || user.department === 'finance';
}
if (userHasAccessToFinancialReports(user)) {
// Allow access
}
4. Implement Automated Testing
Tests serve as both documentation and a safety net for changes. A comprehensive test suite gives developers confidence to refactor and extend code without fear of breaking existing functionality.
Types of tests to consider:
- Unit tests: Test individual functions and methods
- Integration tests: Test how components work together
- End-to-end tests: Test complete user flows
5. Use Version Control Effectively
Version control is not just for backup; it's a communication tool. Write meaningful commit messages that explain why changes were made, not just what was changed.
Good commit message example:
Fix calculation of user discount
The previous implementation incorrectly applied the volume discount
to premium users even when they qualified for a better membership discount.
This change ensures users always get the most favorable discount.
Fixes issue #123
6. Code Reviews With Maintainability Focus
When reviewing code (or having your code reviewed), explicitly consider maintainability alongside correctness. Questions to ask include:
- Would a new team member understand this code without explanation?
- Is there any duplication that could be extracted?
- Are functions and classes focused on single responsibilities?
- Are naming conventions clear and consistent?
- Is the code unnecessarily complex for the problem it solves?
Refactoring Unmaintainable Code
Sometimes you inherit code that already has maintainability issues. Here's a systematic approach to improving it:
1. Add Tests First
Before making any changes, add tests that verify the current behavior. This gives you confidence that your refactoring doesn't break existing functionality.
2. Identify Problem Areas
Look for code smells that indicate maintainability issues:
- Long methods or functions
- Duplicate code
- Large classes with low cohesion
- Complex conditional logic
- Inappropriate intimacy (classes that know too much about each other)
3. Refactor Incrementally
Make small, focused changes rather than massive rewrites. Each change should be testable and verifiable.
4. Improve Naming and Documentation
Often, simply improving names and adding strategic comments can dramatically improve maintainability without changing functionality.
5. Extract Reusable Components
Look for opportunities to create reusable functions or classes that encapsulate common functionality.
Real-World Example: From Interview Solution to Production Code
Let's examine how a typical interview-style solution evolves into maintainable production code.
The Problem: Finding Pairs with Target Sum
Given an array of integers and a target sum, find all pairs of numbers that add up to the target.
Interview Solution
function findPairs(arr, target) {
const result = [];
const seen = {};
for (let i = 0; i < arr.length; i++) {
const complement = target - arr[i];
if (seen[complement]) {
result.push([arr[i], complement]);
}
seen[arr[i]] = true;
}
return result;
}
This solution works correctly and has O(n) time complexity, which would likely impress in an interview. However, it has several maintainability issues:
- Cryptic variable names (
arr
,seen
) - No input validation
- No documentation
- Unclear handling of edge cases
Production-Ready Solution
/**
* Finds all pairs of numbers in an array that sum to a target value.
* Each pair is included only once, regardless of how many times its elements
* appear in the input array.
*
* @param {number[]} numbers - The array of numbers to search through
* @param {number} targetSum - The target sum to find
* @returns {Array} - Array of pairs that sum to the target
* @throws {Error} - If the input is invalid
*/
function findNumberPairsWithSum(numbers, targetSum) {
// Validate inputs
if (!Array.isArray(numbers)) {
throw new Error('First argument must be an array');
}
if (typeof targetSum !== 'number') {
throw new Error('Target sum must be a number');
}
const result = [];
const seenNumbers = new Map();
const addedPairs = new Set();
for (const currentNumber of numbers) {
if (typeof currentNumber !== 'number') {
continue; // Skip non-numeric values
}
const complementNumber = targetSum - currentNumber;
// If we've seen the complement before, we found a pair
if (seenNumbers.has(complementNumber)) {
// Create a unique key for this pair to avoid duplicates
const pairKey = [Math.min(currentNumber, complementNumber),
Math.max(currentNumber, complementNumber)].toString();
if (!addedPairs.has(pairKey)) {
result.push([currentNumber, complementNumber]);
addedPairs.add(pairKey);
}
}
// Mark this number as seen
seenNumbers.set(currentNumber, true);
}
return result;
}
// Example usage
try {
const numbers = [3, 4, 5, 6, 7];
const targetSum = 10;
const pairs = findNumberPairsWithSum(numbers, targetSum);
console.log(`Pairs that sum to ${targetSum}:`, pairs);
} catch (error) {
console.error('Error finding pairs:', error.message);
}
The production version addresses maintainability concerns by:
- Using descriptive variable and function names
- Adding comprehensive documentation
- Including input validation
- Handling edge cases (non-numeric values, duplicate pairs)
- Using modern JavaScript constructs (for...of, Map, Set)
- Including error handling
- Providing example usage
Balancing Immediate Needs with Long-Term Maintainability
While maintainable code is the goal, real-world constraints sometimes require pragmatic compromises. Here's how to balance immediate needs with long-term maintainability:
1. Consider the Lifespan of the Code
One-off scripts or prototypes may not warrant the same level of maintainability investment as core business logic that will be used for years.
2. Document Technical Debt
When you must take shortcuts, document them as technical debt. Use comments or tickets to highlight areas that need future improvement.
// TODO: This implementation is inefficient for large datasets.
// Consider replacing with a more optimized algorithm if performance becomes an issue.
function searchLargeDataset(data, query) {
// Simple but potentially inefficient implementation
return data.filter(item => item.includes(query));
}
3. Use the Boy Scout Rule
"Leave the code better than you found it." Make small maintainability improvements whenever you touch existing code, even if you can't completely refactor it.
4. Prioritize Critical Areas
Focus maintainability efforts on code that:
- Changes frequently
- Has a high cost of failure
- Is central to your business logic
- Is worked on by multiple developers
Maintainability in Different Programming Paradigms
Different programming paradigms offer various approaches to maintainability:
Object-Oriented Programming
OOP improves maintainability through:
- Encapsulation: Hiding implementation details
- Inheritance: Reusing code through class hierarchies
- Polymorphism: Allowing different implementations of the same interface
However, deep inheritance hierarchies can reduce maintainability. Prefer composition over inheritance when possible.
Functional Programming
Functional programming enhances maintainability through:
- Pure functions: Functions without side effects
- Immutability: Data that doesn't change after creation
- Function composition: Building complex functions from simple ones
These characteristics make code more predictable and easier to test.
Procedural Programming
Even in procedural code, maintainability can be improved by:
- Grouping related functions together
- Using consistent naming conventions
- Limiting global state
- Creating clear module boundaries
Tools and Practices That Support Maintainability
Several tools and practices can help teams maintain code quality:
1. Static Analysis Tools
Tools like ESLint, SonarQube, and TypeScript can catch potential issues before they make it into production.
2. Code Formatting Tools
Formatters like Prettier ensure consistent code style without manual effort.
3. Continuous Integration
CI pipelines can run tests, linters, and other quality checks automatically on every commit.
4. Documentation Generation
Tools like JSDoc can generate documentation from code comments, encouraging developers to keep documentation up-to-date.
5. Code Review Checklists
Standardized review checklists help teams consistently evaluate code for maintainability issues.
Conclusion: Beyond Working Code
At AlgoCademy, we believe that the journey from coding interview success to professional excellence requires a shift in mindset. While your solutions may work perfectly in an interview or classroom setting, professional software development demands code that can be maintained and extended over time.
The principles and practices outlined in this guide aren't just academic exercises; they're essential skills for any developer who wants to create lasting value. By focusing on maintainability alongside functionality, you'll write code that:
- Continues to deliver value as requirements change
- Enables your team to work efficiently and confidently
- Reduces the total cost of ownership for your software
- Demonstrates your growth from a coder to a software engineer
Remember that maintainable code isn't about perfection; it's about making deliberate choices that balance immediate needs with long-term viability. By consistently applying these principles, your code won't just work today; it will continue to work well into the future.
As you continue your learning journey with AlgoCademy, we encourage you to practice these maintainability principles in every coding challenge you tackle. The habits you form now will shape your success as a professional developer.